链栈类的实现
这个由于比较简单,就直接贴代码了,记录一下自己写过这个~
// 结点类
template <class T>
class Node
{
public:
Node() = default;
Node(T data, Node<T>* next)
{
this->_data = data;
this->_next = next;
}
public:
T _data;
Node<T>* _next;
};
// 链栈类
template <class T>
class StackLink
{
public:
// 构造函数初始化链栈
StackLink()
{
phead = nullptr;
count = 0;
}
// 析构函数回收内存
~StackLink()
{
while(phead != nullptr)
{
Node<T>* temp = phead;
phead = phead->_next;
delete temp;
temp = nullptr;
}
}
// 返回链栈中结点个数
int size()
{
return count;
}
// 判断栈是否为空
bool isEmpty()
{
bool flag = false;
if(phead == nullptr)
{
flag = true;
}
return flag;
}
// 入栈
void push(T data)
{
Node<T>* newNode = new Node<T>(data, phead);
phead = newNode;
count++;
}
// 出栈
T pop()
{
if(phead != nullptr)
{
T temp_data = phead->_data;
Node<T>* temp_p = phead;
phead = phead->_next;
delete temp_p;
temp_p = nullptr;
count--;
return temp_data;
}
else
{
cout << "栈中没有元素,无法出栈!" << endl;
return 0;
}
}
// 取栈顶元素
T getHead()
{
if(phead != nullptr)
{
return phead->_data;
}
else
{
cout << "栈中没有元素,无法出栈!" << endl;
return 0;
}
}
private:
Node<T>* phead; // 指向栈顶
int count; // 记录栈中元素个数
};
测试
由于上述为模板类,测试时为方便起见,我们还是用 int 类型
// 测试
void test01()
{
StackLink<int> SL;
for(int i = 0; i < 10; i++)
{
SL.push(i);
}
cout << "栈的大小为:" << SL.size() << endl;
while (SL.size() != 0)
{
cout << SL.pop() << " ";
}
cout << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
运行结果
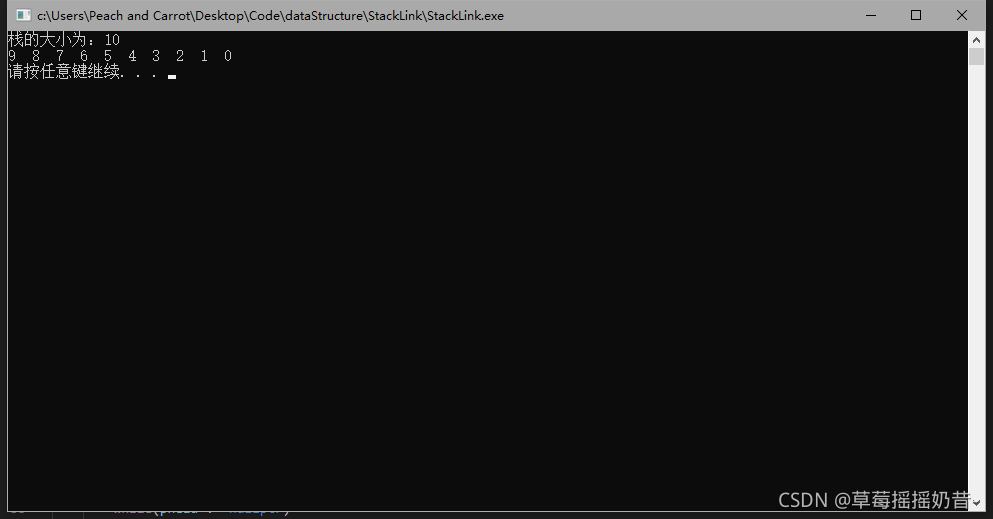
|