<<数据结构>>算法实现与解析——2.3
线性表的链式表示和实现
面向对象面向君,不负代码不负卿!
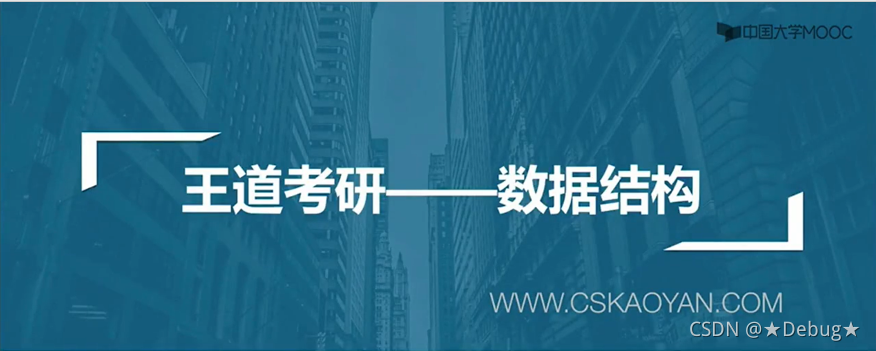
直接上代码!来吧,展示! 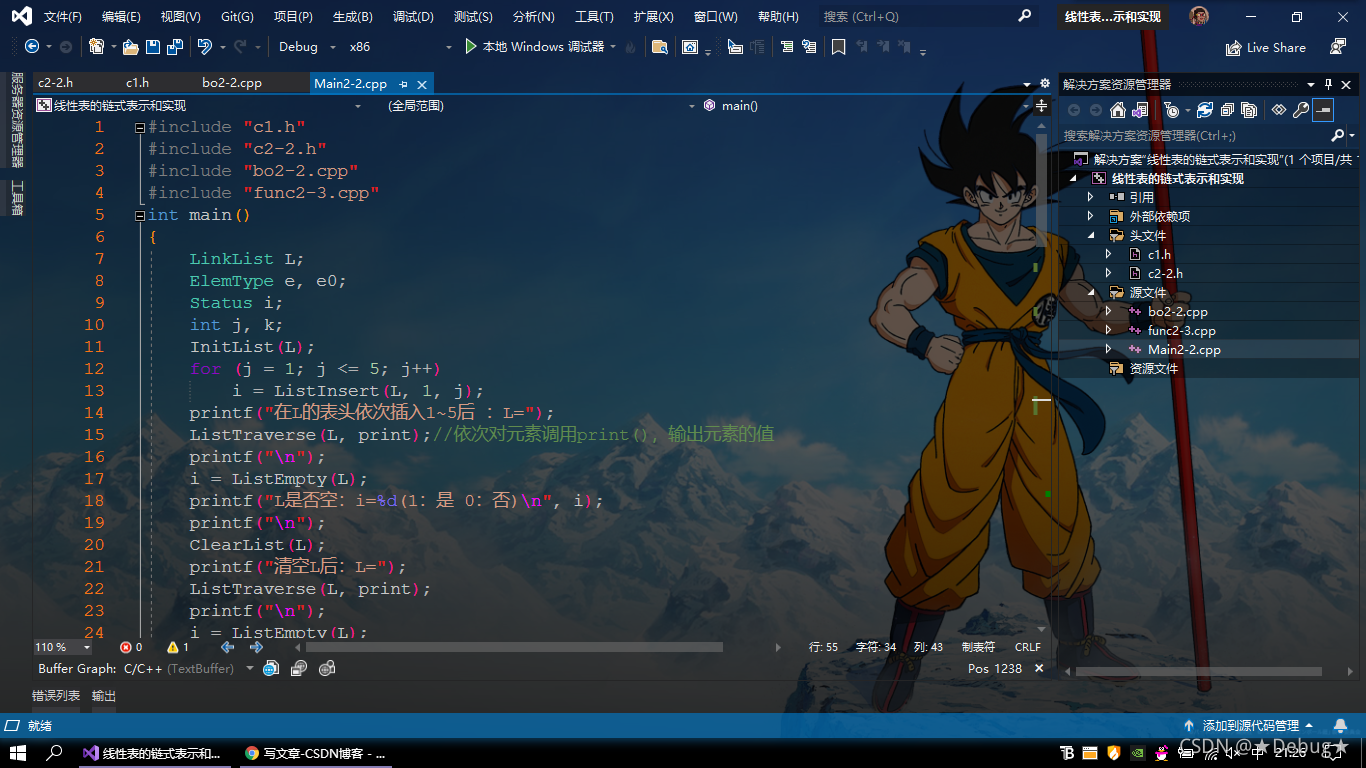 相关头文件!
#include "c1.h"
#pragma once
#pragma once
#include <string.h>
#include <ctype.h>
#include <malloc.h>
#include <limits.h>
#include <stdio.h>
#include <stdlib.h>
#include <io.h>
#include <math.h>
#include <process.h>
#include <iostream>
using namespace std;
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
typedef int Status;
typedef int Boolean;
typedef int ElemType;
#include "c2-2.h"
#pragma once
#include "c1.h"
#include "c2-2.h"
typedef struct LNode
{
ElemType data;
struct LNode* next;
}LNode,*LinkList;
函数的实现
#include "bo2-2.cpp"
#include "c1.h"
#include "c2-2.h"
inline void InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (!L)
exit(OVERFLOW);
L->next = NULL;
}
inline void DestroyList(LinkList& L)
{
LinkList q;
while (L)
{
q = L->next;
free(L);
L = q;
}
}
inline void ClearList(LinkList& L)
{
LinkList p, q;
p = L->next;
while (p)
{
q = p->next;
free(p);
p = q;
}
L->next = NULL;
}
inline Status ListEmpty(LinkList L)
{
if (L->next)
return FALSE;
else
return TRUE;
}
inline int ListLength(LinkList L)
{
int i = 0;
LinkList p = L->next;
while (p)
{
i++;
p = p->next;
}
return i;
}
inline Status GetElem(LinkList L, int i, ElemType& e)
{
int j = 1;
LinkList p = L->next;
while (p && j < i)
{
p = p->next;
j++;
}
if (!p || j > i)
return ERROR;
e = p->data;
return OK;
}
inline int LocateElem(LinkList L, ElemType e, Status(*compare)(ElemType, ElemType))
{
int i = 0;
LinkList p = L->next;
while (p)
{
i++;
if (compare(p->data, e))
return i;
p = p->next;
}
return 0;
}
inline Status PriorElem(LinkList L, ElemType cur_e, ElemType& pre_e)
{
LinkList q, p = L->next;
while (p->next)
{
q = p->next;
if (q->data == cur_e)
{
pre_e = p->data;
return OK;
}
p = q;
}
return INFEASIBLE;
}
inline Status NextElem(LinkList L, ElemType cur_e, ElemType& next_e)
{
LinkList p = L->next;
while (p->next)
{
if (p->data == cur_e)
{
next_e = p->next->data;
return OK;
}
p = p->next;
}
return INFEASIBLE;
}
inline Status ListInsert(LinkList L, int i, ElemType e)
{
int j = 0;
LinkList p = L, s;
while (p && j < i - 1)
{
p = p->next;
j++;
}
if (!p || j > i-1)
return ERROR;
s = (LinkList)malloc(sizeof(LNode));
s->data = e;
s->next = p->next;
p->next = s;
return OK;
}
inline Status ListDelete(LinkList L, int i, ElemType& e)
{
int j = 0;
LinkList p = L, q;
while (p->next && j < i - 1)
{
p = p->next;
j++;
}
if (!p->next || j > i - 1)
return ERROR;
q = p->next;
p->next = q->next;
e = q->data;
free(q);
return OK;
}
inline void ListTraverse(LinkList L, void(*vi)(ElemType))
{
LinkList p = L->next;
while(p)
{
vi(p->data);
p = p->next;
}
printf("\n");
}
#include "fun2-3.cpp"
#include "c1.h"
#include "c2-2.h"
inline Status equal(ElemType c1, ElemType c2)
{
if (c1 == c2)
return TRUE;
else
return FALSE;
}
inline int comp(ElemType a, ElemType b)
{
if (a == b)
return 0;
else
return (a - b) / abs(a + b);
}
inline void print(ElemType c)
{
printf("%d ", c);
}
inline void print2(ElemType c)
{
printf("%c ", c);
}
inline void print1(ElemType& c)
{
printf("%d ", c);
}
程序的入口-主函数
#include "Main2-2.cpp"
#include "c1.h"
#include "c2-2.h"
#include "bo2-2.cpp"
#include "func2-3.cpp"
int main()
{
LinkList L;
ElemType e, e0;
Status i;
int j, k;
InitList(L);
for (j = 1; j <= 5; j++)
i = ListInsert(L, 1, j);
printf("在L的表头依次插入1~5后 :L=");
ListTraverse(L, print);
printf("\n");
i = ListEmpty(L);
printf("L是否空:i=%d(1:是 0:否)\n", i);
printf("\n");
ClearList(L);
printf("清空L后:L=");
ListTraverse(L, print);
printf("\n");
i = ListEmpty(L);
printf("L是否空:i=%d(1:是 0:否)\n", i);
printf("\n");
for (j = 1; j <= 10; j++)
ListInsert(L, j, j);
printf("在L的表尾依次插入1~10后:L=");
ListTraverse(L, print);
printf("\n");
GetElem(L, 5, e);
printf("第5个元素的值为%d\n", e);
printf("\n");
for (j = 0; j <= 1; j++)
{
k = LocateElem(L, j, equal);
if (k)
printf("第%d个元素的值为%d\n\n", k, j);
else
printf("没有值为%d的元素\n\n", j);
}
for (j = 1; j <= 2; j++)
{
GetElem(L, j, e0);
i = PriorElem(L, e0, e);
if (i == INFEASIBLE)
printf("元素%d无前驱\n\n", e0);
else
printf("元素%d的前驱为%d\n\n", e0, e);
}
for (j = ListLength(L) - 1; j <= ListLength(L); j++)
{
GetElem(L, j, e0);
i = NextElem(L, e0, e);
if (i == INFEASIBLE)
printf("元素%d无后继\n\n", e0);
else
printf("元素%d的后继为%d\n\n", e0, e);
}
k = ListLength(L);
for (j = k + 1; j >= k; j--)
{
i = ListDelete(L, j, e);
if (i == ERROR)
printf("删除第%d个元素失败\n\n", j);
else
printf("删除第%d个元素成功,其值为%d\n\n", j, e);
}
printf("依次输出L的元素:");
ListTraverse(L, print);
DestroyList(L);
printf("\n");
printf("销毁L后:L=%u\n", L);
return 0;
}
程序运行结果图!
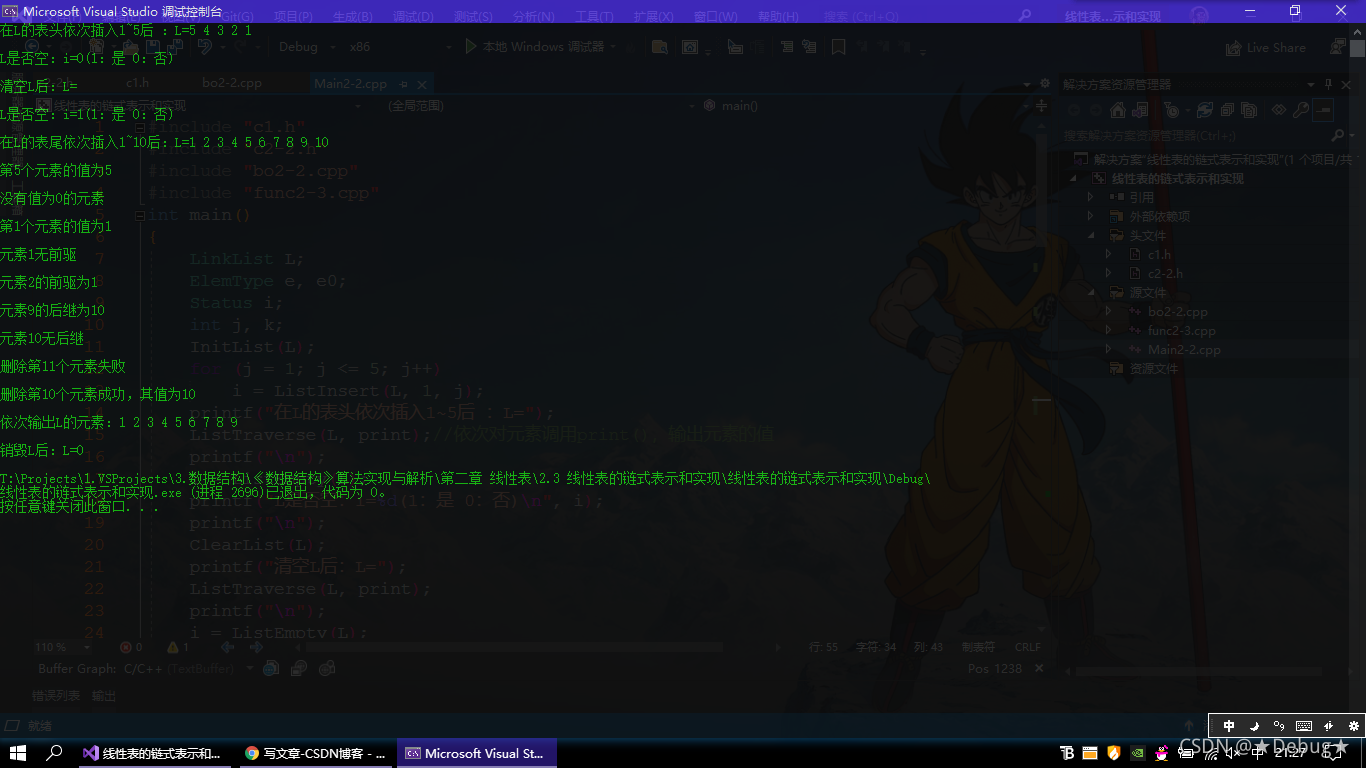
面向对象面向君,不负代码不负卿!
|