#include<iostream>
#include<bits/stdc++.h>
using namespace std;
class cube
{
int len,width,height;
public:
void set_len()
{
cout<<"请输入立方体的长度是 ";cin>>len;
}
void set_width()
{
cout<<"请输入立方体的宽度是 ";cin>>width;
}
void set_height()
{
cout<<"请输入立方体的高度是 ";cin>>height;
}
int get_len()
{
return len;
}
int get_width()
{
return width;
}
int get_height()
{
return height;
}
int get_volume()
{
return len*width*height ;
}
int get_calculate()
{
return (len*width+width*height+len*height)*2;
}
bool IS_same(cube &c2)//用引用就不用拷贝俩份值了;
{
if(height==c2.height&&width==c2.width&&len==c2.len)
{
return true;
}
return false;
}
};
int main()
{
cube c1,c2;
c1.set_height();
c1.set_len();
c1.set_width();
c2.set_height();
c2.set_len();
c2.set_width();
cout<<"两个立方体是否相等:"<<c1.IS_same(c2);
//cout<<"立方体的体积是:"<<cube1.get_volume()<<endl;
//cout<<"立方体的面积是: "<<cube1.get_calculate()<<endl;
system("pause");
}
1:
C++使用system( “pause “);来暂停黑窗口
在编写的c++程序中,如果是窗口,有时会一闪就消失了,如果不想让其消失,在程序中添加:
system(“pause”); 注意:不要再return 的语句之后加,那样就执行不到了
2:
类中的属性和行为我们统一称为 成员
属性? 成员属性 成员变量
行为 成员函数 成员方法
3:访问权限:
public? 公共权限? ? 类内类外都可以访问
protected ?保护权限? 类内可以访问类外不可以访问
private? ? 私有权限? ?类内可以访问类外不可以访问
protected private 在继承的使用上会有区别
class 默认权限中是private;
#include<iostream>
#include<bits/stdc++.h>
using namespace std;
class Student
{
public:
string M_name;
protected:
int M_passcord;
private:
string M_car;
void func()
{
cin>>M_name;
cin>>M_passcord;
cin>>M_car;//类内可以更改;
}
};
int main()
{
Student s1;
s1.M_name="yushiqi";
s1.M_car="法拉利";
}
?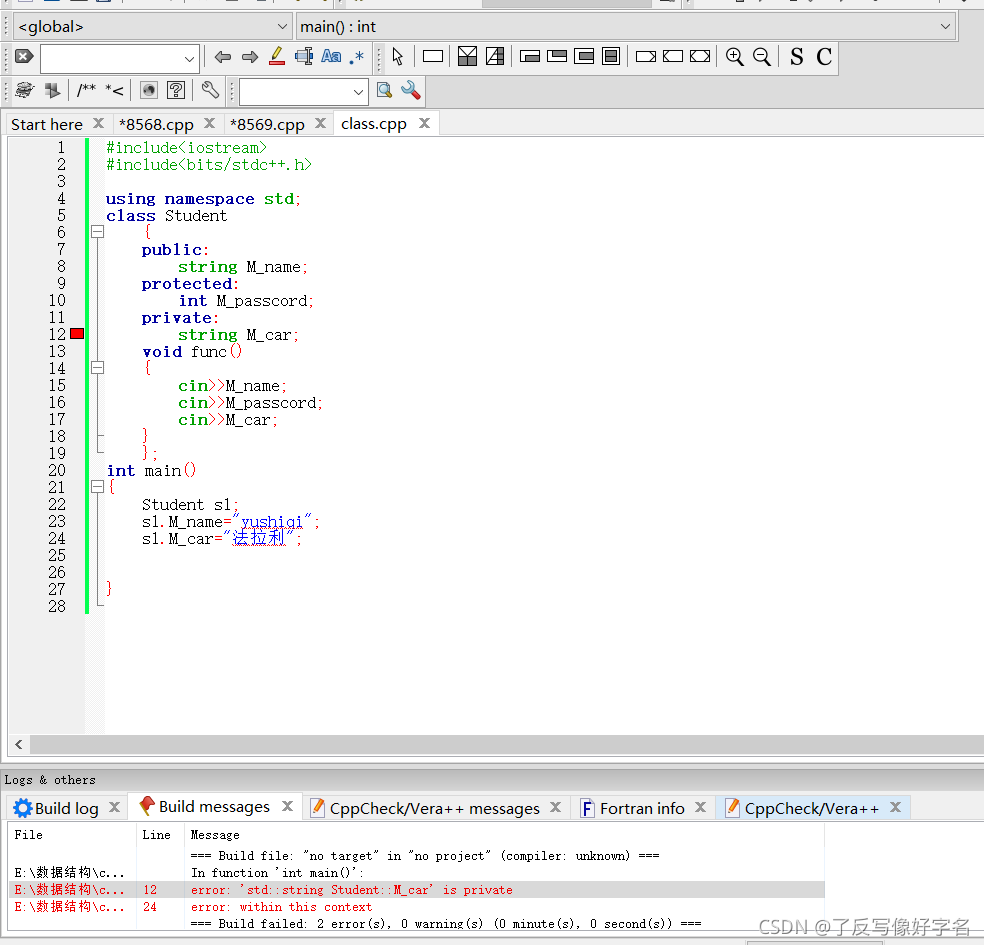
C++封装案例1:
#include<iostream>
#include<bits/stdc++.h>
using namespace std;
class cube
{
int len,width,height;
public:
void set_len()
{
cout<<"请输入立方体的长度是 ";cin>>len;
}
void set_width()
{
cout<<"请输入立方体的宽度是 ";cin>>width;
}
void set_height()
{
cout<<"请输入立方体的高度是 ";cin>>height;
}
int get_len()
{
return len;
}
int get_width()
{
return width;
}
int get_height()
{
return height;
}
int get_volume()
{
return len*width*height ;
}
int get_calculate()
{
return (len*width+width*height+len*height)*2;
}
bool IS_same(cube &c2)//用引用就不用拷贝俩份值了;
{
if(height==c2.height&&width==c2.width&&len==c2.len)
{
return true;
}
return false;
}
};
int main()
{
cube c1,c2;
c1.set_height();
c1.set_len();
c1.set_width();
c2.set_height();
c2.set_len();
c2.set_width();
cout<<"两个立方体是否相等:"<<c1.IS_same(c2);
//cout<<"立方体的体积是:"<<cube1.get_volume()<<endl;
//cout<<"立方体的面积是: "<<cube1.get_calculate()<<endl;
system("pause");
}
|