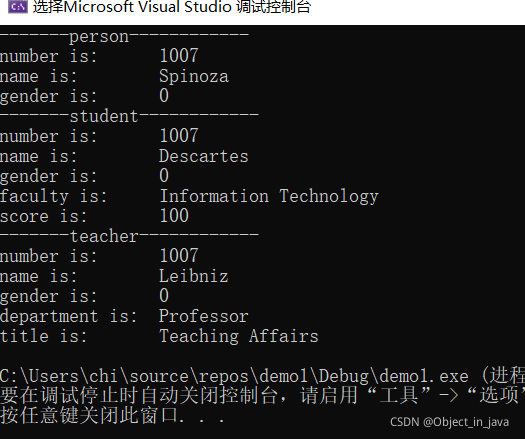
#include<iostream>
#include<time.h>
#include<math.h>
#include<string.h>
using namespace std;
enum Gender_ {
Male,
Female
};
class Person
{
public:
Person(){}
Person(int number,string name,Gender_ gender){
number_ = number;
name_ = name;
gender_ = gender;
}
~Person(){}
void Print() {
cout << "number is:\t" <<number_ <<endl;
cout << "name is:\t" <<name_<<endl;
cout << "gender is:\t" <<gender_ <<endl;
}
protected:
string name_;
int number_;
Gender_ gender_;
};
class Student: public Person{
public:
Student() {}
Student(int number, string name, Gender_ gender, string faculty, int score):Person(number,name,gender) {
number_ = number;
name_ = name;
gender_ = gender;
faculty_ = faculty;
score_ = score;
}
~Student(){}
void Print() {
Person::Print();
cout << "faculty is:\t" << faculty_ << endl;
cout << "score is:\t" << score_ << endl;
}
protected:
string faculty_;
int score_;
};
class Teacher : public Person {
public:
Teacher(){}
Teacher(int number, string name, Gender_ gender, string department, string title) :Person(number, name, gender) {
department_ = department;
title_ = title;
}
void Print() {
Person::Print();
cout << "department is:\t" << department_ << endl;
cout << "title is:\t" << title_ << endl;
}
protected:
string department_;
string title_;
};
int main() {
Person person(1007, "Spinoza", Male);
Student student(1007, "Descartes", Male, "Information Technology",100);
Teacher teacher(1007, "Leibniz", Male, "Professor", "Teaching Affairs");
cout << "-------person------------\n";
person.Print();
cout << "-------student------------\n";
student.Print();
cout << "-------teacher------------\n";
teacher.Print();
return 0;
}
|