本周小结:数据类型,指针,数组运用;
一。数据结构:
1.枚举:enum :枚举类型的名字(名字1,名字2,...,名字n){从0开始一直到n}
枚举实际上是以整数(类型为int)作为内部计算和外部输入输出的,当需要一些可以排列起来的常量值时,定义枚举的意义九四给了这些常量的名字。
这是慕课上关于枚举的例题:
enum colors{red,yellow,green};
创建三个常量,red的值是0,yellow的值是1,green的值是2;
#include<stdio.h>
enum color{ red,yellow,green};
void f(enum color c);
int main(void)
{
enum color t = red;
scanf("%d",&t);
f(t);
return 0;
}0
void f(enum color c)
{
printf("%d\n",c);
}
套路:自动计数的枚举(慕课上的)这个突出枚举使用的好处
#include<stdio.h>
enum COLOR {RED,YELLOW,GREEN,NUMCOLORS};
int main( int argc,char const *argc[])
{
int color = -1;
char *ColorNames[NumCOLORS] = {"red","yellow","green",};
char *colorName = NULL;
printf("请输入你喜欢的颜色的代码:");
scanf("%d",&color);
if( color >=0 && color < NumCOLORS){
colorName ColorNames[color];
}else {
colorName = "unknown";
}
printf("你喜欢的颜色是%s\n",colorName);
return 0;
}
声名枚举量的时候可以指定值
enum COLOR{RED=1,YELLOW,GREEN=5}
2.结构:
1.声明结构类型:
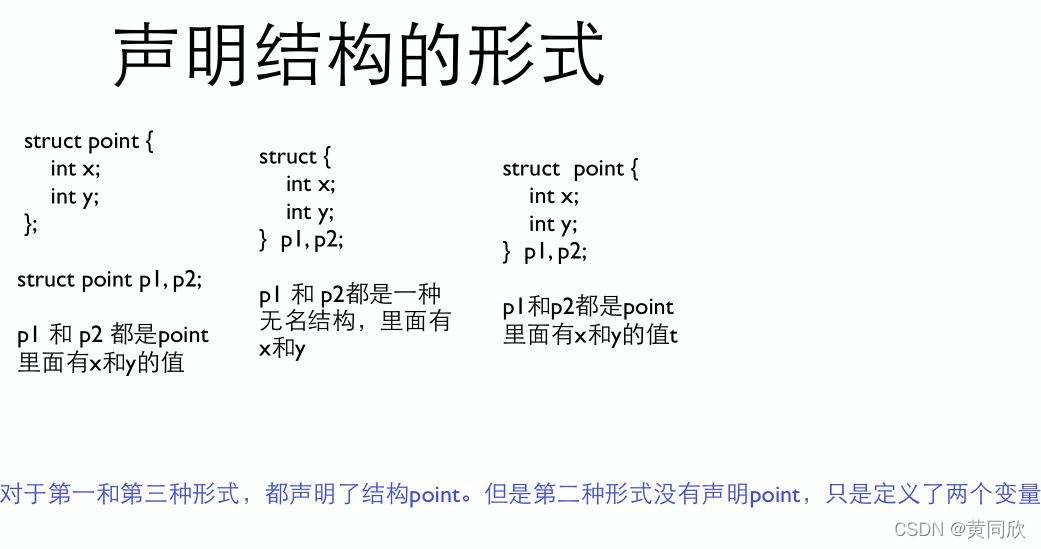
?2.结构用。运算符和名字访问器成员
例:today.day
student.firstName
3.结构与函数
输入结构->
#include<stdio.h>
struct point{
int x;
int y;
};
void getStruct(struct point);
void output(struct point);
int main()
{
struct point y = {0,0};
getStruct(y);
output(y);
}
void getStruct(struct point p)
{
scanf("%d",&p.x);
scanf("%d",&p.y);
printf("%d,%d\t",p.x,p.y);
}
N
void output(struct point p)
{
printf("%d,%d",p.x,p.y);
}
因为函数读入p的数值之后,没有任何东西回到main,所以y还是{0.0}(这个地方当时想不通)
解决方案: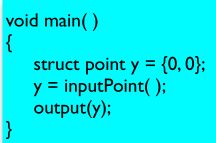 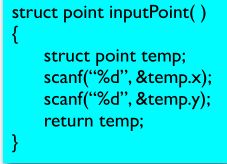
?4.结构指针参数:
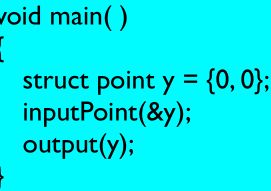 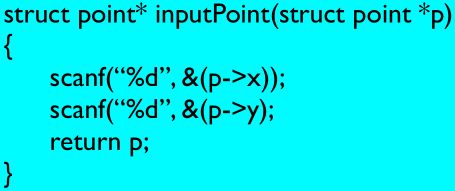
?二.本周练习:
1.结构类型(学生成绩统计)(菜鸟)
#include <stdio.h>
struct student
{
char name[50];
int roll;
float marks;
} s;
int main()
{
printf("输入信息:\n");
printf("名字: ");
scanf("%s", s.name);
printf("编号: ");
scanf("%d", &s.roll);
printf("成绩: ");
scanf("%f", &s.marks);
printf("显示信息:\n");
printf("名字: ");
puts(s.name);
printf("编号: %d\n",s.roll);
printf("成绩: %.1f\n", s.marks);
return 0;
}
?2.结构(复数相加)(菜鸟)
#include <stdio.h>
typedef struct complex
{
float real;
float imag;
} complex;
complex add(complex n1,complex n2);
int main()
{
complex n1, n2, temp;
printf("第一个复数 \n");
printf("输入实部和虚部:\n");
scanf("%f %f", &n1.real, &n1.imag);
printf("\n第二个复数 \n");
printf("输入实部和虚部:\n");
scanf("%f %f", &n2.real, &n2.imag);
temp = add(n1, n2);
printf("Sum = %.1f + %.1fi", temp.real, temp.imag);
return 0;
}
complex add(complex n1, complex n2)
{
complex temp;
temp.real = n1.real + n2.real;
temp.imag = n1.imag + n2.imag;
return(temp);
}
?3.指针访问数组
#include <stdio.h>
int main()
{
int data[5], i;
printf("输入元素: ");
for(i = 0; i < 5; ++i)
scanf("%d", data + i);
printf("你输入的是: \n");
for(i = 0; i < 5; ++i)
printf("%d\n", *(data + i));
return 0;
}
4.二维数组的叠加
#include<stdio.h>
int main()
{
int a[10][10];
int i,j,x,k;
scanf("%d",&x);
for(k=0;k<x-1;k++)
{
for(i=k;i<x-k;i++)
{
for(j=k;j<x-k;j++)
a[i][j]=k+1;
}
}
for(i=0;i<x;i++)
{
for(j=0;j<x;j++)
{
printf("%4d ",a[i][j]);
}
printf("\n\n");
}
}
5.二维数组的转置
#include<stdio.h>
int main()
{
int a[3][3]={1,2,3,4,5,6,7,8,9};
int i,j;
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
printf("%d",a[i][j]);
}
printf("\n");
}
printf("\n");
for(j=0;j<3;j++)
{
for(i=0;i<3;i++)
{
printf("%d",a[i][j]);
}
printf("\n");
}
}
6.杨辉三角形
#include<stdio.h>
int main()
{
int a[10][10];
int i,j,x,z;
scanf("%d",&x);
for(i=0;i<x;i++)
{
for(j=0;j<x;j++)
{
if(j==0||i==j)
{
a[i][j]=1;
}
else
{
a[i][j]=a[i-1][j-1]+a[i-1][j];
}
}
}
for(i=0;i<x;i++)
{
for(j=0;j<x;j++)
{
if(i>=j)
printf("%d ",a[i][j]);
}
printf("\n");
}
}
这个环形二维数组(本周c课老师出的题,本想根据杨辉三角敲出来,但是还存在一些问题)
#include<stdio.h>
int main()
{
int a[10][10]={0};
int i,j,x,n=1;
scanf("%d",&x);
for(int y=2;y>0;y--)
{
for(j=0;j<x;j++)
{
a[0][j]=n++;
}
printf("%d\n",j);
for(i=1;i<x;i++)
{
a[i][x]=n++;
}
printf("%d\n",i);
for(j--;i>0;j--)
{
a[x][j]=n++;
}
printf("%d\n",j);
for(i--;i>0;i--)
{
a[i][0]=n++;
}
printf("%d",i);
}
for(i=0;i<x;i++)
{
for(j=0;j<x;j++)
{
printf("%d",a[i][j]);
}
}
}
|