界面
主要控件有
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.Timer timer_load;
private System.Windows.Forms.Timer timer_gameover;
private System.Windows.Forms.MenuStrip menuStrip1;
private System.Windows.Forms.ToolStripMenuItem 游戏ToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem 新游戏ToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem 信息统计ToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem 选项ToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem 退出ToolStripMenuItem;
运行时界面: 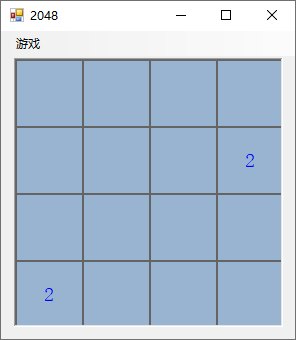
代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _2048
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
this.CenterToScreen();
}
private void Form1_Load(object sender, EventArgs e)
{
this.timer_load.Interval = 100;
this.timer_gameover.Interval = 100;
timer_load_Tick(null, null);
}
private void timer_load_Tick(object sender, EventArgs e)
{
this.timer_load.Stop();
this.game.Init(4);
this.game.Generate();
CreateLabel();
ShowMap();
}
private void timer_gameover_Tick(object sender, EventArgs e)
{
this.timer_gameover.Stop();
MessageBox.Show("game over");
}
private void 新游戏ToolStripMenuItem_Click(object sender, EventArgs e)
{
this.timer_load.Start();
}
private void 信息统计ToolStripMenuItem_Click(object sender, EventArgs e)
{
}
private void 选项ToolStripMenuItem_Click(object sender, EventArgs e)
{
}
private void 退出ToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
private void Form1_KeyDown(object sender, KeyEventArgs e)
{
if (this.game.IsGameOver()) return;
if (Keys.Up == e.KeyCode)
{
this.game.Operate(CGame.eOpt.Up);
}
else if (Keys.Down == e.KeyCode)
{
this.game.Operate(CGame.eOpt.Down);
}
else if (Keys.Left == e.KeyCode)
{
this.game.Operate(CGame.eOpt.Left);
}
else if (Keys.Right == e.KeyCode)
{
this.game.Operate(CGame.eOpt.Right);
}
this.game.Generate();
ShowMap();
if (this.game.IsGameOver())
{
this.timer_gameover.Start();
}
}
public void CreateLabel()
{
int w = this.game.GetSizeWidth();
int h = this.game.GetSizeHeight();
if (0 == w || 0 == h) return;
int cubeSize = Math.Min(this.panel1.Size.Width / w, this.panel1.Size.Height / h);
Point pt = new Point();
Font ft = null;
Size sz = new Size();
sz.Width = cubeSize;
sz.Height = cubeSize;
if (0 < w)
{
pt.Y = 0;
for (int i = 0; i < w; ++i)
{
pt.X = h * cubeSize;
for (int j = 0; j < h; ++j)
{
Label labelCube = new Label();
labelCube.Name = "label_" + i.ToString() + "_" + j.ToString();
labelCube.Tag = i.ToString() + "," + j.ToString();
labelCube.BorderStyle = BorderStyle.FixedSingle;
labelCube.TextAlign = ContentAlignment.MiddleCenter;
pt.X = j * cubeSize;
pt.Y = i * cubeSize;
labelCube.Location = pt;
labelCube.Size = sz;
if (null == ft)
{
ft = new Font(labelCube.Font.Name, 14, labelCube.Font.Style);
}
labelCube.Font = ft;
labelCube.BackColor = SystemColors.ActiveCaption;
this.panel1.Controls.Add(labelCube);
}
pt.Y += cubeSize;
}
}
}
public void ShowMap()
{
int r = this.game.GetSizeWidth();
int c = this.game.GetSizeHeight();
for (int i = 0; i < r; ++i)
{
for (int j = 0; j < c; ++j)
{
UpdateCube(i, j);
}
}
}
public void UpdateCube(int row, int col)
{
if (null == game) return;
int count = this.panel1.Controls.Count;
if (row >= this.game.GetSizeWidth() || col >= this.game.GetSizeHeight()) return;
string name = "label_" + row.ToString() + "_" + col.ToString();
object obj = this.panel1.Controls.Find(name, false).First();
if (null != obj)
{
Label lab = obj as Label;
ShowCube(lab, this.game.GetCube(row, col));
}
}
public void ShowCube(Label labelCube, CCube c)
{
if (null == labelCube) return;
if (null == c) return;
labelCube.Text = (0 == c.GetValue()) ? "" : (Math.Pow(2, c.GetValue())).ToString();
switch (c.GetValue())
{
case 1: labelCube.ForeColor = Color.Blue; break;
case 2: labelCube.ForeColor = Color.Green; break;
case 3: labelCube.ForeColor = Color.OrangeRed; break;
case 4: labelCube.ForeColor = Color.DarkBlue; break;
case 5: labelCube.ForeColor = Color.DarkOliveGreen; break;
case 6: labelCube.ForeColor = Color.BlueViolet; break;
case 7: labelCube.ForeColor = Color.DarkOrange; break;
case 8: labelCube.ForeColor = Color.PaleVioletRed; break;
case 9: labelCube.ForeColor = Color.Brown; break;
case 10: labelCube.ForeColor = Color.DeepPink; break;
case 11: labelCube.ForeColor = Color.Crimson; break;
case 12: labelCube.ForeColor = Color.DarkOrchid; break;
case 13: labelCube.ForeColor = Color.DarkRed; break;
case 14: labelCube.ForeColor = Color.MediumBlue; break;
case 15: labelCube.ForeColor = Color.DarkViolet; break;
case 16: labelCube.ForeColor = Color.Gold; break;
default: labelCube.ForeColor = Color.Black; break;
}
}
private CGame game = new CGame();
}
public partial class CCube
{
private int level;
public CCube()
{
this.level = 0;
}
public void Clear()
{
this.level = 0;
}
public int GetValue()
{
return this.level;
}
public void SetValue(int value)
{
if (0 > value) return;
this.level = value;
}
public void swap(ref CCube rhs)
{
int value = this.level;
this.level = rhs.level;
rhs.level = value;
}
}
public partial class CGame
{
public enum eOpt
{
Left,
Right,
Up,
Down,
}
public CGame()
{
Clear();
}
public void Init(int size)
{
Init(size, size);
}
public void Init(int row, int col)
{
if (null == this.map
|| row != this.map.GetLength(0) || col != this.map.GetLength(1))
{
this.map = new CCube[row, col];
this.row = row;
this.col = col;
}
for (int i = 0; i < row; ++i)
{
for (int j = 0; j < col; ++j)
{
this.map[i, j] = new CCube();
}
}
Clear();
this.isGameOver = false;
}
public int GetSizeWidth() { return this.row; }
public int GetSizeHeight() { return this.col; }
public void Clear()
{
if (null == this.map) return;
int r = this.map.GetLength(0);
int c = this.map.GetLength(1);
for (int i = 0; i < r; ++i)
{
for (int j = 0; j < c; ++j)
{
this.map[i, j].Clear();
}
}
this.isGameOver = true;
}
public bool IsGameOver() { return this.isGameOver; }
public CCube GetCube(int row, int col)
{
if (0 > row || row >= this.row) return null;
if (0 > col || col >= this.col) return null;
if (null == this.map) return null;
return map[row, col];
}
private void SetValue(int row, int col, int value)
{
if (0 > row || row >= this.row) return;
if (0 > col || col >= this.col) return;
if (null == this.map) return;
this.map[row, col].SetValue(value);
}
public void Generate()
{
Generate(2);
}
public void Generate(int count)
{
if (null == this.map) return;
Random rd = new Random();
int _count = 0;
for (int k = 0; k < count; ++k)
{
int r = rd.Next(this.row);
int c = rd.Next(this.col);
bool bFound = false;
for (int i = 0; i < this.row; ++i)
{
for (int j = 0; j < this.col; ++j)
{
int _r = (r + i) % this.row;
int _c = (c + j) % this.col;
if (0 == this.GetCube(_r, _c).GetValue())
{
bFound = true;
++_count;
SetValue(_r, _c, 1);
break;
}
}
if (bFound)
{
break;
}
}
}
if (0 >= _count)
{
this.isGameOver = true;
}
}
public void Operate(eOpt opt)
{
switch (opt)
{
case eOpt.Up:
{
OperateUp();
}
break;
case eOpt.Down:
{
OperateDown();
}
break;
case eOpt.Left:
{
OperateLeft();
}
break;
case eOpt.Right:
{
OperateRight();
}
break;
default:
break;
}
foreach (CCube c in this.map)
{
if (11 == c.GetValue())
{
this.isGameOver = true;
break;
}
}
}
private void OperateUp()
{
for (int j = 0; j < this.col; ++j)
{
for (int i = 1; i < this.row; ++i)
{
DealCube_Up(i, j);
}
}
}
private void OperateDown()
{
for (int j = 0; j < this.col; ++j)
{
for (int i = this.row - 2; 0 <= i; --i)
{
DealCube_Down(i, j);
}
}
}
private void OperateLeft()
{
for (int i = 0; i < this.row; ++i)
{
for (int j = 1; j < this.col; ++j)
{
DealCube_Left(i, j);
}
}
}
private void OperateRight()
{
for (int i = 0; i < this.row; ++i)
{
for (int j = this.col - 1; 0 <= j; --j)
{
DealCube_Right(i, j);
}
}
}
private void DealCube_Up(int row, int col)
{
if (0 != this.map[row, col].GetValue())
{
int index = row - 1;
if (0 <= index)
{
if (this.map[row, col].GetValue() == this.map[index, col].GetValue())
{
this.map[index, col].SetValue(this.map[index, col].GetValue() + 1);
this.map[row, col].Clear();
}
else if (0 == this.map[index, col].GetValue())
{
this.map[row, col].swap(ref this.map[index, col]);
DealCube_Up(index, col);
}
}
}
}
private void DealCube_Down(int row, int col)
{
if (0 != this.map[row, col].GetValue())
{
int index = row + 1;
if (this.row > index)
{
if (this.map[row, col].GetValue() == this.map[index, col].GetValue())
{
this.map[index, col].SetValue(this.map[index, col].GetValue() + 1);
this.map[row, col].Clear();
}
else if (0 == this.map[index, col].GetValue())
{
this.map[row, col].swap(ref this.map[index, col]);
DealCube_Down(index, col);
}
}
}
}
private void DealCube_Left(int row, int col)
{
if (0 != this.map[row, col].GetValue())
{
int index = col - 1;
if (0 <= index)
{
if (this.map[row, col].GetValue() == this.map[row, index].GetValue())
{
this.map[row, index].SetValue(this.map[row, index].GetValue() + 1);
this.map[row, col].Clear();
}
else if (0 == this.map[row, index].GetValue())
{
this.map[row, col].swap(ref this.map[row, index]);
DealCube_Left(row, index);
}
}
}
}
private void DealCube_Right(int row, int col)
{
if (0 != this.map[row, col].GetValue())
{
int index = col + 1;
if (this.col > index)
{
if (this.map[row, col].GetValue() == this.map[row, index].GetValue())
{
this.map[row, index].SetValue(this.map[row, index].GetValue() + 1);
this.map[row, col].Clear();
}
else if (0 == this.map[row, index].GetValue())
{
this.map[row, col].swap(ref this.map[row, index]);
DealCube_Right(row, index);
}
}
}
}
private bool isGameOver = true;
private int row;
private int col;
private CCube[,] map = null;
}
}
|