1、ctype.h
ctype.h头文件中定义了一系列对字符的数据类型的判断 更多函数请参考:http://c.biancheng.net/ref/isalnum.html
#include <stdio.h>
#include <ctype.h>
#define PRINTF(format, ...) printf("("__FILE__":%d) %s: "format,__LINE__,__FUNCTION__, ##__VA_ARGS__)
#define PRINT_INT(value) PRINTF(#value":%d \n", value)
#define PRINT_CHAR(value) PRINTF(#value":%c \n", value)
int main(){
PRINT_INT(isalnum('A'));
PRINT_INT(isalpha('A'));
PRINT_INT(isblank(' '));
PRINT_INT(iscntrl('\t'));
PRINT_INT(isdigit('a'));
PRINT_CHAR(tolower('A'));
PRINT_CHAR(toupper('a'));
return 0;
}
#ifndef stdio_h
#include <stdio.h>
#endif
#ifndef ctype_h
#include <ctype.h>
#endif
#define PRINTLN(format) printf("%s %d:"format"\n",__FUNCTION__,__LINE__)
#define PRINTFLN(format, ...) printf(#format":"format"\n", ##__VA_ARGS__)
int main(){
PRINTLN("检测参数字符是否为数字或字母");
printf("%d\n", isalnum('a'));
printf("%d\n", isalnum('B'));
printf("%d\n", isalnum('2'));
printf("%d\n", isalnum('-'));
PRINTLN("检测参数字符是否为字母");
printf("%d\n", isalpha('2'));
printf("%d\n", isalpha('a'));
printf("%d\n", isalpha('A'));
PRINTLN("检测参数字符是否为十进制数字");
printf("%d\n",isdigit('a'));
printf("%d\n",isdigit('1'));
PRINTLN("检测参数字符是否为十六进制数字");
printf("%d\n",isxdigit('1'));
printf("%d\n",isxdigit('A'));
printf("%d\n",isxdigit('K'));
PRINTLN("检测所传字符是否为小写字母");
printf("%d\n",islower('a'));
printf("%d\n",islower('B'));
printf("%d\n",islower('2'));
PRINTLN("检测所传字符是否为大写字母");
printf("%d\n",isupper('A'));
printf("%d\n",isupper('a'));
printf("%d\n",isupper('2'));
PRINTLN("检测参数是否为标点符号");
printf("%d\n",ispunct('c'));
printf("%d\n",ispunct('2'));
printf("%d\n",ispunct('A'));
printf("%d\n",ispunct('.'));
PRINTLN("检测参数是否为空白字符");
printf("%d\n",isspace(' '));
printf("%d\n",isspace(' '));
printf("%d\n",isspace('a'));
return 0;
}
2、stdlib.h
stdlib.h 头文件中定义了一些变量和宏以及函数。 详细可参考:这里
2.1、atoX系列函数
其中atoX(const char *str) 系列函数拥有将字符串转换类型的功能。
#ifndef stdio_h
#include <stdio.h>
#endif
#ifndef stdlib_h
#include <stdlib.h>
#endif
#define PRINTF(format, ...) printf(format, ##__VA_ARGS__)
#define PRINTLN(value) PRINTF(value"\n")
#define PRINTF_F(value) PRINTF(#value": %f\n", value)
#define PRINTF_I(value) PRINTF(#value": %i\n", value)
int main(){
PRINTLN("把参数字符串转为一个浮点数(类型为double)");
PRINTF_F(atof("1"));
PRINTF_F(atof("1.2a"));
PRINTF_F(atof("a"));
PRINTF_F(atof("Y"));
PRINTLN("把参数字符串转为一个整数");
PRINTF_I(atoi("a"));
PRINTF_I(atoi("2.22"));
PRINTF_I(atoi("3"));
PRINTLN("把参数转为long int");
PRINTF_I(atol("3"));
return 0;
}
上方代码调用结果如下 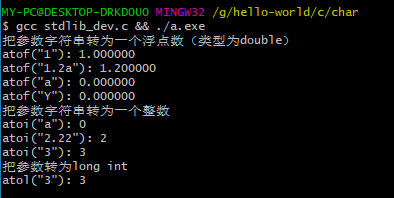
2.2、strtoX系列函数
strtoX(const char *str, char **endptr) 相关的函数功能和atoX 系列函数功能差不多,但是可重复解析,更安全,更强大
|