C++运算符重载
分类 运算符重载:赋予运算符具有操作自定义类型功能 类重载:重载函数是类的成员函数 友元重载:重载函数是友元函数 注意:重载适用于自定义类型,实质是调用函数的过程。 形式:
函数返回值 函数名 (参数){
函数体
}
函数名:opertor + 运算符 组成函数名
参数个数等于操作数,操作数即用户需要几个对象进行运算操作数就为多少。
举个例子为什么要重载,如下面代码,针对自定义类型Score想要实现stu1+stu2加法,由于自定义类型不能之间使用系统运算符,因此引入了运算符重载。
#include<iostream>
using namespace std;
struct Score{
int math;
int chinese;
};
int main(){
Score stu1{123,121};
Score stu2{111,121};
Score stu3 = stu1+stu2;
}
上述代码报错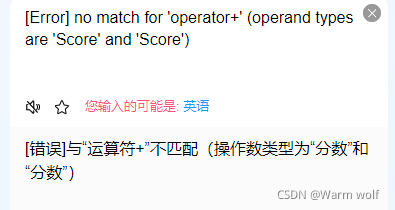
只需在程序中加上重载函数即可实现自定义类型Score的stu1+stu2;
Score operator+(Score stu1,Score stu2) {
Score p;
p.chinese= stu1.chinese+stu2.chinese;
p.math = stu1.math+stu2.math;
return p;
}
注:结构体的重载写法想当于类中友元重载的写法。
- 友元重载
函数语句 friend 自定义类型名称 operator重载运算符(参数){ 想要实现的运算表达式 }
#include<iostream>
using namespace std;
class Student{
public:
Student(){
}
Student(string str,int n)
:name(str),age(n){
}
void print(){
cout<<"我的名字是:"<<name<<endl;
cout<<"我的年纪是:"<<age<<endl;
}
friend Student operator+(Student s1,Student s2){
Student s3;
s3.name = s1.name+s2.name;
s3.age = s1.age+s2.age;
return s3;
}
protected:
string name;
int age;
};
int main(){
Student stu1={"李华",23};
Student stu2={"徐磊",12};
Student stu34;
stu1.print();
stu2.print();
stu34= stu2+stu1;
stu34.print();
}
运行截图 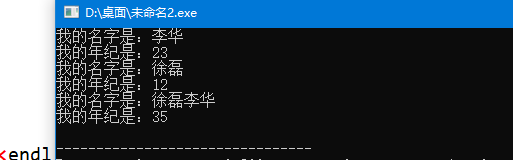
- 类的成员函数重载
重载过程解析:参考上面的类名Student,.stu1+stu2 解析为 stu1.operator+(stu2)。若采用类的成员函数重载方式,函数语句如下: 函数语句 自定义类型名称 operator重载运算符(参数){ 想要实现的运算表达式。 } 不同于友元函数的是参数个数等于操作数减1.因为自身类就可以代表一个参数,用this指整指向即可。
Student operator+(Student s2){
Student s3;
s3.name = this->name+s2.name;
s3.age = this->age+s2.age;
return s3;
}
特殊的重载方式: 成员函数重载时,当重载运算符遇到普通数据 Student student = stu1+1;
Student student = 1+stu1;
大伙儿可以思考一下:上面那种表示会报错了? 为什么? 当有多个操作数重载时两种方式都正确吗?
- 流重载(输入、输出重载<< >>)
流:数据从一端流到另外一端 流对象: cin输入流(istream)对象、cout输出流(ostream)对象 注:流重载只能通过友元函数重载,其返回值必须是流对象的引用。
上述类中自我介绍需要以下函数
cout<<"我的名字是:"<<name<<endl;
cout<<"我的年纪是:"<<age<<endl;
流重载想要实现的就是当输入 cout<<stu1时直接能将上述语句要实现的功能实现,具体语法如下:
friend 流对象的引用 operator重载运算符(流对象的引用,自定义类型的引用)
#include<iostream>
using namespace std;
class Student{
public:
Student(){
}
Student(string str,int n)
:name(str),age(n){
}
friend ostream& operator<<(ostream& out,Student stu){
out<<"我的名字是:"<<stu.name<<endl;
out<<"我的年龄是:"<<stu.age<<endl;
}
Student operator+(Student s2){
Student s3;
s3.name = this->name+s2.name;
s3.age = this->age+s2.age;
return s3;
}
protected:
string name;
int age;
};
int main(){
Student stu1={"李华",23};
Student stu2={"徐磊",12};
Student stu34;
cout<<stu1;
cout<<stu2;
stu34= stu2+stu1;
cout<<stu34;
}
再封装的写法:友元+成员函数的写法(将操作数据的部分用成员函数封装) 其实就是将原有的流重载函数语句//此时out就当做cout使用 out<<“我的名字是:”<<stu.name<<endl; out<<“我的年龄是:”<<stu.age<<endl; 改成调用成员函数,
void out(ostream& out){
out<<"我的名字是:"<<this->name<<endl;
out<<"我的年龄是:"<<this->age<<endl;
}
friend ostream& operator<<(ostream& out,Student stu){
stu.out(out);
return out;
}
- c++中不能被重载的运算符
. (成员访问运算符)
.* (成员指针访问运算符)
:: (域运算符)
sizeof (长度运算符)
?: (条件运算符)
= () [ ] ->不能重载为友元函数
单目运算符最好重载为类的成员函数
双目运算符最好重载成类的友元函数
重载时不能改变C++的优先级、操作数至少有一个是自定义类型。
如有错误还请各位博友指正!!!!谢谢
|