一、STL
- 标准模板库(Standard Template Library,简称STL)定义了一套概念体系,为泛型程序设计提供了逻辑基础
- STL中的各个类模板、函数模板的参数都是用这个体系中的概念来规定的。
- 使用STL的模板时,类型参数既可以是C++标准库中已有的类型,也可以是自定义的类型——只要这些类型是所要求概念的模型。
组件 | 描述 |
---|
容器(Containers) | 容器是用来管理某一类对象的集合。C++ 提供了各种不同类型的容器,比如 deque、list、vector、map 等。 | 算法(Algorithms) | 算法作用于容器。它们提供了执行各种操作的方式,包括对容器内容执行初始化、排序、搜索和转换等操作。 | 迭代器(iterators) | 迭代器用于遍历对象集合的元素。这些集合可能是容器,也可能是容器的子集。 |
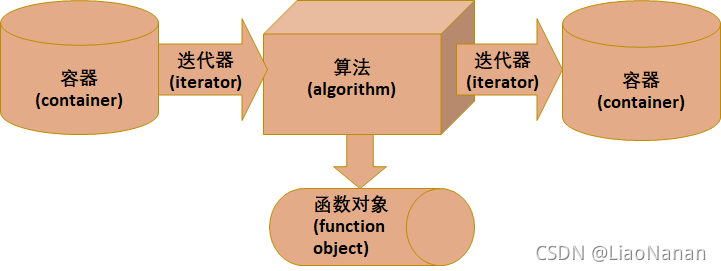
二、STL应用
2.1 结合容器和迭代器解决序列变换(如取反、平方、立方)
代码:
#include <iostream>
#include <vector>
using namespace std;
template<typename T>
class negatation {
public:
T operator()(T input)
{
return -input;
}
};
template<typename T>
class square {
public:
T operator()(T input)
{
return pow(input, 2);
}
};
template<typename T>
class cubic {
public:
T operator()(T input)
{
return pow(input, 3);
}
};
template<typename input, class function>
void traverse(input first, input last, ostream& os, function op)
{
for (; first != last; ++first)
os << op(*first) << " ";
}
int main() {
const int N = 5;
vector<int> s(N);
for (int i = 0; i < N; i++)
cin >> s[i];
cout << "取反:";
traverse(s.begin(), s.end(), cout, negatation<int>());
cout << endl;
cout << "平方:";
traverse(s.begin(), s.end(), cout, square<int>());
cout << endl;
cout << "立方:";
traverse(s.begin(), s.end(), cout, cubic<int>());
cout << endl;
return 0;
}
运行结果: 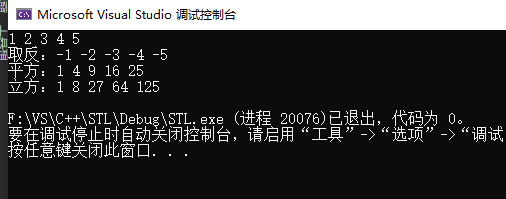
2.2 结合容器和迭代器解决像素变换(二值化)
代码:
#include <iostream>
#include <vector>
using namespace std;
template<typename T>
class Pixelate {
public:
int _value;
Pixelate(int value = 128) : _value(value) {}
T operator()(T input)
{
return input < _value ? 0 : 1;
}
};
template<typename input, class function>
void traverse(input first, input last, ostream& os, function op)
{
for (; first != last; ++first)
os << op(*first) << " ";
}
int main() {
const int N = 5;
vector<int> s(N);
for (int i = 0; i < N; i++)
cin >> s[i];
cout << "二值化:";
traverse(s.begin(), s.end(), cout, Pixelate<int>());
cout << endl;
return 0;
}
运行结果: 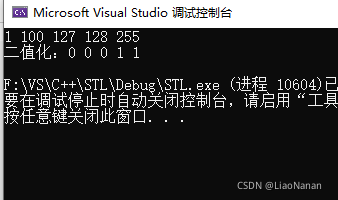
2.3 用set存储学生信息,并进行增删改查操作
代码:
#include <iostream>
#include <vector>
#include <set>
using namespace std;
template<typename input>
void traverse(input first, input last, ostream& os)
{
for (; first != last; ++first)
os << *first << " ";
}
class student_info {
public:
string _str_id;
string _str_name;
student_info(string id, string name) {
_str_id = id;
_str_name = name;
}
friend ostream& operator<<(ostream& os, const student_info& stduent)
{
os << stduent._str_id << " " << stduent._str_name;
return os;
}
friend bool operator<(const student_info& stduent1, const student_info& stduent2) {
return stduent1._str_id < stduent2._str_id;
}
};
int main() {
vector<student_info> students;
students.push_back(student_info("25048", "Huang Huacong"));
students.push_back(student_info("25058", "Wang Kailai"));
students.push_back(student_info("25059", "Li Ao"));
students.push_back(student_info("25060", "Lei Wenhui"));
set<student_info> studentSet(students.begin(), students.end());
cout << "student set的遍历结果为:[";
traverse(studentSet.begin(), studentSet.end(), cout);
cout << "]" << endl;
studentSet.erase(student_info("25059", "Li Ao"));
cout << "student set(deleted)的遍历结果为:[";
traverse(studentSet.begin(), studentSet.end(), cout);
cout << "]" << endl;
const char* num = "25060";
set<student_info>::iterator it;
for (it = studentSet.begin(); it != studentSet.end(); it++)
{
if (((*it)._str_id).compare(num) == 0)
cout << num << "学生姓名为:" << (*it)._str_name << endl;
}
}
运行结果: 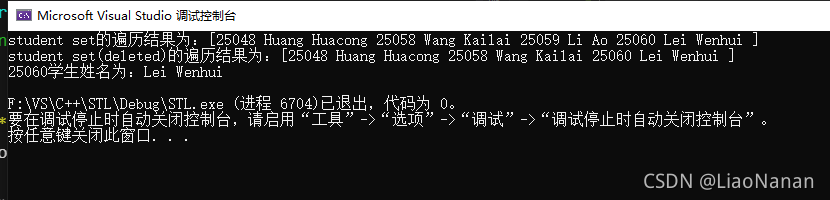
上面代码还缺少修改操作,但由于Set的迭代器iterator有const修饰,因此只能对它进行读取而不能进行修改。
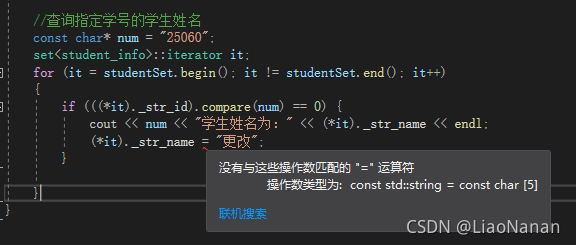
2.4 使用map统计字符串中每个字符出现的次数
代码:
#include <iostream>
#include<map>
using namespace std;
int main() {
map<char, int> word_count;
const char* word = "LiaoNanan";
for (int i = 0; i < strlen(word); i++)
{
++word_count[word[i]];
}
map<char, int>::iterator iter;
for (iter = word_count.begin(); iter != word_count.end(); iter++)
{
cout << "[" << iter->first << "] = " << iter->second << endl;
}
}
运行结果: 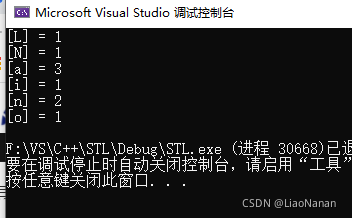
|