引用:C++实现线程安全的单例模式 - myd620 - 博客园
单例模式-地鼠文档
5. 单例模式 — Graphic Design Patterns
单例模式(Singleton Pattern):单例模式确保某一个类只有一个实例,而且自行实例化并向整个系统提供这个实例,这个类称为单例类,它提供全局访问的方法。
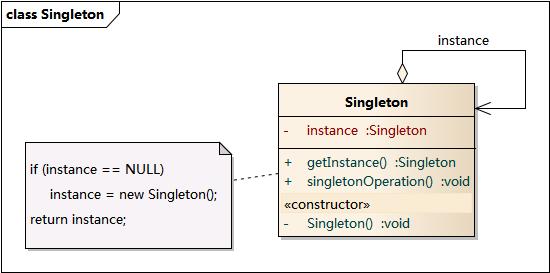
一、go语言版
package main import "sync" import "fmt" type Singleton struct{} var singleton *Singleton var once sync.Once func GetInstance() *Singleton { ?? ?once.Do(func(){ ?? ??? ?singleton = &Singleton{} ?? ?}) ?? ?return ?singleton? } func main() { ?? ?ins1 := GetInstance() ?? ?ins2 := GetInstance() ?? ?if ins1 == ins2 { ?? ??? ?fmt.Printf("instance is equel\n") ?? ?} }
二、c++语言版
#include <iostream> #include <pthread.h> using namespace std; template <class T> class singleton { private: ? ? singleton(); ? ? singleton(const singleton&){}; ? ? singleton &operator=(const singleton&){}; ? ? static T* m_instance; ? ? static pthread_once_t m_once; public: ? ? static void init(); ? ? static T* GetInstance(); }; class Test { public: ? ? Test() { ? ? ? ? cout << "test construct" << endl; ? ? } ? ? void set(int a) { ? ? ? ? cout << "test show" << endl; ? ? ? ? a = a_; ? ? } ? ? int get() const { ? ? ? ? return a_; ? ? } private: ? ? int a_; }; template <class T> void singleton<T>::init() { ? ? cout << "init " << endl; ? ? ? m_instance = new T(); } template <class T> T *singleton<T>::GetInstance() { ? ? pthread_once(&m_once, init); ? ? return m_instance; } template <class T> pthread_once_t singleton<T>::m_once = PTHREAD_ONCE_INIT; template <class T> T *singleton<T>::m_instance = NULL; int main() { ? ? Test *t1 = singleton<Test>::GetInstance(); ? ? Test *t2 = singleton<Test>::GetInstance(); ? ?? ? ? cout << "addread t1 " << static_cast<void *>(t1) << endl; ? ? cout << "addread t2 " << static_cast<void *>(t2) << endl; ? ? ? ? return 0; }
三、c语言版
#include <stdio.h> #include <pthread.h> #include <malloc.h> #include <stdlib.h> typedef struct _DATA { ? ? int a; ? ? void *data; }DATA; DATA *data; pthread_once_t m_once; void init() { ? ? data = (DATA *)malloc(sizeof(DATA)); ? ?if(NULL == data) { ? ? ? ?printf("init failed\n"); ? ? ? ?exit(-1); ? ?} else { ? ? ? ?printf("init success\n"); ? ?} } DATA *GetInstance() { ? ? pthread_once(&m_once, init); ? ? return data; } int main() { ? ? DATA *data,*data2; ? ? data = GetInstance(); ? ? data->a = 10; ? ? data2 = GetInstance(); ? ? printf("data1=%d data2=%d\n",data->a, data2->a); ? ? return 0; }
|