1. STL容器——string
1.1 标准库中的string类是什么?
- string是表示字符串的字符串类
- 该类的接口与常规容器的接口基本相同,再添加了一些专门用来操作string的常规操作。
- string在底层实际是:basic_string模板类的别名,typedef basic_string<char, char_traits, allocator>
string; - 不能操作多字节或者变长字符的序列。
1.2 string有哪些成员函数?
C++官网中关于string类的文档介绍
1.3 string类常用的成员函数使用方法?
- 构造、拷贝构造
void Test()
{
string s1;
string s2("hello bit");
string s3(s2);
}
- string类对象容量操作
void Test1()
{
string s("hello world!!!");
cout << s.size() << endl;
cout << s.length() << endl;
cout << s.capacity() << endl;
s.resize(20,'a');
s.resize(3);
s.reserve(50);
s.clear();
s.empty();
}
- string类对象的访问和遍历操作
void Test2()
{
string s("hello world!");
cout << s[2] << endl;
for(size_t i=0;i<s.size(),++i)
{
cout << s[i] << endl;
}
string::iterator it = s.begin();
while(it != s.end())
{
cout << *it;
it++;
}
for(auto c : s)
{
cout << c;
}
}
- string类对象的修改操作
void Test3()
{
string s("hello world!");
s.push_back(' ');
s.append("abc");
s += 'd';
s += "efg";
cout << s.c_str() << endl;
cout << s.find('o', 6) << endl;
cout << s.rfind(' ', 8) << endl;
s.erase(0 , 4);
string s1("123456789");
s1.swap(s);
}
- string类常用的非成员函数
void Test4()
{
string s("hello world!");
cout << s << endl;
cin >> s;
getline(cin,s);
string s1("hello");
cout << (s > s1) << (s <= s1) <<end;
}
2. 模拟实现string
2.1 模拟实现一些常用的接口,声明这些成员函数
string.h
namespace zhy{
class string
{
public:
static const size_t npos;
typedef char* iterator;
string(const char* str = "");
string(const string& s);
string& operator=(const string& s);
~string();
size_t size();
size_t capacity();
bool empty();
void reserve(size_t capcity);
void resize(size_t n, char c = '\0');
void clean();
char operator[](size_t i);
iterator begin();
iterator end();
void push_back(char c);
void append(const char* str);
void operator+=(char c);
void operator+=(const char* s);
void operator+=(const string& str);
size_t find(char c, size_t pos = 0);
size_t find(const char* s, size_t pos = 0);
void insert(size_t pos, char c);
void insert(size_t pos, char* s);
void erase(size_t pos, size_t len = npos);
void swap(string& temp);
const char* c_str();
bool operator<(const string& s);
bool operator<=(const string& s);
bool operator>(const string& s);
bool operator>=(const string& s);
bool operator==(const string& s);
bool operator!=(const string& s);
private:
char* _str;
size_t _size;
size_t _capacity;
};
ostream& operator<<(ostream& out, zhy::string& s);
istream& operator>>(istream& in, zhy::string& s);
}
上述这些成员函数和非成员函数是string中比较常用的,下面来用代码简单实现他们
2.2 代码实现string常用的成员函数
这里的构造函数必须我们自己实现,因为默认生成的构造函数只实现了简单的值拷贝(浅拷贝),在对象扩容或修改内容时会出现问题,下面看看浅拷贝与深拷贝的区别,以及深拷贝如何解决存在的问题: 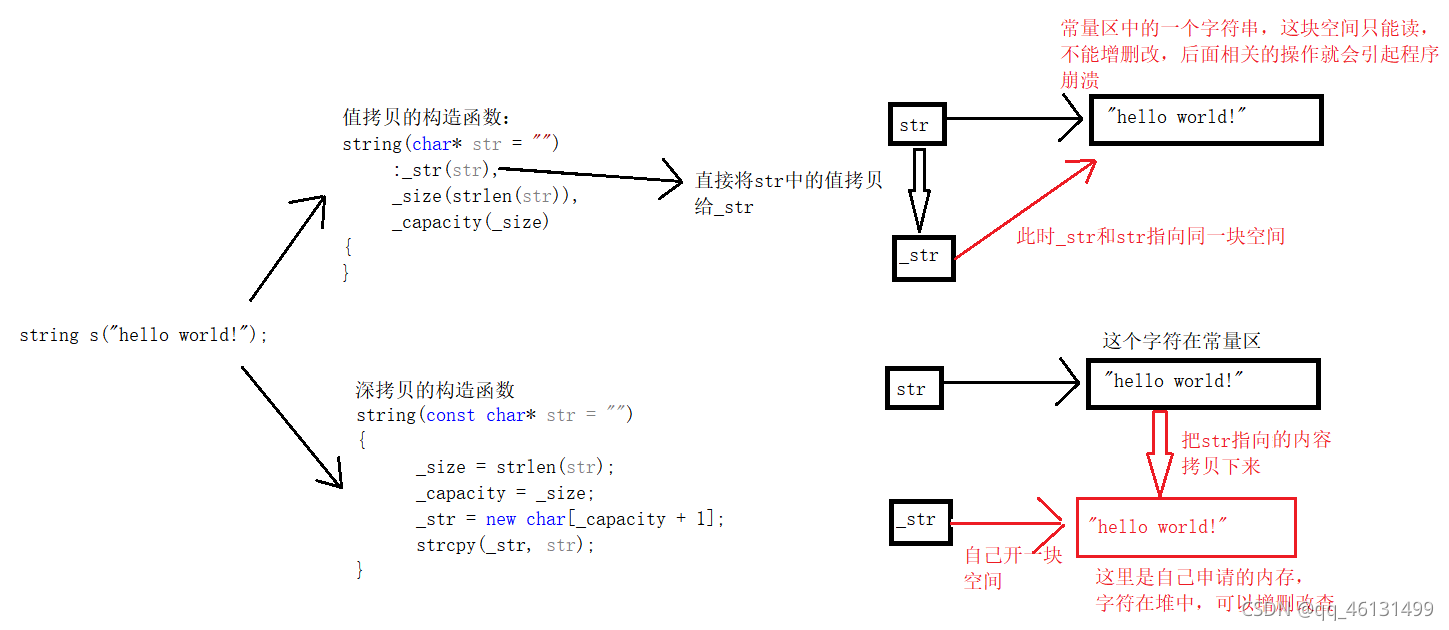
const size_t zhy::string::npos = -1;
zhy::string::string(const char* str)
{
_size = strlen(str);
_capacity = _size;
_str = new char[_capacity + 1];
strcpy(_str, str);
}
拷贝构造函数有两种写法:现代写法和传统写法。 传统写法:与上面构造函数的写法类似,直接开辟空间然后将字符串拷贝进开出的空间中; 现代写法:用传入的对象构造一个临时对象,直接将当前对象与临时对象交换内容即可。 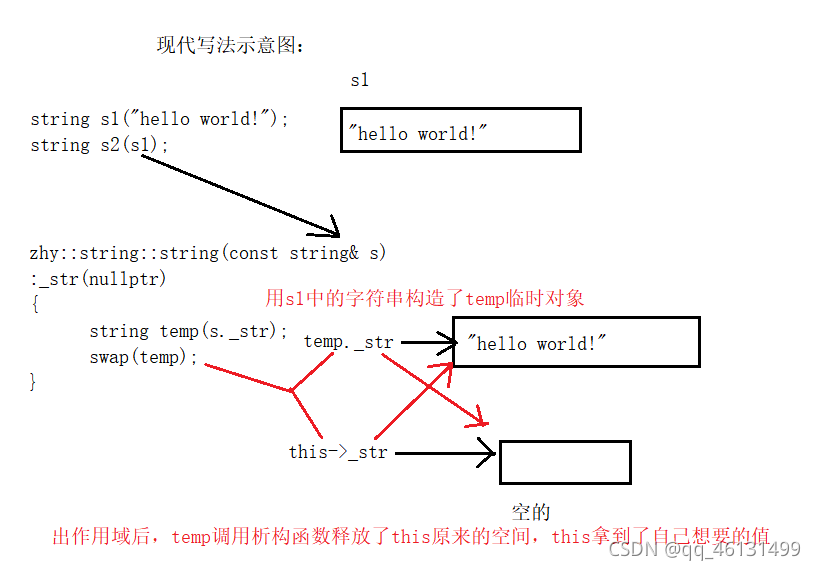
zhy::string::string(const string& s)
:_str(nullptr)
{
string temp(s._str);
swap(temp);
}
用现代写法对对象进行赋值
zhy::string& zhy::string:: operator=(const string& s)
{
if (this != &s)
{
string temp(s);
swap(temp);
}
return *this;
}
zhy::string::~string()
{
if (_str)
{
delete[] _str;
_str = nullptr;
_capacity = _size = 0;
}
}
直接返回对象的成员变量_size,_size记录着字符串的有效字符个数
size_t zhy::string::size()
{
return _size;
}
返回对象的成员变量_capacity,_capacity记录着字符串分配到的内存大小
size_t zhy::string::capacity()
{
return _capacity;
}
如果字符串中有效字符的个数为0,则代表该字符串是空串,返回true
bool zhy::string::empty()
{
if (_size == 0)
return true;
else
return false;
}
要注意每次修改后’\0’位置的变化和_size、_capacity的变化 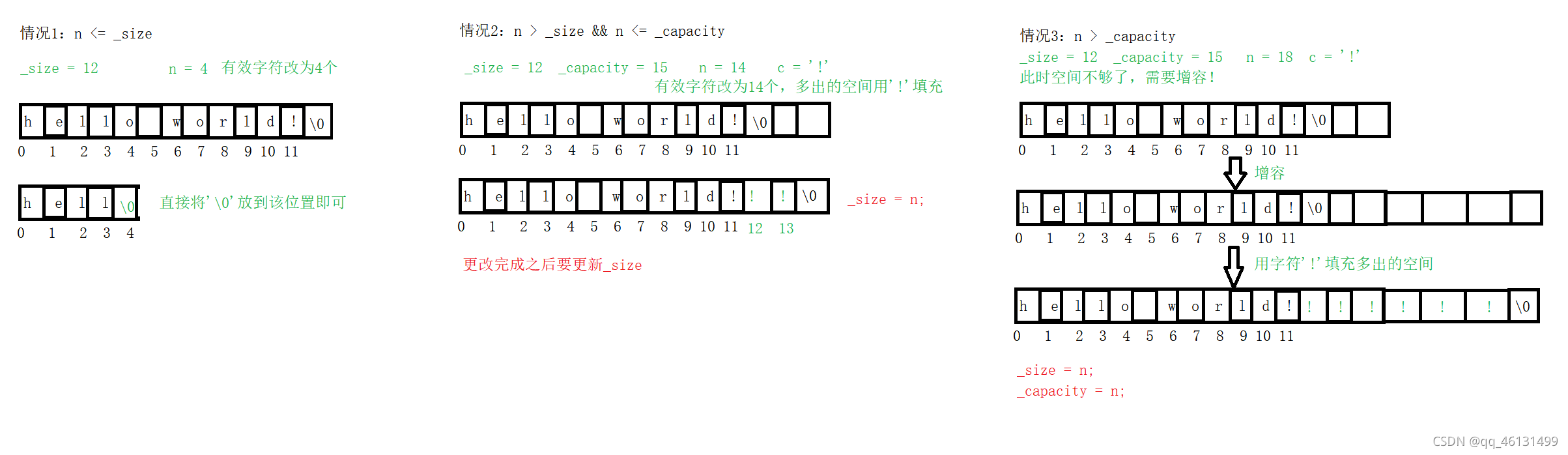
void zhy::string::resize(size_t n, char c)
{
if (n > _size)
{
if (n>_capacity)
{
reserve(n);
}
for (size_t i = _size; i < n; i++)
{
_str[i] = c;
}
}
_str[n] = '\0';
_size = n;
}
void zhy::string::reserve(size_t capacity)
{
if (capacity > _capacity)
{
char* str = new char[capacity + 1];
strcpy(str, _str);
delete[] _str;
_str = str;
_capacity = capacity;
}
}
让对象中的有效字符变为0个,只需要将_size改为0,’\0’放在第一个位置。
void zhy::string::clean()
{
_size = 0;
_str[_size] = '\0';
}
char zhy::string:: operator[](size_t i)
{
assert(i < _size);
return _str[i];
}
zhy::string::iterator zhy::string::begin()
{
return _str;
}
zhy::string::iterator zhy::string:: end()
{
return _str + _size;
}
void zhy::string::push_back(char c)
{
if (_size == _capacity)
reserve(2 * _capacity+1);
_str[_size] = c;
_size++;
_str[_size] = '\0';
}
void zhy::string::append(const char* str)
{
size_t len = strlen(str);
for (size_t i = 0; i < len; i++)
{
push_back(str[i]);
}
}
这些运算符重载直接复用上面的函数就可以
void zhy::string:: operator+=(char c)
{
push_back(c);
}
void zhy::string::operator+=(const char* s)
{
append(s);
}
void zhy::string::operator+=(const string& str)
{
append(str._str);
}
简单地遍历查找
size_t zhy::string::find(char c, size_t pos)
{
for (size_t i = pos; i < _size; i++)
{
if (_str[i] == c)
return i;
}
return npos;
}
size_t zhy::string::find(const char* s, size_t pos)
{
const char* pch = strstr(_str + pos, s);
if (pch != nullptr)
{
return pch - _str;
}
else
return npos;
}
插入字符和插入字符串方法相同,插入字符串需要移动字符的更多,下图是插入字符串的过程: 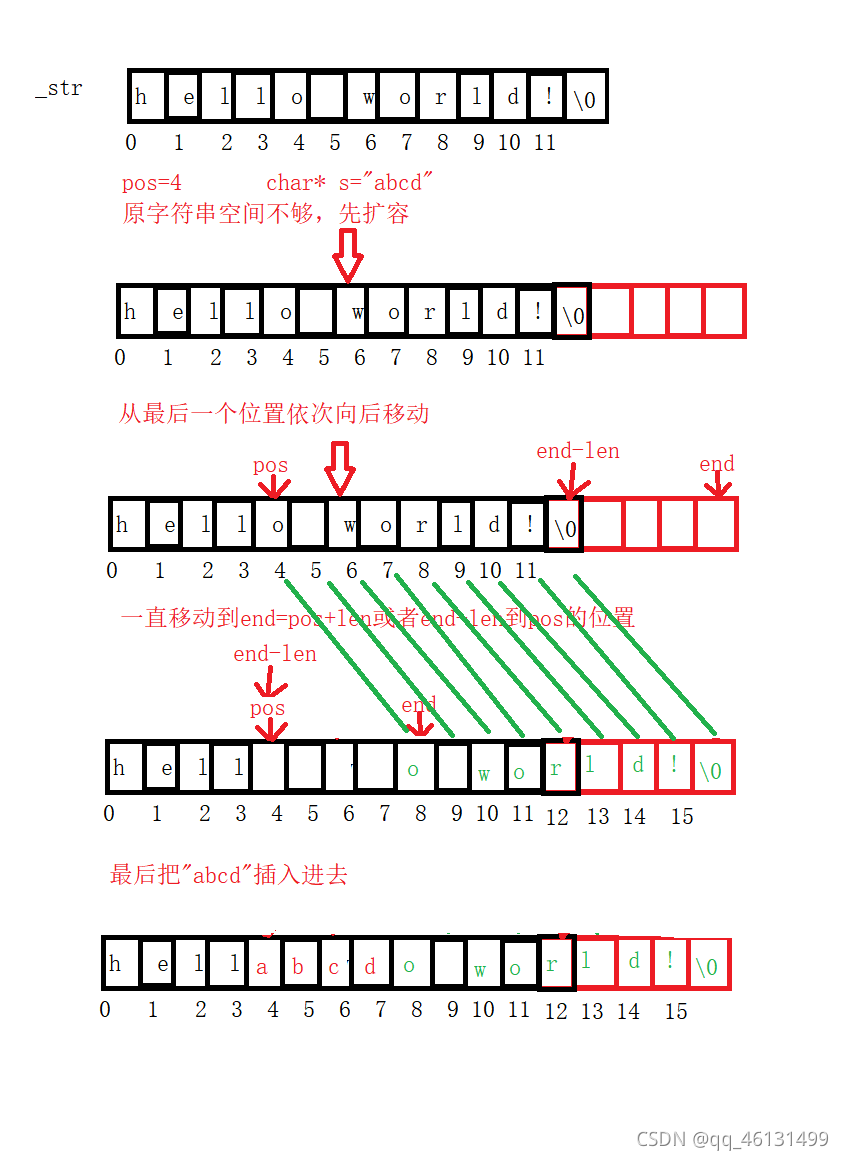
void zhy::string::insert(size_t pos, char c)
{
assert(pos <= _size);
if (_size == _capacity)
{
reserve(2 * _capacity + 1);
}
size_t end = _size + 1;
while (end > pos)
{
_str[end] = _str[end - 1];
end--;
}
_str[pos] = c;
_size++;
}
void zhy::string::insert(size_t pos, char* s)
{
assert(pos <= _size);
size_t len = strlen(s);
if (_size+len > _capacity)
{
reserve(_size + len);
}
size_t end = _size + len;
while (end >= pos + len)
{
_str[end] = _str[end - len];
end--;
}
strncpy(_str + pos, s, len);
_size += len;
}
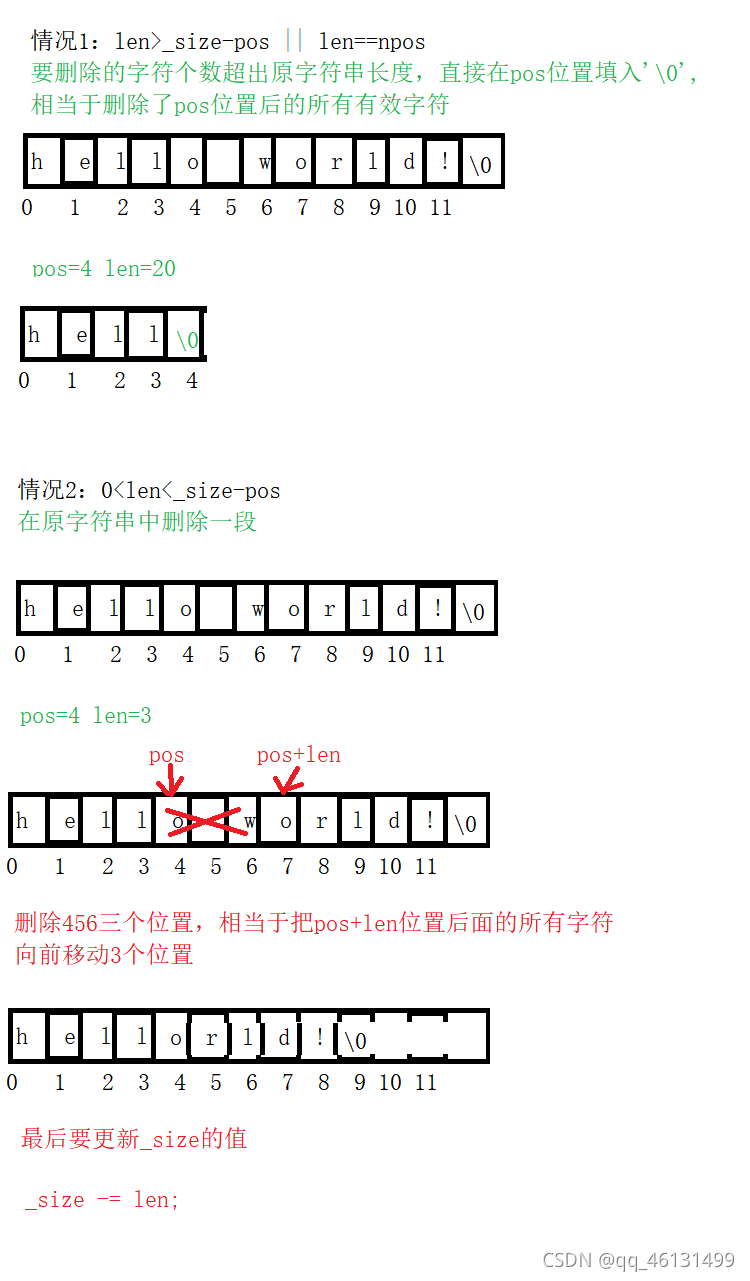
void zhy::string::erase(size_t pos, size_t len)
{
assert(pos < _size);
if (len >= _size - pos || len == npos)
{
_str[pos] = '\0';
_size = pos;
}
else
{
size_t first = pos + len;
while (first <= _size)
{
_str[first - len] = _str[first];
first++;
}
_size -= len;
}
}
void zhy::string::swap(string& temp)
{
_size = strlen(temp._str);
_capacity = _size;
std::swap(_str, temp._str);
}
const char* zhy::string::c_str()
{
return _str;
}
字符串比较大小的规则:
- 如果两字符串长度相等,比较两个字符串中第一个字符的ASCII码值,如果两值相同,则比较第二个字符,若相同,则比较第三个…如果全相同,则两字符串相等。如果比较过程中有两字符ASCII码值不同,哪个字符的ASCII值大,则该字符所在的字符串就大。
例:abcdef>abcdee ; abcd==abcd ; abc<bca - 如果两字符串长度不等,则它们不会相等,大小的比较按照上面的规则进行,如果在较短字符结束前比出大小,则结束。 如果比较到较短字符串末尾还没比出大小,则较短字符串小于长字符串。
例:abc>abaddd ; abc<abcaaa ; bca>abcjjj 注:标准库中关系运算符的重载是在类外实现的,复用比较方便直观,这里我将它们实现为类的成员函数,复用时需要用this指针调用
bool zhy::string::operator==(const string& s)
{
size_t i1 = 0, i2 = 0;
while (i1 < _size&&i2 < s._size)
{
if (_str[i1] != s._str[i2])
return false;
else
{
i1++;
i2++;
}
}
if (i1 == _size&&i2 == s._size)
return true;
else
return false;
}
bool zhy::string::operator<(const string& s)
{
size_t i1 = 0, i2 = 0;
while (i1 < _size&&i2 < s._size)
{
if (_str[i1]>s._str[i2])
return false;
else
{
i1++;
i2++;
}
}
if (i1 == _size&&i2 != s._size)
return true;
else
return false;
}
bool zhy::string::operator<=(const string& s)
{
if (this->operator<(s) || this->operator==(s))
return true;
else
return false;
}
bool zhy::string::operator>(const string& s)
{
if (this->operator<=(s))
return false;
else
return true;
}
bool zhy::string::operator>=(const string& s)
{
if (this->operator<(s))
return false;
else
return true;
}
bool zhy::string::operator!=(const string& s)
{
if (this->operator==(s))
return false;
else
return true;
}
ostream& zhy::operator<<(ostream& out, zhy::string& s)
{
for (size_t i = 0; i < s.size(); i++)
{
out << s[i];
}
return out;
}
istream& zhy::operator>>(istream& in, zhy::string& s)
{
s.resize(0);
char c;
while (1)
{
in.get(c);
if (c == ' ' || c == '\n')
{
break;
}
else
{
s.push_back(c);
}
}
return in;
}
2.3 完整代码
string.h
#pragma once
#include <assert.h>
#include <iostream>
using namespace std;
namespace zhy{
class string
{
public:
static const size_t npos;
typedef char* iterator;
string(const char* str = "");
string(const string& s);
string& operator=(const string& s);
~string();
size_t size();
size_t capacity();
bool empty();
void reserve(size_t capcity);
void resize(size_t n, char c = '\0');
void clean();
char operator[](size_t i);
iterator begin();
iterator end();
void push_back(char c);
void append(const char* str);
void operator+=(char c);
void operator+=(const char* s);
void operator+=(const string& str);
size_t find(char c, size_t pos = 0);
size_t find(const char* s, size_t pos = 0);
void insert(size_t pos, char c);
void insert(size_t pos, char* s);
void erase(size_t pos, size_t len = npos);
void swap(string& temp);
const char* c_str();
bool operator<(const string& s);
bool operator<=(const string& s);
bool operator>(const string& s);
bool operator>=(const string& s);
bool operator==(const string& s);
bool operator!=(const string& s);
private:
char* _str;
size_t _size;
size_t _capacity;
};
ostream& operator<<(ostream& out, zhy::string& s);
istream& operator>>(istream& in, zhy::string& s);
}
string.cpp
#include "string.h"
const size_t zhy::string::npos = -1;
zhy::string::string(const char* str)
{
_size = strlen(str);
_capacity = _size;
_str = new char[_capacity + 1];
strcpy(_str, str);
}
zhy::string::string(const string& s)
:_str(nullptr)
{
string temp(s._str);
swap(temp);
}
zhy::string& zhy::string:: operator=(const string& s)
{
if (this != &s)
{
string temp(s);
swap(temp);
}
return *this;
}
zhy::string::~string()
{
if (_str)
{
delete[] _str;
_str = nullptr;
_capacity = _size = 0;
}
}
size_t zhy::string::size()
{
return _size;
}
size_t zhy::string::capacity()
{
return _capacity;
}
bool zhy::string::empty()
{
if (_size == 0)
return true;
else
return false;
}
void zhy::string::reserve(size_t capacity)
{
if (capacity > _capacity)
{
char* str = new char[capacity + 1];
strcpy(str, _str);
delete[] _str;
_str = str;
_capacity = capacity;
}
}
void zhy::string::resize(size_t n, char c)
{
if (n > _size)
{
if (n>_capacity)
{
reserve(n);
}
for (size_t i = _size; i < n; i++)
{
_str[i] = c;
}
}
_str[n] = '\0';
_size = n;
}
void zhy::string::clean()
{
_size = 0;
_str[_size] = '\0';
}
char zhy::string:: operator[](size_t i)
{
assert(i < _size);
return _str[i];
}
zhy::string::iterator zhy::string::begin()
{
return _str;
}
zhy::string::iterator zhy::string:: end()
{
return _str + _size;
}
void zhy::string::push_back(char c)
{
if (_size == _capacity)
reserve(2 * _capacity+1);
_str[_size] = c;
_size++;
_str[_size] = '\0';
}
void zhy::string::append(const char* str)
{
size_t len = strlen(str);
for (size_t i = 0; i < len; i++)
{
push_back(str[i]);
}
}
void zhy::string:: operator+=(char c)
{
push_back(c);
}
void zhy::string::operator+=(const char* s)
{
append(s);
}
void zhy::string::operator+=(const string& str)
{
append(str._str);
}
size_t zhy::string::find(char c, size_t pos)
{
for (size_t i = pos; i < _size; i++)
{
if (_str[i] == c)
return i;
}
return npos;
}
size_t zhy::string::find(const char* s, size_t pos)
{
const char* pch = strstr(_str + pos, s);
if (pch != nullptr)
{
return pch - _str;
}
else
return npos;
}
void zhy::string::insert(size_t pos, char c)
{
assert(pos <= _size);
if (_size == _capacity)
{
reserve(2 * _capacity + 1);
}
size_t end = _size + 1;
while (end > pos)
{
_str[end] = _str[end - 1];
end--;
}
_str[pos] = c;
_size++;
}
void zhy::string::insert(size_t pos, char* s)
{
assert(pos <= _size);
size_t len = strlen(s);
if (_size+len > _capacity)
{
reserve(_size + len);
}
size_t end = _size + len;
while (end >= pos + len)
{
_str[end] = _str[end - len];
end--;
}
strncpy(_str + pos, s, len);
_size += len;
}
void zhy::string::erase(size_t pos, size_t len)
{
assert(pos < _size);
if (len >= _size - pos || len == npos)
{
_str[pos] = '\0';
_size = pos;
}
else
{
size_t first = pos + len;
while (first <= _size)
{
_str[first - len] = _str[first];
first++;
}
_size -= len;
}
}
void zhy::string::swap(string& temp)
{
_size = strlen(temp._str);
_capacity = _size;
std::swap(_str, temp._str);
}
const char* zhy::string::c_str()
{
return _str;
}
|