首先我将这个题用递归方法先实现一下,再改成非递归
递归编程实现:
#include<iostream>
using namespace std;
#define TElemType char
typedef struct BiTNode{
TElemType data;
struct BiTNode *lchild,*rchild;
}BiTNode,*BiTree;
void CreateBiTree(BiTree &T)
{
TElemType ch;
cin>>ch;
if(ch=='#')T=NULL;
else
{
T=new BiTNode;
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
}
int Depth(BiTree &T)
{
int m,n;
if(T==NULL)return 0;
else
{
m=Depth(T->lchild);
n=Depth(T->rchild);
if(m>n)return (m+1);
else return (n+1);
}
}
void DestroyTree(BiTree &T)
{
if(T!=NULL)
{
DestroyTree(T->lchild);
DestroyTree(T->rchild);
printf("销毁结点: %c\n",T->data);
delete T;
T=NULL;
}
return;
}
int main()
{
BiTree T=NULL;
printf("先序建立二叉树:\n");
CreateBiTree(T);
printf("\n此二叉树深度为:\n%d\n",Depth(T));
DestroyTree(T);
printf("\n成功销毁二叉树!\n");
return 0;
}
现在在此基础上,写出非递归的代码:
#include<iostream>
using namespace std;
#define TElemType char
typedef struct BiTNode{
TElemType data;
struct BiTNode *lchild,*rchild;
}BiTNode,*BiTree;
void CreateBiTree(BiTree &T)
{
char ch;
cin>>ch;
if(ch=='#')T=NULL;
else
{
T=new BiTNode;
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
}
#define MAXQSIZE 100
#define QElemType BiTNode*
typedef struct
{
QElemType *base;
int front;
int rear;
}SqQueue;
bool InitQueue(SqQueue &Q)
{
Q.base=new QElemType[MAXQSIZE];
if(!Q.base)exit(EOVERFLOW);
Q.front=Q.rear=0;
return true;
}
int QueueLength(SqQueue Q)
{
return (Q.rear-Q.front+MAXQSIZE)%MAXQSIZE;
}
bool EnQueue(SqQueue &Q,QElemType e)
{
if((Q.rear+1)%MAXQSIZE==Q.front)return false;
Q.base[Q.rear]=e;
Q.rear=(Q.rear+1)%MAXQSIZE;
return true;
}
bool DeQueue(SqQueue &Q,QElemType &e)
{
if(Q.front==Q.rear)return false;
e=Q.base[Q.front];
Q.front=(Q.front+1)%MAXQSIZE;
return true;
}
int DepthTree(BiTree &T)
{
SqQueue Q;
InitQueue(Q);
int high=0;
BiTNode* p=T;
QElemType e;
if(p)EnQueue(Q,p);
while(Q.front!=Q.rear)
{
high++;
int length=QueueLength(Q);
for(int i=0;i<length;i++)
{
DeQueue(Q,e);
if(e->lchild)EnQueue(Q,e->lchild);
if(e->rchild)EnQueue(Q,e->rchild);
}
}
delete Q.base;
Q.base=NULL;
return high;
}
void DestroyTree(BiTree &T)
{
if(T!=NULL)
{
DestroyTree(T->lchild);
DestroyTree(T->rchild);
printf("销毁结点: %c\n",T->data);
delete T;
T=NULL;
}
return;
}
int main()
{
BiTree T=NULL;
printf("先序建立二叉树:\n");
CreateBiTree(T);
printf("\n此二叉树深度为:\n%d\n",DepthTree(T));
DestroyTree(T);
printf("\n成功销毁二叉树!\n");
return 0;
}
我的算法思想:利用队列实现非递归,首先将每一层所有结点的地址作为元素入队,之后逐个出队并将其左右孩子入队,直到队列中都是下一层的元素,上述操作大多是在函数中的while循环内完成的(祖先结点地址入队除外),而在循环的一开始首先要判读队列是否为空,如果不为空表明队列中加入了新的一层,那么层数就要加一,最后的层数即为树的高度。
给出后一种非递归算法的运行截图: 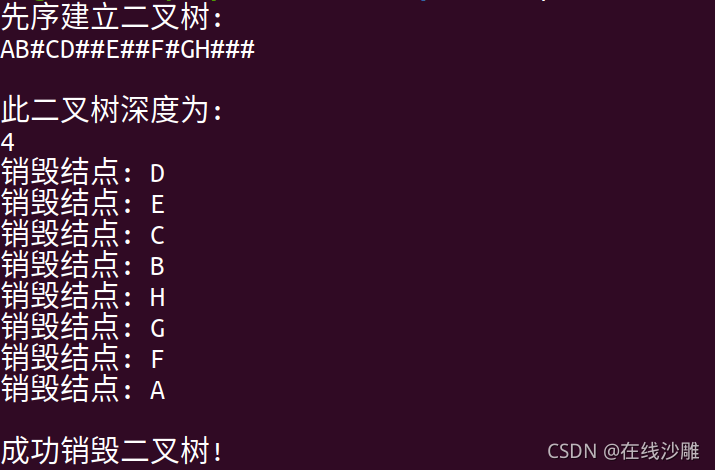
|