??我们选择两个字符二维数组,第一个二维数组用来表示雷的状态,’1‘表示此位置有雷,’0‘表示此位置没有雷;另一个二维数组负责展示扫雷情况,每个位置上的数代表它周围8个格子中雷的个数,最初这个二位数组全用*填满。
??要模拟的和网页版扫雷功能类似,难点在于如果扫到的位置不是雷且周围雷的个数是0,自动扫周围的格子,然后同理往下递归。
??所以实现这个功能我们自然而然想到递归,发现展开的值是0时自动展开周围的8个格子,但是这里的痛点在于它可能会造成反复展开同一格子的问题造成死递归,对于这个痛点,我们定义一个二维整形数组state,0表示次位置开没有被展开过,1表示这个位置已经被展开过了。
??还有一个小痛点就是如果边界的位置展开后是0,那么在访问周围元素的时候可能会越界,这个我们加上一个if(在边界内)就可以解决了。
??实现代码如下:
game.h
#pragma once
#include <stdio.h>
#include <Windows.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define ROW 9
#define COL 9
#define ROWS ROW+2
#define COLS COL+2
#define COUNT 10
void game();
void Initboard(char board[ROWS][COLS], int rows, int cols, char set);
void Displayboard(char board[ROWS][COLS], int row, int col);
void setmine(char board[ROWS][COLS], int row, int col);
void Findmine(char mine[ROWS][COLS],char show[ROWS][COLS], int row, int col);
int Getminecount(char mine[ROWS][COLS], int x, int y);
void mark(char show[ROWS][COLS], int row, int col);
void unfoldneighbor(char show[ROWS][COLS], char mine[ROWS][COLS], int row, int col, int x, int y,int state[][COLS]);
void unfoldone(char show[ROWS][COLS], char mine[ROWS][COLS], int row, int col, int x, int y, int state[][COLS]);
game.c
#include "game.h"
void game()
{
char mine[ROWS][COLS] = { 0 };
char show[ROWS][COLS] = { 0 };
Initboard(mine, ROWS, COLS, '0');
Initboard(show, ROWS, COLS, '*');
Displayboard(show, ROW, COL);
setmine(mine, ROW, COL);
Findmine(mine, show, ROW, COL);
}
void Initboard(char board[ROWS][COLS], int rows, int cols, char set)
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
board[i][j] = set;
}
}
}
void Displayboard(char board[ROWS][COLS], int row, int col)
{
printf("-------------------------\n");
printf(" ");
for (int i = 1; i <= col; i++)
{
printf("%d ", i);
}
printf("\n");
for (int i = 1; i <= row; i++)
{
printf("%d ", i);
for (int j = 1; j <= col; j++)
{
printf("%c ", board[i][j]);
}
printf("\n");
}
}
void setmine(char board[ROWS][COLS], int row, int col)
{
int count = COUNT;
while (count)
{
int x = rand() % row + 1;
int y = rand() % col + 1;
if (board[x][y] != '1')
{
board[x][y] = '1';
count--;
}
}
}
int Getminecount(char mine[ROWS][COLS], int x, int y)
{
int ret = 0;
for (int i = x - 1; i <= x + 1; i++)
{
for (int j = y - 1; j <= y + 1; j++)
{
if (mine[i][j] == '1')
{
ret++;
}
}
}
return ret;
}
void Findmine(char mine[ROWS][COLS],char show[ROWS][COLS], int row, int col)
{
int x, y;
int win = 0;
int state[ROWS][COLS] = { 0 };
while (win<COL*ROW-COUNT)
{
char whethermark[10] = { 0 };
printf("请输入扫雷的坐标->");
scanf("%d %d", &x, &y);
if (x >= 1 && x <= ROW && y >= 1 && y <= COL)
{
if (mine[x][y] == '1')
{
printf("很不幸,你被炸死了\n");
Displayboard(mine, ROW, COL);
break;
}
else
{
int count = Getminecount(mine, x, y);
if (count != 0)
{
win += 1;
unfoldone(show, mine, ROW, COL, x, y,state);
system("cls");
Displayboard(show, ROW, COL);
Sleep(2000);
}
else
{
unfoldneighbor(show, mine, row, col, x, y,state);
int nums = 0;
for (int i = 1; i <= ROW ; i++)
{
for (int j = 1; j <= COL; j++)
{
if (state[i][j] == 1)
{
nums++;
}
}
}
win = nums;
system("cls");
Displayboard(show, ROW, COL);
Sleep(2000);
}
while (1)
{
printf("是否要标记雷?YES OR NO\n");
scanf("%s", whethermark);
if (strcmp(whethermark, "YES") == 0)
{
mark(show, ROW, COL);
}
else
{
break;
}
}
}
}
else
{
printf("输入错误请重新输入\n");
}
}
if (win == COL * ROW - COUNT)
{
printf("恭喜你扫雷成功!\n");
printf("再来一把?\n");
}
}
void mark(char show[ROWS][COLS], int row, int col)
{
int x, y;
target:
printf("请输入要标记为雷的坐标->");
scanf("%d %d", &x, &y);
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
system("cls");
show[x][y] = '#';
Displayboard(show, ROW, COL);
return;
}
else
{
printf("输入错误 请重新输入\n");
goto target;
}
}
void unfoldone(char show[ROWS][COLS], char mine[ROWS][COLS], int row, int col, int x, int y,int state[][COLS])
{
if (state[x][y] == 0)
{
state[x][y] = 1;
int count = Getminecount(mine, x, y);
show[x][y] = '0' + count;
}
}
void unfoldneighbor(char show[ROWS][COLS], char mine[ROWS][COLS], int row, int col,int x,int y,int state[][COLS])
{
if (state[x][y] == 0)
{
state[x][y] = 1;
int count1 = Getminecount(mine, x, y);
show[x][y] = '0' + count1;
for (int i = x - 1; i <= x + 1; i++)
{
for (int j = y - 1; j <= y + 1; j++)
{
if (i >= 1 && i <= ROW && j >= 1 && j <= COL)
{
if (state[i][j] == 0)
{
int count = Getminecount(mine, i, j);
if (count != 0)
{
unfoldone(show, mine, row, col, i, j, state);
}
else
{
unfoldneighbor(show, mine, row, col, i, j, state);
}
}
}
}
}
}
else
{
return;
}
}
void rule()
{
printf("这是一个扫雷游戏\n");
printf("操作注意:\n");
printf("当你要输入扫雷的坐标是,请以\"a b\"形式输入\n");
printf("当你要标记某位置为雷时,请输入YES然后回车\n");
printf("如果不标记雷的位置,请输入NO然后回车\n");
printf("千万不要在此步输入带空格的输入会导致后续输入混乱\n");
printf("游戏功能与胜负规则:\n");
printf("当扫到的位置没有雷,展示的数字表明在此位置周围八个格子中类的个数\n");
printf("本游戏模拟网页版本扫雷,为提升游戏效率,如果扫到的位置周围没有雷,会自动扫周围的位置直到遇到周围有雷的位置位置\n");
printf("当你把所有的非雷位置都扫掉以后,游戏将获得胜利\n");
printf("祝您游戏愉快\n");
}
test.c
#include "game.h"
void menu()
{
printf("*****************************\n");
printf("*****************************\n");
printf("*******这是一个扫雷游戏******\n");
printf("*****************************\n");
printf("********1.play 2.rule********\n");
printf("*****************************\n");
printf("***********0.exit************\n");
printf("*****************************\n");
}
int main()
{
int input = 0;
srand((unsigned)time(NULL));
do
{
menu();
printf("请输入->");
scanf("%d", &input);
switch (input)
{
case 0:printf("退出游戏\n"); break;
case 1:system("cls"); game(); break;
case 2:system("cls"); rule(); break;
default:printf("输入错误请重新输入\n"); break;
}
} while (input);
return 0;
}
功能测试
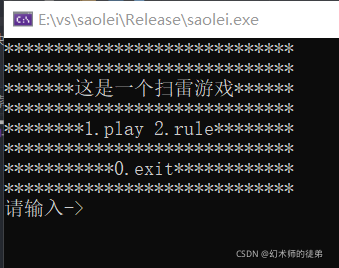 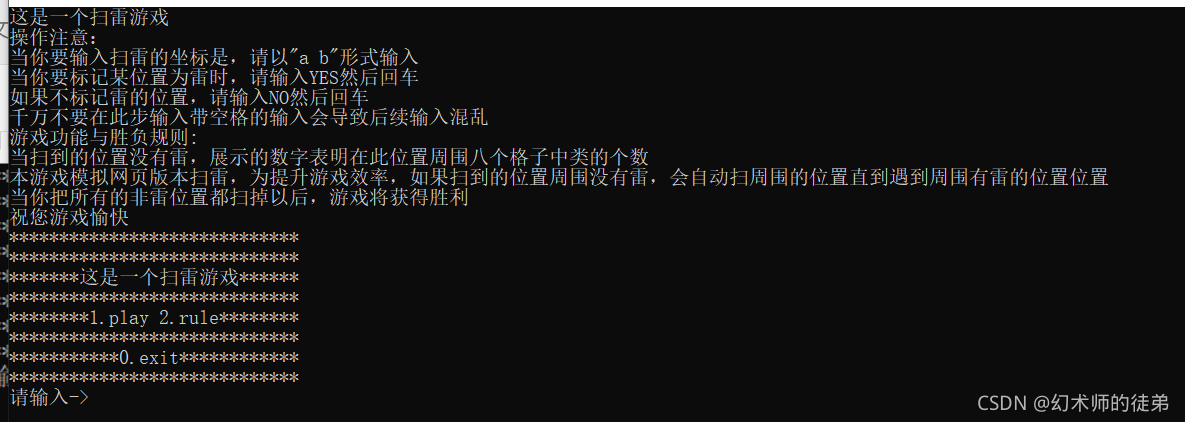 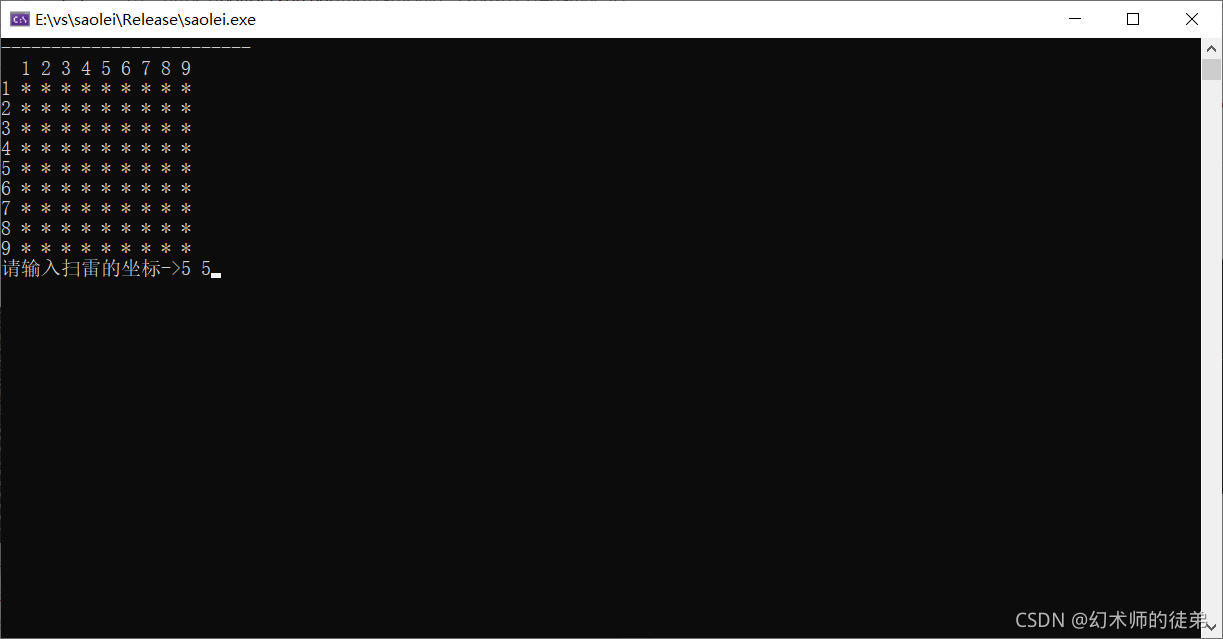 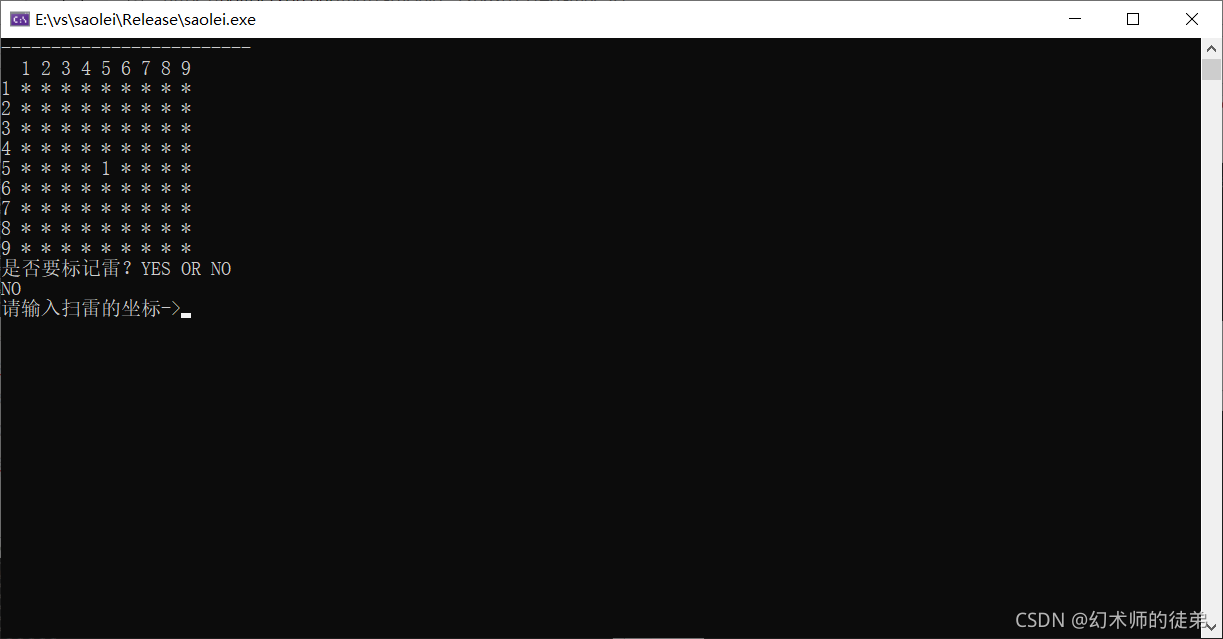 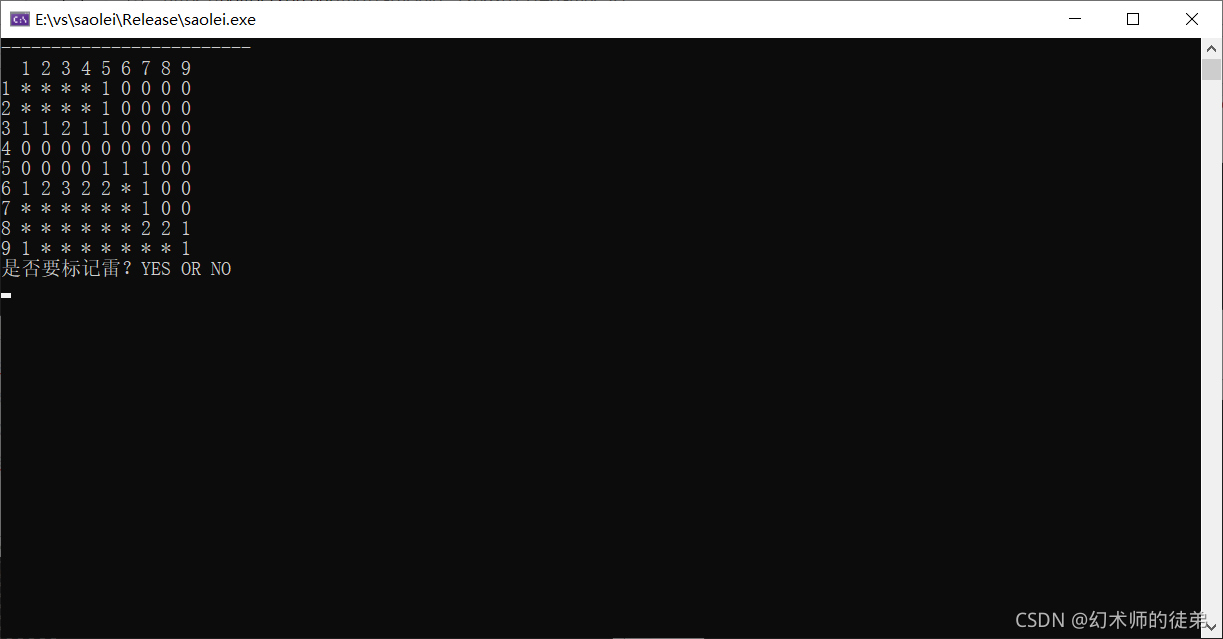
|