本博文源于课后习题陈维兴的《C++对象程序设计》,第三章课后习题的两道题目,代码实现
题目1:
建立类cylinder,类cylinder的构造函数被传递了两个double值,分别表示半径和高度。用类cylinder计算圆柱体的体积,并存储在一个double变量中,用类cylinder计算圆柱体的体积,并存储在一个double变量中。在类cylinder中包含一个成员函数vol(),用来显示每个cylinder对象的体积。
题目1思路分析
他说什么就是什么。建立构造函数,建立vol成员函数。
题目1完整实现
#include<iostream>
#define PI 3.14159
using namespace std;
class cylinder {
public:
cylinder(double r1,double h1){
r = r1;
h = h1;
}
void vol(){
v = PI*r*r*h;
cout << "vol:" << v << endl;
}
private:
double r;
double h;
double v;
};
int main()
{
cylinder cy(2,3);
cy.vol();
return 0;
}
题目1测试效果
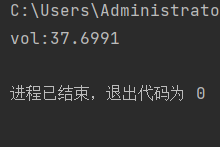
题目2
构建一个类Stock,含字符串stockcode及整形数据成员quantity、双精度类型数据成员price,构造函数含3个参数,当定义Stock类对象时,将对象的第1个字符串参数赋给数值stockcode,第二个和第三个参数赋给quantity和price。未设置第2和第3个参数,quantity的值为1000,price的值为8.98,成员函数print()没有形参,需使用this指针,显示对象数据成员的内容。假设类Stock第1个对象的3个参数分别为"6000001",3000和5.67;第2个对象第1各个数据成员的值是“60001”,第2个和第3个数据成员的取默认值,编写程序分别是显示这两个对象数据成员的值。
题目2分析
他说什么就是什么,直接将文字变成代码即可。
题目2完整实现
#include<iostream>
#include <cstring>
using namespace std;
class Stock{
private:
char *str;
int quantity;
double price;
public:
Stock(const char *str1,int q=1000,double p=8.98){
str = new char[strlen(str1) + 1];
strcpy(str,str1);
quantity = q;
price = p;
}
void print(){
cout << this->str << endl;
cout << this->quantity << endl;
cout << this->price << endl;
}
~Stock(){
delete []str;
}
};
int main()
{
Stock st("600001",3000,5.67);
Stock st2("600001");
Stock st3("600001");
st.print();
st2.print();
st3.print();
}
题目2代码演示
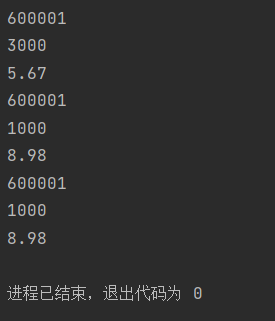
|