案例描述
- 公司今天招聘了10个员工(ABCDEFGHIJ),10名员工进入公司之后,需要指派员工在那个部门工作
- 员工信息有: 姓名 工资组成;部门分为:策划、美术、研发
- 随机给10名员工分配部门和工资
- 通过multimap进行信息的插入 key(部门编号) value(员工)
- 分部门显示员工信息
实现步骤
- 创建10名员工,放到vector中
- 遍历vector容器,取出每个员工,进行随机分组
- 分组后,将员工部门编号作为key,具体员工作为value,放入到multimap容器中
- 分部门显示员工信息
#include <iostream>
using namespace std;
#include <string>
#include<algorithm>
#include<deque>
#include <stack>
#include <queue>
#include <list>
#include <set>
#include <map>
#include<vector>
class person
{
public:
string p_name;
int p_salary;
};
void creatMember(vector<person> &p)
{
string str = "ABCDEFGHIJ";
for (int i = 0; i < 10; i++)
{
person per;
per.p_name = "员工";
per.p_name += str[i];
per.p_salary = rand() % 10000 + 10000;
p.push_back(per);
}
}
void test01(vector<person>&v,multimap<int,person>&m)
{
for (vector<person>::iterator it = v.begin(); it != v.end(); it++)
{
int num = rand() % 3;
m.insert(make_pair(num, *it));
}
}
void printmulmap(multimap<int, person>& m)
{
for (multimap<int, person>::iterator it = m.begin(); it != m.end(); it++)
{
cout << "姓名:" << it->second.p_name << " 工资:" << it->second.p_salary << " 部门编号:" << it->first << endl;
}
}
int main() {
vector<person> p;
multimap<int, person>m;
creatMember(p);
test01(p,m);
printmulmap(m);
system("pause");
return 0;
}
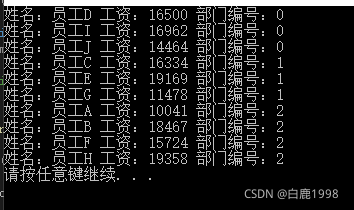
|