The standard form of a polynomial of degree
n
n
n is
P
(
x
)
=
a
n
x
n
+
a
n
?
1
x
n
?
1
+
?
+
a
1
x
+
a
0
P(x)=a_nx_n+a_{n?1}x_{n?1}+?+a_1x+a_0
P(x)=an?xn?+an?1?xn?1?+?+a1?x+a0?. Given that
a
n
=
1
a_n=1
an?=1 and all the
n
n
n roots
r
1
,
.
.
.
,
r
n
{ r_1, ..., r_n}
r1?,...,rn? of
P
(
x
)
P(x)
P(x) are integers. Then
P
(
x
)
P(x)
P(x) can be written as
(
x
?
r
1
)
(
x
?
r
2
)
?
(
x
?
r
n
)
(x?r_1)(x?r_2)?(x?r_n)
(x?r1?)(x?r2?)?(x?rn?). Your job is to write
P
(
x
)
P(x)
P(x) in its standard form with all roots given.
Input Specification:
Each input file contains one test case. For each case, the first line gives a positive
n
(
≤
10
)
n (≤10)
n(≤10) which is the degree of
P
(
x
)
P(x)
P(x). Then n integer roots are given in the next line, separated by spaces.
Output Specification:
For each case, output the coefficients
a
i
(
i
=
n
?
1
,
?
,
0
)
a_i(i=n?1,?,0)
ai?(i=n?1,?,0) in a line. All the numbers must be separated by one space, and there must be no extra space at the beginning or the end of the line. It is guaranteed that all the calculation will be within the range of int.
Sample Input:
3
-1 4 -1
Sample Output:
-2 -7 -4
Solution:
#include <iostream>
#include <vector>
using namespace std;
int roots[15];
int coeff[15];
int tmp[15];
int main(){
int n;
scanf("%d", &n);
for(int i = 0; i < n; ++i) scanf("%d", &roots[i]);
for(int i = 0; i < n; ++i) roots[i] = -roots[i];
coeff[1] = 1;
coeff[0] = roots[0];
for(int i = 1; i < n; ++i){
for(int j = 0; j <= i; ++j) tmp[j] = coeff[j];
for(int j = i + 1; j > 0; --j) coeff[j] = coeff[j - 1];
coeff[0] = 0;
for(int j = 0; j <= i; ++j) coeff[j] += roots[i] * tmp[j];
}
for(int i = n - 1; i >= 0; --i){
if(i == 0) printf("%d\n", coeff[i]);
else printf("%d ", coeff[i]);
}
return 0;
}
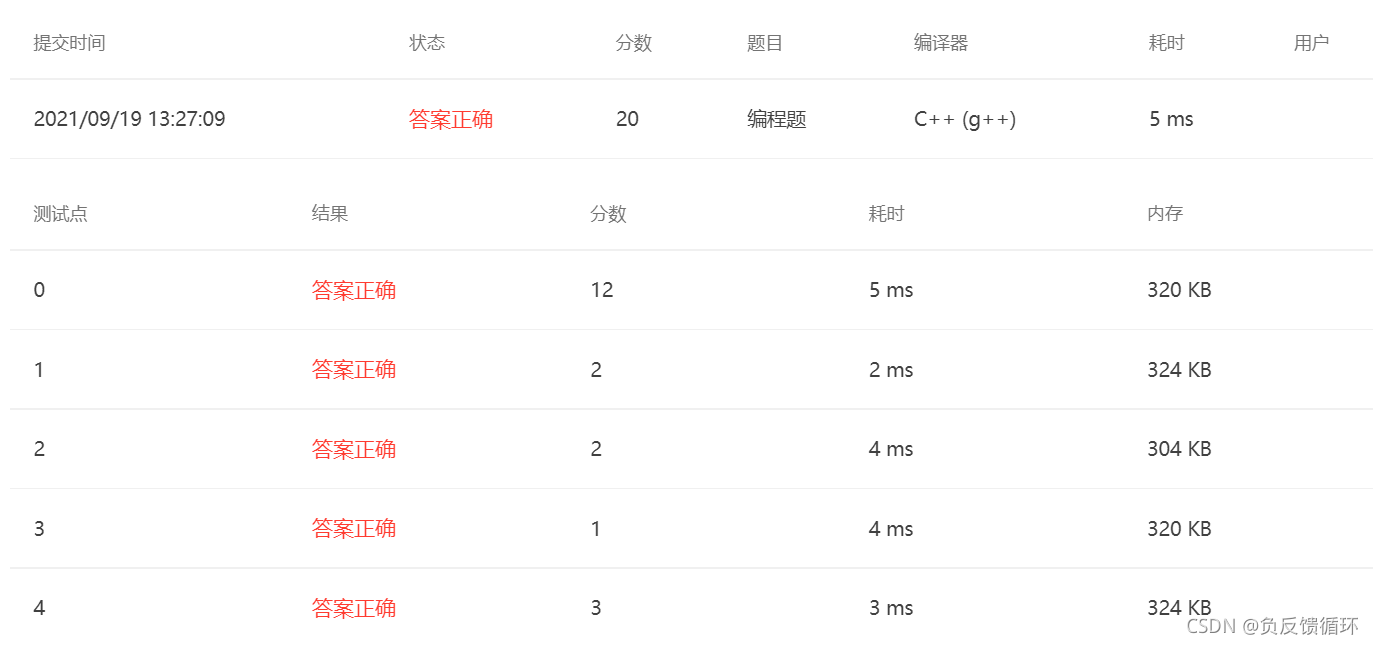
|