这是关于C++项目集的第二篇文章,一个关于企业的职工管理系统
职工管理系统:
代码一共有6百行左右,限于篇幅在此处列出主要部分 [注意噢~]。不过所有代码我都放在了码云和GitHub里,更加方便阅读! 码云地址:https://gitee.com/huazhige/cpp-project-from-0-to-all
你也也可以来我的公众号 【华枝歌】,只需回复C++项目从0到全部 我把整个系列代码打包发你,快捷省心。
代码:
main实现所有系统需求:
1.首先进入欢迎界面,让用户输入不同选择,进入不同选项 1.1. 0 – 退出 1.2. 1 – 增加职工 1.3. 2 – 删除职工 1.4. 3 – 显示所有职工信息 1.5. 4 – 修改职工信息 1.6. 5 – 查找职工 1.7. 6 – 按职工编号进行排序 1.8. 7 – 清空文档
main函数:
#include<iostream>
#include"workerManager.h"
#include"employee.h"
#include"manager.h"
#include"boss.h"
using namespace std;
int main() {
WorkerManager wm;
int choice = 0;
while (true){
wm.showMenu();
cout << "请输入您的选择:";
cin >> choice;
switch (choice)
{
case 0:
wm.exitSystem();
break;
case 1:
wm.addEmp();
break;
case 2:
wm.showEmp();
break;
case 3:
wm.delEmp();
break;
case 4:
wm.modEmp();
break;
case 5:
wm.findEmp();
break;
case 6:
wm.sortEmp();
break;
case 7:
wm.cleanFile();
break;
default:
system("cls");
break;
}
}
return 0;
}
0.退出- 退出管理系统
exitSystem();
void WorkerManager::exitSystem() {
cout << "欢迎下次使用" << endl;
exit(0);
}
1.增加职工,添加新职工,可以批量添加多个职工,职工分为普通员工、经理以及总裁
addEmp();
void WorkerManager::addEmp() {
int addnum = 0;
cout << "请输入增加职工数量:" << endl;
cin >> addnum;
if (addnum > 0) {
int newSize = this->m_EmpNum + addnum;
Worker** newSpace = new Worker*[newSize];
if (this->m_EmpArray != NULL) {
for (int i = 0; i < this->m_EmpNum; ++i) {
newSpace[i] = this->m_EmpArray[i];
}
}
for (int i = 0; i < addnum; ++i) {
int id;
string name;
int dpt_id;
cout << "输入第" << i + 1 << "个新职工编号" << endl;
cin >> id;
cout << "输入第" << i + 1 << "个新职工姓名" << endl;
cin >> name;
cout << "请选择该职工岗位:" << endl;
cout << "1、普通职工" << endl;
cout << "2、经理" << endl;
cout << "3、老板" << endl;
cin >> dpt_id;
Worker* worker = NULL;
switch (dpt_id)
{
case 1:
worker = new Employee(id, name, dpt_id);
break;
case 2:
worker = new Manager(id, name, dpt_id);
break;
case 3:
worker = new Boss(id, name, dpt_id);
default:
break;
}
newSpace[this->m_EmpNum + i] = worker;
}
delete[] this->m_EmpArray;
this->m_EmpArray = newSpace;
this->m_EmpNum = newSize;
cout << "添加成功 " << addnum << " 名新职工" << endl;
this->save();
this->m_fileIsEmpty = false;
}
else {
cout << "输入有误" << endl;
}
system("pause");
system("cls");
}
2.显示所有职工信息,先判断文件是否存在或是否清空,如果存在并且有记录的情况下,展示文件内记录内所有职工的信息
showEmp();
void WorkerManager::showEmp() {
if (this->m_fileIsEmpty) {
cout << "文件不存在或为空" << endl;
}
else {
for (int i = 0; i < this->m_EmpNum; ++i) {
this->m_EmpArray[i]->showInfo();
}
}
system("pause");
system("cls");
}
3.删除职工,可以按照职工编号进行删除职工
delEmp();
void WorkerManager::delEmp() {
if (this->m_fileIsEmpty) {
cout << "没有职工记录" << endl;
}
else {
cout << "输入将要删除的职工的编号:" << endl;
int id = 0;
cin >> id;
int get = this->isExist(id);
if (get != -1) {
for (int i = get; i < this->m_EmpNum; ++i) {
this->m_EmpArray[i] = this->m_EmpArray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功!" << endl;
}
else {
cout << "删除失败,该编号职工不存在,请重新操作!" << endl;
}
}
system("pause");
system("cls");
}
4.修改职工信息,可以按照用户输入的职工编号,修改职工新的信息,并保存到文件中
modEmp();
void WorkerManager::modEmp() {
if (this->m_fileIsEmpty) {
cout << "没有职工记录" << endl;
}
else {
cout << "请输入修改职工编号:" << endl;
int id;
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {
delete this->m_EmpArray[ret];
int newId = 0;
string newName = "";
int newdId = 0;
cout << "查到编号:" << id << "员工,请输入新职工号:" << endl;
cin >> newId;
cout << "请输入新姓名:" << endl;
cin >> newName;
cout << "请输入新岗位:" << endl;
cout << "1、普通职工" << endl;
cout << "2、经理" << endl;
cout << "3、老板" << endl;
cin >> newdId;
Worker* worker = NULL;
switch (newdId) {
case 1:
worker = new Employee(newId, newName, newdId);
break;
case 2:
worker = new Manager(newId, newName, newdId);
break;
case 3:
worker = new Boss(newId, newName, newdId);
break;
}
this->m_EmpArray[ret] = worker;
cout << "修改成功" << endl;
this->save();
}
else {
cout << "没有此职工" << endl;
}
}
system("pause");
system("cls");
}
5.查找职工信息,查找有两种方式,一个按职工编号查找,一个按姓名查找,如果用户查找的内容不为空显示该职工信息,如果为空提示不存在要查找的职工
findEmp();
void WorkerManager::findEmp() {
if (this->m_fileIsEmpty) {
cout << "没有职工记录" << endl;
}
else {
cout << "请输入查找方式:" << endl;
cout << "1:按职工编号查找" << endl;
cout << "2:按职工姓名查找" << endl;
int select = 0;
cin >> select;
if (select == 1) {
int id;
cout << "请输入要查询的职工号:";
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {
cout << "查找成功!该职工信息:" << endl;
this->m_EmpArray[ret]->showInfo();
}
else {
cout << "没有查找到此人" << endl;
}
}
else if (select == 2) {
string name;
cout << "请输入要查找职工的姓名:";
cin >> name;
bool hasfind = false;
for (int i = 0; i < this->m_EmpNum; ++i) {
if (this->m_EmpArray[i]->m_Name == name) {
cout << "查找成功!" << endl;
cout << "职工信息为: " << endl;
this->m_EmpArray[i]->showInfo();
hasfind = true;
}
}
if (!hasfind) {
cout << "没查询到此职工" << endl;
}
}
else {
cout << "输入错误!请重新操作!" << endl;
}
}
system("pause");
system("cls");
}
6.按职工编号进行排序,用户可以将输入的所有职工按照职工编号进行升序或者降序排列,并存入文件
sortEmp();
void WorkerManager::sortEmp() {
if (this->m_fileIsEmpty) {
cout << "没有职工信息" << endl;
system("pause");
system("cls");
}
else {
cout << "选择职工排序方式:" << endl;
cout << "1:按职工编号升序排序" << endl;
cout << "2:按职工编号降序排序" << endl;
int select = 0;
cin >> select;
for (int i = 0; i < this->m_EmpNum; ++i) {
int key = i;
for (int j = i + 1; j < this->m_EmpNum; ++j) {
if (select == 1) {
if (this->m_EmpArray[key]->m_Id > this->m_EmpArray[j]->m_Id) {
key = j;
}
}
else {
if (this->m_EmpArray[key]->m_Id < this->m_EmpArray[j]->m_Id) {
key = j;
}
}
}
if (i != key) {
Worker* temp;
temp = this->m_EmpArray[i];
this->m_EmpArray[i] = this->m_EmpArray[key];
this->m_EmpArray[key] = temp;
}
}
cout << "排序成功!排序后结果为:" << endl;
this->save();
this->showEmp();
}
}
7.清空文档,可以将文件内当前的记录全部清空。
cleanFile();
void WorkerManager::cleanFile() {
cout << "确认清空职工信息?" << endl;
cout << "1:确认" << endl;
cout << "2:返回" << endl;
int select = 0;
cin >> select;
if (select == 1) {
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
if (this->m_EmpArray!=NULL) {
for (int i = 0; i < this->m_EmpNum; ++i) {
if (this->m_EmpArray[i] != NULL) {
delete this->m_EmpArray[i];
}
}
this->m_EmpNum = 0;
delete[] this->m_EmpArray;
this->m_EmpArray = NULL;
this->m_fileIsEmpty = true;
}
cout << "清空成功" << endl;
}
system("pause");
system("cls");
}
本系统设计到的知识点为:面向对象中的封装、继承、多态以及文件IO流,在设计中有Worker职工的基类,以及分别派生类为普通员工、经理、以及总裁,基类中有纯虚函数子类分别作了实现。然后有个文件管理类,对用户做出不同的选择分别做不同的处理。可以对系统进行基本的增删改查功能。
需要全部或者整个C++实战项目训练系列的代码可以来我的公众号 【华枝歌】,只需回复C++项目从0到全部 我把整个系列代码打包发你,快捷省心。
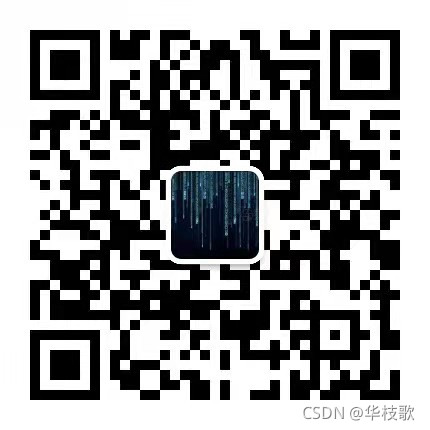
|