C++类的组合
前言
提示:以下是本篇文章正文内容,下面案例可供参考
一、以另一个类的对象为数据成员
1.构造函数的写法
#include <iostream>
#include <string>
using namespace std;
class Boy
{
public:
Boy(string name, int age) :name(name), age(age){}
void print()
{
cout << name << "\t" << age << endl;
}
protected:
void printDATA()
{
cout << name << "\t" << age << endl;
}
string name;
int age;
};
class Girl
{
public:
Girl(string boyName, int boyAge, string girlName) :boy(boyName, boyAge)
{
this->girlName = girlName;
}
void print()
{
boy.print();
cout << girlName << endl;
}
protected:
string girlName;
Boy boy;
};
int main()
{
Girl nv("nan", 18,"nv");
nv.print();
while (1);
return 0;
}
运行结果: 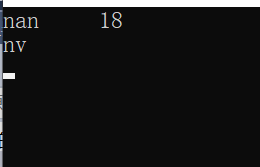
- 类的组合构造顺序问题:构造顺序之和定义对象顺序有关,和初始化参数列表无关
#include <iostream>
#include <string>
using namespace std;
class A
{
public:
A(string str) :str(str)
{
cout << str;
}
string str;
};
class B
{
public:
B(string str) :str(str)
{
cout << str;
}
string str;
};
class C
{
public:
C(string str) :str(str)
{
cout << str;
}
string str;
};
class D
{
public:
D(string stra, string strb, string strc) :b(strb), c(strc), a(stra)
{
cout << "D" << endl;
}
A a;
B b;
C c;
};
int main()
{
D d("A", "B", "C");
while (1);
return 0;
}
运行结果: 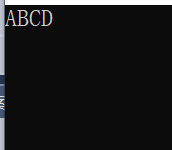
二、类中类
#include <iostream>
#include <string>
using namespace std;
struct Node
{
int data;
Node* next;
Node()
{
this->next = nullptr;
}
Node(int data)
{
this->next = nullptr;
this->data = data;
}
};
class List
{
public:
List()
{
headNode = new Node;
}
void push_front(int data)
{
Node* newNode = new Node(data);
newNode->next = headNode->next;
headNode->next = newNode;
}
protected:
Node *headNode;
public:
class iterator
{
public:
iterator(Node* pMove=nullptr) :pMove(pMove){}
void operator=(Node *pMove)
{
this->pMove = pMove;
}
bool operator != (Node* pMove)
{
return this->pMove != pMove;
}
iterator operator++()
{
pMove = pMove->next;
return iterator(pMove);
}
Node* operator*()
{
return pMove;
}
protected:
Node *pMove;
};
Node* begin()
{
return headNode->next;
}
Node* end()
{
return nullptr;
}
};
class A
{
public:
enum time{first,second};
protected:
enum date{ mon, sut, tus };
};
int main()
{
List list;
for (int i = 0; i < 3; i++)
{
list.push_front(i);
}
List::iterator iter;
for (iter = list.begin(); iter != list.end(); ++iter)
{
cout << (*iter)->data;
}
cout << A::time::first << endl;
while (1);
return 0;
}
运行结果: 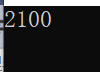
三、C++中默认的函数
- 1.默认构造函数
- 2.默认拷贝构造函数
- 3.默认析构函数
- 4.默认赋值运算
#include <iostream>
#include <string>
using namespace std;
class A
{
public:
A() = default;
A(A& object) = default;
A& operator=(A& object) = default;
~A() = default;
protected:
};
int main()
{
A a;
A b = a;
A c;
c = a;
while (1);
return 0;
}
|