C++多态
哔哩哔哩黑马:https://www.bilibili.com/video/BV1et411b73Z?p=138&spm_id_from=pageDriver
计算器
#include<iostream>
#include<string>
using namespace std;
class Caculator
{
private:
public:
Caculator(){};
~Caculator(){};
int m_Num1;
int m_Num2;
int getResult(string oper)
{
if (oper == "+")
{
return m_Num1 + m_Num2;
}
else if (oper == "-")
{
return m_Num1 - m_Num2;
}
else if (oper == "*")
{
return m_Num1 * m_Num2;
}
else
{
cout<<"未定义"<<endl;
}
}
};
void test01()
{
Caculator cacu;
cacu.m_Num1 = 10;
cacu.m_Num2 = 10;
cout<<cacu.getResult("+")<<endl;
}
class Cacul
{
private:
public:
Cacul(){};
~Cacul(){};
virtual int getResult()=0;
};
class ADD : public Cacul
{
private:
int m_Num1;
int m_Num2;
public:
ADD(int n1, int n2):m_Num1(n1),m_Num2(n2){};
~ADD(){};
int getResult()
{
return m_Num1 + m_Num2;
}
};
class SUB : public Cacul
{
private:
int m_Num1;
int m_Num2;
public:
SUB(int n1, int n2):m_Num1(n1),m_Num2(n2){};
~SUB(){};
int getResult()
{
return m_Num1 - m_Num2;
}
};
class MUL : public Cacul
{
private:
int m_Num1;
int m_Num2;
public:
MUL(int n1, int n2):m_Num1(n1),m_Num2(n2){};
~MUL(){};
int getResult()
{
return m_Num1 * m_Num2;
}
};
int doCaculate(Cacul& ca)
{
cout<<ca.getResult()<<endl;
}
void test02()
{
ADD myadd(10,20);
doCaculate(myadd);
Cacul *ca = new MUL(10,30);
cout<<ca->getResult()<<endl;
delete ca;
}
int main()
{
test02();
return 0;
}
组装电脑
电脑主要组成部件为 CPU(用于计算),显卡(用于显示),内存条(用于存储) 将每个零件封装出抽象基类,并且提供不同的厂商生产不同的零件,例如Intel厂商和Lenovo厂商 创建电脑类提供让电脑工作的函数,并且调用每个零件工作的接口 测试时组装三台不同的电脑进行工作
#include<iostream>
#include<string>
using namespace std;
class CPU
{
private:
public:
CPU(){
cout <<"构造CPU: "<<endl;
};
virtual ~CPU(){
cout <<"析构CPU: "<<endl;
};
virtual void caculate() = 0;
};
class IntelCPU : public CPU
{
private:
string *cpu_name;
public:
IntelCPU(string name)
{
cpu_name = new string(name);
cout <<"构造 Intel CPU: "<<*cpu_name<<endl;
}
~IntelCPU()
{
if (cpu_name != nullptr)
{
delete cpu_name;
cpu_name = nullptr;
}
cout<<"调用 IntelCPU 析构"<<endl;
}
void caculate()
{
cout<<"Intel CPU "<<*cpu_name<<" 开始计算!"<<endl;
}
};
class AMDCPU : public CPU
{
private:
string *cpu_name;
public:
AMDCPU(string name)
{
cpu_name = new string(name);
cout <<"构造 AMD CPU: "<<*cpu_name<<endl;
}
~AMDCPU()
{
if (cpu_name != nullptr)
{
delete cpu_name;
cpu_name = nullptr;
}
cout<<"调用 AMDCPU 析构"<<endl;
}
void caculate()
{
cout<<"AMD CPU "<<*cpu_name<<" 开始计算!"<<endl;
}
};
class GPU
{
private:
public:
GPU(){
cout <<"构造GPU: "<<endl;
};
virtual ~GPU(){
cout <<"析构GPU: "<<endl;
};
virtual void dispaly() = 0;
};
class IntelGPU : public GPU
{
private:
string *gpu_name;
public:
IntelGPU(string name)
{
gpu_name = new string(name);
cout <<"构造 Intel GPU: "<<*gpu_name<<endl;
}
~IntelGPU()
{
if (gpu_name != nullptr)
{
delete gpu_name;
gpu_name = nullptr;
}
cout<<"调用 IntelGPU 析构"<<endl;
}
void dispaly()
{
cout<<"Intel GPU "<<*gpu_name<<" 开始显示画面!"<<endl;
}
};
class NvidiaGPU : public GPU
{
private:
string *gpu_name;
public:
NvidiaGPU(string name)
{
gpu_name = new string(name);
cout <<"构造 NvidiaGPU: "<<*gpu_name<<endl;
}
~NvidiaGPU()
{
if (gpu_name != nullptr)
{
delete gpu_name;
gpu_name = nullptr;
}
cout<<"调用 NvidiaGPU 析构"<<endl;
}
void dispaly()
{
cout<<"NvidiaGPU "<<*gpu_name<<" 开始显示画面!"<<endl;
}
};
class RAM
{
private:
public:
RAM(){
cout <<"构造RAM: "<<endl;
};
virtual ~RAM(){
cout <<"析构RAM: "<<endl;
};
virtual void load() = 0;
};
class KingstomRAM : public RAM
{
private:
string *ran_name;
string *ran_size;
public:
KingstomRAM(string name, string size)
{
ran_name = new string(name);
ran_size = new string(size);
cout <<"构造 KingstomRAM: "<<*ran_name<<endl;
}
~KingstomRAM()
{
if (ran_name != nullptr)
{
delete ran_name;
ran_name = nullptr;
}
if (ran_size != nullptr)
{
delete ran_size;
ran_size = nullptr;
}
cout<<"调用 KingstomRAM 析构"<<endl;
}
void load()
{
cout<<"KingstomRAM "<<*ran_name<<"("<<*ran_size<<")"<<" 正在加载数据!"<<endl;
}
};
class GlowyRAM : public RAM
{
private:
string *ran_name;
string *ran_size;
public:
GlowyRAM(string name, string size)
{
ran_name = new string(name);
ran_size = new string(size);
cout <<"构造 GlowyRAM: "<<*ran_name<<endl;
}
~GlowyRAM()
{
if (ran_name != nullptr)
{
delete ran_name;
ran_name = nullptr;
}
if (ran_size != nullptr)
{
delete ran_size;
ran_size = nullptr;
}
cout<<"调用 GlowyRAM 析构"<<endl;
}
void load()
{
cout<<"GlowyRAM "<<*ran_name<<"("<<*ran_size<<")"<<" 正在加载数据!"<<endl;
}
};
class Computer
{
private:
CPU *m_cpu;
GPU *m_gpu;
RAM *m_ran;
public:
Computer(CPU *cpu, GPU *gpu, RAM *ran)
{
m_cpu = cpu;
m_gpu = gpu;
m_ran = ran;
cout<<"构造Computer"<<endl;
}
~Computer()
{
if (m_cpu != nullptr)
{
delete m_cpu;
m_cpu = nullptr;
}
if (m_gpu != nullptr)
{
delete m_gpu;
m_gpu = nullptr;
}
if (m_ran != nullptr)
{
delete m_ran;
m_ran = nullptr;
}
cout<<"析构Computer"<<endl;
};
void work()
{
m_cpu->caculate();
m_gpu->dispaly();
m_ran->load();
}
};
void test01()
{
Computer computer1(new IntelCPU("i3"), new NvidiaGPU("1060"), new KingstomRAM("Hike","8G"));
computer1.work();
cout<<"----------------------------------"<<endl;
Computer computer2(new IntelCPU("i7"), new NvidiaGPU("3060"), new KingstomRAM("Hike","32G"));
computer2.work();
cout<<"----------------------------------"<<endl;
Computer computer3(new AMDCPU("R5"), new IntelGPU("5700"), new GlowyRAM("悍将","4G"));
computer3.work();
cout<<"----------------------------------"<<endl;
}
int main()
{
test01();
return 0;
}
运行结果: 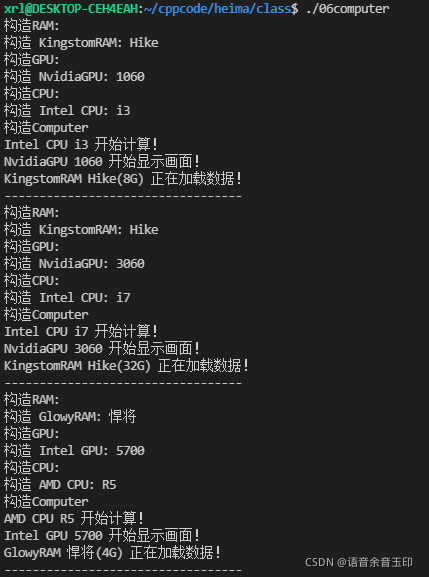 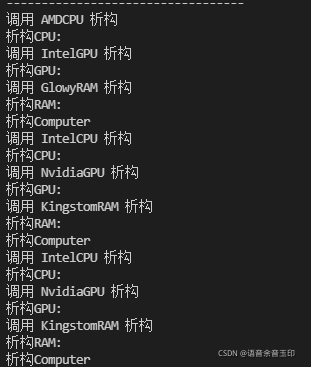
|