实验要求
- 撰写自己的算法和函数,结合容器和迭代器解决序列变换(如取反、平方、立方),像素变换(二值化、灰度拉伸);
- 用set存储学生信息,并进行增删改查操作;
- 输入一个字符串,用map统计每个字符出现的次数并输出字符及对应的次数
实验内容及测试代码
取反与平方函数
用一般函数来实现取反和平方
void transInv(int a[], int b[], int nNum) {
for (int i = 0; i < nNum; i++) {
b[i] = -a[i];
}
}
void transSqr(int a[], int b[], int nNum) {
for (int i = 0; i < nNum; i++) {
b[i] = a[i]*a[i];
}
}
用模板函数来实现取反和平方
template < typename T>
void transInvT(T a[], T b[], int nNum) {
for (int i = 0; i < nNum; i++) {
b[i] = -a[i];
}
}
template < typename T>
void transSqrT(T a[], T b[], int nNum) {
for (int i = 0; i < nNum; i++) {
b[i] = a[i] * a[i];
}
}
输出模板函数
template < typename T>
void outputCont(string strNme, ostream& os, T begin, T end) {
os << strNme << ": ";
for (; begin != end; begin++) {
os << *begin << " ";
}
os << endl;
}
测试函数
void test1() {
const int N = 5;
int a[N] = { 1,2,3,4,5 };
outputCont("a", cout, a, a + N);
int b[N];
transInv(a, b, N);
outputCont("Inv a", cout, b, b + N);
transSqr(a, b, N);
outputCont("Sqr a", cout, b, b + N);
transInvT(a, b, N);
outputCont("Inv a T", cout, b, b + N);
transSqrT(a, b, N);
outputCont("Sqr a T", cout, b, b + N);
}
运行结果如下 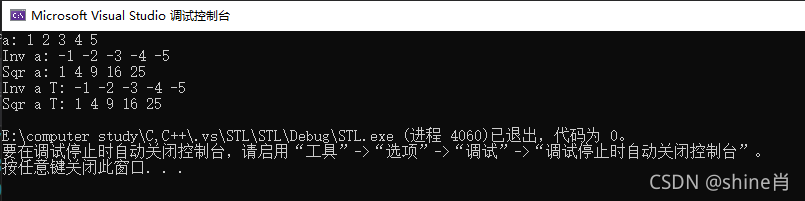
像素变换函数
像素变换函数
template <typename inputIter, typename outputIter, typename MyOperator>
void transInvT(inputIter begInput, inputIter endInput,
outputIter begOutPut, MyOperator op)
{
for (; begInput != endInput; begInput++, begOutPut++)
{
*begOutPut = op(*begInput);
}
}
template<typename T>
class MyThreshold {
public:
MyThreshold(int n = 128) : _nThreshold(n){}
int operator()(T val)
{
return val < _nThreshold ? 0 : 1;
}
int _nThreshold;
};
测试函数
void test2(){
const int N = 5;
int a[N] = { 1,2,3,4,5 };
int b[N];
vector<double> vb(N);
vector<double> vc(N);
transInvT(a, a + N, b, InvT<int>);
transInvT(a, a + N, vb.begin(), InvT<int>);
outputCont("Inv a by iter", cout, vb.begin(), vb.end());
transInvT(a, a + N, vb.begin(), MyThreshold<int>(2));
outputCont("Inv a by treshold", cout, vb.begin(), vb.end());
}
运行结果如下 
用set存储学生信息,并进行增删改查操作
构造一个students类
class studentInfo {
public:
studentInfo(string strNo, string strName) {
_strNo = strNo;
_strName = strName;
}
string _strNo;
string _strName;
friend ostream& operator<<(ostream& os, const studentInfo& info)
{
os << info._strNo << " " << info._strName;
return os;
}
friend bool operator<(const studentInfo& info1, const studentInfo& info2) {
return info1._strNo < info2._strNo;
}
};
|