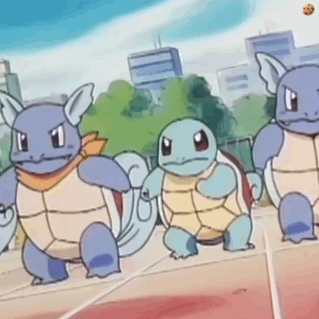
系列文章目录
前言
掌握常见特殊类的设计方式。
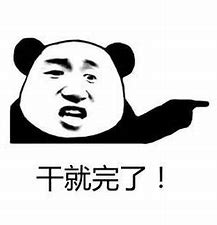
一、请设计一个类,只能在堆上创建对象
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
#include<memory>
using namespace std;
class HeapOnly
{
public:
static HeapOnly* GetObj()
{
return new HeapOnly;
}
private:
HeapOnly()
{}
HeapOnly(HeapOnly&);
HeapOnly(HeapOnly&) = delete;
};
int main()
{
std::shared_ptr<HeapOnly> sp1(HeapOnly::GetObj());
return 0;
}
二、请设计一个类,只能在栈上创建对象
- 方法一:同上将构造函数私有化,然后设计静态方法创建对象返回即可。
- 方法二:屏蔽new,因为new在底层调用void* operator new(size_t size)函数,只需将该函数屏蔽掉即可。注意:也要防止定位new。
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
using namespace std;
class StackOnly
{
public:
static StackOnly GetObj()
{
return StackOnly();
}
private:
StackOnly(){}
void* operator new(size_t size) = delete;
};
int main()
{
StackOnly p = StackOnly::GetObj();
return 0;
}
三、请设计一个类,不能被拷贝
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
using namespace std;
class CopyBan
{
public:
CopyBan()
{}
private:
CopyBan(const CopyBan&);
CopyBan operator=(const CopyBan&);
CopyBan(const CopyBan&) = delete;
CopyBan operator=(const CopyBan&) = delete;
};
int main()
{
CopyBan a;
CopyBan b(a);
CopyBan c;
c = a;
return 0;
}
四、请设计一个类,不能被继承
C++98:中构造函数私有化,派生类中调不到基类的构造函数。则无法继承。 C++11:方法final关键字,final修饰类,表示该类不能被继承。
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
using namespace std;
class NoInherit final
{
public:
static NoInherit GetInstance()
{
return NoInherit();
}
private:
NoInherit()
{}
};
int main()
{
NoInherit a = NoInherit::GetInstance();
return 0;
}
五、请设计一个类,只能创建一个对象(单例模式)
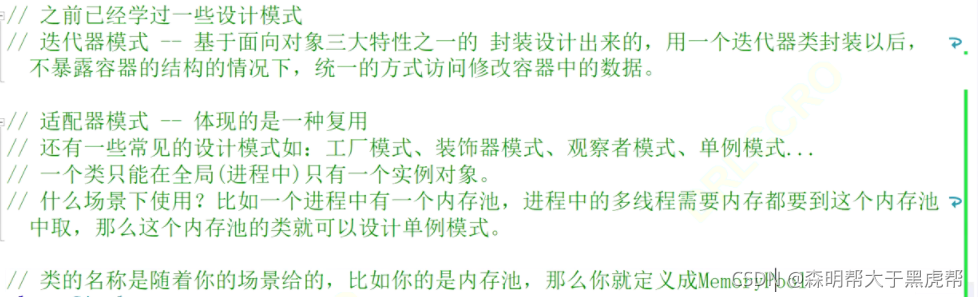 设计模式:
设计模式( Design Pattern)是 一套被反复使用、多数人知晓的、经过分类的、代码设计经验的总结。 为什么会产生设计模式这样的东西呢?就像人类历史发展会产生兵法。最开始部落之间打仗时都是人拼人的对砍。后来春秋战国时期,七国之间经常打仗,就发现打仗也是有套路的,后来孙子就总结出了《孙子兵法》。孙子兵法也是类似。
单例模式:
一个类只能创建一个对象,即单例模式,该模式可以保证系统中该类只有一个实例,并提供一个访问它的全局访问点,该实例被所有程序模块共享。 比如在某个服务器程序中,该服务器的配置信息存放在一个文件中,这些配置数据由一个单例对象统一读取,然后服务进程中的其他对象再通过这个单例对象获取这些配置信息,这种方式简化了在复杂环境下的配置管理。
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
#include<thread>
#include<vector>
#include<Windows.h>
using namespace std;
class Singleton
{
public:
static Singleton* GetInstance()
{
Sleep(1000);
if (_pinst == nullptr)
{
_pinst = new Singleton;
}
return _pinst;
}
private:
Singleton()
{}
Singleton(const Singleton&);
Singleton& operator=(const Singleton&);
static Singleton* _pinst;
};
Singleton* Singleton::_pinst = nullptr;
int main()
{
vector<std::thread> vthread;
int n = 10;
for (int i = 0; i < n; i++)
{
vthread.push_back(thread([]()
{
cout << Singleton::GetInstance() << endl;
}));
}
for (auto& t : vthread)
{
t.join();
}
return 0;
}
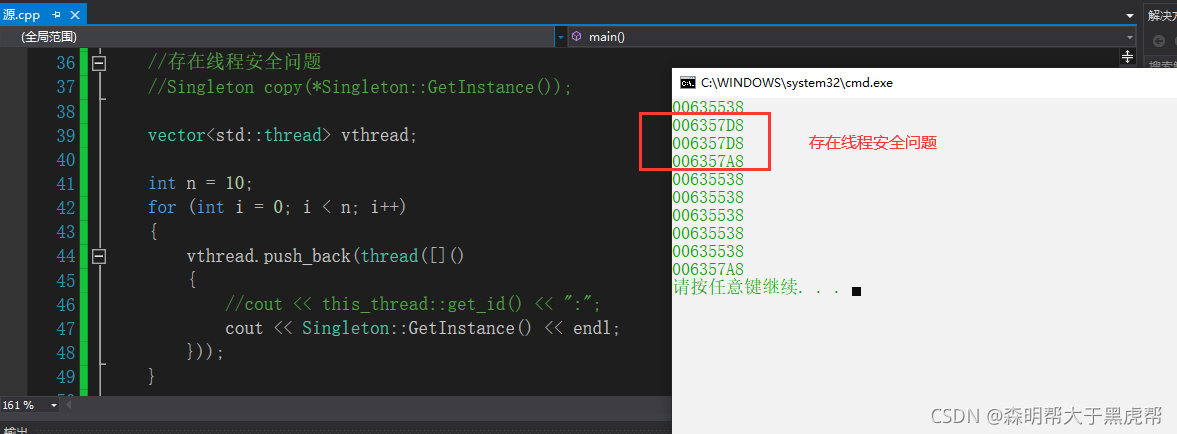
1.单例模式之饿汉模式
饿汉模式
优点:简单
缺点:可能会导致进程启动慢,且如果有多个单例类对象实例启动顺序不确定
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
#include<vector>
#include<thread>
using namespace std;
namespace yyw
{
class Singleton
{
public:
static Singleton* GetInstance()
{
return &_inst;
}
Singleton(const Singleton&) = delete;
Singleton& operator=(const Singleton&) = delete;
private:
Singleton()
{}
static Singleton _inst;
};
Singleton Singleton::_inst;
int x()
{
vector<std::thread> vthread;
int n = 10;
for (int i = 0; i < n; i++)
{
vthread.push_back(thread([]()
{
cout << Singleton::GetInstance() << endl;
}));
}
for (auto& t : vthread)
{
t.join();
}
return 0;
}
}
int main()
{
yyw::x();
return 0;
}
2.单例模式之懒汉模式
懒汉
优点:第一次使用实例对象时,创建对象。进程启动无负载。多个单例实例启动顺序自由控制。
缺点:复杂
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
#include<thread>
#include<vector>
#include<Windows.h>
#include<mutex>
using namespace std;
namespace Lazy_MAN
{
class Singleton
{
public:
static Singleton* GetInstance()
{
Sleep(1000);
if (_pinst == nullptr)
{
unique_lock<mutex> lock(_mtx);
if (_pinst == nullptr)
{
_pinst = new Singleton;
}
}
return _pinst;
}
static void DelInstance()
{
delete _pinst;
_pinst = nullptr;
}
private:
Singleton()
{}
Singleton(const Singleton&);
Singleton& operator=(const Singleton&);
static Singleton* _pinst;
static mutex _mtx;
};
class GC
{
public:
~GC()
{
Singleton::DelInstance();
}
};
static GC gc;
Singleton* Singleton::_pinst = nullptr;
mutex Singleton::_mtx;
int main()
{
vector<std::thread> vthread;
int n = 10;
for (int i = 0; i < n; i++)
{
vthread.push_back(thread([]()
{
cout << Singleton::GetInstance() << endl;
}));
}
for (auto& t : vthread)
{
t.join();
}
return 0;
}
}

总结
以上就是今天要讲的内容,本文介绍了一些特殊类设计以及单例模式,特殊类在面试中经常出现,我们务必掌握。另外如果上述有任何问题,请懂哥指教,不过没关系,主要是自己能坚持,更希望有一起学习的同学可以帮我指正,但是如果可以请温柔一点跟我讲,爱与和平是永远的主题,爱各位了。 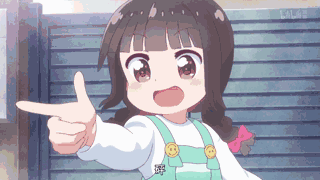
|