基于C语言编写的贪吃蛇 开发环境:VS2019
这是一个基于C语言链表开发的贪吃蛇游戏 其实贪吃蛇游戏要解决的主要问题就是 1、这个游戏的基本组成元素和架构 2、如何初始化贪吃蛇并正常行走 3、如何判断事件发生
代码中运用到了键盘虚拟键判断、终端窗口大小的改变、光标的定位以及输出字体的颜色
转载本文章,请标注原创! 若有不懂的地方,可以在评论区给我留言 代码如下:
#include <stdio.h>
#include <windows.h>
#include <stdlib.h>
#include <math.h>
#define R 'R'
#define L 'L'
#define U 'U'
#define D 'D'
typedef struct Node
{
int x;
int y;
struct Node* next;
}snake;
char FORWARD_DIRECTION;
snake* head, * p, * nexthead;
snake* food;
int grade = 0;
int addGrade = 5;
int sleepTime = 250;
void hideCursor();
void gotoxy(int x, int y);
void color(int c);
void createMap();
void initSnake();
void speedUp();
void speedDown();
void createFood();
void collideWall();
void collideSelf();
void moveSnake();
void gradeBoard();
void endGame();
void keyboardControl();
void welcometoGame();
void hideCursor()
{
CONSOLE_CURSOR_INFO cursor;
cursor.bVisible = FALSE;
cursor.dwSize = sizeof(cursor);
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorInfo(handle, &cursor);
}
void gotoxy(int x, int y)
{
COORD c = { x, y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), c);
}
void color(int c)
{
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), c);
}
void createMap()
{
system("mode con cols=150 lines=30");
system("cls");
color(3 | FOREGROUND_INTENSITY);
for (int i = 1; i <= 40; i++)
{
printf("■");
}
for (int i = 1; i <= 28; i++)
{
gotoxy(0, i);
printf("■");
gotoxy(78, i);
printf("■");
}
gotoxy(0, 29);
for (int i = 1; i <= 40; i++)
{
printf("■");
}
}
void initSnake()
{
snake* tail;
color(15);
head = (snake*)malloc(sizeof(snake));
head->x = 36;
head->y = 12;
p = head;
for (int i = 1; i <= 4; i++) {
tail = (snake*)malloc(sizeof(snake));
tail->x = head->x + 2 * i;
tail->y = head->y;
p->next = tail;
p = tail;
}
p->next = NULL;
p = head;
gotoxy(head->x, head->y);
while (head != NULL)
{
printf("■");
head = head->next;
}
gotoxy(149, 29);
}
void speedUp()
{
if (sleepTime > 50)
{
sleepTime = sleepTime - 50; addGrade++;
}
}
void speedDown()
{
if (sleepTime < 450)
{
sleepTime = sleepTime + 50; addGrade--;
}
}
void createFood()
{
byte i;
head = p;
food = (snake*)malloc(sizeof(snake));
food->next = NULL;
while (1)
{
i = 0;
food->x = rand() % 75 + 2;
food->y = rand() % 25 + 2;
while (head != NULL)
{
if (food->x == head->x && food->y == head->y)
i = 1;
head = head->next;
}
head = p;
if (food->x % 2 == 0 && food->y % 2 == 0 && i == 0)
break;
}
gotoxy(food->x, food->y);
color(12 | FOREGROUND_INTENSITY);
printf("■");
}
void collideWall() {
if (head->x == 0)
endGame();
if (head->x == 78)
endGame();
if (head->y == 0)
endGame();
if (head->y == 29)
endGame();
}
void collideSelf() {
head = head->next;
while (head != NULL)
{
if (head->x == p->x && head->y == p->y)
endGame();
head = head->next;
}
head = p;
}
void moveSnake()
{
color(15);
head = p;
if (FORWARD_DIRECTION == L)
{
nexthead = (snake*)malloc(sizeof(snake));
nexthead->x = head->x - 2;
nexthead->y = head->y;
nexthead->next = head;
head = nexthead;
p = head;
collideWall();
collideSelf();
gotoxy(head->x, head->y);
printf("■");
if (head->x == food->x && head->y == food->y)
{
free(food);
grade = grade + addGrade;
createFood();
}
else {
while (head->next->next != NULL)
{
head = head->next;
}
gotoxy(head->next->x, head->next->y);
printf(" ");
free(head->next);
head->next = NULL;
}
head = p;
}
if (FORWARD_DIRECTION == U)
{
nexthead = (snake*)malloc(sizeof(snake));
nexthead->x = head->x;
nexthead->y = head->y - 1;
nexthead->next = head;
head = nexthead;
p = head;
gotoxy(head->x, head->y);
printf("■");
collideWall();
collideSelf();
if (head->x == food->x && head->y == food->y)
{
free(food);
grade = grade + addGrade;
createFood();
}
else {
while (head->next->next != NULL)
{
head = head->next;
}
gotoxy(head->next->x, head->next->y);
printf(" ");
free(head->next);
head->next = NULL;
}
head = p;
}
if (FORWARD_DIRECTION == R)
{
nexthead = (snake*)malloc(sizeof(snake));
nexthead->x = head->x + 2;
nexthead->y = head->y;
nexthead->next = head;
head = nexthead;
p = head;
gotoxy(head->x, head->y);
printf("■");
collideWall();
collideSelf();
if (head->x == food->x && head->y == food->y)
{
free(food);
grade = grade + addGrade;
createFood();
}
else {
while (head->next->next != NULL)
{
head = head->next;
}
gotoxy(head->next->x, head->next->y);
printf(" ");
free(head->next);
head->next = NULL;
}
head = p;
}
if (FORWARD_DIRECTION == D)
{
nexthead = (snake*)malloc(sizeof(snake));
nexthead->x = head->x;
nexthead->y = head->y + 1;
nexthead->next = head;
head = nexthead;
p = head;
gotoxy(head->x, head->y);
printf("■");
collideWall();
collideSelf();
if (head->x == food->x && head->y == food->y)
{
free(food);
grade = grade + addGrade;
createFood();
}
else {
while (head->next->next != NULL)
{
head = head->next;
}
gotoxy(head->next->x, head->next->y);
printf(" ");
free(head->next);
head->next = NULL;
}
head = p;
}
}
void gradeBoard()
{
gotoxy(100, 12);
color(4);
printf("得分:%d ", grade);
gotoxy(100, 14);
printf("每个食物得分: %d分", addGrade);
gotoxy(100, 16);
printf("碰墙或碰到自己即Game Over.");
gotoxy(100, 18);
printf("↑ ↓ ← →控制蛇的移动");
gotoxy(100, 20);
printf("F1 加速,F2 减速");
gotoxy(100, 22);
printf("空格space:暂停游戏");
gotoxy(100, 24);
printf("ESC :退出游戏");
}
void endGame() {
system("cls");
gotoxy(66, 10);
color(12 | FOREGROUND_INTENSITY);
printf("游戏结束");
gotoxy(64, 12);
printf("您的得分为:%d", grade);
gotoxy(61, 14);
printf("再按一次ESC退出游戏");
while (1)
{
Sleep(300);
if (GetAsyncKeyState(VK_ESCAPE))
exit(0);
}
}
void keyboardControl()
{
FORWARD_DIRECTION = L;
while (1)
{
gradeBoard();
if (GetAsyncKeyState(VK_UP) && FORWARD_DIRECTION != D)
{
FORWARD_DIRECTION = U;
}
else if (GetAsyncKeyState(VK_DOWN) && FORWARD_DIRECTION != U)
{
FORWARD_DIRECTION = D;
}
else if (GetAsyncKeyState(VK_LEFT) && FORWARD_DIRECTION != R)
{
FORWARD_DIRECTION = L;
}
else if (GetAsyncKeyState(VK_RIGHT) && FORWARD_DIRECTION != L)
{
FORWARD_DIRECTION = R;
}
else if (GetAsyncKeyState(VK_F1))
{
speedUp();
}
else if (GetAsyncKeyState(VK_F2))
{
speedDown();
}
else if (GetAsyncKeyState(VK_SPACE))
{
while (1)
{
if (GetAsyncKeyState(VK_ESCAPE))
{
endGame();
}
gotoxy(100, 8);
color(4);
printf("Pause.");
Sleep(300);
if (GetAsyncKeyState(VK_SPACE))
{
gotoxy(100, 8);
printf("Start.");
break;
}
}
}
else if (GetAsyncKeyState(VK_ESCAPE))
{
endGame();
}
moveSnake();
Sleep(sleepTime);
}
}
void welcometoGame()
{
char n;
color(15);
gotoxy(42, 10);
printf("Wecome to snake.");
color(12);
gotoxy(20, 27);
printf("按1开始游戏");
gotoxy(65, 27);
printf("按任意除1按键退出游戏");
color(3 | FOREGROUND_INTENSITY);
gotoxy(40, 14);
printf("每个食物得分: %d分", addGrade);
gotoxy(38, 16);
printf("碰墙或咬到自己即Game Over.");
gotoxy(38, 18);
printf("↑ ↓ ← →控制蛇的移动");
gotoxy(41, 20);
printf("F1 加速,F2 减速");
gotoxy(40, 22);
printf("空格space:暂停游戏");
gotoxy(43, 24);
printf("ESC :退出游戏");
gotoxy(43, 28);
printf("please select:");
n = getch();
switch (n)
{
case '1':
{
createMap();
initSnake();
createFood();
keyboardControl();
break;
}
default:exit(0);
}
}
int main()
{
system("mode con cols=100 lines=30");
hideCursor();
welcometoGame();
}
运行截图: 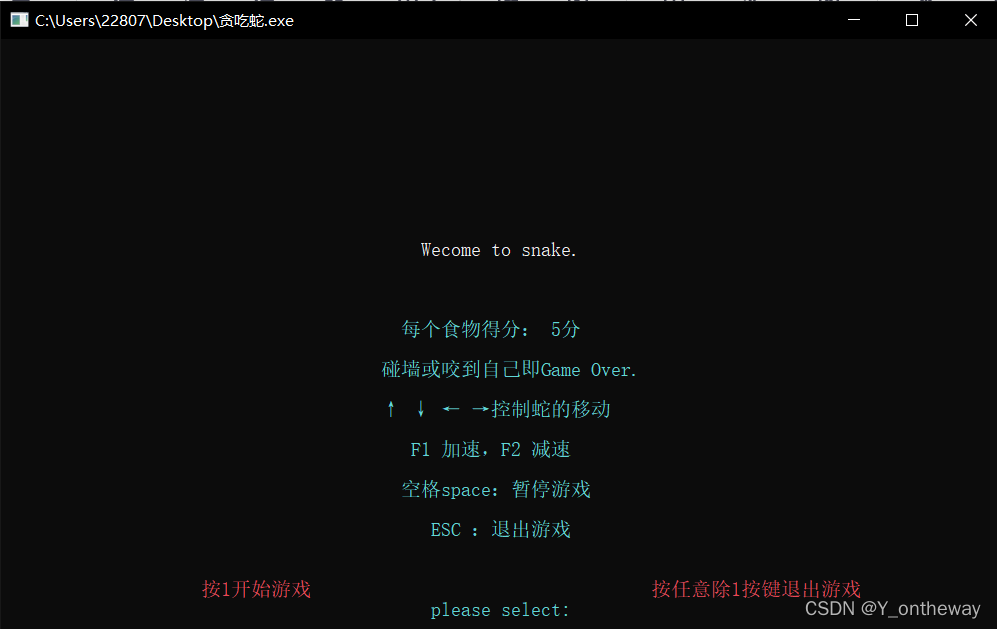 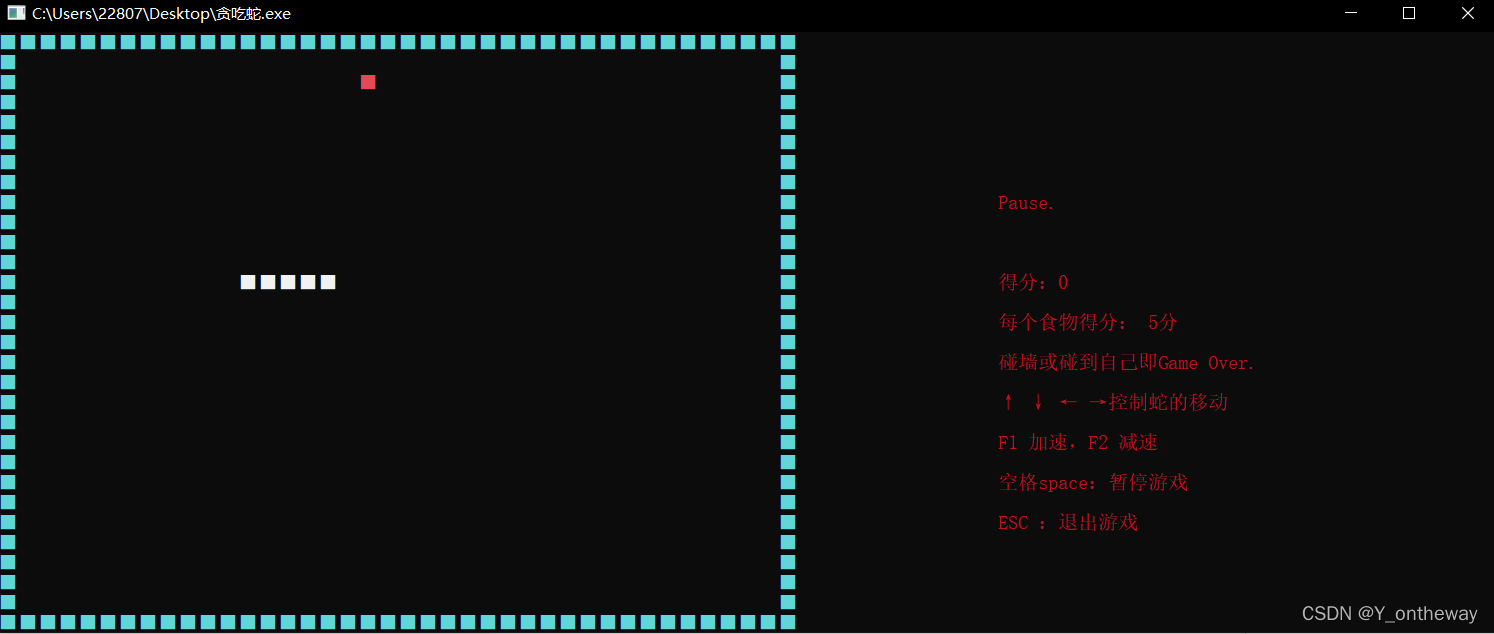 若有疑问或者更好的创意,可以在评论区留言! 若有转载引用本作者的代码,还请通知本作者并标注原创!
|