队列学习笔记–链队和顺序队
参考书籍–《数据结构 c语言版》严蔚敏
1.队列(循环队列)
在队列的顺序存储结构中,除了用一组地址连续的存储单元依次存放从队列头到队列尾的元素之外,尚需附设两个整型变最 front 和 rear分别指示队列头元素及队列尾元素的位置(后面分别称为头指针和尾指针)。
每当插入新的队列尾元素时,尾指针 rear增1; 每当删除队列头元素时, 头指针 front增1。因此,在非空队列中,头指针始终指向队列头元素,而尾指针始终指向队列尾元素的下一个位置。
循环队列可充分利用空间,且减少了时间复杂度,通过移动frong和rear来实现。
如何判断队满与空: 少用一个元素空间, 即队列空间大小为m时,有m-1个元素就认为是队满。这样判断队空的条件即当头、 尾指针的值相同时,则认为队空;而当尾指针在循环意义上加1后是等于头指针, 则认为队满。 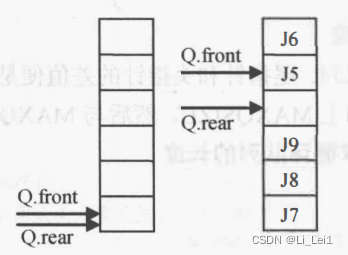 
全部代码如下
#include<stdio.h>
#include<stdlib.h>
#define MAXQSIZE 10
typedef int QElemType;
typedef struct {
QElemType data[MAXQSIZE];
int front;
int rear;
} sqQueue;
sqQueue *QueueCreate() {
sqQueue *q = (sqQueue *)malloc(sizeof(sqQueue));
q->front = q->rear = 0;
printf("初始化完成!\n");
return q;
}
void QueueLength(sqQueue *q) {
printf("队列长度:%d\n",(q->rear-q->front+MAXQSIZE) % MAXQSIZE);
}
void EnterQueue(sqQueue *q, QElemType a) {
if ((q->rear + 1) % MAXQSIZE == q->front) {
printf("队满!\n");
}
else {
q->data[q->rear] = a;
q->rear = (q->rear + 1) % MAXQSIZE;
}
}
void ExitQueue(sqQueue *q) {
if (q->front == q->rear) {
printf("队空!");
}
else {
printf("出队元素:%d\n", q->data[q->front]);
q -> front = (q->front + 1) % MAXQSIZE;
}
}
void PrintQueue(sqQueue *q) {
int t = q->front;
while (q->front != q->rear) {
printf("%d ", q->data[t]);
++t;
t %= MAXQSIZE;
if (t == q->rear) {
printf("\n");
break;
}
}
}
int main() {
sqQueue *q;
int le;
q = QueueCreate();
for (int i = 1; i < 6; ++i) {
EnterQueue(q, i);
}
PrintQueue(q);
ExitQueue(q);
QueueLength(q);
ExitQueue(q);
QueueLength(q);
PrintQueue(q);
EnterQueue(q, 10);
EnterQueue(q, 20);
PrintQueue(q);
return 0;
}
2.链队
一个链队显然需要两个分别指示队头和队尾的指针(分别称为头指针和尾指针)才能唯一确定。 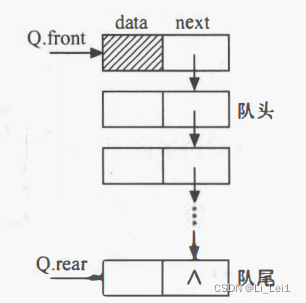 链队的操作即为单链表插入和删除操作的特殊情况,只是需进一步修改尾指针或头指针。链队只在链表头处插入,在链表尾部删除。 在链队出队操作时还要考虑当队列中最后一个元素被删后,队列尾指针也丢 失了,因此需对队尾指针重新赋值(指向头结点)。
队列的链式存储结构: 这与单链表有些不同,多了头指针和尾指针,而单链表只有头结点或头指针
typedef int QElemType;
typedef struct QueueNode {
QElemType data;
struct QueueNode *next;
} Queuenode, *QueuePtr;
typedef struct {
QueuePtr front;
QueuePtr rear;
} LinkQueue;
全部代码如下:
#include<stdio.h>
#include<stdlib.h>
#define N 10
typedef int QElemType;
typedef struct QueueNode {
QElemType data;
struct QueueNode *next;
} Queuenode, *QueuePtr;
typedef struct {
QueuePtr front;
QueuePtr rear;
} LinkQueue;
LinkQueue CreateQueuelist(LinkQueue Q) {
QueuePtr L = (QueuePtr)malloc(sizeof(Queuenode));
Q.front = Q.rear = L;
Q.front->next = NULL;
return Q;
}
LinkQueue EntQueuelist(LinkQueue Q, QElemType a) {
QueuePtr temp = (QueuePtr)malloc(sizeof(Queuenode));
temp->data = a;
temp->next = NULL;
Q.rear->next = temp;
Q.rear = temp;
return Q;
}
void ExtQueuelist(LinkQueue Q) {
if (Q.front == Q.rear) {
printf("空队列!\n");
}
else {
QueuePtr temp;
temp = Q.front->next;
Q.front->next = temp->next;
printf("出队元素:%d\n", temp->data);
if (Q.rear == temp) {
Q.rear = Q.front;
}
free(temp);
}
}
void PrintQueue(LinkQueue Q) {
QueuePtr h;
h = Q.front->next;
if (h == NULL) {
printf("空链表\n");
}
while (h) {
printf("%d ", h->data);
h = h->next;
}
printf("\n");
}
int main() {
LinkQueue Q;
Q = CreateQueuelist(Q);
for (int i = 0; i < 6; ++i) {
Q = EntQueuelist(Q, i+1);
}
PrintQueue(Q);
ExtQueuelist(Q);
Q = EntQueuelist(Q, 100);
ExtQueuelist(Q);
PrintQueue(Q);
return 0;
}
|