职工管理项目模型在编程作业随处可见,如【学生信息管理系统】、【图书馆管理系统】等等 这里给出【IT公司职工管理系统】的实现👇 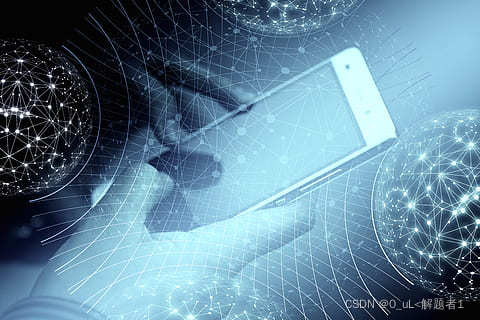 系统要求描述: 有一家小小的IT公司,只有三类员工:普通程序员、项目经理、公司董事,每个员工只有编号ID、姓名、代表职位的dID(1,2,3)。 要求你制作一个完善职工管理系统: 实现添加、查找、删除、清空、修改等操作 由于这是公司所用,不能使用那种只能使用一下的临时程序🌀
1.单文件?
#include<iostream>
#include<string>
using namespace std;
#include<fstream>
constexpr auto myFILE = "empFile.txt";
typedef unsigned int ui;
class Worker {
public:
Worker(ui id, string name, string dId) :m_id(id), m_name(name), m_DeptId(dId) {}
virtual void showInfo() = 0;
virtual string getDeptName() = 0;
ui m_id;
string m_name;
string m_DeptId;
};
class Employee :public Worker {
public:
Employee(ui id, string name, string dId) :Worker(id, name, dId) {}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:完成经理交给的项目,满足客户需求" << endl;
}
string getDeptName() {
return string("普通程序员");
}
};
class Manager :public Worker {
public:
Manager(ui id, string name, string dId) :Worker(id, name, dId) {}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:完成老板的任务,下发任务给普通程序员" << endl;
}
string getDeptName() {
return string("项目经理");
}
};
class Boss :public Worker {
public:
Boss(ui id, string name, string dId) :Worker(id, name, dId) {}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:管理公司职责,全局掌控,发布任务" << endl;
}
string getDeptName() {
return string("IT公司董事");
}
};
class workerManager
{
private:
int m_EmpNum;
Worker** m_Emparray;
bool m_FileIsEmpty;
void save() {
ofstream ofs;
ofs.open(myFILE, ios::out);
for (int i = 0; i < this->m_EmpNum; i++) {
ofs << this->m_Emparray[i]->m_id << ' '
<< this->m_Emparray[i]->m_name << ' '
<< this->m_Emparray[i]->m_DeptId << endl;
cout << "Id为" << this->m_Emparray[i]->m_id << "的员工" << "保存成功" << endl;
}
ofs.close();
return;
}
void up_qssort(Worker** m_Emparray, int l, int r) {
int i = l, j = r, flag = this->m_Emparray[l + (r - l) / 2]->m_id;
do {
while (this->m_Emparray[i]->m_id < flag)i++;
while (this->m_Emparray[j]->m_id > flag)j--;
if (i <= j) {
swap(this->m_Emparray[i]->m_id, this->m_Emparray[j]->m_id);
i++;
j--;
}
} while (i <= j);
if (l < j)up_qssort(m_Emparray, l, j);
if (i < r)up_qssort(m_Emparray, i, r);
}
void low_qssort(Worker** m_Emparray, int l, int r) {
int i = l, j = r, flag = this->m_Emparray[l + (r - l) / 2]->m_id;
do {
while (this->m_Emparray[i]->m_id > flag)i++;
while (this->m_Emparray[j]->m_id < flag)j--;
if (i <= j) {
swap(this->m_Emparray[i]->m_id, this->m_Emparray[j]->m_id);
i++;
j--;
}
} while (i <= j);
if (l < j)low_qssort(m_Emparray, l, j);
if (i < r)low_qssort(m_Emparray, i, r);
}
public:
workerManager() {
ifstream ifs;
ifs.open(myFILE, ios::in);
if (!ifs.is_open()) {
cout << "-----------------------------------------------------" << endl;
cout << "提示:--!!!文件未创建,运行系统可自动生成!!! --" << endl;
cout << "-----------------------------------------------------" << endl;
this->m_EmpNum = 0;
this->m_Emparray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof()) {
cout << "--------------------------------------------------------" << endl;
cout << "提示:--!!!职工管理文件为空,等待添加数据信息!!!--" << endl;
cout << "--------------------------------------------------------" << endl;
this->m_EmpNum = 0;
this->m_Emparray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
int num = this->get_EmpNum();
cout << "--------------------------------------------------------" << endl;
cout << "提示:--《《《我公司现有的员工为:" << num << "位》》》 ---" << endl;
cout << "--------------------------------------------------------" << endl;
this->m_EmpNum = num;
this->m_Emparray = new Worker * [this->m_EmpNum];
this->init_Emp();
}
void init_Emp() {
ifstream ifs;
ifs.open(myFILE, ios::in);
ui id;
string name;
string dId;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> dId) {
Worker* worker = NULL;
if (dId == "1") {
worker = new Employee(id, name, dId);
}
else if (dId == "2") {
worker = new Manager(id, name, dId);
}
else {
worker = new Boss(id, name, dId);
}
this->m_Emparray[index++] = worker;
}
ifs.close();
}
int get_EmpNum() {
ifstream ifs;
ifs.open(myFILE, ios::in);
ui id;
string name;
string dId;
int cnt = 0;
while (ifs >> id && ifs >> name && ifs >> dId) {
cnt++;
}
return cnt;
}
int Find_worker(int id) {
int index = -1;
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i]->m_id == id) {
index = i;
break;
}
}
return index;
}
void Show_Menu() {
cout << " 《开始菜单》 " << endl;
cout << " ***********************************************" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ********** 欢迎使用程序员管理系统 ********" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ********** | 0.退出管理程序 | ********" << endl;
cout << " ********** | 1.增加职工信息 | ********" << endl;
cout << " ********** | 2.显示职工信息 | ********" << endl;
cout << " ********** | 3.删除离职职工 | ********" << endl;
cout << " ********** | 4.修改职工信息 | ********" << endl;
cout << " ********** | 5.查找职工信息 | ********" << endl;
cout << " ********** | 6.按照编号排序 | ********" << endl;
cout << " ********** | 7.清空职工档案 | ********" << endl;
cout << " ********** | 8.现有员工总数 | ********" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ***********************************************" << endl;
}
void Start() {
while (true) {
this->Show_Menu();
cout << endl;
cout << "Acting.........." << endl;
cout << " ********** -------请输入选项------ ******" << endl;
int select;
cin >> select;
switch (select)
{
case 0:
this->exitSystem();
break;
case 1:
this->Add_Emp();
break;
case 2:
this->Show_Emp();
break;
case 3:
this->del_Emp();
break;
case 4:
this->Mod_Emp();
break;
case 5:
this->Find_theEmp();
break;
case 6:
this->Sort_Emp();
break;
case 7:
this->Clean_file();
break;
case 8:
this->gettotal();
break;
default:
cout << "无此类选项,请确认后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void exitSystem() {
cout << "确定退出吗?再次输入0可取消此次操作" << endl;
bool jd;
cin >> jd;
if (jd) {
cout << "------已退出系统,欢迎下次使用--------" << endl;
system("pause");
exit(0);
}
else {
cout << "-------成功取消本次操作,系统继续执行------" << endl;
system("pause");
system("cls");
return;
}
}
void Add_Emp() {
cout << " 请输入所添加员工的数量:" << endl;
int addNum = 0;
cin >> addNum;
while (addNum <= 0) {
cout << "输入错误,请重新输入!!!" << endl;
cin >> addNum;
}
int newSize = this->m_EmpNum + addNum;
Worker** newSpace = new Worker * [newSize];
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
newSpace[i] = this->m_Emparray[i];
}
}
cout << "执行添加操作!____..." << endl;
for (int i = 0; i < addNum; i++) {
ui id;
string name;
string dSelect;
Worker* worker = NULL;
cout << "请输入第 " << i + 1 << " 个新员工的编号(数字形式): " << endl;
cin >> id;
int index = this->Find_worker(id);
while (index != -1) {
cout << "wrong!系统已存在此Id,请重新输入!----【注:按照数字形式输入】" << endl;
cin >> id;
index = this->Find_worker(id);
}
cout << "请输入第 " << i + 1 << " 个新员工的姓名: " << endl;
cin >> name;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通程序员" << endl;
cout << "2.项目经理" << endl;
cout << "3.公司董事" << endl;
cin >> dSelect;
while (dSelect != "1" && dSelect != "2" && dSelect != "3") {
cout << "选项输入错误,请按照规则重新输入!!!" << endl;
cin >> dSelect;
}
if (dSelect == "1") {
worker = new Employee(id, name, "1");
}
else if (dSelect == "2") {
worker = new Manager(id, name, "2");
}
else {
worker = new Boss(id, name, "3");
}
newSpace[this->m_EmpNum + i] = worker;
cout << "--------已成功添加一个新员工!!!--------" << endl;
cout << endl;
}
delete[]this->m_Emparray;
this->m_Emparray = newSpace;
this->m_EmpNum = newSize;
cout << "成功添加 " << addNum << " 位新职工" << endl;
this->m_FileIsEmpty = false;
this->save();
system("pause");
system("cls");
return;
}
void Show_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
}
else {
for (int i = 0; i < this->m_EmpNum; i++) {
this->m_Emparray[i]->showInfo();
}
}
system("pause");
system("cls");
return;
}
void del_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
return;
}
int id;
cout << "请输入想要删除的职工编号(数字串形式)———" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index == -1)cout << "删除失败!系统暂未录入该员工的信息!" << endl;
else {
for (int i = index; i < this->m_EmpNum - 1; i++) {
this->m_Emparray[i] = this->m_Emparray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功!!!" << endl;
}
system("pause");
system("cls");
return;
}
void Mod_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
return;
}
int id;
cout << "请输入想要修改的职工编号(数字串形式)———" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index == -1)cout << "无法修改!系统暂未录入该员工的信息!" << endl;
else {
delete this->m_Emparray[index];
ui new_id;
string new_name;
string new_dId;
cout << "已查到:Id为" << id << "的职工!执行修改操作——";
cout << "请输入新的Id..." << endl;
cin >> new_id;
cout << "请输入新的姓名..." << endl;
cin >> new_name;
cout << "请输入新的岗位编号..." << endl;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通程序员" << endl;
cout << "2.项目经理" << endl;
cout << "3.公司董事" << endl;
cin >> new_dId;
while (new_dId != "1" && new_dId != "2" && new_dId != "3") {
cout << "选项输入错误,请按照规则重新输入!!!" << endl;
cin >> new_dId;
}
Worker* worker = NULL;
if (new_dId == "1") {
worker = new Employee(new_id, new_name, "1");
}
else if (new_dId == "2") {
worker = new Manager(new_id, new_name, "2");
}
else {
worker = new Boss(new_id, new_name, "3");
}
this->m_Emparray[index] = worker;
cout << "|||修改成功|||" << endl;
this->save();
}
system("pause");
system("cls");
return;
}
void Find_theEmp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
system("puase");
system("cls");
return;
}
cout << "请输入您想要的查找方式..." << endl;
cout << "1.按Id查找 ------ 2.按职工姓名查找" << endl;
cout << "选择..." << endl;
string select;
cin >> select;
while (select != "1" && select != "2") {
cout << "选项输入错误!请正确输入 1|2 !" << endl;
cin >> select;
}
if (select == "1") {
int id;
cout << "请输入要【查找】职工的编号:" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index == -1)cout << "系统暂未录入该Id的职工信息!" << endl;
else {
cout << "查找成功!该员工的信息如下——" << endl;
this->m_Emparray[index]->showInfo();
}
}
else {
string name;
bool no_nameflag = true;
cout << "请输入所要查找的姓名:" << endl;
cin >> name;
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i]->m_name == name) {
no_nameflag = false;
cout << "查找成功!" << endl;
this->m_Emparray[i]->showInfo();
}
}
if (no_nameflag)cout << "姓名为:" << name << "的员工暂未录入系统!" << endl;
}
system("pause");
system("cls");
return;
}
void Sort_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件" << endl;
system("pause");
system("cls");
return;
}
cout << "请选择排序方式..." << endl;
cout << "0 降序/ 1 升序" << endl;
bool isupp;
cin >> isupp;
if (isupp) {
this->up_qssort(this->m_Emparray, 0, this->m_EmpNum - 1);
}
else {
this->low_qssort(this->m_Emparray, 0, this->m_EmpNum - 1);
}
cout << "排序完毕!!!" << endl;
this->save();
system("pause");
system("cls");
return;
}
void Clean_file() {
cout << "确认清空?" << endl;
cout << "1.确定" << endl;
cout << "0.取消" << endl;
bool jd;
cin >> jd;
if (jd) {
ofstream ofs(myFILE, ios::trunc);
ofs.close();
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_Emparray[i];
this->m_Emparray[i] = NULL;
}
}
delete[] this->m_Emparray;
this->m_Emparray = NULL;
this->m_EmpNum = 0;
this->m_FileIsEmpty = true;
cout << "清空成功!" << endl;
system("pause");
system("cls");
return;
}
}
void gettotal() {
cout << "公司现有的员工总数为:" << this->m_EmpNum << endl;
system("pause");
system("cls");
return;
}
~workerManager() {
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i] != NULL)delete this->m_Emparray[i];
}
}
delete[] this->m_Emparray;
this->m_Emparray = NULL;
}
};
using namespace std;
int main() {
workerManager works;
works.Start();
system("pause");
return 0;
}
🐉🐉🐴🐴分割线------------------
2.双(多)文件?
【1】workerManager.h
#pragma once
#include<iostream>
#include<string>
using namespace std;
#include<fstream>
constexpr auto myFILE ="empFile.txt";
typedef unsigned int ui;
class Worker {
public:
Worker(ui id, string name, string dId):m_id(id),m_name(name),m_DeptId(dId){}
virtual void showInfo() = 0;
virtual string getDeptName() = 0;
ui m_id;
string m_name;
string m_DeptId;
};
class Employee :public Worker {
public:
Employee(ui id, string name, string dId):Worker(id,name,dId){}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:完成经理交给的项目,满足客户需求" << endl;
}
string getDeptName() {
return string("普通程序员");
}
};
class Manager :public Worker {
public:
Manager(ui id, string name, string dId) :Worker(id, name, dId) {}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:完成老板的任务,下发任务给普通程序员" << endl;
}
string getDeptName() {
return string("项目经理");
}
};
class Boss :public Worker {
public:
Boss(ui id, string name, string dId) :Worker(id, name, dId) {}
void showInfo() {
cout << "职工编号:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:管理公司职责,全局掌控,发布任务" << endl;
}
string getDeptName() {
return string("IT公司董事");
}
};
class workerManager
{
private:
int m_EmpNum;
Worker** m_Emparray;
bool m_FileIsEmpty;
void save() {
ofstream ofs;
ofs.open(myFILE,ios::out);
for (int i = 0; i < this->m_EmpNum; i++) {
ofs << this->m_Emparray[i]->m_id << ' '
<< this->m_Emparray[i]->m_name << ' '
<< this->m_Emparray[i]->m_DeptId << endl;
cout << "Id为" << this->m_Emparray[i]->m_id << "的员工" << "保存成功" << endl;
}
ofs.close();
return;
}
void up_qssort(Worker** m_Emparray, int l, int r) {
int i = l, j = r, flag = this->m_Emparray[l + (r - l) / 2]->m_id;
do {
while (this->m_Emparray[i]->m_id < flag)i++;
while (this->m_Emparray[j]->m_id > flag)j--;
if (i <= j) {
swap(this->m_Emparray[i]->m_id, this->m_Emparray[j]->m_id);
i++;
j--;
}
} while (i <= j);
if (l < j)up_qssort(m_Emparray, l, j);
if (i < r)up_qssort(m_Emparray, i, r);
}
void low_qssort(Worker** m_Emparray, int l, int r) {
int i = l, j = r, flag = this->m_Emparray[l + (r - l) / 2]->m_id;
do {
while (this->m_Emparray[i]->m_id > flag)i++;
while (this->m_Emparray[j]->m_id < flag)j--;
if (i <= j) {
swap(this->m_Emparray[i]->m_id, this->m_Emparray[j]->m_id);
i++;
j--;
}
} while (i <= j);
if (l < j)low_qssort(m_Emparray, l, j);
if (i < r)low_qssort(m_Emparray, i, r);
}
public:
workerManager() {
ifstream ifs;
ifs.open(myFILE, ios::in);
if (!ifs.is_open()) {
cout << "-----------------------------------------------------" << endl;
cout << "提示:--!!!文件未创建,运行系统可自动生成!!! --" << endl;
cout << "-----------------------------------------------------" << endl;
this->m_EmpNum = 0;
this->m_Emparray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof()) {
cout << "--------------------------------------------------------" << endl;
cout << "提示:--!!!职工管理文件为空,等待添加数据信息!!!--" << endl;
cout << "--------------------------------------------------------" << endl;
this->m_EmpNum = 0;
this->m_Emparray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
int num = this->get_EmpNum();
cout << "--------------------------------------------------------" << endl;
cout << "提示:--《《《我公司现有的员工为:"<<num<<"位》》》 ---" << endl;
cout << "--------------------------------------------------------" << endl;
this->m_EmpNum = num;
this->m_Emparray = new Worker * [this->m_EmpNum];
this->init_Emp();
}
void init_Emp() {
ifstream ifs;
ifs.open(myFILE,ios::in);
ui id;
string name;
string dId;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> dId) {
Worker* worker = NULL;
if (dId == "1") {
worker = new Employee(id, name, dId);
}
else if (dId == "2") {
worker = new Manager(id, name, dId);
}
else {
worker = new Boss(id, name, dId);
}
this->m_Emparray[index++] = worker;
}
ifs.close();
}
int get_EmpNum() {
ifstream ifs;
ifs.open(myFILE, ios::in);
ui id;
string name;
string dId;
int cnt = 0;
while (ifs >> id && ifs >> name && ifs >> dId) {
cnt++;
}
return cnt;
}
int Find_worker(int id) {
int index = -1;
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i]->m_id == id) {
index = i;
break;
}
}
return index;
}
void Show_Menu() {
cout << " 《开始菜单》 " << endl;
cout << " ***********************************************" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ********** 欢迎使用程序员管理系统 ********" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ********** | 0.退出管理程序 | ********" << endl;
cout << " ********** | 1.增加职工信息 | ********" << endl;
cout << " ********** | 2.显示职工信息 | ********" << endl;
cout << " ********** | 3.删除离职职工 | ********" << endl;
cout << " ********** | 4.修改职工信息 | ********" << endl;
cout << " ********** | 5.查找职工信息 | ********" << endl;
cout << " ********** | 6.按照编号排序 | ********" << endl;
cout << " ********** | 7.清空职工档案 | ********" << endl;
cout << " ********** | 8.现有员工总数 | ********" << endl;
cout << " ********** ---------------------- ********" << endl;
cout << " ***********************************************" << endl;
}
void Start() {
while (true) {
this->Show_Menu();
cout << endl;
cout << "Acting.........." << endl;
cout << " ********** -------请输入选项------ ******" << endl;
int select;
cin >> select;
switch (select)
{
case 0:
this->exitSystem();
break;
case 1:
this->Add_Emp();
break;
case 2:
this->Show_Emp();
break;
case 3:
this->del_Emp();
break;
case 4:
this->Mod_Emp();
break;
case 5:
this->Find_theEmp();
break;
case 6:
this->Sort_Emp();
break;
case 7:
this->Clean_file();
break;
case 8:
this->gettotal();
break;
default:
cout << "无此类选项,请确认后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void exitSystem() {
cout << "确定退出吗?再次输入0可取消此次操作" << endl;
bool jd;
cin >> jd;
if (jd) {
cout << "------已退出系统,欢迎下次使用--------" << endl;
system("pause");
exit(0);
}
else {
cout << "-------成功取消本次操作,系统继续执行------" << endl;
system("pause");
system("cls");
return;
}
}
void Add_Emp() {
cout << " 请输入所添加员工的数量:" << endl;
int addNum = 0;
cin >> addNum;
while (addNum <=0) {
cout << "输入错误,请重新输入!!!" << endl;
cin >> addNum;
}
int newSize = this->m_EmpNum + addNum;
Worker** newSpace = new Worker * [newSize];
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
newSpace[i] = this->m_Emparray[i];
}
}
cout << "执行添加操作!____..." << endl;
for (int i = 0; i < addNum; i++) {
ui id;
string name;
string dSelect;
Worker* worker = NULL;
cout << "请输入第 " << i + 1 << " 个新员工的编号(数字形式): " << endl;
cin >> id;
int index = this->Find_worker(id);
while(index != -1) {
cout << "wrong!系统已存在此Id,请重新输入!----【注:按照数字形式输入】" << endl;
cin >> id;
index = this->Find_worker(id);
}
cout << "请输入第 " << i + 1 << " 个新员工的姓名: " << endl;
cin >> name;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通程序员" << endl;
cout << "2.项目经理" << endl;
cout << "3.公司董事" << endl;
cin >> dSelect;
while (dSelect != "1" && dSelect != "2" && dSelect != "3") {
cout << "选项输入错误,请按照规则重新输入!!!" << endl;
cin >> dSelect;
}
if (dSelect == "1") {
worker = new Employee(id, name, "1");
}
else if (dSelect == "2") {
worker = new Manager(id, name, "2");
}
else {
worker = new Boss(id, name, "3");
}
newSpace[this->m_EmpNum + i] = worker;
cout << "--------已成功添加一个新员工!!!--------" << endl;
cout << endl;
}
delete[]this->m_Emparray;
this->m_Emparray = newSpace;
this->m_EmpNum = newSize;
cout << "成功添加 " << addNum << " 位新职工" << endl;
this->m_FileIsEmpty = false;
this->save();
system("pause");
system("cls");
return;
}
void Show_Emp(){
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
}
else {
for (int i = 0; i < this->m_EmpNum; i++) {
this->m_Emparray[i]->showInfo();
}
}
system("pause");
system("cls");
return;
}
void del_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
return;
}
int id;
cout << "请输入想要删除的职工编号(数字串形式)———" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index ==-1)cout << "删除失败!系统暂未录入该员工的信息!" << endl;
else {
for (int i = index; i < this->m_EmpNum - 1; i++) {
this->m_Emparray[i] = this->m_Emparray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功!!!" << endl;
}
system("pause");
system("cls");
return;
}
void Mod_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
return;
}
int id;
cout << "请输入想要修改的职工编号(数字串形式)———" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index == -1)cout << "无法修改!系统暂未录入该员工的信息!" << endl;
else {
delete this->m_Emparray[index];
ui new_id;
string new_name;
string new_dId;
cout << "已查到:Id为" << id << "的职工!执行修改操作——";
cout << "请输入新的Id..." << endl;
cin >> new_id;
cout << "请输入新的姓名..." << endl;
cin >> new_name;
cout << "请输入新的岗位编号..." << endl;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通程序员" << endl;
cout << "2.项目经理" << endl;
cout << "3.公司董事" << endl;
cin >> new_dId;
while (new_dId != "1" && new_dId != "2" && new_dId != "3") {
cout << "选项输入错误,请按照规则重新输入!!!" << endl;
cin >> new_dId;
}
Worker* worker = NULL;
if (new_dId == "1") {
worker = new Employee(new_id, new_name, "1");
}
else if (new_dId == "2") {
worker = new Manager(new_id,new_name, "2");
}
else {
worker = new Boss(new_id, new_name, "3");
}
this->m_Emparray[index] = worker;
cout << "|||修改成功|||" << endl;
this->save();
}
system("pause");
system("cls");
return;
}
void Find_theEmp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件!!!" << endl;
system("puase");
system("cls");
return;
}
cout << "请输入您想要的查找方式..." << endl;
cout << "1.按Id查找 ------ 2.按职工姓名查找" << endl;
cout << "选择..." << endl;
string select;
cin >> select;
while (select != "1" && select != "2") {
cout << "选项输入错误!请正确输入 1|2 !" << endl;
cin >> select;
}
if (select == "1") {
int id;
cout << "请输入要【查找】职工的编号:" << endl;
cin >> id;
int index = this->Find_worker(id);
if (index == -1)cout << "系统暂未录入该Id的职工信息!" << endl;
else {
cout << "查找成功!该员工的信息如下——" << endl;
this->m_Emparray[index]->showInfo();
}
}
else {
string name;
bool no_nameflag = true;
cout << "请输入所要查找的姓名:" << endl;
cin >> name;
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i]->m_name == name) {
no_nameflag = false;
cout << "查找成功!" << endl;
this->m_Emparray[i]->showInfo();
}
}
if (no_nameflag)cout << "姓名为:" << name << "的员工暂未录入系统!" << endl;
}
system("pause");
system("cls");
return;
}
void Sort_Emp() {
if (this->m_FileIsEmpty) {
cout << "系统暂无职工信息或者未创建文件" << endl;
system("pause");
system("cls");
return;
}
cout << "请选择排序方式..." << endl;
cout << "0 降序/ 1 升序" << endl;
bool isupp;
cin >> isupp;
if (isupp) {
this->up_qssort(this->m_Emparray, 0, this->m_EmpNum-1);
}
else {
this->low_qssort(this->m_Emparray, 0, this->m_EmpNum - 1);
}
cout << "排序完毕!!!" << endl;
this->save();
system("pause");
system("cls");
return;
}
void Clean_file() {
cout << "确认清空?" << endl;
cout << "1.确定" << endl;
cout << "0.取消" << endl;
bool jd;
cin >> jd;
if (jd) {
ofstream ofs(myFILE, ios::trunc);
ofs.close();
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_Emparray[i];
this->m_Emparray[i] = NULL;
}
}
delete[] this->m_Emparray;
this->m_Emparray = NULL;
this->m_EmpNum = 0;
this->m_FileIsEmpty = true;
cout << "清空成功!" << endl;
system("pause");
system("cls");
return;
}
}
void gettotal() {
cout <<"公司现有的员工总数为:"<< this->m_EmpNum << endl;
system("pause");
system("cls");
return ;
}
~workerManager() {
if (this->m_Emparray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_Emparray[i] != NULL)delete this->m_Emparray[i];
}
}
delete[] this->m_Emparray;
this->m_Emparray = NULL;
}
};
【2】workerManager.cpp
#include "workerManager.h"
【3】源.cpp
#include"workerManager.h"
using namespace std;
int main() {
workerManager works;
works.Start();
system("pause");
return 0;
}
3.效果展示?
【1】开始菜单
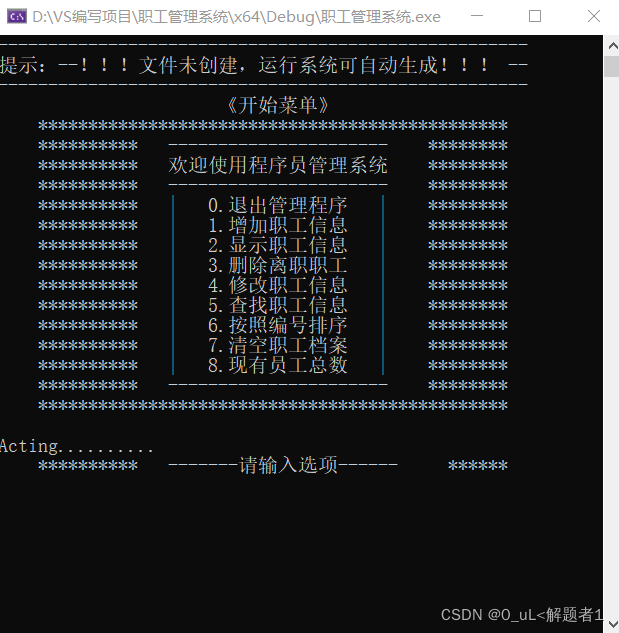 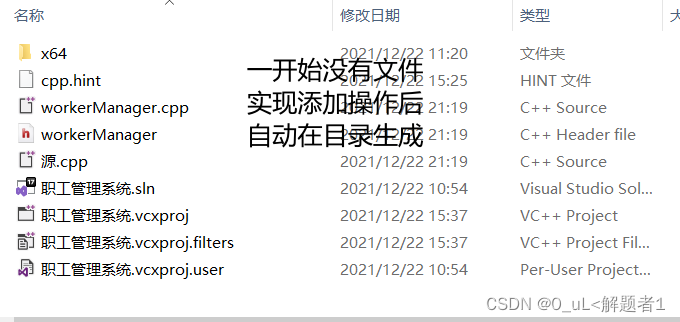
【2】添加职工
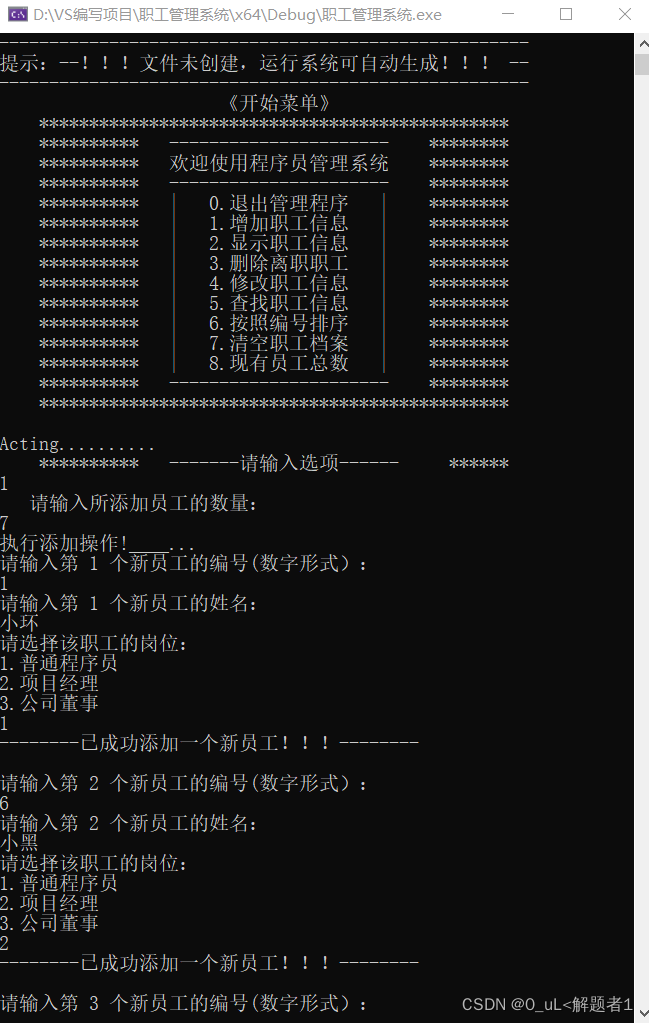
… … 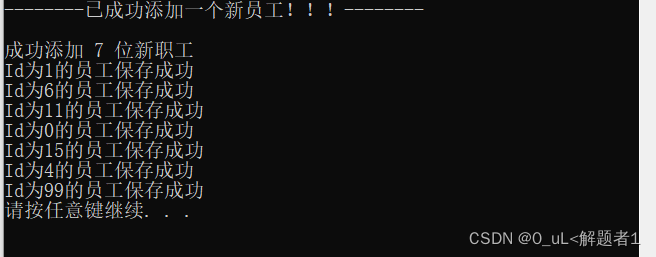
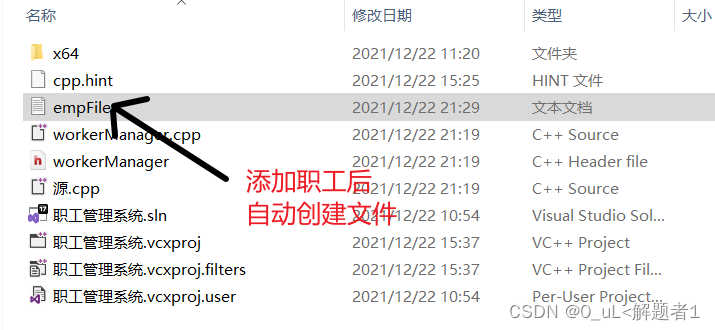 打开看看👇 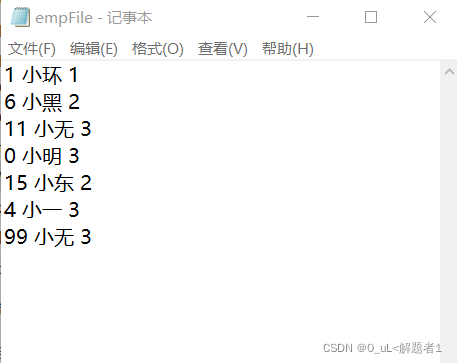 成功纳入职工数据ヾ(?°?°?)ノ゙
【3】查看全部职工信息
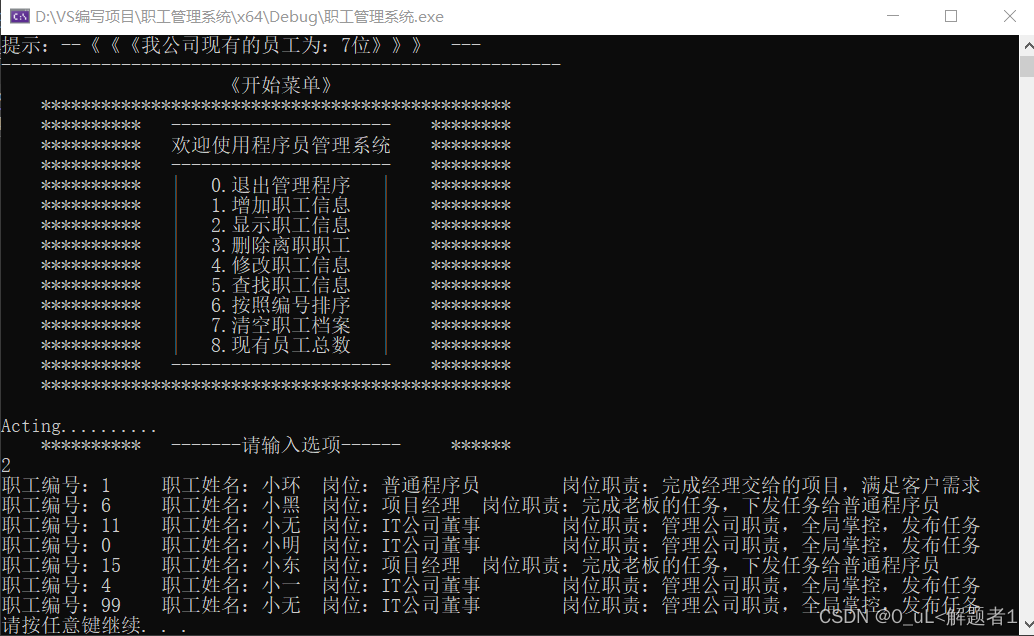
【4】按编号删除职工
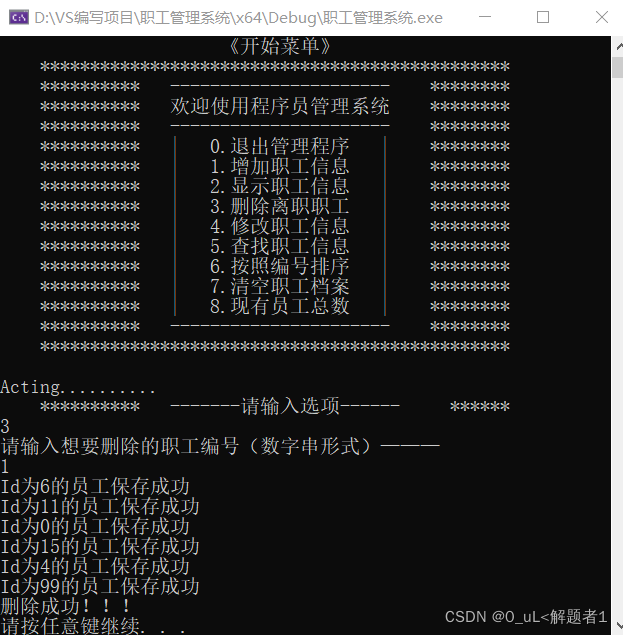 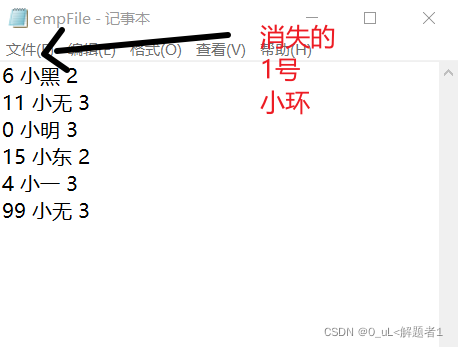
【5】修改职工信息
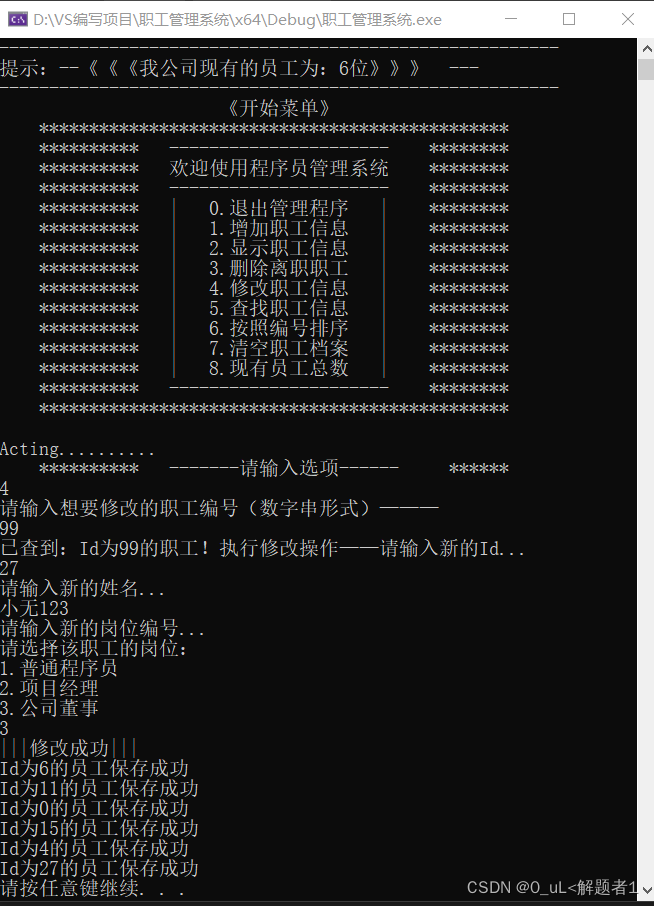 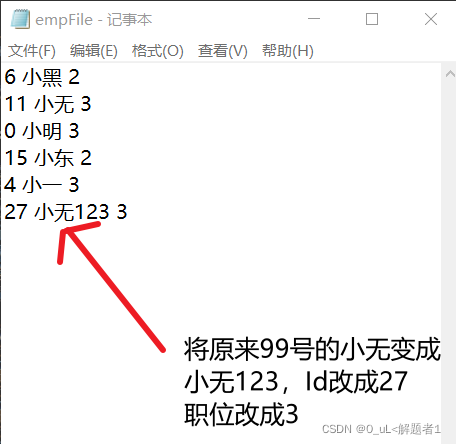
【5】查找职工
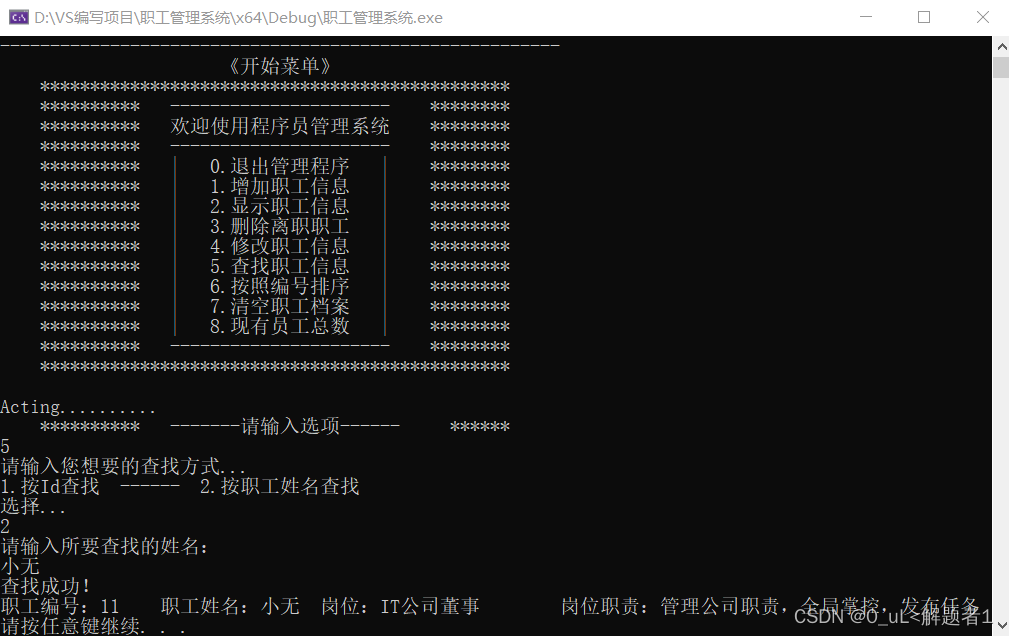
【6】对系统内的职工进行排序
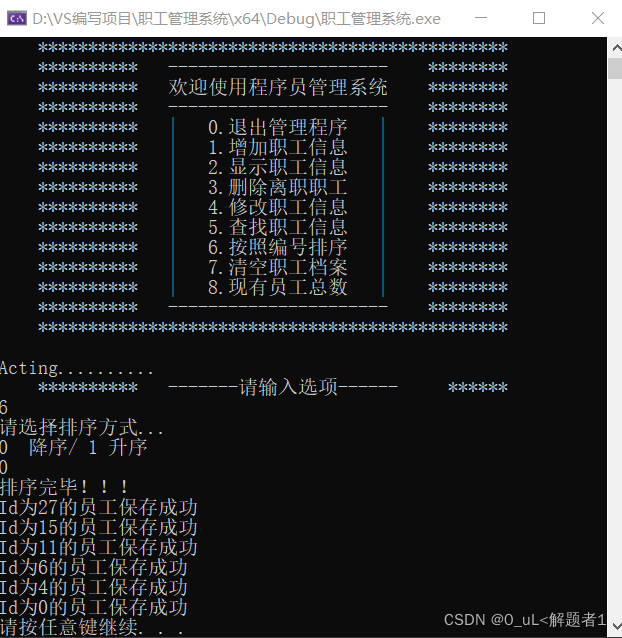
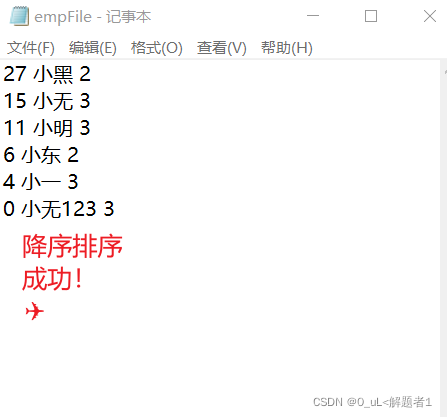
【7】清空档案——
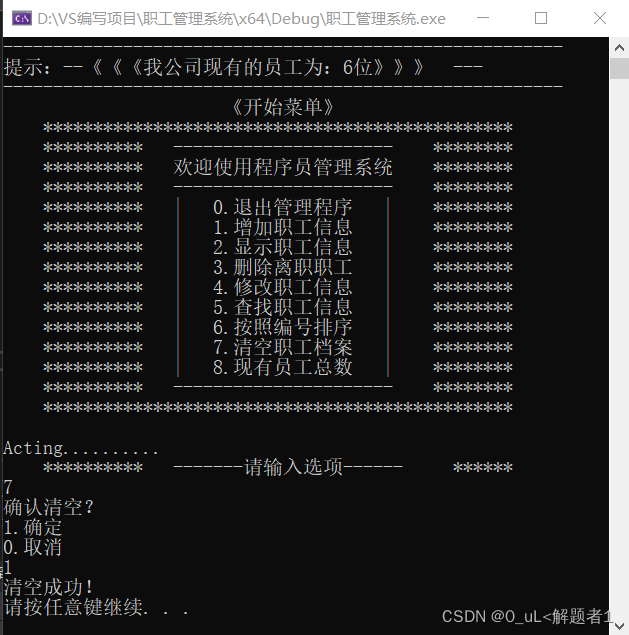 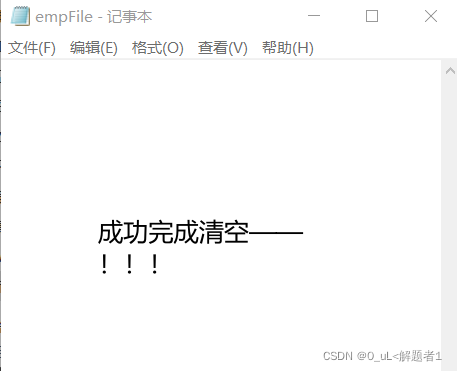
【8】现有员工总数
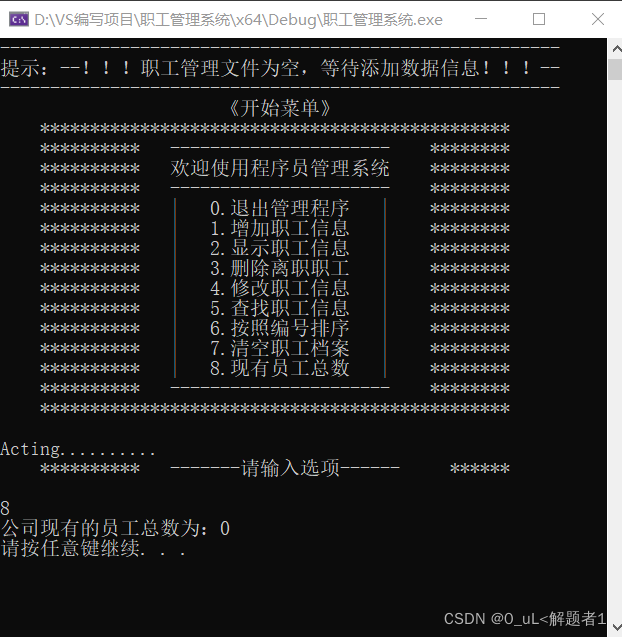
【9】退出职工操作系统
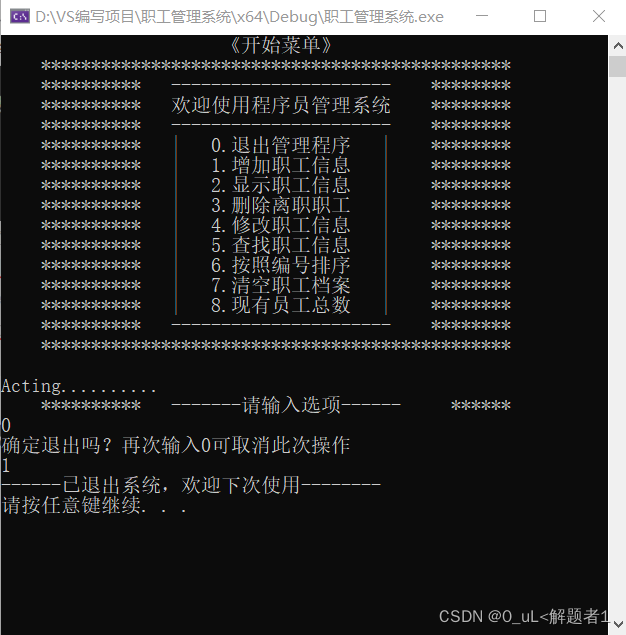
4.后记
完成练手项目后,我开始玩学校的大作业了ヾ(?°?°?)ノ゙ 最好将上述代码CV到IDE中,对CB玩家要稍微修一下O(∩_∩)O~
|