1. std::thread创建和使用
#include <thread>
#include <iostream>
#include <string>
#include <chrono>
using namespace std;
void func(int num, string str)
{
for (int i = 0; i < 10; ++i)
{
cout << "子线程: i = " << i << "num: "
<< num << ", str: " << str << endl;
}
}
void func1()
{
for (int i = 0; i < 10; ++i)
{
cout << "子线程: i = " << i << endl;
}
}
int main()
{
cout << "主线程的线程ID: " << this_thread::get_id() << endl;
thread t(func, 520, "i love you");
thread t1(func1);
cout << "线程t 的线程ID: " << t.get_id() << endl;
cout << "线程t1的线程ID: " << t1.get_id() << endl;
return 0;
}
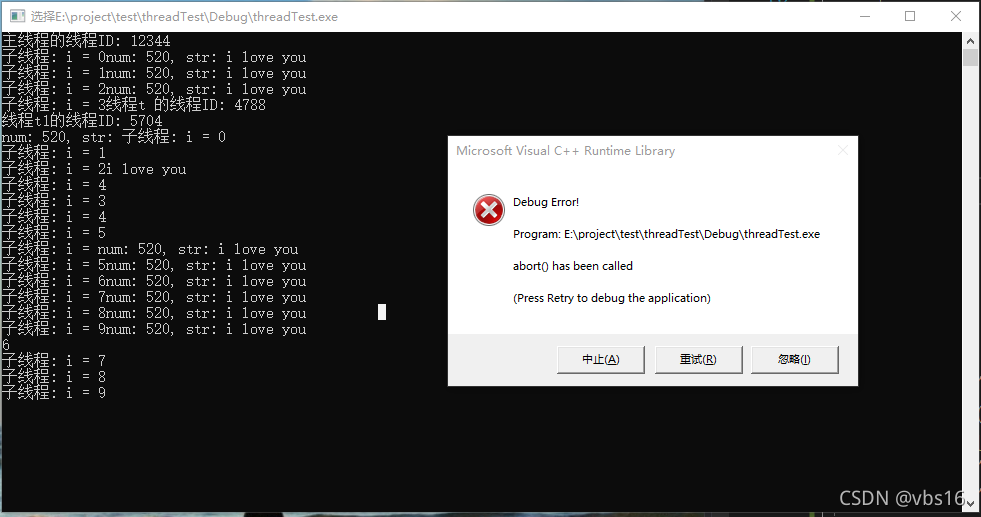
2. join()
阻塞当前线程直至 *this 所标识的线程结束其执行。
int main()
{
cout << "主线程的线程ID: " << this_thread::get_id() << endl;
thread t(func, 520, "i love you");
thread t1(func1);
cout << "线程t 的线程ID: " << t.get_id() << endl;
cout << "线程t1的线程ID: " << t1.get_id() << endl;
t.join();
t1.join();
return 0;
}
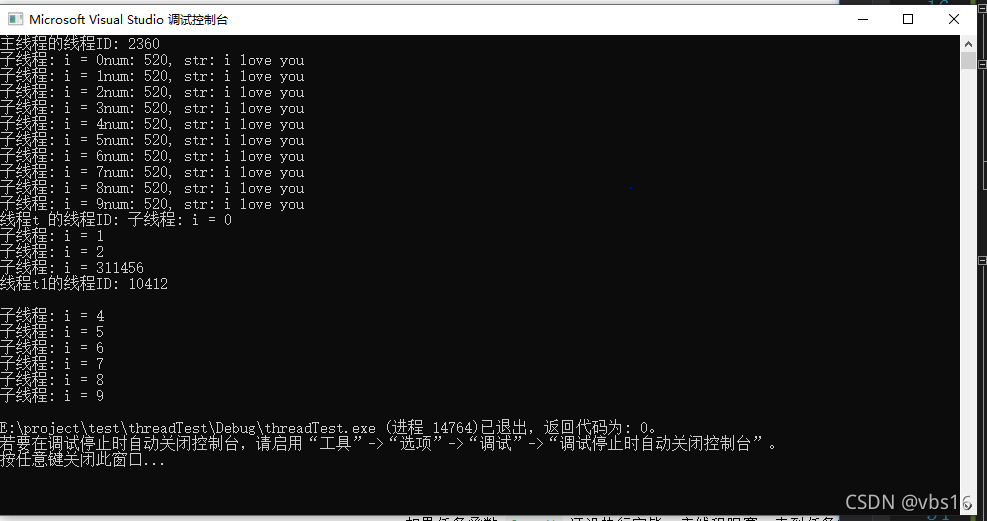
3. detach()
从 thread 对象分离执行线程,允许执行独立地持续。一旦该线程退出,则释放任何分配的资源。 调用 detach 后 *this 不再占有任何线程。
int main()
{
cout << "主线程的线程ID: " << this_thread::get_id() << endl;
thread t(func, 520, "i love you");
thread t1(func1);
cout << "线程t 的线程ID: " << t.get_id() << endl;
cout << "线程t1的线程ID: " << t1.get_id() << endl;
t.detach();
t1.detach();
this_thread::sleep_for(chrono::seconds(5));
}
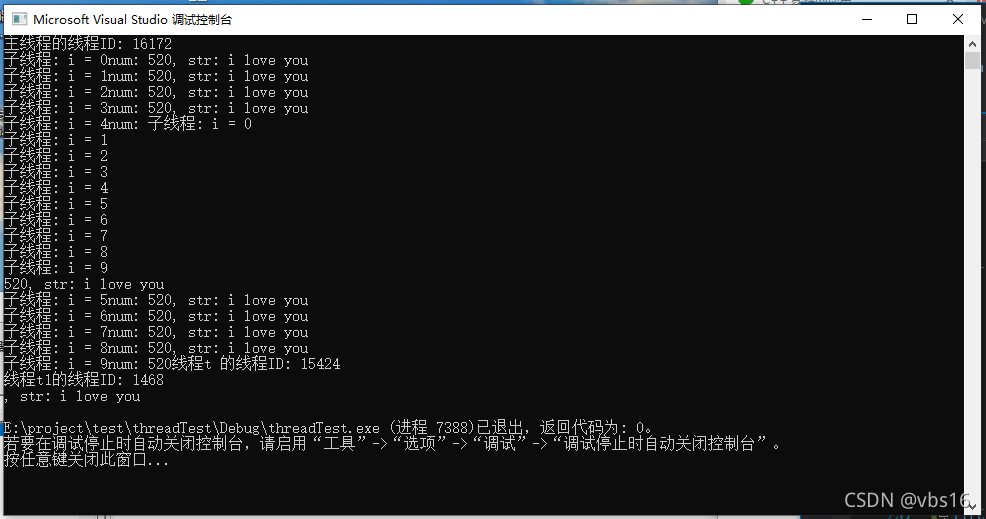
4. joinable()
检查 std::thread 对象是否标识活跃的执行线程。具体而言,若 get_id() != std::thread::id() 则返回 true 。故默认构造的 thread 不可结合。 结束执行代码,但仍未结合的线程仍被当作活跃的执行线程,从而可结合。
#include <iostream>
#include <thread>
#include <chrono>
void foo()
{
std::this_thread::sleep_for(std::chrono::seconds(1));
}
int main()
{
std::thread t;
std::cout << "before starting, joinable: " << std::boolalpha << t.joinable()
<< '\n';
t = std::thread(foo);
std::cout << "after starting, joinable: " << t.joinable()
<< '\n';
t.join();
std::cout << "after joining, joinable: " << t.joinable()
<< '\n';
}
输出
before starting, joinable: false
after starting, joinable: true
after joining, joinable: false
5. 静态函数 hardware_concurrency
int main()
{
int num = thread::hardware_concurrency();
cout << "CPU number: " << num << endl;
}
输出
CPU NUMBER: 8
参考
高效程序员 C++ 线程的使用 cppreference.com std::thread
|