问题描述
迷你搜索软件 H 大家的日常生活都离不开搜索引擎,搜索引擎结合了计算机和数学里面的好多技术,下面我们做个非常简单的模拟。假设有str1如下,要求用户键入要搜索的字符串,判断该字符串是否是str1的子字符串,如果是显示第一次匹配的下标,否则显示不是子字符串。 str1=“hello world” Input:ell Output: ell are found in str1 at index 1; Input ole Output: ole are not found in str1.
以下是一种思路
0.1问题分析:注意题目里用户键入的字符串长度是未知的,为了简便,你可以预定义一个稍大的静态字符数组。 在具体的算法实现过程中,请考虑以下不同情况,假设target代表要搜索的子字符串,src代表源字符串:我们知道,至少需要2重循环,外循环用于在src上逐一寻找第一个匹配字符,内循环用于在找到第一匹配字符的情况下,搜索余下字符是否匹配。 第一阶段:target和src需要比较的最大次数如何确定?即外循环次数 (1)target比src长:外部需要0次循环 (2)target 和src等长: 外部需要1次循环 (3)target 比 src短:外部需要length(src)-length(target)次循环 这说明当比较时,如果src余下的部分小于target长度,终止搜索 第二阶段:target和src在某次具体比较中的不同情况 (1)target[j] (j=0)和src[i]不相等,显然仅需要i++后继续比较,j不变 (2)target[j](j=0)和src[i]相等,显然i++,j++继续比较,由于i,j增长同步,可以写作target[j],src[i+j]
以下是简便的思路,采用双指针
代码
#include<iostream>
#include<string>
using namespace std;
void menu();
int search(char*,char*,int,int);
int main() {
menu();
return (EXIT_SUCCESS);
}
int search(char* src, char* tg, int l1, int l2) {
int i, j,flag;
i = 0, j = 0, flag = 0;
while (i <= l1)
if (j < l2) {
if (*(src + i) == *(tg + j))
j++;
else
j = 0;
i++;
}
else {
flag = 1;
break;
}
return (flag)?i-l2:-1;
}
void menu() {
int n;
char ch;
string src,target;
while (true) {
cout << "按ENTER键以继续" << endl;
cin.ignore(1024, '\n');
cin.get();
system("cls");
cout << "s----------更改SRC字符串" << endl;
cout << "t------------查找TARGET字符串" << endl;
cout << "q---------退出" << endl;
cout << "目前的SRC字符串为:";
for (int i = 0; i < src.length(); i++)
cout << src[i];
cout << endl;
cout << ">>";
cin >> ch;
switch (ch)
{
case ('S'):
case ('s'):
cout << "input the src string:" << endl;
cin >> src;
break;
case('t'):
case('T'):
n = -1;
cout << "input the target string:" << endl;
cin >> target;
n = search(&src[0], &target[0], src.length(), target.length());
if (n != -1)
cout << "first find target at the index of " << n << endl;
else
cout << "not find target" << endl;
break;
case ('q'):
case('Q'):
return;
break;
default:
cout << "输入不合法!请重试!" << endl;
break;
}
}
}
运行截图
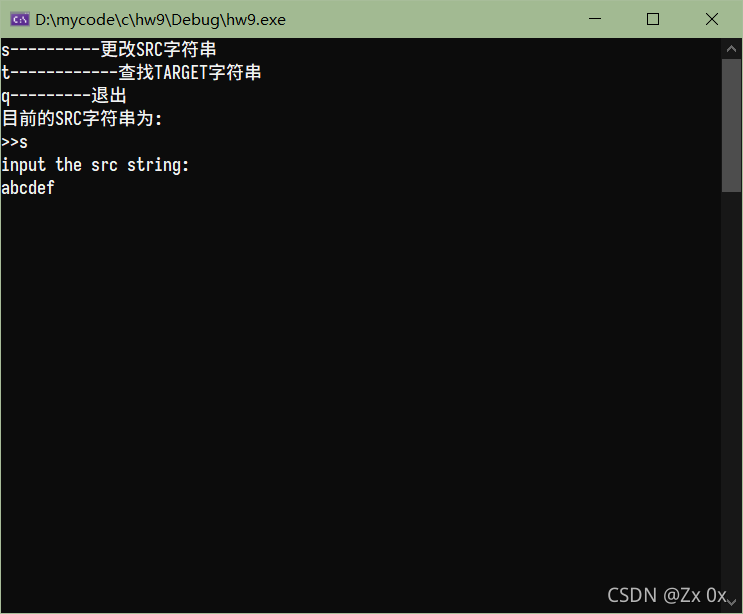 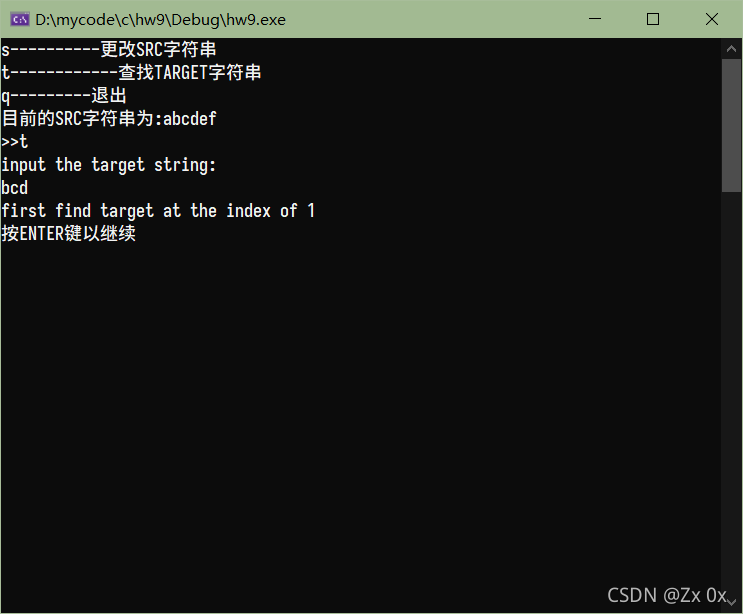 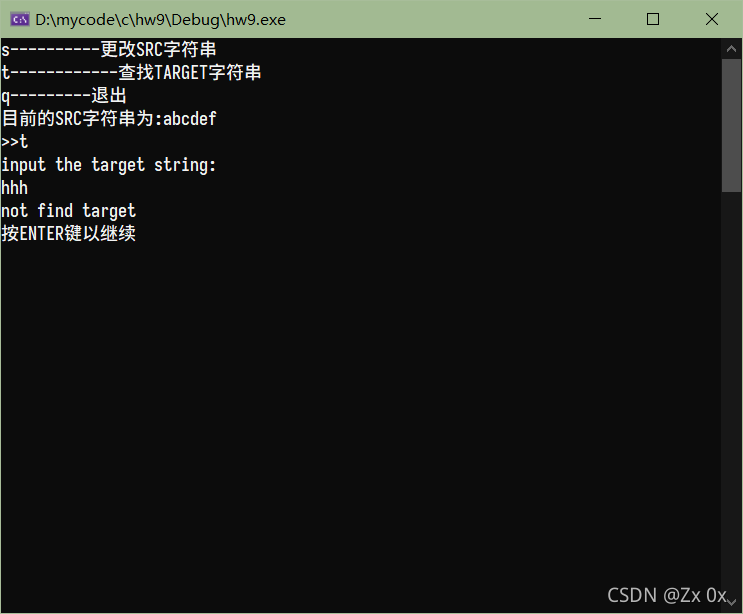
|