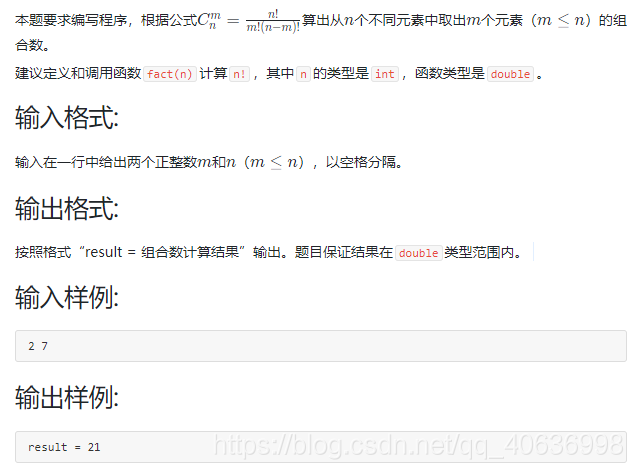
?首先输入样例就不对,应该是7 2,别弄错了,导致不能出结果21
方法一:
直接来,简单明了,完成作业
#include<stdio.h>
double fact(int b)
{
int a = 0;
double fact = 1;
//分析:以b等于3为例
//a=1>>fact=1*1>>a=2>>fact=1*2>>a=3>>fact=2*3
//a=4>3跳出for循环
for (a = 1; a <= b; a++)
{
fact *= a;
}
return fact;
}
int main()
{
int m, n = 0;
double result;
scanf("%d %d", &m, &n);
result = fact(n) / (fact(m) * fact(n - m));
printf("result = %f", result);
return 0;
}
方法二:(有递归)
#include<stdio.h>
int fact(int num);
int main()
{
//从n个元素中取m个组合
int n = 0;
int m = 0;
scanf("%d %d", &n,&m);
double ret = fact(n) / (fact(m) * fact(n - m));
printf("%.2f\n", ret);
}
int fact(int num)//求num的阶乘的函数
//5=5*4!=5*(4*3*2*1)
//4=4*3!=4*(3*2*1)
//递归的思想,列出函数,直接对着写
{
if (num < 0)
{
return 0;
}
if (num == 1||num == 0)
{
return 1;
}
if (num > 1)
{
return num * fact(num - 1);
}
}
方法三:(不用递归,看不懂递归的看这里)
函数定义与调用就是顺着来,我们要从m个数中拿n个数组合,那么函数就直接用

?我们想要求n的阶乘,我们就直接用
? ? ?
?总的来说,先写框架,最后补齐函数定义即可
#include<stdio.h>
double fact(int i, int j);
int fac(int k);
int main()
{
int n = 0;
int m = 0;
//scanf("%d %d\n",&n,&m);//错误示范,这样\n会要你多输入一个数
scanf("%d %d", &n, &m);
double ret = fact(n, m);
printf("%.2f\n", ret);
return 0;
}
int fac(int k)//求k的阶乘
{
int result = 1;
while (k != 0)
{
result = result * k;
k--;
}
return result;
}
double fact(int i, int j)//从i个元素中取j个进行组合
{
//求i,j,(i-j)的阶乘//i!/j!*(i-j)!
//定义新函数求阶乘 //int fac(int k);
int x = fac(i);
int y = fac(j);
int z = fac(i - j);
return x/(y*z);
}
|