/********************************************* 说明:计算倒计时间或已逝去时
主要函数: char *countdown(char *dsttimes); 函数接受字串格式 如2036年10月10日10时10分10秒 要写成这样:20361010101010
注意:共14位数字,中间无空格,必须要上述格式输入,否则会计算有误。
此函数用于计算未来时间点或者是已经逝去的时间点距至今相距多长时间
倒计时/已逝时间:精确到秒 *********************************************/
#include<stdio.h>
#include<time.h>
#include<string.h>
#include<stdlib.h>
#include<conio.h>
void delay_ms(long ms)
{
long usec=ms*1000;
clock_t end;
end=clock()+(clock_t)(usec);
while(clock()<end);
}
char* countdown(char *dsttimes)
{
int d;
int h;
int m;
int s;
struct dsttime
{
int year;
int mon;
int mday;
int hour;
int min;
int sec;
char years[5];
char mons[3];
char mdays[3];
char hours[3];
char mins[3];
char secs[3];
}dsttime;
time_t dst;
double times;
static char str_times[100]={'\0'};
char *p=dsttimes;
struct tm dstt;
strncpy(dsttime.years,p,4);
dsttime.year=atoi(dsttime.years);
dsttime.mons[0]=*(p+4);
dsttime.mons[1]=*(p+5);
dsttime.mons[2]='\0';
dsttime.mon=atoi(dsttime.mons);
dsttime.mdays[0]=*(p+6);
dsttime.mdays[1]=*(p+7);
dsttime.mdays[2]='\0';
dsttime.mday=atoi(dsttime.mdays);
dsttime.hours[0]=*(p+8);
dsttime.hours[1]=*(p+9);
dsttime.hours[2]='\0';
dsttime.hour=atoi(dsttime.hours);
dsttime.mins[0]=*(p+10);
dsttime.mins[1]=*(p+11);
dsttime.mins[2]='\0';
dsttime.min=atoi(dsttime.mins);
dsttime.secs[0]=*(p+12);
dsttime.secs[1]=*(p+13);
dsttime.secs[2]='\0';
dsttime.sec=atoi(dsttime.secs);
dstt.tm_year=dsttime.year-1900;
dstt.tm_mon=dsttime.mon-1;
dstt.tm_mday=dsttime.mday;
dstt.tm_hour=dsttime.hour;
dstt.tm_min=dsttime.min;
dstt.tm_sec=dsttime.sec;
dstt.tm_isdst=-1;
dst=mktime(&dstt);
if( dst==-1)
return "unknown";
times=difftime(dst,time(NULL));
d=(int) (times/86400);
h=(int) ((times-d*86400.0)/3600);
m=(int) ((times-d*86400.0-h*3600.0)/
60.0);
s=(int) (times-d*86400.0-h*3600.0-m*60.0);
if(times>0.0)
{
snprintf(str_times,98,"距%s年%s月%s日%s时%s分%s秒\n倒计时:%d日%d时%d分%d秒",dsttime.years,dsttime.mons,dsttime.mdays,dsttime.hours,dsttime.mins,dsttime.secs,d,h,m,s);
}
else
{
snprintf(str_times,98,"距%s年%s月%s日%s时%s分%s秒\n已逝去:%d日%d时%d分%d秒",dsttime.years,dsttime.mons,dsttime.mdays,dsttime.hours,dsttime.mins,dsttime.secs,-d,-h,-m,-s);
}
return str_times;
}
int main()
{
char str[29]={NULL};
printf("精确计算倒计时/已逝时间\n");
printf("例如:2026年10月10日23时00分05秒\n");
printf("只需输入:20261010230005\n");
printf("必须严格按上述格式输入,否则结果会有误!\n");
printf("请输入日期时间:");
fgets(str,15,stdin);
while(1)
{
printf("%s\n\",countdown(str));
delay_ms(999);
clrscr();
}
return 0;
}
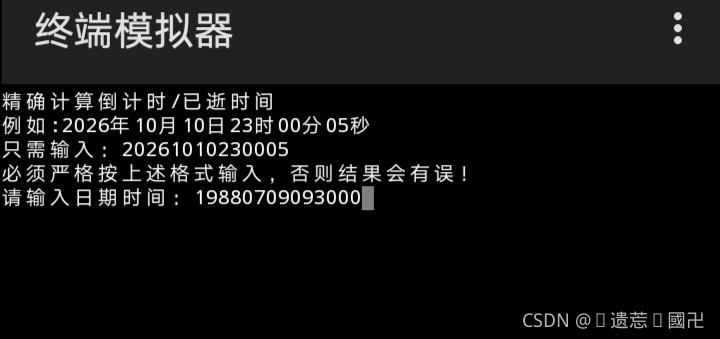 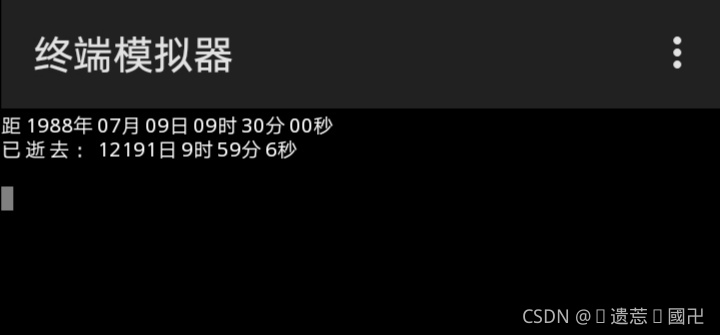 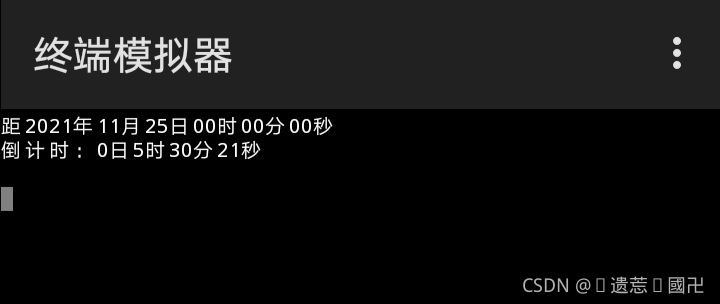
|