STL:STL是c++中的标准模板库,在其中有很多大佬封装的模板类,帮助我们的代码,使c++的编程更加方便。 下面介绍string类的一些常见用法:
1、string的构造函数
string();
string(const char*s);
string(size_t n,char c);
string(const string&s);
使用举例:
string s1;
cout << "s1 = "<< s1 << endl;
char *s = "hello world!";
string s2(s);
cout << "s2 = " << s2 << endl;
string s3(5, 'c');
cout << "s3 = " << s3 << endl;
string s4 = s2;
cout << "s4 = " << s4 << endl;
结果展示: 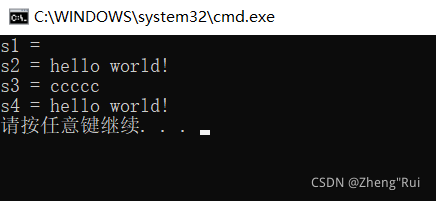
2.string常用的容量操作
size_t size();
size_t lenth();
size_t capacity();
bool empty();
void clear();
void reserve(size_t n=0);
void resize(size_t n,char c);
void resize(size_t n,char c);
使用举例:
string s1 = "abcdefg";
cout << "size = " << s1.size() << endl;
cout << "length = " << s1.length() << endl;
cout << "capacity = " << s1.capacity() << endl;
if (s1.empty())
cout << "it is empty!" << endl;
else
cout << "it is not empty!" << endl;
s1.reserve(20);
cout << "capacity = " << s1.capacity() << endl;
s1.resize(5);
cout << "size = " << s1.size() << endl;
s1.resize(10, 'c');
cout << s1 << endl;
s1.clear();
cout << "size = " << s1.size() << endl;
结果展示: 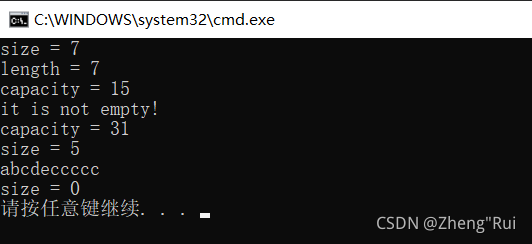
3.string对象的访问及常用的遍历操作
char& operator[](int i);
begin() + end();
rbegin() + rend();
使用举例:
string s1 = "abcdefg";
for (int i = 0; i < s1.size(); i++)
{
cout << s1[i];
}
cout << endl;
string::iterator it = s1.begin();
while (it != s1.end())
{
cout << *it;
it++;
}
cout << endl;
string::reverse_iterator rit = s1.rbegin();
while (rit != s1.rend())
{
cout << *rit;
rit++;
}
cout << endl;
结果展示: 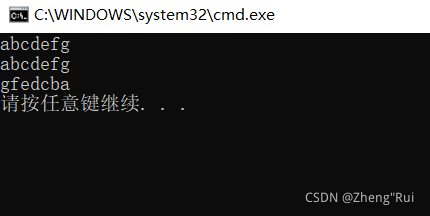
4.string类的对象修改操作
void push_back(char c);
void append(const char* s);
string& operator+=(char *s);
const char* c_str()const;
size_t find(char c,size_t pos = 0)const;
size_t rfind(char c,size_t pos = npos)const;
使用举例:
string s1 = "abc";
s1.push_back('d');
s1.push_back('e');
s1.push_back('f');
cout << "s1 = " << s1 << endl;
s1.append("ghijk");
cout << "s1 = " << s1 << endl;
s1 += "lmn";
cout << "s1 = " << s1 << endl;
const char* cstr = s1.c_str();
for (int i = 0; i < strlen(cstr); i++)
cout << cstr[i];
cout << endl;
s1.push_back('c');
cout << "first-pos = " << s1.find('c') << endl;
cout << "last-pos = " << s1.rfind('c') << endl;
结果展示: 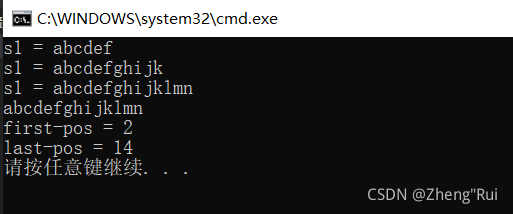
5.string类的非成员函数
string operator+(const string& lhs, const string& rhs);
istream& operator>>(istream& is,string& str);
ostream& operator<<(ostream& os,string& str);
istream& getline(istream& is,string& str);
bool operator==(const string& lhs,const string& rhs);
……
使用举例:
string s1 = "abc";
string s2 = "def";
string s3 = s1 + s2;
cout << "s3 = " << s3 << endl;
cin >> s1;
cout << "s1 = " << s1 << endl;
getchar();
getline(cin, s2);
cout << "s2 = " << s2 << endl;
if (s1 == s2)
cout << "s1 = s2" << endl;
else
cout << "s1 != s2" << endl;
结果展示: 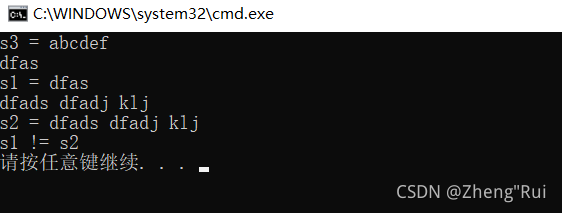
结语:
注意,string中有很多的内部方法,这里只介绍了其中常用的部分,而且,其中很多方法都有它的重载函数,这里只介绍了其中一种,剩下的方法可以自行查阅,用法大致相同。
|