#include <iostream>
#include <string>
#include <map>
using namespace std;
struct StructTest
{
std::string str1;
std::string str2;
double diameter;
double length;
StructTest() : str1(""), str2(""), diameter(0.), length(0.) {}
StructTest(const std::string& str1, const std::string& str2, double diameter,
double length)
: str1(str1), str2(str1), diameter(diameter), length(length)
{
}
bool operator = (const StructTest& c) const
{
if (!str1.compare(c.str1) && !str2.compare(c.str2) && abs(diameter - c.diameter) < 0.0001
&& abs(length - c.length) < 0.0001)
{
return true;
}
return false;
}
bool operator < (const StructTest& c) const
{
if (str1 < c.str1)
{
return str1 < c.str1;
}
if (str2 < c.str2)
{
return str2 < c.str2;
}
if (diameter < c.diameter)
{
return diameter < c.diameter;
}
if (length < c.length)
{
return length < c.length;
}
return false;
}
};
int main()
{
map<StructTest, int> test1;
StructTest tag;
tag.str1 = "chen";
tag.str2 = "111";
tag.diameter = 1;
tag.length = 100;
test1.insert(make_pair(tag, 22));
std::cout << "find new one\n";
StructTest key;
if (test1.find(key) == test1.end())
{
std::cout << "not find\n";
}
else
{
std::cout << "find\n";
}
std::cout << "\nfind itself \n";
if (test1.find(tag) == test1.end())
{
std::cout << "not find\n";
}
else
{
std::cout << "find\n";
}
getchar();
return 0;
}
输出
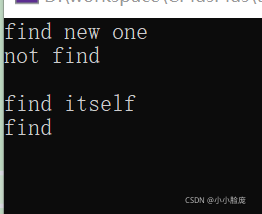
?
之前也没有使用map做过类似的功能,调BUG的过程中发现,如果在结构体中没有写 < 的重载,直接使用map的find会有问题。
当然这个和map本身的结构有关
<<<未完待续>>>
|