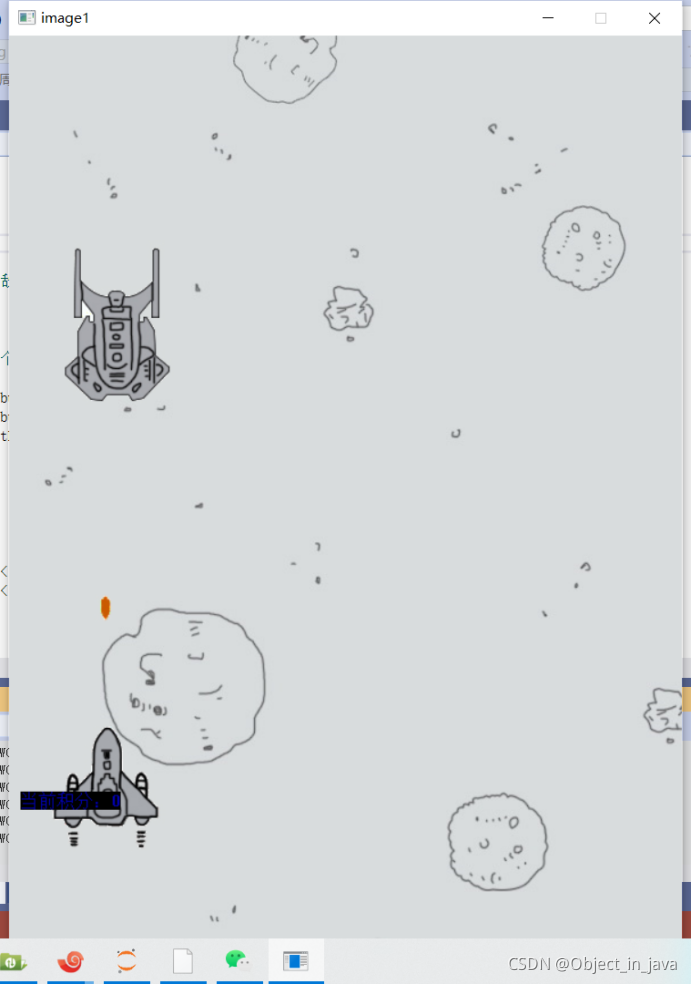
#include<graphics.h>
#include<conio.h>
#include <mmsystem.h>
#include<Windows.h>
#include<math.h>
#include<stdio.h>
#pragma comment(lib,"Winmm.lib")
class preGraph {
public:
preGraph(int w, int h)
{
initgraph(w, h);
BeginBatchDraw();
}
~preGraph()
{
EndBatchDraw();
closegraph();
}
};
IMAGE img_bk;
IMAGE img_plane_mask;
IMAGE img_plane;
IMAGE img_bullet;
IMAGE img_bullet_mask;
IMAGE img_enemyplane;
IMAGE img_enemyplane_mask;
int width;
int height;
int score;
int planeX, planeY;
int planeWidth, planeHeight;
int bulletX, bulletY;
int enemy_x, enemy_y;
int enemyWidth, enemyHeight;
int speed;
bool isExplode;
bool bulletExit;
void start()
{
mciSendString("open .\\飞机大战图片音乐素材\\game_music.mp3 alias bkmusic", NULL, 0, NULL);
mciSendString("play bkmusic repeat", NULL, 0, NULL);
loadimage(&img_bk, ".\\飞机大战图片音乐素材\\background.jpg");
width = img_bk.getwidth();
height = img_bk.getheight();
loadimage(&img_plane_mask, ".\\飞机大战图片音乐素材\\planeNormal_1.jpg");
loadimage(&img_plane, ".\\飞机大战图片音乐素材\\planeNormal_2.jpg");
planeHeight = img_plane.getheight();
planeWidth = img_plane.getwidth();
loadimage(&img_bullet_mask, ".\\飞机大战图片音乐素材\\bullet1.jpg");
loadimage(&img_bullet, ".\\飞机大战图片音乐素材\\bullet2.jpg");
loadimage(&img_enemyplane, ".\\飞机大战图片音乐素材\\enemyPlane2.jpg");
loadimage(&img_enemyplane_mask, ".\\飞机大战图片音乐素材\\enemyPlane1.jpg");
enemyHeight = img_enemyplane.getheight();
enemyWidth = img_enemyplane.getwidth();
planeX = width * 0.5 + 10;
planeY = height * 0.5 + 10;
enemy_x = width * 0.5;
enemy_y = 0;
bulletY = planeY;
bulletX = planeX;
isExplode = false;
bulletExit = false;
score = -1;
}
void show()
{
putimage(0, 0, &img_bk, SRCCOPY);
putimage(planeX, planeY, &img_plane_mask, NOTSRCERASE);
putimage(planeX, planeY, &img_plane, SRCINVERT);
putimage(enemy_x, enemy_y, &img_enemyplane_mask, NOTSRCERASE);
putimage(enemy_x, enemy_y, &img_enemyplane, SRCINVERT);
putimage(bulletX, bulletY, &img_bullet_mask, NOTSRCERASE);
putimage(bulletX, bulletY, &img_bullet, SRCINVERT);
settextcolor(BLUE);
TCHAR s[] = _T("当前积分:");
outtextxy(10, height - 200, s);
TCHAR s_12[5];
_stprintf_s(s_12, _T("%d"), score);
outtextxy(90, height - 200, s_12);
FlushBatchDraw();
Sleep(10);
}
void updateWithoutInput()
{
bulletY -= 3;
if (bulletY <= 0) {
bulletExit = false;
}
if (enemy_y < height ) {
enemy_y += 2;
}
else {
enemy_y = 0;
}
if (bulletX >= enemy_x && bulletX <= enemy_x + enemyWidth &&
bulletY >= enemy_y && bulletY <= enemy_y + enemyHeight) {
enemy_x = rand() % width;
enemy_y = -40;
bulletY = -85;
score++;
bulletExit = false;
}
if (abs(planeX - enemy_x) < planeWidth/2 + enemyWidth/2 - 10 &&
abs(planeY - enemy_y) < planeHeight/2 + enemyHeight/2 - 10) {
isExplode = true;
}
}
void updateWithInput()
{
char input;
if (_kbhit())
{
input = _getch();
switch (input)
{
case ' ':
if (bulletExit == false) {
bulletExit = true;
bulletX = planeX + img_plane.getwidth() / 2 - 17;
bulletY = planeY - img_bullet.getheight() + 5;
}
}
}
ExMessage m;
while (peekmessage(&m, EM_MOUSE, PM_NOREMOVE))
{
m = getmessage();
if (m.message == WM_MOUSEMOVE)
{
planeX = m.x - (planeWidth / 2 - 5);
planeY = m.y - (planeHeight / 2 + 30);
}
if (m.message == WM_LBUTTONDOWN && bulletExit == false)
{
bulletExit = true;
bulletX = planeX + img_plane.getwidth() / 2 - 17;
bulletY = planeY - img_bullet.getheight() + 5;
}
}
}
void lose() {
EndBatchDraw();
cleardevice();
char str[] = "Game over!";
outtextxy(width / 2 - 10, height / 2, str);
_getch();
closegraph();
}
int main()
{
start();
preGraph initgp(width, height);
while (1)
{
show();
updateWithoutInput();
updateWithInput();
if (isExplode == true) {
lose();
}
}
_getch();
return 0;
}
|