1. 对象使用过程中背后调用了哪些方法
#include<iostream>
using namespace std;
class Test
{
public:
Test(int a=10):ma(a)
{
cout << "Test(int)" << endl;
}
~Test()
{
cout << "~Test()" << endl;
}
Test(const Test& t) :ma(t.ma)
{
cout << "Test(const Test& t)" << endl;
}
Test& operator=(const Test& t)
{
cout << "operator=" << endl;
ma = t.ma;
return *this;
}
private:
int ma;
};
int main()
{
Test t1;
Test t2(t1);
Test t3 = t1;
Test t4 = Test(20);
cout << "--------------------" << endl;
t4 = t2;
t4 = Test(30);
t4 = (Test)30;
t4 = 30;
cout << "---------------------" << endl;
Test *p = &Test(40);
const Test& ref = Test(50);
cout << "---------------------" << endl;
return 0;
}
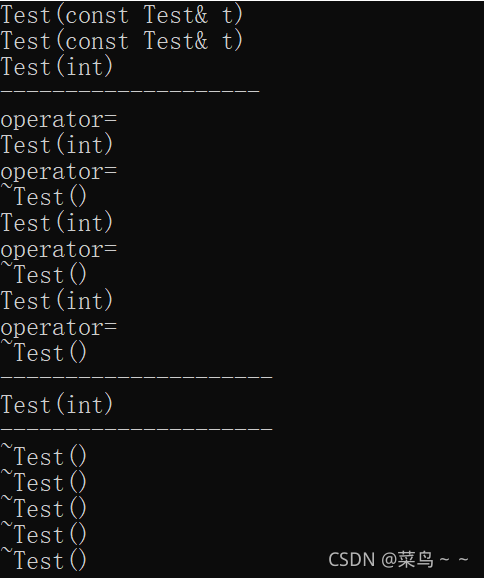
C++编译器对于对象构造的优化:用临时对象生成新对象的时候,临时对象就不产生了,直接构造新对象就可以了
#include<iostream>
using namespace std;
class Test
{
public:
Test(int a=5,int b=5):ma(a),mb(b)
{
cout << "Test(int,int)" << endl;
}
~Test()
{
cout << "~Test()" << endl;
}
Test(const Test& src) :ma(src.ma), mb(src.mb)
{
cout << "Test(const Test& src)" << endl;
}
void operator=(const Test& src)
{
ma = src.ma;
mb = src.mb;
cout << "operator=" << endl;
}
private:
int ma;
int mb;
};
Test t1(10, 10);
int main()
{
Test t2(20, 20);
Test t3 = t2;
static Test t4 = Test(30, 30);
t2 = Test(40, 40);
t2 = (Test)(50, 50);
t2 = 60;
Test* p1 = new Test(70, 70);
Test* p2 = new Test[2];
const Test& p4 = Test(90, 90);
delete p1;
delete[]p2;
}
Test t5(100, 100);
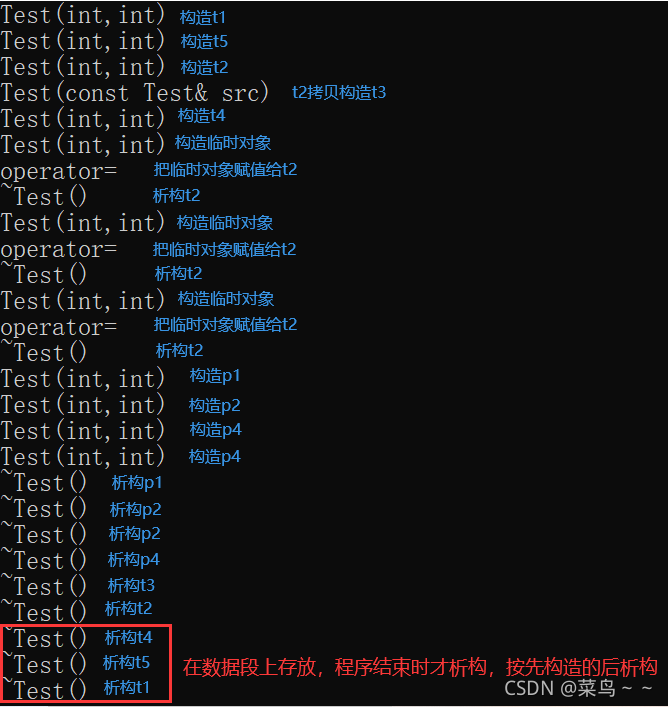
2. 函数调用过程中对象背后调用的方法
#include<iostream>
using namespace std;
class Test
{
public:
Test(int a = 10) :ma(a)
{
cout << "Test(int)" << endl;
}
~Test()
{
cout << "~Test()" << endl;
}
Test(const Test& t) :ma(t.ma)
{
cout << "Test(const Test& t)" << endl;
}
Test& operator=(const Test& t)
{
cout << "operator=" << endl;
ma = t.ma;
return *this;
}
int getData()const
{
return ma;
}
private:
int ma;
};
Test GetObject(Test t)
{
int val = t.getData();
Test tmp(val);
return tmp;
}
int main()
{
Test t1;
Test t2;
t2 = GetObject(t1);
return 0;
}
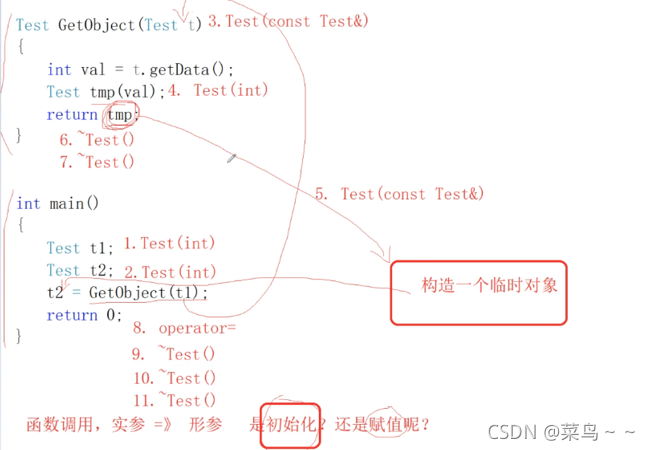 不能返回局部的或者临时对象的引用或指针
3. 对象优化的规则
- 函数参数传递过程中,对象优先按引用传递,不要按值传递
Test GetObject(Test &t)
{
int val = t.getData();
Test tmp(val);
return tmp;
}
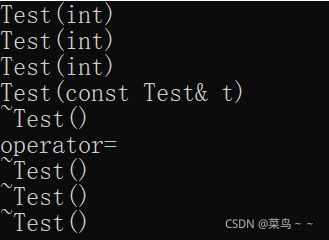
4. CMyString
代码问题
#include <iostream>
using namespace std;
class String
{
public:
String(const char* str = nullptr)
{
cout << "String(const char*)" << endl;
if (str != nullptr)
{
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
}
else
{
m_data = new char[1];
*m_data = '\0';
}
}
String(const String& src)
{
cout << "String(const String& src)" << endl;
m_data = new char[strlen(src.m_data) + 1];
strcpy(m_data, src.m_data);
}
~String()
{
cout << "~String()" << endl;
delete[]m_data;
m_data = nullptr;
}
String& operator=(const String& src)
{
cout << "operator=" << endl;
if (&src == this)
{
return *this;
}
delete[]m_data;
m_data = new char[strlen(src.m_data) + 1];
strcpy(m_data, src.m_data);
return *this;
}
const char* c_str() {
return m_data;
}
private:
char* m_data;
};
String get_string(String& str) {
const char* pstr = str.c_str();
String tmp(pstr);
return tmp;
}
int main() {
String str1("11111111111111111");
String str2;
str2 = get_string(str1);
cout << str2.c_str() << endl;
return 0;
}
添加带右值引用参数的拷贝构造和赋值函数
String(String&& src){
cout << "String(const String&& src)" << endl;
m_data = src.m_data;
src.m_data = nullptr;
}
String& operator=(String&& src){
cout << "operator=(String&& src)" << endl;
if (&src == this)
{
return *this;
}
delete[]m_data;
m_data = src.m_data;
src.m_data = nullptr;
return *this;
}
CMyString在vector上的应用 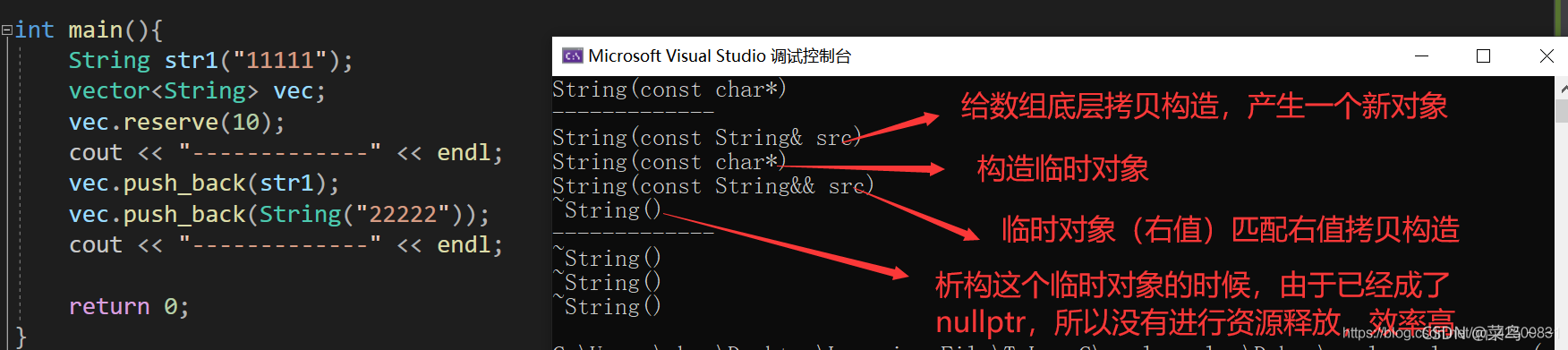
5.move和forward
std::move:移动语义,得到右值类型 std::forward:类型的完美转发,得到真实的左/右值
template<typename Ty2>
void construct(T* p, Ty2&& val)
{
new (p) T(std::forward<Ty2>(val));
}
template<typename Ty1>
void push_back(Ty1&& val)
{
if (full())
{
expand();
}
_allocator.construct(_last, std::forward<Ty1>(val));
_last++;
}
函数模板的类型推演 + 引用折叠
String&& + && = String&&
String& + && = String&
|