EX1
#include <iostream>
using namespace std;
class point
{
int x;
int y;
public:
point():x(1),y(1){}
point(int a, int b):x(a),y(b){}
int getx(){return x;}
int gety(){return y;}
};
int main()
{
point* p[2] = {new point(1, 2), new point(3, 4)};
point p1(3,3);
point p2[3]={{2,2},{3,3},{4,4}};
point p3[3];
point* pt = &p1;
point* pi = p3;
for (int i=0;i<2;i++)
{
cout<<"p["<<i<<"].x="<<p2[i].getx()<<"\t";
cout<<"p["<<i<<"].y="<<p2[i].gety()<<endl;
}
cout<<"point pt -> x:"<<pt->getx()<<endl;
cout<<"point (*pt).x:"<<(*pt).getx()<<endl;
}
EX2
#include "iostream"
using namespace std;
class A
{
int i;
public:
A(int k = 0)
{
i = k;
}
void display()
{
cout << "i=" << i << endl;
}
};
int main()
{
A *p = new A;
p->display();
delete p;
p = new A(8);
p->display();
delete p;
p = new A[3];
A *q = p;
for (int i = 0; i < 3; i++)
{
(q++)->display();
}
delete[] p;
return 0;
}
EX3
#include <iostream>
using namespace std;
class Student
{
int num;
float score;
public:
Student(int n, float s) : num(n), score(s) {}
void display();
};
void Student::display()
{
cout << num << " " << score << endl;
}
int main()
{
Student stud[5] = {
Student(101, 78.5), Student(102, 85.5), Student(103, 98.5),
Student(104, 100.0), Student(105, 95.5)};
Student *p = stud;
for (int i=0;i<=2;p=p+2,i++)
p->display();
return 0;
}
注意
-
对象数组中的元素必须是同一个类的对象 -
生成对象数组时,将针对每个数组元素按其下标的排列顺序依次调用一个构造函数。 -
定义对象数组时可以直接初始化,也可以通过赋值语句进行赋值。 -
对象数组在定义过程中进行元素初始化时调用有参构造函数;如果只定义而不进行初始化,则调用无参构造函数。 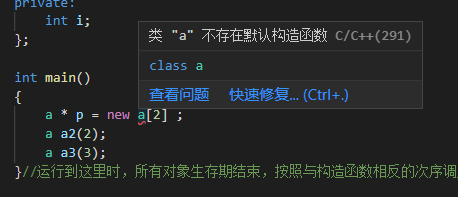 没初始化,调用无参构造函数。 -
对数组元素赋值时(用赋值语句),系统先调用有参构造函数创建无名对象,并将其值赋给响应对象数组元素,最后再调用析构函数将无名释放。
|