实验目的:
1.掌握C++语言多态性的基本概念 2.掌握运算符重载函数的声明和定义方法
运算符重载:
1.运算符重载是通过创建运算符重载函数来实现的,运算符重载函数可以是在类外定义的普通函数,也可以是类的成员函数或友元函数。 C++为运算符重载提供了一种方法,即在进行运算符重载时,必须定义一个运算符重载函数,其名字为operator,后随一个要重载的运算符。
2.运算符重载的函数格式: 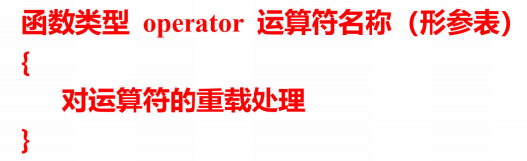 3.C++中绝大多数的运算符允许重载,不能重载的运算符有以下几个: . 成员访问运算符; .* 成员指针访问运算符; :: 作用域运算符; Sizeof 长度运算符; ?: 条件运算符; 4.C++语言只能已有的运算符进行重载,不允许用户自己定义运算符;
实验程序:
1.编写一个程序,实现两个复数的相加,类外定义运算符重载函数;
#include "sy7.h"
using namespace std;
class Complex {
public:
double real;
double imag;
Complex(double r=0, double i=0)
{
real = r;
imag = i;
}
};
Complex operator+(Complex co1, Complex co2)
{
Complex temp;
temp.real = co1.real + co2.real;
temp.imag = co1.imag + co2.imag;
return temp;
}
int main()
{
Complex com1(1.1, 2.2), com2(3.3, 4.4), total1, total2;
total1 = operator+(com1, com2);
cout << "real1=" << total1.real << " " << "imag1=" << total1.imag << endl;
total2 = com1 + com2;
cout << "real2=" << total2.real << " " << "imag2=" << total2.imag << endl;
return 0;
}
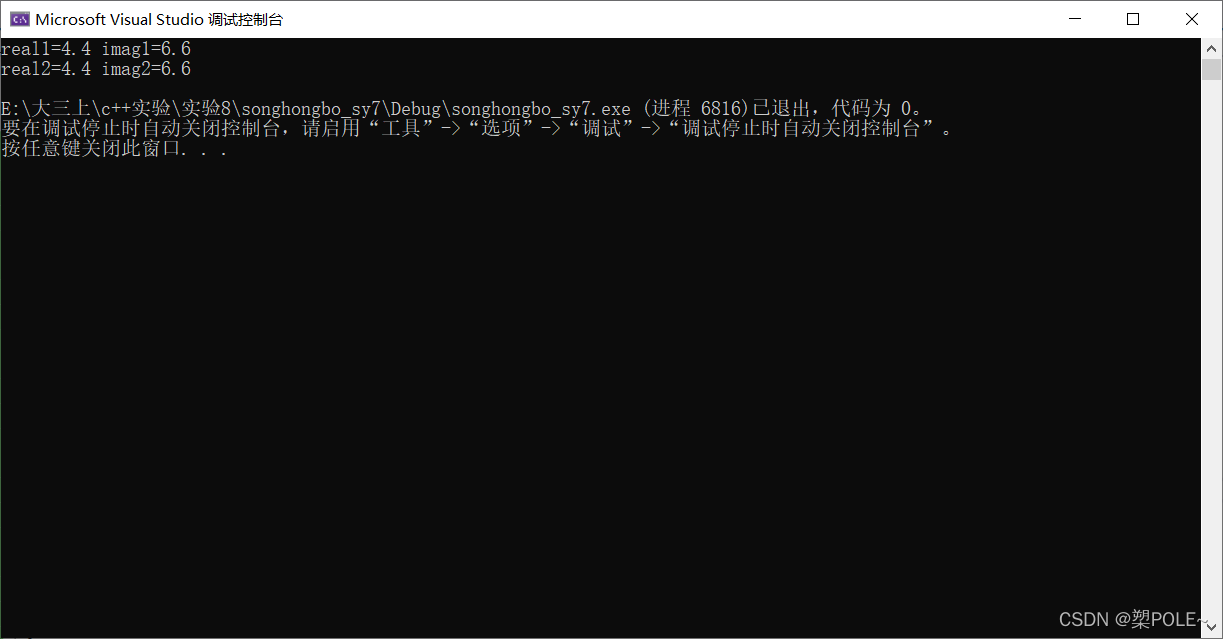
2.编写一个程序,实现两个复数的相加,友元运算符重载函数。
#include "sy7.h"
using namespace std;
class Complex {
double real;
double imag;
public:
Complex(double r = 0, double i = 0)
{
real = r;
imag = i;
}
void print();
friend Complex operator+(Complex co1, Complex co2);
};
Complex operator+(Complex co1, Complex co2)
{
Complex temp;
temp.real = co1.real + co2.real;
temp.imag = co1.imag + co2.imag;
return temp;
}
void Complex::print()
{
cout << "total real=" << real << " " << "total imag=" << imag << endl;
}
int main()
{
Complex com1(1.1, 2.2), com2(3.3, 4.4), total1;
total1 = com1 + com2;
total1.print();
return 0;
}
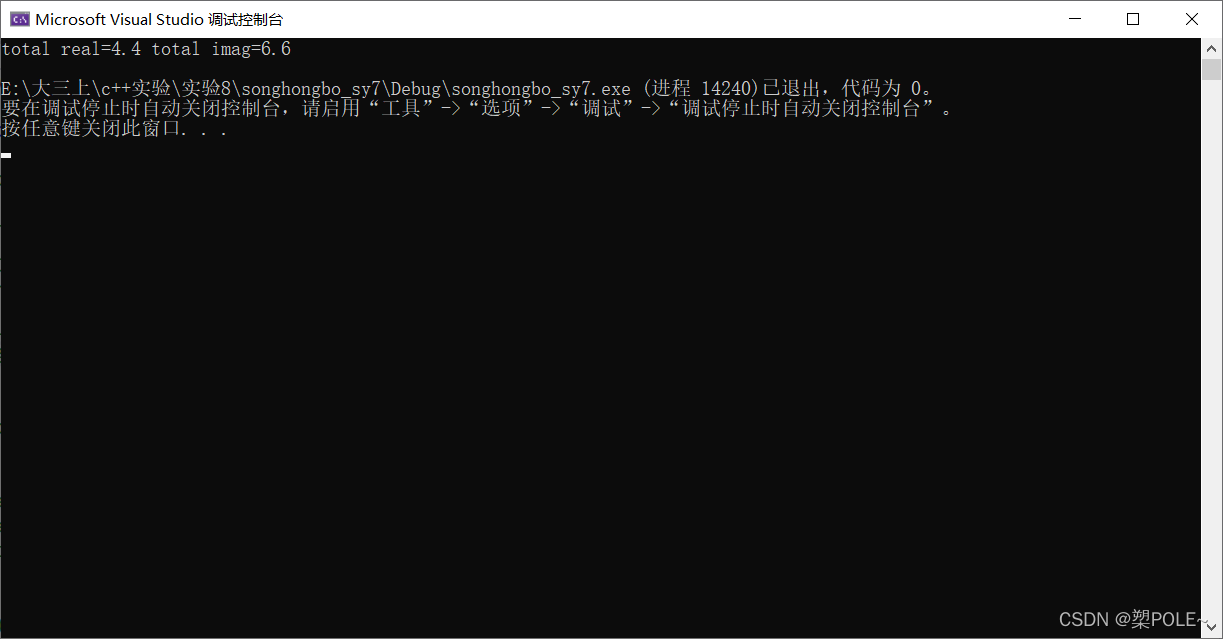 3.编写一个程序,实现两个复数的相加,成员运算符重载函数。 对双目运算符而言,成员运算符重载函数的形参表中仅有一个参数,它作为运算符的右操作数。 另一个操作数(左操作数)是隐含的,是该类的当前对象,他是通过this指针隐含传递给函数。
#include "sy7.h"
using namespace std;
class Complex {
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0)
{
real = r;
imag = i;
}
void print();
Complex operator+(Complex c);
};
Complex Complex::operator+(Complex c)
{
Complex temp;
temp.real = real + c.real;
temp.imag = imag + c.imag;
return temp;
}
void Complex::print()
{
cout << "total real=" << real << " " << "total imag=" << imag << endl;
}
int main()
{
Complex com1(2.3, 4.6), com2(3.6, 2.8), total1;
total1 = com1 + com2;
total1.print();
return 0;
}
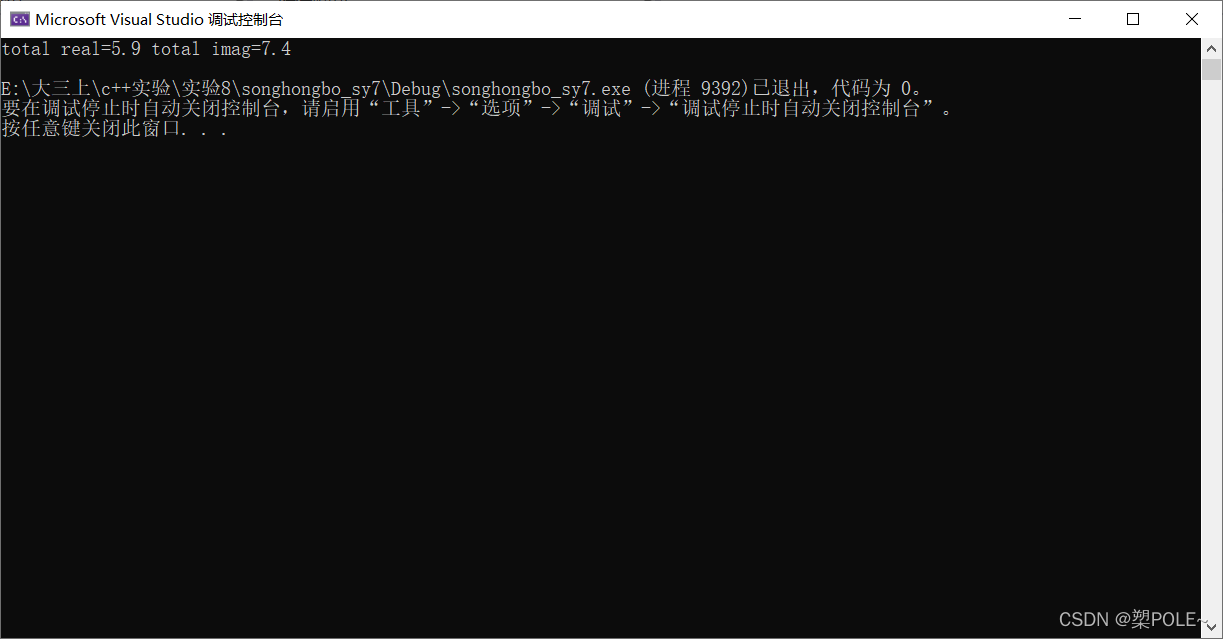
实验内容 :
编写一个程序,实现两个复数的乘法:
#include "sy7.h"
using namespace std;
class Complex {
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0)
{
real = r;
imag = i;
}
void print();
friend Complex operator*(Complex &a, Complex &b);
};
Complex operator*(Complex &a, Complex &b)
{
Complex temp;
temp.real = a.real * b.real-a.imag*b.imag;
temp.imag = a.real * b.imag+a.imag*b.real;
return temp;
}
void Complex::print()
{
cout << real;
if (imag > 0)
{
cout << "+";
}
if (imag != 0)
{
cout << imag << 'i' << endl;
}
}
int main()
{
Complex com1(1.1, 2.2), com2(3.3, 4.4), total1;
total1 = com1 * com2;
total1.print();
return 0;
}
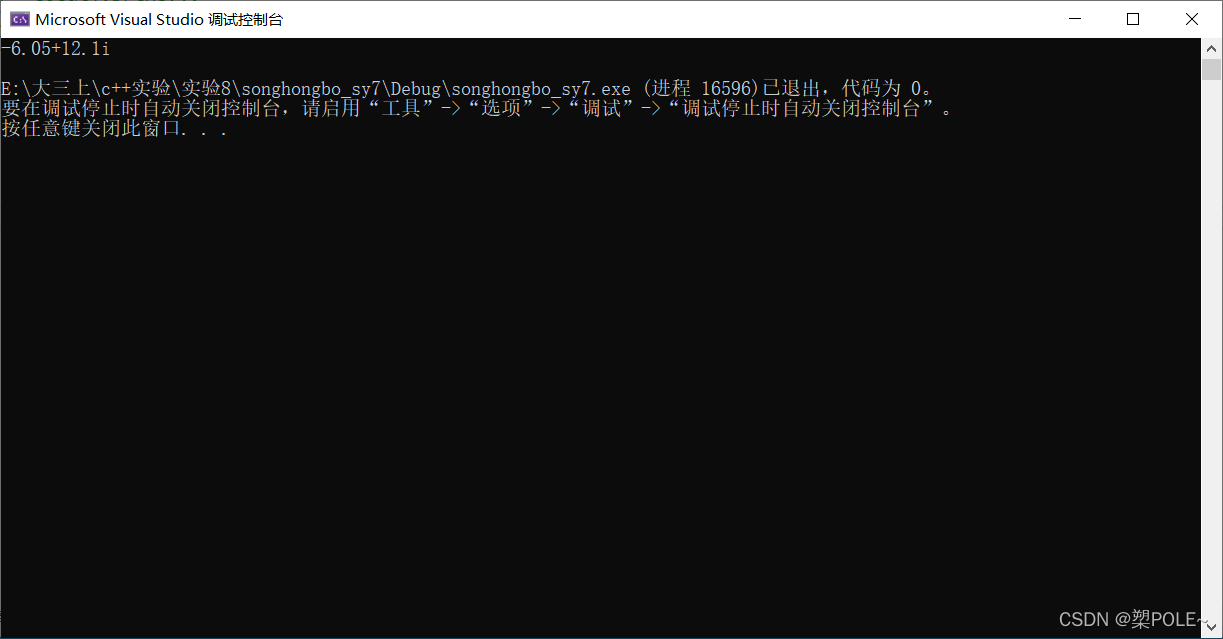 当用友元函数重载双目运算符时,两个操作数都要传递给运算符重载函数。
|