一、什么是C语言
二、第一个C语言程序
三、数据类型
四、变量、常量
五、字符串+转义字符+注释
六、选择语句
七、循环语句
八、函数
九、数组
十、操作符
十一、常见关键字
十二、define定义常量和宏
十三、指针
十四、结构体
一、什么是C语言
计算机语言:C、C++、JAVA、Python......
C语言是一种常见的计算机语言!是人和计算机交流的语言。
C语言是一种通用的计算机编程语言,广泛地运用于底层开发。具有良好的跨平台的特性,以一个标准写出的C语言程序可以在许多电脑平台上进行编译。
C语言是一门面向计算过程的计算机编程语言,其主要编译器有:Clang、GCC、WIN-TC、SUBLIME、MSVC、Turbo C等。
C语言:
? ? 1、C语言是一种底层语言
? ? 2、C语言是一种小型语言
? ? 3、C语言是一种包容性语言
C语言的优点:高效、可移植、功能强大、灵活、标准库、与UNIX系统的集成。
C语言的缺点:C语言更容易隐藏错误、C程序可能会难以理解、C程序可能会难以修改。
二、第一个C语言程序
步骤:
1、创建新项目
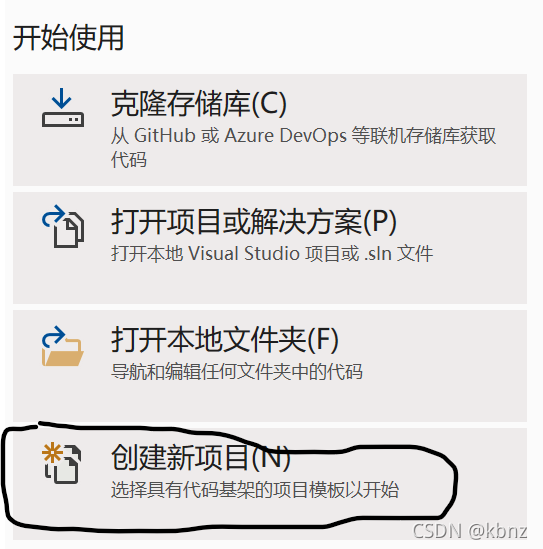
?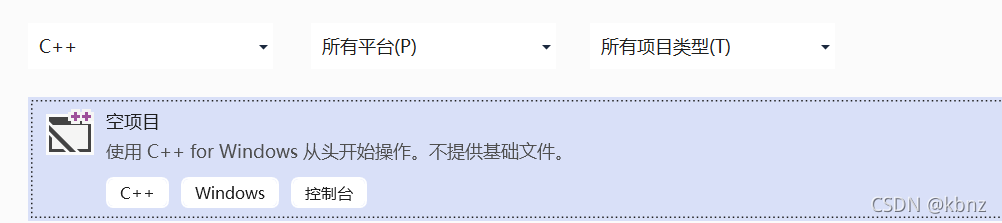
? 在源文件下创建一个.c文件
本地 Windows 调试器快捷按键:Ctrl+Fn+F5
2、写代码
#include<stdio.h>//stdio--->standard input output 标准输入输出库
int main()
{
printf("hello world\n");//printf 打印函数,使用时需要应用头文件。“”内为打印的内容,\n换行。
return 0;
}
三、数据类型
1、种类:
char? ? ? ? ? ? ? ? ? ? ? ? ?字符数据类型
short (int)? ? ? ? ? ? ? ? ?短整型
int? ? ? ? ? ? ? ? ? ? ? ? ? ? 整形
long (int)? ? ? ? ? ? ? ? ? 长整型
long long (int)? ? ? ? ? 更长的整型
float? ? ? ? ? ? ? ? ? ? ? ? ?单精度浮点数
double? ? ? ? ? ? ? ? ? ? ?双精度浮点数
2、大小
sizeof()? ?是求类型的大小,单位是字节(byte)。一个字节等于八个比特(bit)。
#include <stdio.h>
int main()
{
printf("%d\n", sizeof(char));
printf("%d\n", sizeof(short));
printf("%d\n", sizeof(int));
printf("%d\n", sizeof(long));
printf("%d\n", sizeof(long long));
printf("%d\n", sizeof(float));
printf("%d\n", sizeof(double));
return 0;
}
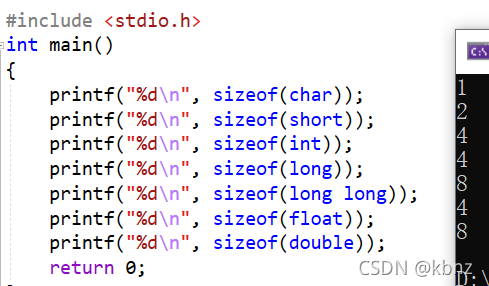
????????
#include <stdio.h>
int main()
{
char ch = 'w';
short a = 1;
int b = 2;
long c = 3;
long long d = 4;
float e = 0.2f;
double f = 0.22;
printf("%c\n", ch);//%c--->打印字符
printf("%d\n", a);//%d---->以十进制的形式打印整型
printf("%d\n", b);
printf("%d\n", c);
printf("%d\n", d);
printf("%f\n", e);//%f----->以十进制的形式打印浮点数
printf("%f\n", f);
return 0;
}
四、变量、常量
变量:
1、变量分为局部变量和全局变量。
局部变量:一般将定义在函数中的变量称为局部变量,其只能在函数内部。
全局变量:一般将定义在全局作用域中的变量,即函数外的变量,称之为全局变量。
#include <stdio.h>
int weight = 500;//定义在函数外的变量--->全局变量
int main()
{
int age = 30;//定义在函数内的变量----->局部变量
printf("%d\n", weight);
printf("%d\n", age);
return 0;
}
2、变量的作用域和生命周期
局部变量的作用域是:变量所在的局部范围。
全局变量的作用域是:整个工程。
局部变量的生命周期是:进入作用域生命周期开始,出作用域生命周期结束。
全局变量的生命周期是:整个程序的生命周期。
#include <stdio.h>
int age = 20;/
int main()
{
int build = 3;
{
int bleed = 20;//变量bleed的生命周期是在{}之内,在{}之外无法打印。
}
printf("%d\n", age);
printf("%d\n", build);
printf("%d\n", bleed);
return 0;
}

#include <stdio.h>
int age = 20;//age的生命周期是整个程序,所以在那也能打印出来。
int main()
{
int build = 3;//在与前一个大括号相同位置对应的另一个括号之内,都能打印出来
{
printf("%d\n", age);
printf("%d\n", build);
}
printf("%d\n", age);
printf("%d\n", build);
return 0;
}
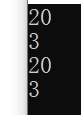
#include <stdio.h>
int a = 100;
int main()
{
int a = 10;
printf("%d\n", a);//当局部变量和全局变量一样时,局部变量优先。
return 0;
}
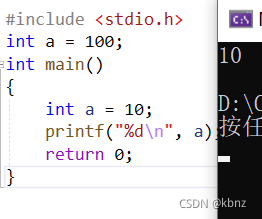
#include <stdio.h>
int a = 100;
int main()
{
printf("%d\n", a);//当局部变量在printf函数后面时,局部与全局变量一致时,全局变量优先。
int a = 200;
return 0;
}
?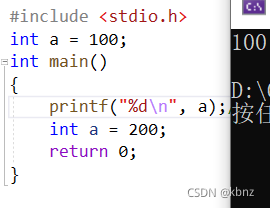
变量的使用:(计算两个数之和)
#include <stdio.h>
int main()
{
int num1 = 0;
int num2 = 0;
int sum = 0;
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("%d\n", sum);
return 0;
}
?常量:
1、常量分为:字面常量、const修饰的常变量、#define定义的标示符常量、枚举常量
字面常量:数字,0、1、2......
const修饰的常变量:const能将变量修饰为常量,使变量不被改变变,但其本质仍是常量。
#include <stdio.h>
int main()
{
const int a = 30;
printf("a = %d\n", a);
a = 50;
printf("a = %d\n", a);
return 0;
}
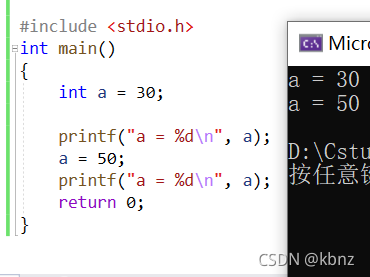
?
#include <stdio.h>
int main()
{
int a = 30;
printf("a = %d\n", a);
a = 50;
printf("a = %d\n", a);
return 0;
}
?
?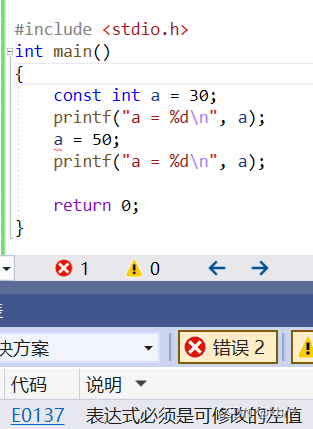
#include <stdio.h>
int main()
{
const int a = 4;
char arr[4] = {'a','b','c','\0'};
printf("%s\n", arr);
return 0;
}
?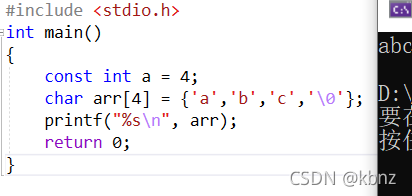
#include <stdio.h>
int main()
{
const int a = 4;
char arr[a] = { 'a','b','c','\0' };
printf("%s\n", arr);
return 0;
}
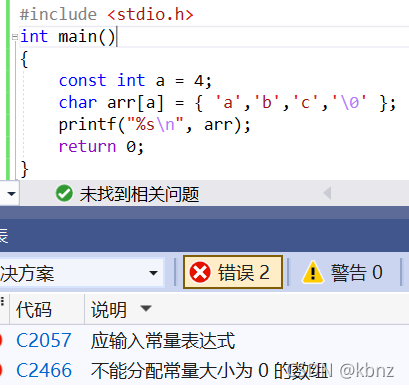
#define定义的标示符常量:就等于常量。
#include <stdio.h>
#define MALE 50
int main()
{
printf("%d\n", MALE);
char arr1[4] = { 'a','b','c','\0' };
char arr2[MALE] = { 'a','c','e','\0' };
printf("%s\n", arr1);
printf("%s\n", arr2);
return 0;
}
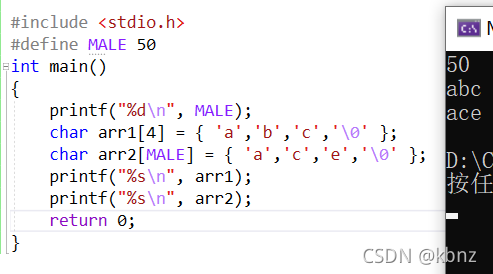
?枚举常量:一一列举出来。
#include <stdio.h>
enum Sex
{
MALE,
FEMALE,
SECRET
};
int main()
{
printf("%d\n", MALE);
printf("%d\n", FEMALE);
printf("%d\n", SECRET);
return 0;
}
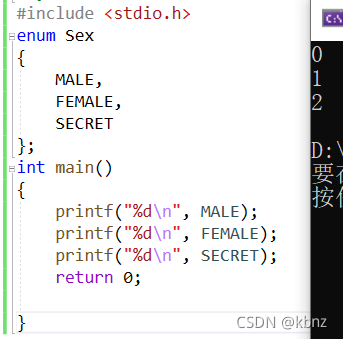
#include <stdio.h>
enum Month
{
JANUARY,
FEBRUARY,
MARCH,
APRIL,
MAY,
JUNE,
JULY,
AUGUST,
SEPTEMBER,
OCTOBER,
NOVEMBER,
DECEMBER
};
int main()
{
printf("%d\n", JANUARY);
printf("%d\n", FEBRUARY);
printf("%d\n", MARCH);
printf("%d\n", APRIL);
printf("%d\n", MAY);
printf("%d\n", JUNE);
printf("%d\n", JULY);
printf("%d\n", AUGUST);
printf("%d\n", SEPTEMBER);
printf("%d\n", OCTOBER);
printf("%d\n", NOVEMBER);
printf("%d\n", DECEMBER);
return 0;
}
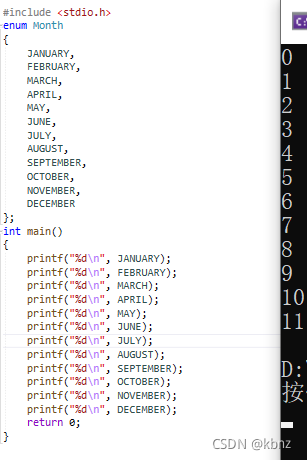
?五、字符串+转义字符+注释1
字符串:
字符串由双引号引起来,字符由单引号引起来。字符串的结束标志是\0。
1)
#include <stdio.h>
int main()
{
printf("hello world\n");
char a = 'k';
printf("%c\n",a);
return 0;
}
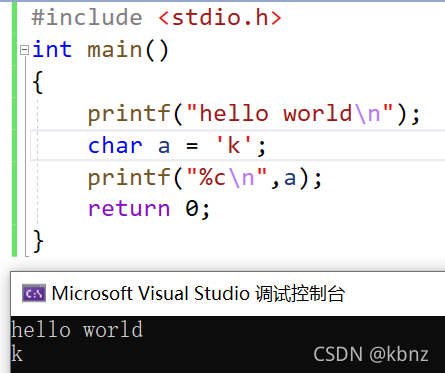
?2)
#include <stdio.h>
int main()
{
char arr1[] = "hello";
char arr2[] = { 'h','e','l','l','o' };
char arr3[] = { 'h','e','l','l','o','\0' };
printf("%s\n", arr1);
printf("%s\n", arr2);
printf("%s\n", arr3);
return 0;
}
?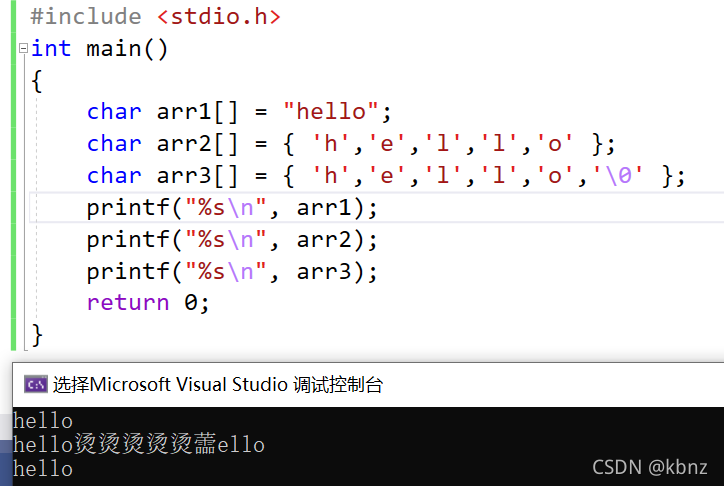
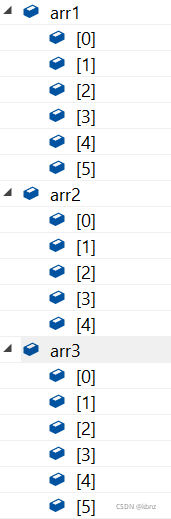
?转义字符
?\?------------->在书写连续多个问号时使用,防止他们被解析成三字母词
? ? ? ??)在有些编译器会被编译成】,加上\能防止被解析为】。
\'--------------->用于表示字符常量'
\"--------------->用于表示一个字符串内部的双引号(这个双引号与前面形成一对)
\\--------------->用于表示反斜杠,防止被解释为一个转义序列符
\a————-->警告字符,蜂鸣
\b-------------->退格符
\f--------------->进纸符
\n-------------->换行
\r--------------->回车
\t--------------->水平制表符
\v--------------->垂直制表符
\ddd------------>ddd表示一到三个八进制数字
\xdd------------->dd表示两个十六进制数字
#include <stdio.h>
int main()
{
printf("\'\n");
printf("\"\n");
printf("\\n\n");
printf("hello\bword\n");
printf("hello\fword\n");
printf("hello\rword\n");
printf("hello\tword\n");
printf("hello\vword\n");
printf("\326\n");
printf("\xa5\n");
return 0;
}
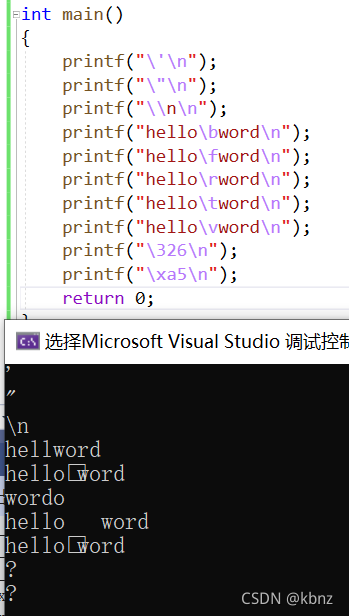
?注释
C语言注释方法:1)//? 2)/*? ? ? */
六、选择语句
if语句和switch语句
if语句:
1、if (表达式) 语句
2、if (表达式) {多条语句}
3、if (表达式) 语句 else (语句)
4、
if (表达式)
语句?
else if (表达式)
语句
...
else?
语句
5、悬空else? else与最近的if匹配
switch 语句:
switch (表达式)
{? ? ? ? ? case 常量表达式 :语句
? ? ? ? ? ?...
? ? ? ? ? ?case 常量表达式 :语句
? ? ? ? ? ?default :语句
}
七、循环语句
while语句:while(表达式)语句
//写一个平方表
#include <stdio.h>
int main()
{
int i, n = 0;
printf("This program prints a table of squares.\n");
printf("Enter number of entries in table: ");
scanf("%d", &n);
i = 1;
while (i <= n)
{
printf("%10d%10d\n", i, i * i);
i++;
}
return 0;
}
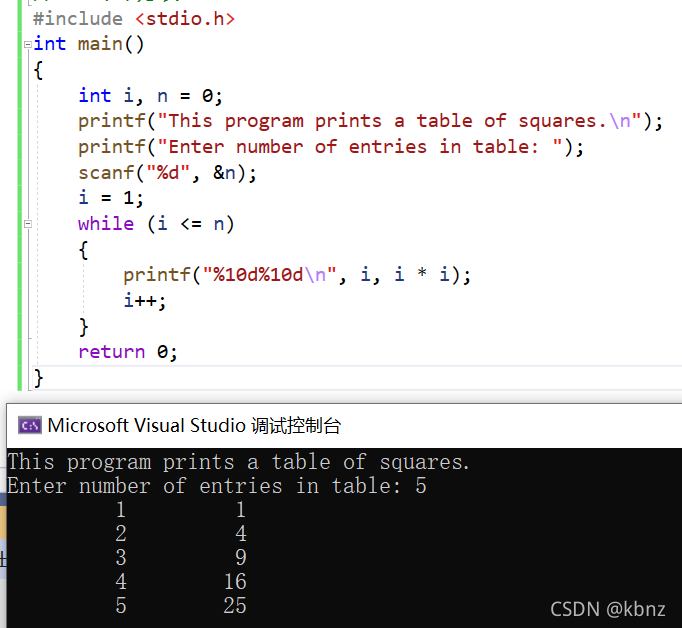
do语句:do语句 while (表达式)
#include <stdio.h>
int main()
{
int i = 10;
do
{
printf("T times %d and counting\n",i);
--i;
} while (i > 0);
return 0;
}
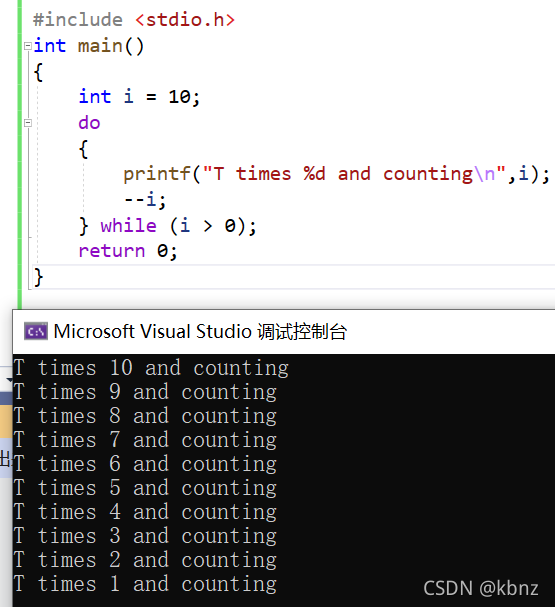
?for语句:for (表达式1;表达式2;表达式3)语句
#include <stdio.h>
int main()
{
int i = 10;
for (i = 10; i > 0; --i)
printf("T times %d and counting\n", i);
return 0;
}
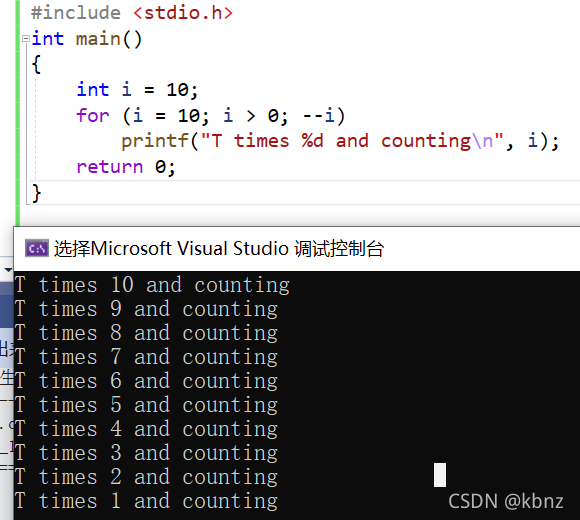
八、函数
函数:函数是C语言的构建块。每个函数本质上是一个自带声明和语句的小程序。可以利用函数把函数划分成小块,这样便于人们理解和修改程序。
函数的特点是:简化代码,代码重复。
#include <stdio.h>
int Add(int x, int y)
{
int z = x + y;
return z;
}
int main()
{
int num1, num2, sum = 0;
printf("输入两个函数值:");
scanf("%d %d", &num1, &num2);
sum = Add(num1, num2);
printf("%d\n", sum);
return 0;
}
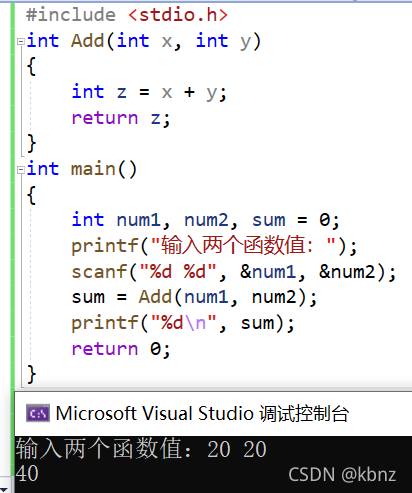
#include <stdio.h>
double average(double a, double b)
{
return (a + b) / 2;
}
int main()
{
double x, y, z = 0.00;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &x, &y, &z);
printf("Average of %g and %g : %g\n", x, y, average(x, y));
printf("Average of %g and %g : %g\n", x, z, average(x, z));
printf("Average of %g and %g : %g\n", y, z, average(y, z));
return 0;
}
?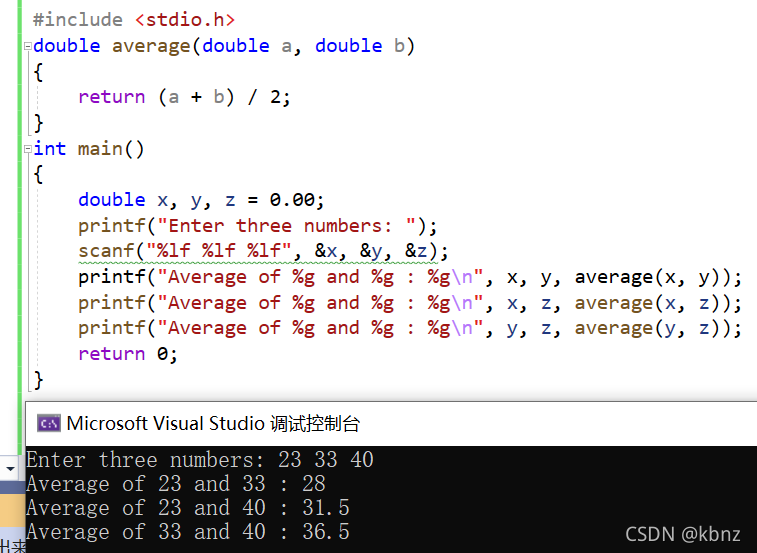
?九、数组
数组:数组是含有多个数据值的数据结构,并且每个数据值具有相同的数据类型,这些数据值称为元素,可以根据元素在数组中所处的位置把他们一个个地选出来。数组是一组相同类型元素的集合。
数组的下标: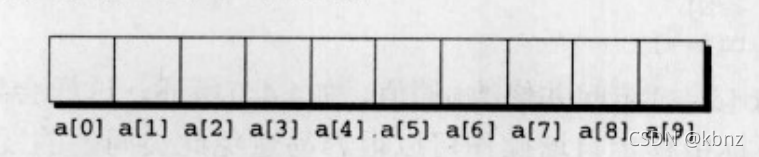
#include <stdio.h>
int main()
{
int i = 0;
int arr[10] = { 1,2,3,4,5,6,7,8,9,10 };
while (i < 10)
{
printf("%d\n", arr[i]);
i++;
}
printf("%d\n", arr[4]);
return 0;
}
?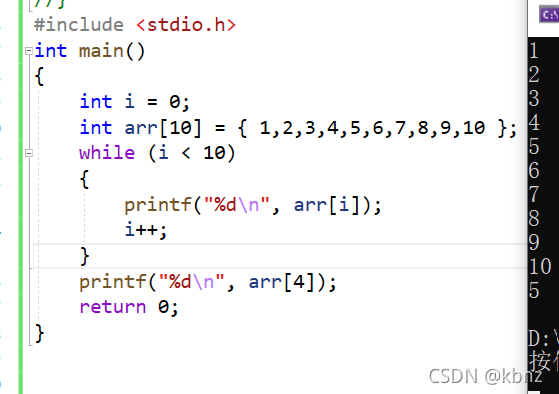
?十、操作符
算数操作符:+ - *? /(取商,整数整除结果为整数,两边有一个小数结果为小数)? %(取余数\取模,此操作符只能用于整数)
#include <stdio.h>
int main()
{
int num1, num2, sum, a, d, e = 0;
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
a = num1 - num2;
d = num1 / num2;
e = num1 % num2;
printf("%d\n%d\n%d\n%d\n", sum, a, d, e);
return 0;
}
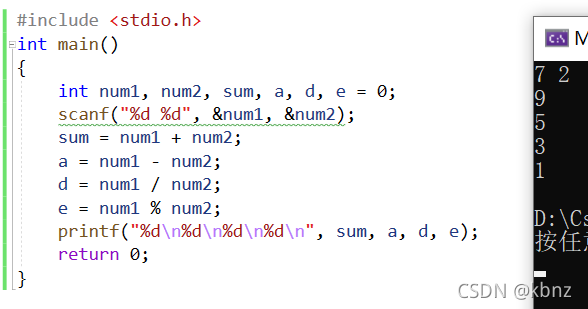
移位操作符:<<(左移位,以二进制的形式)? ? ?>>(右移位,以二进制的形式)
#include <stdio.h>
int main()
{
int a = 3; //a:00000000000000000000000000000011
int b = a >> 1;//b:00000000000000000000000000000001 向右移动一位
int c = a << 1;//c:00000000000000000000000000000110 向左移动一位
printf("b:%d\nc:%d\n", b, c);
return 0;
}
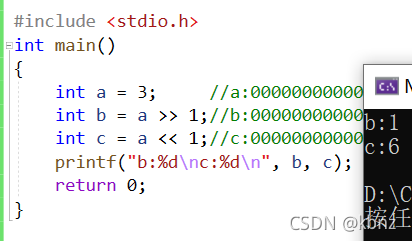
?位操作符:
& :(按位与,二进制的形式,有0为0,都为一为一)
|:(按位或,以二进制的形式,有一为一,都为0为0)?
^:(按位异或,以二进制的形式,&相同为0,不同为一)??
#include <stdio.h>
int main()
{
int a = 3; //00000000 00000000 00000000 00000011
int b = 6; //00000000 00000000 00000000 00000110
int c, d, e = 0;
c = a & b; //00000000 00000000 00000000 00000010 2
d = a | b; //00000000 00000000 00000000 00000111 7
e = a ^ b; //00000000 00000000 00000000 00000101 5
printf("c=%d\nd=%d\ne=%d\n", c, d, e);
return 0;
}
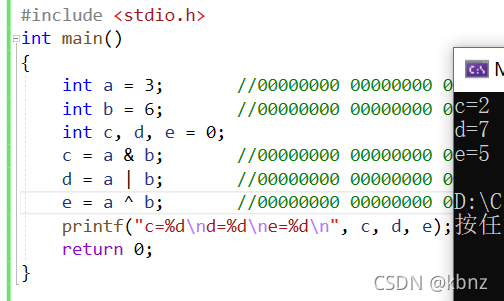
?赋值操作符:=? ?+=? ?-=? ?*=?? /=? ?&=? ? ^=? ? |=? ? >>=? ? <<=
单目操作符(只有一个操作数):
!(逻辑取反,0为假1为真)
-? ? +? ? &? ? sizeof? ?
~(按位取反,二进制的形式。负整数在二进制里有原码,反码,补码。原码1变0 0变1成为反码,反码+1为补码,补码取反)
--? (前置--? 先--后使用,后置-- 先使用后--)? ?
++? (前置++? 先++后使用,后置++? 先使用 后++)? ??
#include <stdio.h>
int main()
{
int num1 = 10;
int num2 = 10;
int num3 = 10;
int num4 = 10;
int b, c, d, e = 0;
b = ++num1;
c = num2++;
d = --num3;
e = num4--;
printf("b=%d\nc=%d\nd=%d\ne=%d\n", b, c, d, e);
return;
}
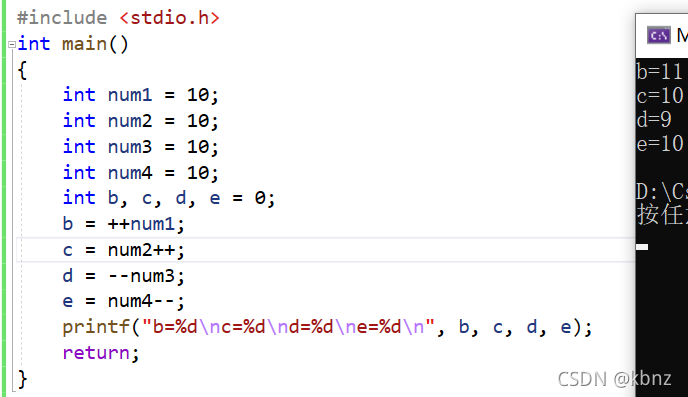
*? ? (类型)? ?
关系操作符:>? ? >=? ? <? ? <=? ? !=? ? ==
#include <stdio.h>
int main()
{
int a, b, MAX = 0;
scanf("%d %d", &a, &b);
if (a > b)
printf("MAX = %d\n", a);
else
printf("MAX = %d\n", b);
return 0;
}
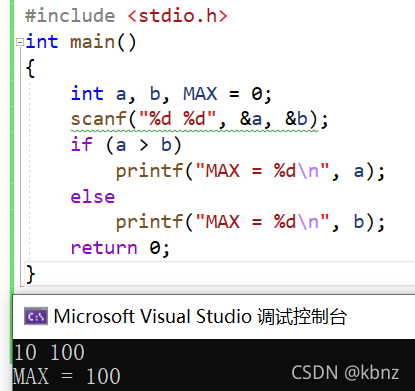
?逻辑操作符:&&(逻辑与)? ? ? ||(逻辑或)
#include <stdio.h>
int main()
{
int a, b = 0;
scanf("%d %d",&a, &b);
if (a > 10 && b > 20)
printf("abc");
else
printf("def");
return 0;
}
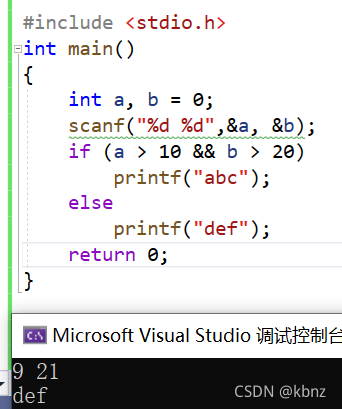
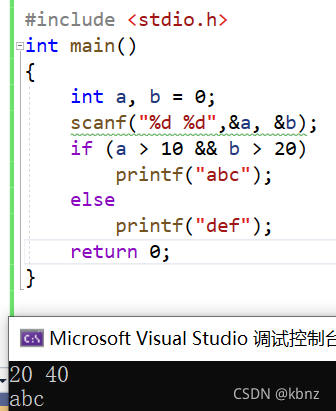
#include <stdio.h>
int main()
{
int a, b = 0;
scanf("%d %d",&a, &b);
if (a > 10 || b > 20)
printf("abc");
else
printf("def");
return 0;
}
?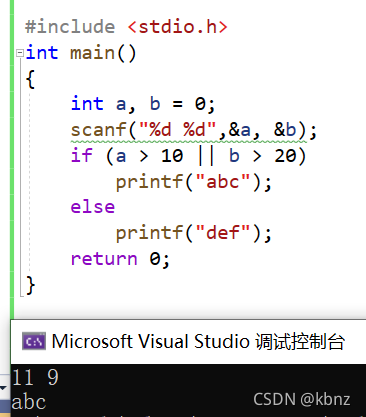
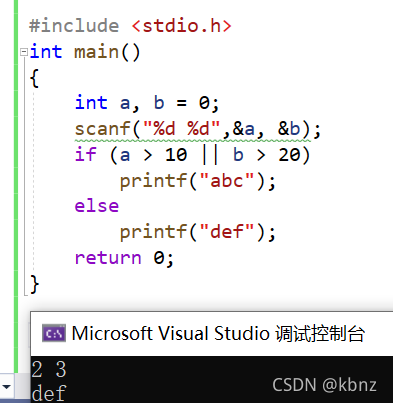
?条件操作符(三目操作符):? exp1 ? exp2 : exp3? ? ? ?(exp1:表达式。 exp2:结果为真时输出。 exp3:结果为假时输出)? ? ?
#include <stdio.h>
int main()
{
int a, b, MAX = 0;
scanf("%d %d", &a, &b);
MAX = (a > b ? a : b);
printf("%d\n", MAX);
return 0;
}
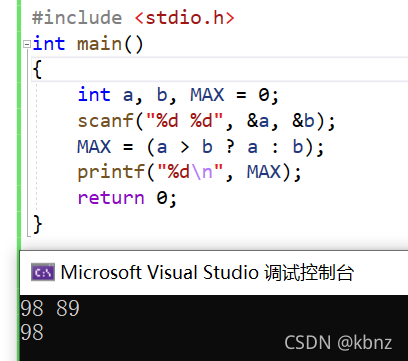
?逗号表达式:? exp1, exp2, exp3, ...expN(逗号表达式从左向右读取,结果为最后一个计算出的结果)
#include <stdio.h>
int main()
{
int a = 2;
int b = 3;
int c = 4;
int d = (b = a + 2, c = b++, a = c * b);// b = 4, c = 5, a = 20;
printf("%d\n", d);
return 0;
}
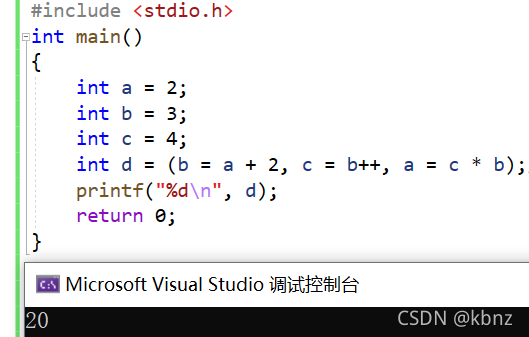
?下标引用、函数调用和结构成员:[ ]? ? ()? ? `? ? ? ->
十一、常见关键字????????
auto(自动,自动变零)? ? ? ? ? ? ? ? ? ? break(终止,在循环中)? ? ? ? ? ? ? ? ? ? ? ? ? ?case? ?
continue(用于循环,继续)? ? ? ? ?const(常属性,修饰变量,修饰指针)? ? ? char? ?? default(switch case 语句? 默认)?do? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?double? ? ? ? enum(枚举)? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? extern(声明外部符号)? ? ? ? ? ? ? ? ? ? ? ? ? ? ? float? ? ? ? ? ? ? ? ? ? ? for? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?goto? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? if? ? ? ? ? ? ? ? ? ? ? ? ?int? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?long? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?while ? ? ? ? ? ? ? ? ? ? ?register(寄存器)? ? ? ? ? ? ? ? ? ? ? ? ?return(返回,函数)? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?short? ? ? ? ? ? ? signed(有符号)? ? ? ? ? ? ? ? ? ? ? ? ? ? sizeof? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? static(静态)? ?struck(结构体)? ? ? ? ? ? ? ? ? ? ? ? ? ? switch? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? else? ? ? ? ? ? ? ? ? ?typedef(类型重定义)? ? ? ? ? ? ? ? ? ?union(联合体)? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?volatile? ? ? ? ? ? ? ? ? ?unsigned(无符号的)? ? ? ? ? ? ? ? ? ? ?void(没,空)? ? ? ? ? ? ? ? ? ? ? ?
#include <stdio.h>
typedef unsigned int u_int;//用u_int来表示 unsined int typedef 类型重定义
int main()
{
unsigned int a = 100;
u_int b = 100;
printf("%d\n%d\n", a, b);
return 0;
}
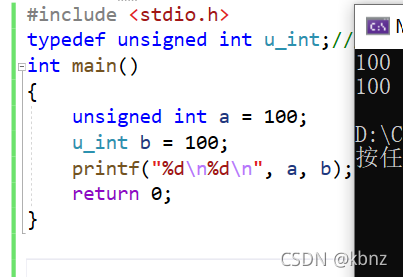
#include <stdio.h>
int main()
{
register int a = 10;//建议将a放入寄存器中,是否放入寄存器中不知道,寄存器变量不能取地址
return 0;
}
static? 修饰局部变量、全局变量、函数。
修饰局部变量时,将局部变量从栈区转换成静态区,改变变量的类型。从而使得静态的局部变量出了自己的作用域也不会被销毁,相当于改变了生命周期。
#include <stdio.h>
void test()
{
int a = 1;
a++;
printf("%d\n", a);
}
int main()
{
int i = 0;
while (i < 10)
{
test();
i++;
}
return 0;
}
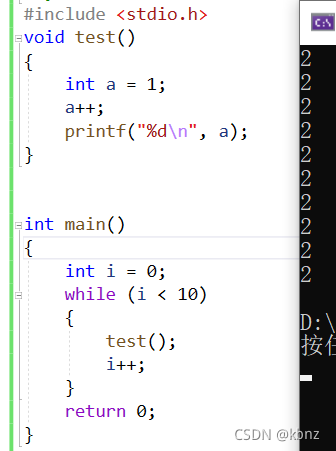
#include <stdio.h>
void test()
{
static int a = 1;
a++;
printf("%d\n", a);
}
int main()
{
int i = 0;
while (i < 10)
{
test();
i++;
}
return 0;
}
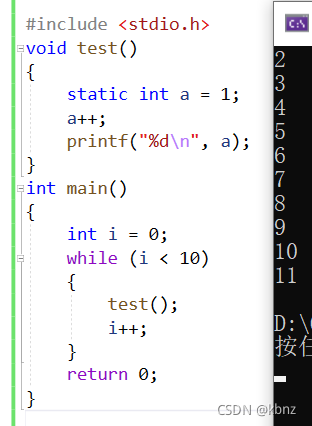
?static修饰全局变量时:全局变量只能在该源文件中选用,不能跨文件使用该变量;当一个全局变量被static修饰时,这个变量的外部链接变成内部属性,使变量只能在自己的的源文件中使用。
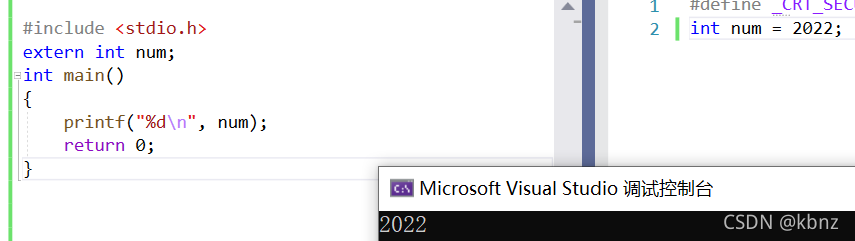
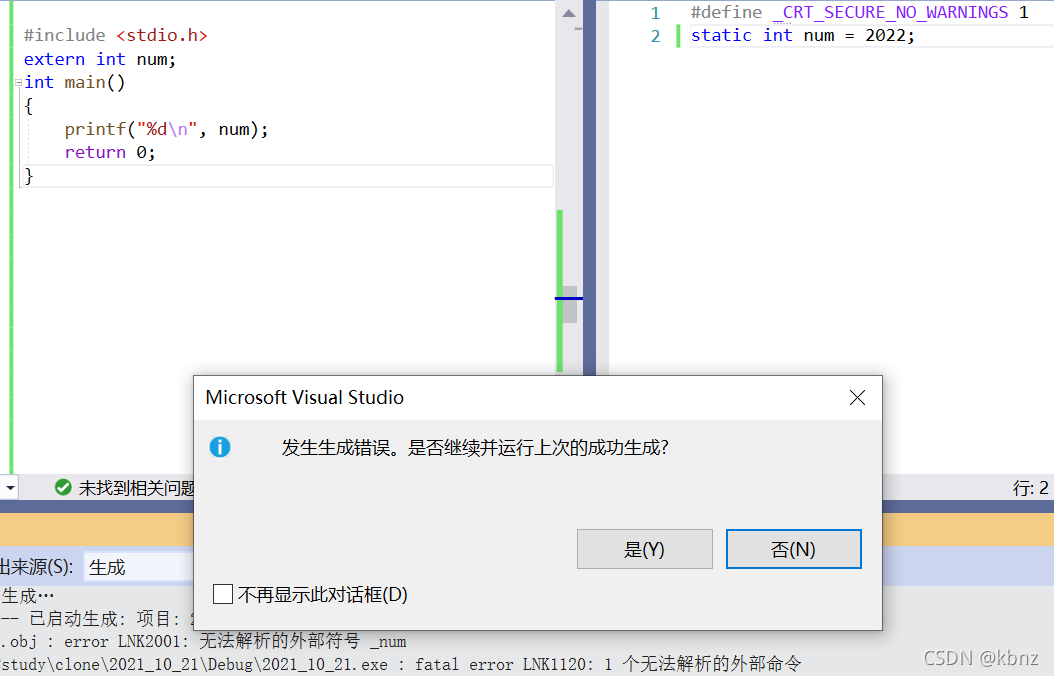
?修饰函数时与修饰全局变量一样
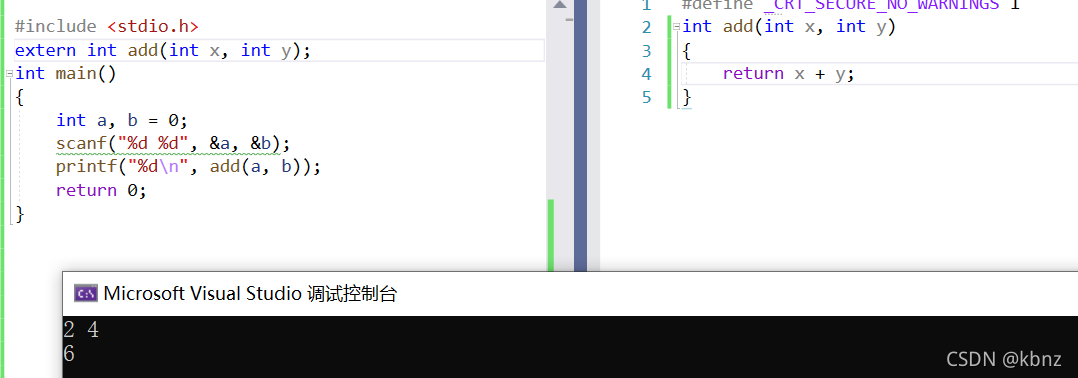
?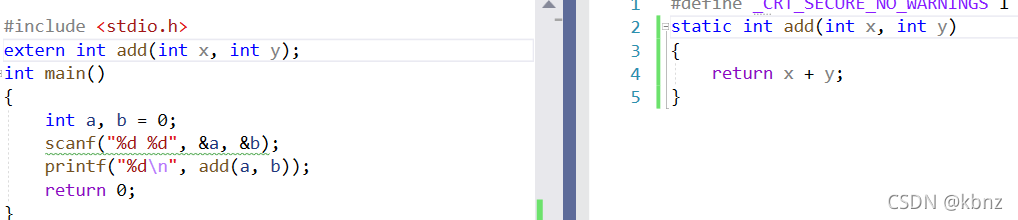

?十二、define定义常量和宏
定义常量:
#include <stdio.h>
#define M 100
#define STR "hehe"//字符串用双引号括起来
int main()
{
printf("%d\n", M);
printf("%s\n", STR);
return 0;
}
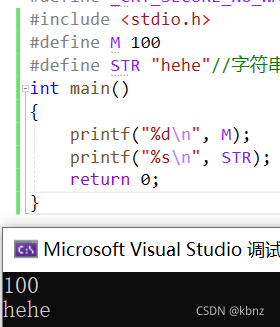
?定义宏:习惯大写
#include <stdio.h>
#define ADD(x,y) ((x) + (y))
int main()
{
int a, b = 0;
scanf("%d %d", &a, &b);
printf("%d\n", ADD(a, b));
return 0;
}
?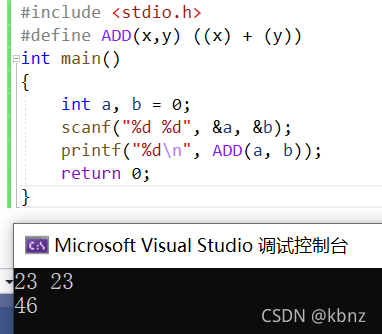
?十三、指针
计算机大都将内存分割成字节,每个字节可以存储8位的信息,每个字节都有唯一的地址,用来和内存中其他其他字节相区别。
内存是电脑上重要的存储器,计算机程序的运行都是在内存中进行的。所以为了有效地使用内存,就把内存划分成一个个小的内存单元每个内存单元的大小是1个字节。为了有效的访问到内存的每个单元,就给内存进行编号,这些编号被称为内存的地址。
在32位的操作系统是4G,在32位的操作系统中指针的变量大小是4个字节。64位的操作系统是8G,64位的操作系统指针变量的大小是8个字节。
x86是32位,x64是64位。
#include <stdio.h>
int main()
{
int a = 10;
int* p = &a;//&取地址操作符,p用来存放地址,所以把P成为执政变量。
*p = 30;//*解引用操作符,找到p中存放地质的变量,并将30这个值赋给它
printf("%p\n", &a);//%p打印地址的形式
printf("%d\n", a);
return 0;
}
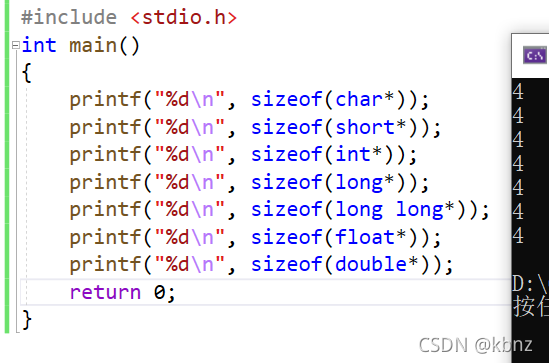
?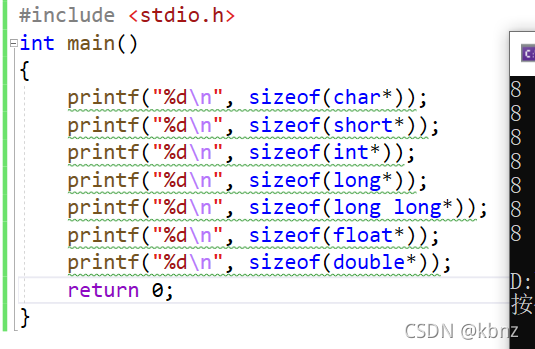
十四、结构体
结构体用来描述一些复杂的东西。
#include <stdio.h>
struct stu
{
char name[20];
int age;
char sex[10];
};
int main()
{
struct stu stu1 = { "学生1", 20, "男" };
struct stu stu2 = { "学生2", 30, "女" };
struct stu stu3 = { "学生3", 15, "保密" };
struct stu* p1 = &stu1;
struct stu* p2 = &stu2;
struct stu* p3 = &stu3;
printf("%s %d %s\n", stu1.name, stu1.age, stu1.sex);
printf("%s %d %s\n", (*p1).name, (*p1).age, (*p1).sex);
printf("%s %d %s\n", p1->name, p1->age, p1->sex);
printf("%s %d %s\n", stu2.name, stu2.age, stu2.sex);
printf("%s %d %s\n", (*p2).name, (*p2).age, (*p2).sex);
printf("%s %d %s\n", p2->name, p2->age, p2->sex);
printf("%s %d %s\n", stu3.name, stu3.age, stu3.sex);
printf("%s %d %s\n", (*p3).name, (*p3).age, (*p3).sex);
printf("%s %d %s\n", p3->name, p3->age, p3->sex);//->解引用操作符
return 0;
}
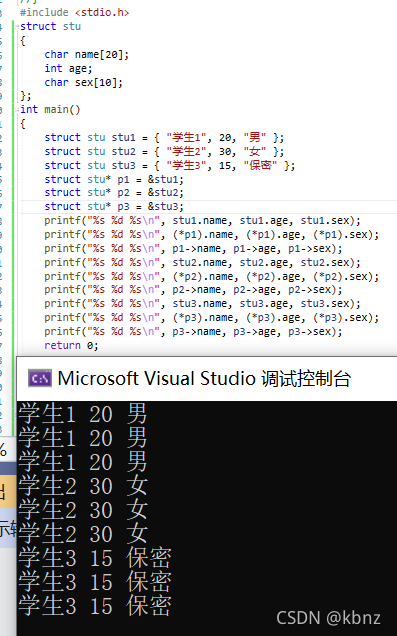
?
|