1、前言
在C语言里,字符串是用字符数组来表示的,而对于应用层而言,会经常用到字符串,而继续使用字符数组,就使得效率非常低. 所以在C++标准库里,通过类string从新自定义了字符串 在使用string类时,必须包含#include头文件以及using namespace std
2、string类的常用接口说明
2.1常用的构造函数使用
函数名称 | 函数说明 |
---|
string() | 构造函数,用于构造一个空类 | string(const char* s) | 用字符串来构造string类对象 | string(size_t n, char c) | 构造含n个字符c的对象 | string(const string&s) | 拷贝构造 |
int main()
{
string s1;
string s2("hello");
string s3(10, 'c');
string s4(s3);
cout << s1 << endl;
cout << s2 << endl;
cout << s3 << endl;
cout << s4 << endl;
return 0;
}
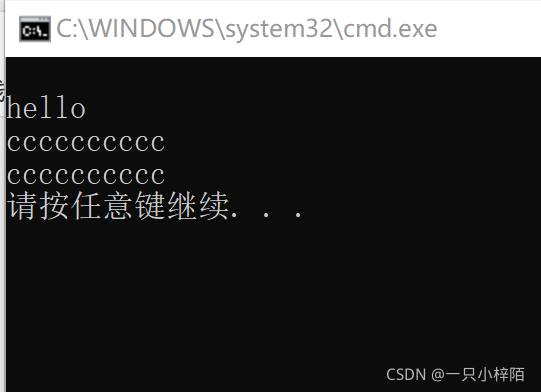
2.2 string类对象的容量操作
函数名称 | 函数说明 |
---|
size | 返回字符串有效字符长度 | capacity | 返回空间大小 | length | 返回字符串有效字符长度 | clear | 清空有效字符 | empty | 检测字符串是否为空串 | reserve | 为字符串预留空间 | resize | 将有效字符的个数改成n个,多出的空间用字符c填充 |
string s1("hello world");
cout << s1.size() << endl;
cout << s1.length() << endl;
cout << s1.capacity() << endl;
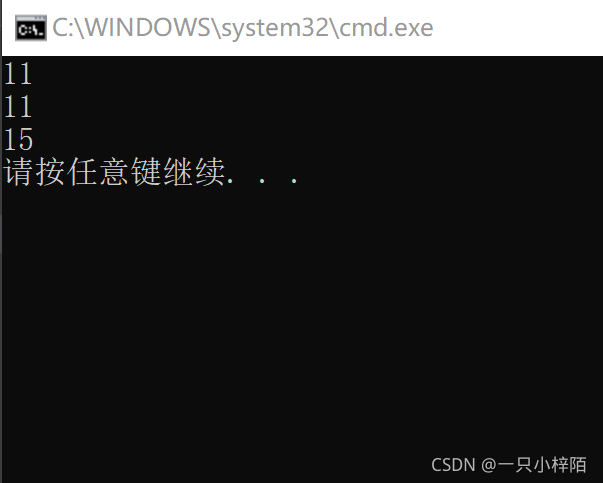
string s1("hello world XXXXXXXXXXXXXXXXXXX");
cout << s1 << endl;
cout << s1.capacity() << endl;
cout << s1.size() << endl;
s1.clear();
cout << "——————————————" << endl;
cout << s1.capacity() << endl;
cout << s1.size() << endl;
cout << s1 << endl;
将s1中的字符串清空,注意清空时只是将size清0,不改变底层空间的大小
string s1("hello world");
s1.resize(20, 'a');
cout << s1 << endl;
cout << s1.size() << endl;
cout << s1.capacity() << endl;
string s("hello world");
s.resize(15);
cout << s.size() << endl;
cout << s.capacity() << endl;
cout << s << endl;
resize(size_t n) 与 resize(size_t n, char c)都是将字符串中有效字符个数改变到n个,不同的是,当字符个数增多时:resize(n)用0来填充多出的元素空间,resize(size_t n, char c)用字符c来填充多出的元素空间。注意:resize在改变元素个数时,如果是将元素个数增多,可能会改变底层容量的大小,如果是将元素个数减少,底层空间总大小不变。
reserve(size_t res_arg=0):为string预留空间,不改变有效元素个数,当reserve的参数小于 string的底层空间总大小时,reserver不会改变容量大小。
2.3 string类对象的修改操作
函数名称 | 函数说明 |
---|
push_back | 尾插字符 | append | 尾插字符串 | operator+= | 尾插字符串 | c_str | 返回C格式字符串 | find | 从字符串pos位置开始往后找字符c,返回该字符在字符串中的位置 | substr | 在str中从pos位置开始,截取n个字符,然后将其返回 |
3、实现一个普通的string
class string
{
public:
string(const char* str = "")
{
if (nullptr == str)
{
assert(false);
return;
}
_str = new char[strlen(str) + 1];
strcpy(_str, str);
}
string(const string& s)
: _str(new char[strlen(s._str) + 1])
{
strcpy(_str, s._str);
}
string& operator=(const string& s)
{
if (this != &s)
{
char* pStr = new char[strlen(s._str) + 1];
strcpy(pStr, s._str);
delete[] _str;
_str = pStr;
}
return *this;
}
~string()
{
if (_str)
{
delete[] _str;
_str = nullptr;
}
}
private:
char* _str;
};
|