通过写这个结构体,也复习了好多C语言知识
场景:
学校正在做毕设项目,每名老师带领5个学生,总共有3名老师,需求如下: 设计学生和老师的结构体(类),其中在老师的结构体(类)中,有老师姓名和一个存放5名学生的数组作为成员,学生的成员有姓名、考试分数,创建数组存放3名老师,通过函数给每个老师及所带的学生赋值最终打印出老师数据以及老师所带的学生数据。
第一版代码:(好多C语言写法)
#include<iostream>
#include<string>
#include<ctime>
using namespace std;
struct Student
{
char name[100];
int score;
};
struct Teacher
{
char name[100];
Student stu[5];
};
typedef struct Name_st
{
char name[100];
}Name;
Name TeacherName[3] =
{
{"张一"},
{"张二"},
{"张三"}
};
Name studentName[5] =
{
{ "李一" },
{ "李二" },
{ "李三" },
{ "李四" },
{ "李五" }
};
void AllocationData(Teacher* Tec, int len)
{
for (int i = 0; i < len; i++)
{
strcpy_s( Tec[i].name, TeacherName[i].name);
for (int j = 0; j < 5; j++)
{
strcpy_s(Tec[i].stu[j].name, studentName[j].name);
Tec[i].stu[j].score = rand() % 50 + 50;
}
}
}
void PrintData(Teacher* Tec,int len)
{
for (int i = 0; i < len; i++)
{
cout << "教师:" << Tec[i].name << endl;
for (int j = 0; j < 5; j++)
{
cout << "\t学生:" << Tec[i].stu[j].name << " 分数:" << Tec[i].stu[j].score << endl;
}
}
}
int main()
{
srand((unsigned int)time(NULL));
Teacher Tec[3];
int len = sizeof(Tec) / sizeof(Teacher);
AllocationData(Tec,len);
PrintData(Tec,len);
return 0;
}
第二版代码:
#include<iostream>
#include<string>
#include<ctime>
using namespace std;
struct Student
{
string name;
int score;
};
struct Teacher
{
string name;
Student stu[5];
};
void AllocationData(Teacher* Tec, int len)
{
string TeacherName[] = { "张一","张二", "张三" };
string studentName[] = { "李一","李二", "李三","李四","李五" };
for (int i = 0; i < len; i++)
{
Tec[i].name = TeacherName[i];
for (int j = 0; j < 5; j++)
{
Tec[i].stu[j].name = studentName[j];
Tec[i].stu[j].score = rand() % 50 + 50;
}
}
}
void PrintData(Teacher* Tec,int len)
{
for (int i = 0; i < len; i++)
{
cout << "教师:" << Tec[i].name << endl;
for (int j = 0; j < 5; j++)
{
cout << "\t学生:" << Tec[i].stu[j].name << " 分数:" << Tec[i].stu[j].score << endl;
}
}
}
int main()
{
srand((unsigned int)time(NULL));
Teacher Tec[3];
int len = sizeof(Tec) / sizeof(Teacher);
AllocationData(Tec,len);
PrintData(Tec,len);
return 0;
}
第三版代码:(结构体替换成类)
#include<iostream>
#include<string>
#include<ctime>
using namespace std;
class Student
{
public:
string name;
int score;
};
class Teacher
{
public:
string name;
Student stu[5];
};
void AllocationData(Teacher* Tec, int len)
{
string TeacherName[] = { "张一","张二", "张三" };
string studentName[] = { "李一","李二", "李三","李四","李五" };
for (int i = 0; i < len; i++)
{
Tec[i].name = TeacherName[i];
for (int j = 0; j < 5; j++)
{
Tec[i].stu[j].name = studentName[j];
Tec[i].stu[j].score = rand() % 50 + 50;
}
}
}
void PrintData(Teacher* Tec,int len)
{
for (int i = 0; i < len; i++)
{
cout << "教师:" << Tec[i].name << endl;
for (int j = 0; j < 5; j++)
{
cout << "\t学生:" << Tec[i].stu[j].name << " 分数:" << Tec[i].stu[j].score << endl;
}
}
}
int main()
{
srand((unsigned int)time(NULL));
Teacher Tec[3];
int len = sizeof(Tec) / sizeof(Teacher);
AllocationData(Tec,len);
PrintData(Tec,len);
return 0;
}
现象:
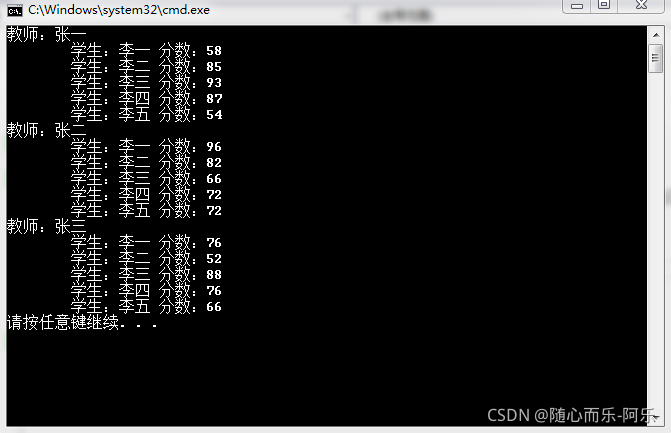
|