反序输出数组
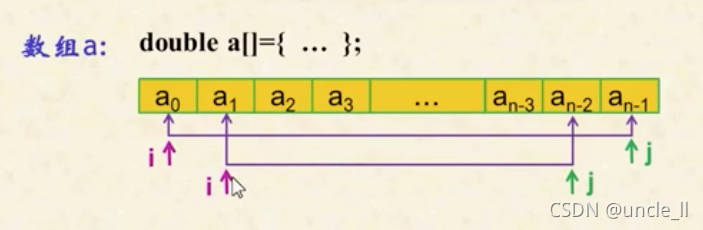
#include<iostream>
#include<stdlib.h>
using namespace std;
// 反序存放各数组元素
void invert(double *x, int n)
{
double t, *i, *j;
i = x;
j = x + n -1;
while(i<j)
{
t = *i;
*i = *j;
*j = t;
i++;
j--;
}
}
int main()
{
double a[5] = {1,2,3,4,5.0};
// double *p = new double[5]; //动态数组
cout <<"原始数组:";
for(int i=0; i<5; i++)
{
cout << a[i] << " ";
}
cout<< endl;
invert(a, 15);
cout <<"反序后数组:";
for(int i=0; i<5; i++)
{
cout << a[i] << " ";
}
cout<< endl;
return 0;
}
字符串排序
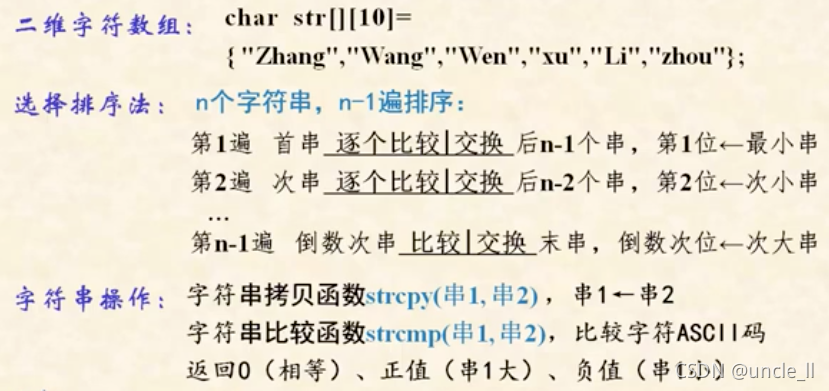
#include <iostream>
#include <cstring>
using namespace std;
void sort(char (*p)[10], int n)
{
for(int i=0; i<n-2; i++)
{
for(int j=i+1; j<n; j++)
{
char t[10];
if(strcmp(p[i], p[j]) > 0)
{
strcpy(t, p[i]);
strcpy(p[i], p[j]);
strcpy(p[j], t);
}
}
}
}
int main()
{
char str[][10] = {"zhang", "wang", "wen", "xu", "li", "zhou"};
// char (*s)[10] = new char[n][10]; // 定义动态数组
sort(str, 6);
for(int i=0; i<6; i++)
{
cout << str[i] << "\t";
}
return 0;
}
自己实现比较函数与复制函数,集成交换函数以及显示字符串
char *mystrcpy(char *s1, char *s2) // copy s2 into s1
{
while(*s2 != 0)
{
*s1++ = *s2++;
}
*s1 = '\0';
}
int mystrcmp(char *s1, char *s2)
{
while(*s1 == *s2 && ! *s1!= 0 && *s2 != 0)
{
s1++;
s2++;
}
return *s1 - *s2; // 正值,前一个大,负值,后一个大
}
void swap(char *p, char *q)
{
char t[10];
mystrcpy(t, p);
mystrcpy(p, q);
mystrcpy(q, t);
}
void show(char (*s)[10], int n)
{
for(int i=0; i<n-1; i++)
{
cout << s[i] << " ";
}
cout << s[n-1] << endl;
}
二进制ip地址转化为点分十进制形式
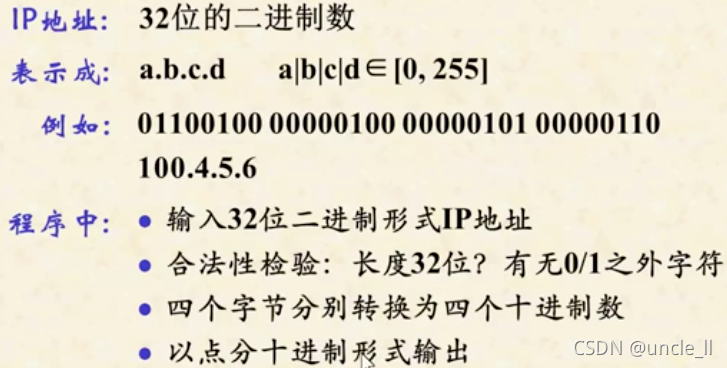
- 实现:
- 检测字符串中是否有01之外的字符
- 二进制数 --> 十进制数
#include <iostream>
#include <cstring>
using namespace std;
// check only contain 0/1 in str
bool check(char *str)
{
for(int i=0; i<32; i++)
{
if(str[i] != '1' && str[i] != '2')
{
return false;
}
}
return true;
}
// 32位二进制数转化为十进制数
// 1101B = (((0*2+1)*2+1)*2+0)*2+1 = 13
int trans(char *Str)
{
int n=0, i;
for(i=0; i<8; i++)
{
if(str[i] =='1')
{
n = n*2 + 1;
}
else
{
n = n*2;
}
}
return n;
}
int main()
{
char IP[33];
cout << "32位二进制IP地址?" << endl;
cin >> IP;
if(strlen(IP) != 32)
{
cout << "IP 长度应该是32位" << endl;
}
else
{
if(!check(IP))
{
cout << "字符串中有0/1之外的字符,非正确IP地址." << endl;
}
else
{
cout << "IP地址对应的点分十进制数写法:" << endl;
cout << trans(IP) << "." << trans(IP+8) << "." << trans(IP+16) << "." << trans(IP+24) << endl;
}
}
return 0;
}
|