知识积累与整理
字符指针数组理解!
#include<stdio.h>
int main()
{
char *c[4] = {"string0", "string1", "string2", "string3"};
char **p;
p = c;
int i;
for(i = 0; i < 4; i++)
{
printf("%s\n", *(p + i));
int j;
for(j = 0; j <7; j++)
{
printf("%c ", *(*(p + i) + j));
}
printf("\n");
}
return 0;
}
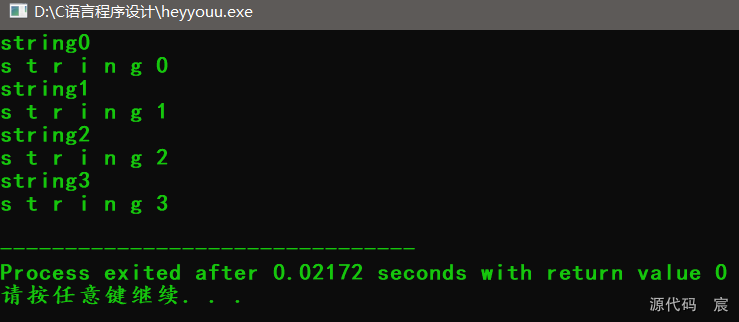  B *s是指向字符串的指针,而**s是指向字符串指针的指针  C不解释太简单了 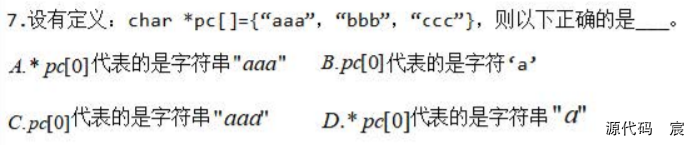 因为pc数组的元素本身都是一级指针又不是二级指针,不存在可以得到’a’这些元素的,故BD排除 对于AC我们可以这么理解,就是pc[0] = “aaa”,pc[1] = “bbb”,pc[2] = “ccc”,就相当于char *p1,*p2,*p3;p1 = “aaa”, p2 = “bbb”, p3 = "ccc"所以选C
全局变量与局部变量
全局变量也称为外部变量,也就是说是在函数外部定义的变量。它不属于哪一个函数,而是属于一个源程序文件。一般情况下其作用域是从定义处开始一直到整个源程序文件结束。
静态变量static
局部静态变量的初值为0(对整型变量)或空字符(对字符型变量)。
函数的隐含储存类型是extern,函数的形参或变量的储存类型为auto
C语言规定,只要在定义函数时不进行存储类型的显式说明,函数的存储类型就隐含为外部类型,即extern型,外部类型允许其他文件中的函数调用。
实际上函数的声明和定义都不需要添加extern关键字,在实际使用的时候也最好不要添加关键字。
如果一个函数是不会被其它文件调用的,那么这个函数应该被声明成static的。
自动类型转换
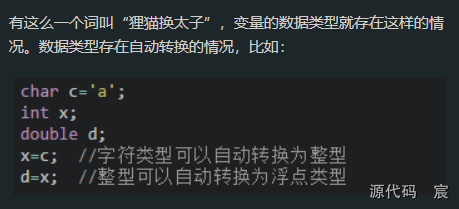 自动转换发生在不同数据类型运算时,在编译的时候自动完成。自动转换遵循的规则就好比小盒子可以放进大盒子里面一样,下图表示了类型自动转换的规则。 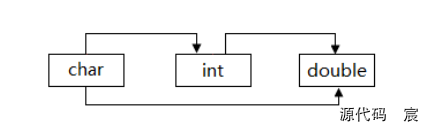 注:字节小的可以向字节大的自动转换,但字节大的不能向字节小的自动转换
赋值运算符和赋值表达式
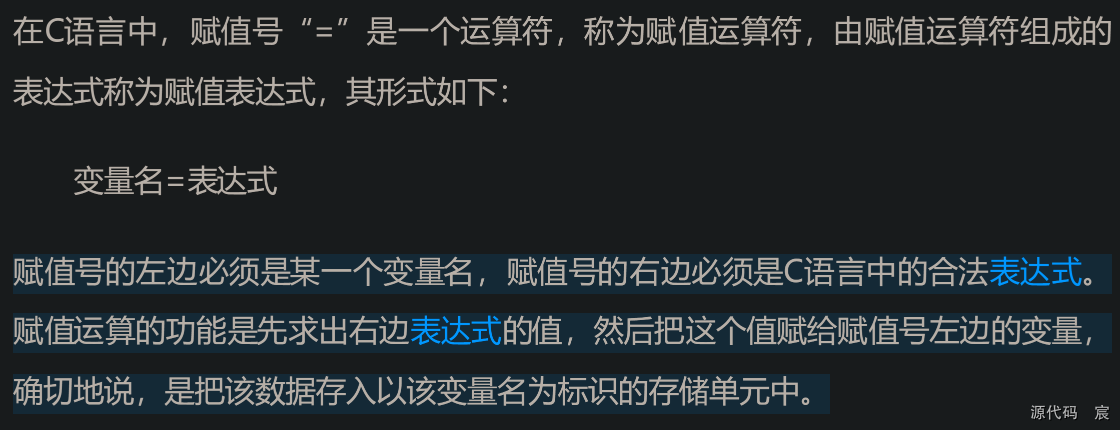 使用赋值表达式要注意以下几个方面:
- ①赋值运算符不是数学中的“等于号”,而是进行“赋予”操作。如:a=5+3 应读作“先进行5+3运算,然后把运算结果赋给变量a”。
- ②赋值运算符的左边只能是变量,不能是常量或表达式,而赋值运算符的右边可以是常量或表达式。如:a+3=5,a+b=c等都是不合法的赋值表达式。
- ③赋值表达式x=y的作用是:将变量y所代表的存储单元中的内容赋给变量x所代表的存储单元,x中原有的数据被替换掉,而变量y所代表的存储单元中的内容不变。简单地说,就是把变量y的值赋给变量x,变量y的值不变,而变量x原有的值被y的值所替换。
- ④可以进行连续的赋值操作,但不可以进行连续的赋初值操作。a=b=5是合法的赋值表达式,由于赋值运算符的结合方向是自右向左,因此这个表达式等价于a=(b=5),即先将5赋给变量b,再把b的值赋给变量a。
- ⑤赋值表达式n=n+1的含义是:取出变量n中的值加1再放回到变量n中,即使变量n中的值增1。
- ⑥当赋值运算符两边的类型不一致时,要进行类型自动转换。
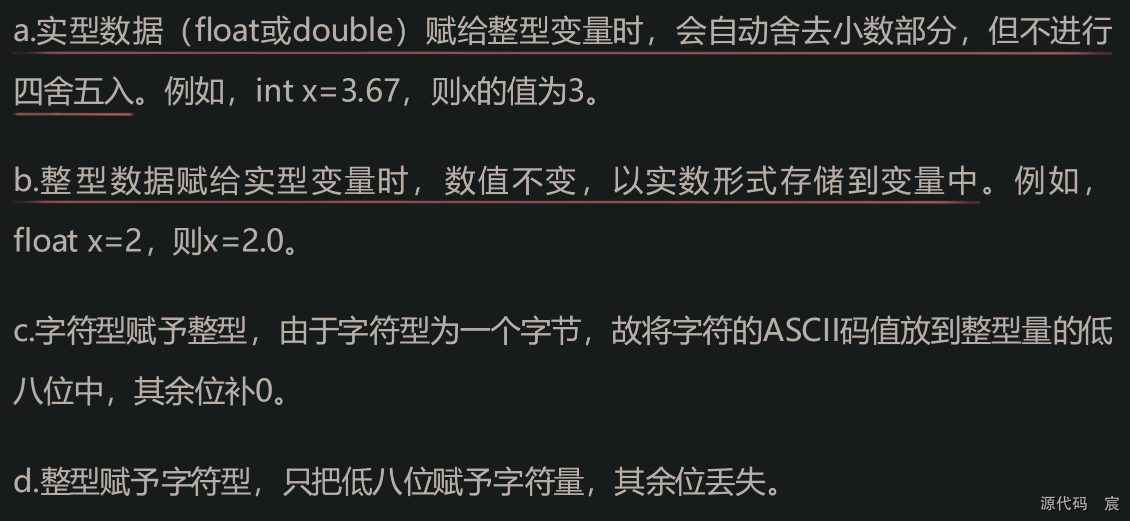
逗号表达式
“,”是C语言提供的一种特殊的运算符,称为逗号运算符。逗号运算符的结合性为从左到右。在所有运算符中,逗号运算符的优先级最低。 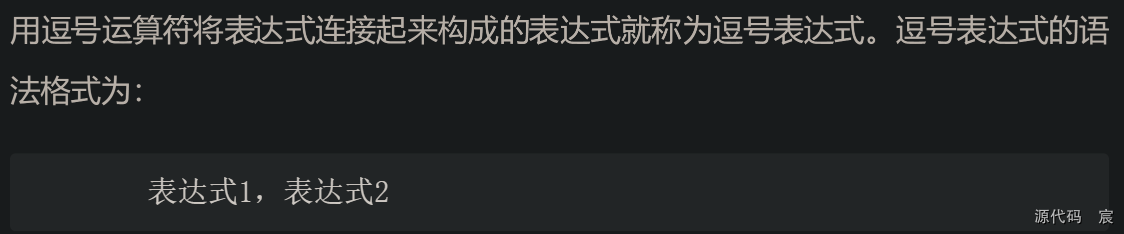 对于逗号表达式做以下几点说明:
- (1)逗号表达式的求解过程是:先求解表达式1,再求解表达式2。整个逗号表达式的值是表达式2的值。
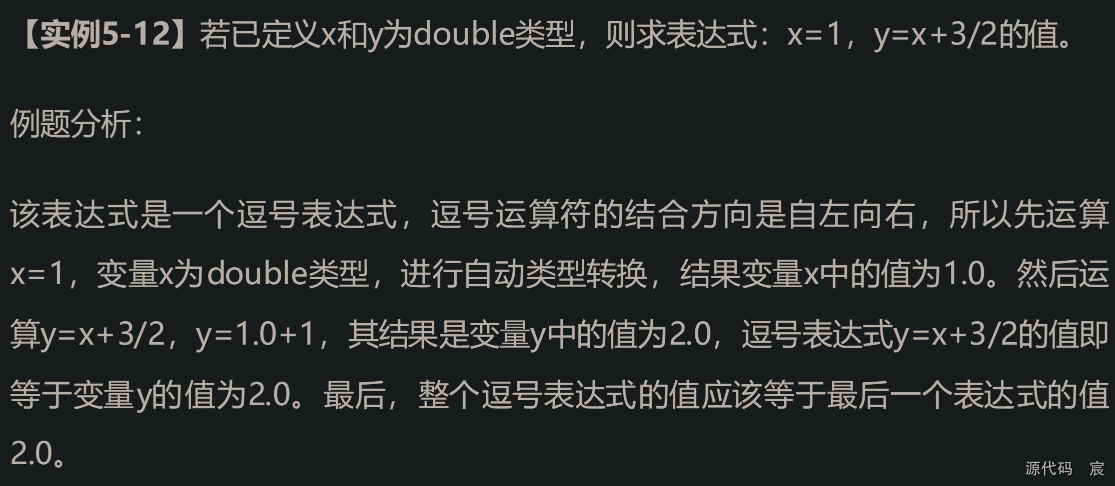 - (2)逗号表达式在求解的过程中要注意各个运算符之间的优先级,逗号运算符的优先级最低。例如:
#include<stdio.h>
int main()
{
int a;
printf("%d\n", (a = 3 * 5, a * 4));
return 0;
}
赋值运算符的优先级别高于逗号运算符,因此应先求解a=3*5(也就是把“a=3*5”作为一个表达式)。经计算和赋值后得到a的值为15,然后求解a*4,得60,因此整个逗号表达式的值为60。 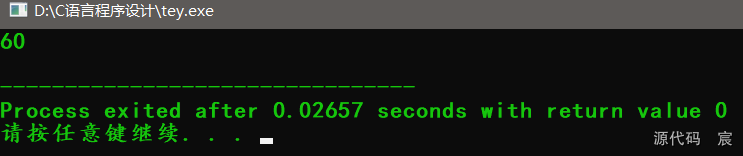
- (3)逗号表达式可以进行嵌套,即表达式1和表达式2本身也可以是逗号表达式。例如:
#include<stdio.h>
int main()
{
int a;
printf("%d\n", ((a = 12, a * 4), a + 5));
return 0;
}
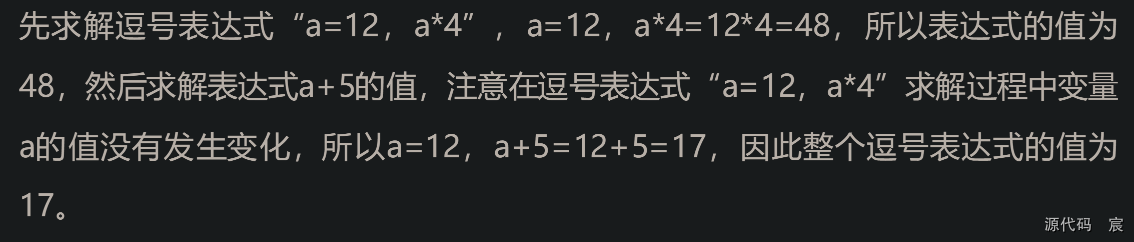
运算符优先级
单目>算术>位移>关系>逻辑>条件>赋值
文件的打开
如果fopen()打开文件失败则返回空指针NULL
#include<stdio.h>
int main()
{
FILE *fp = fopen("test.txt", "r");
if(NULL == fp)
{
printf("can not open the file!\n");
}
else
{
printf("open success!\n");
}
return 0;
}
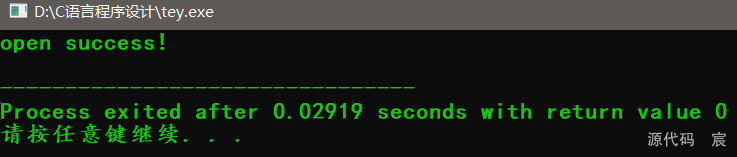
文件定位
移动文件内部位置指针的函数主要有两个,即 rewind 函数和fseek函数。
(1)rewind函数
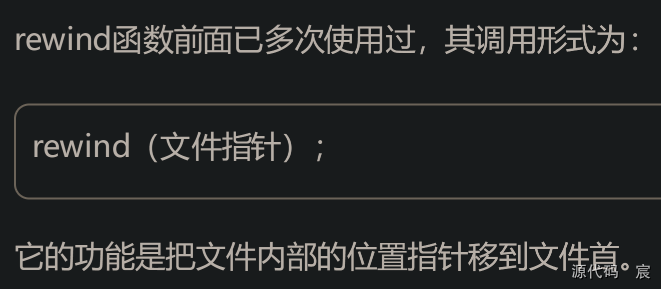
(2)fseek函数
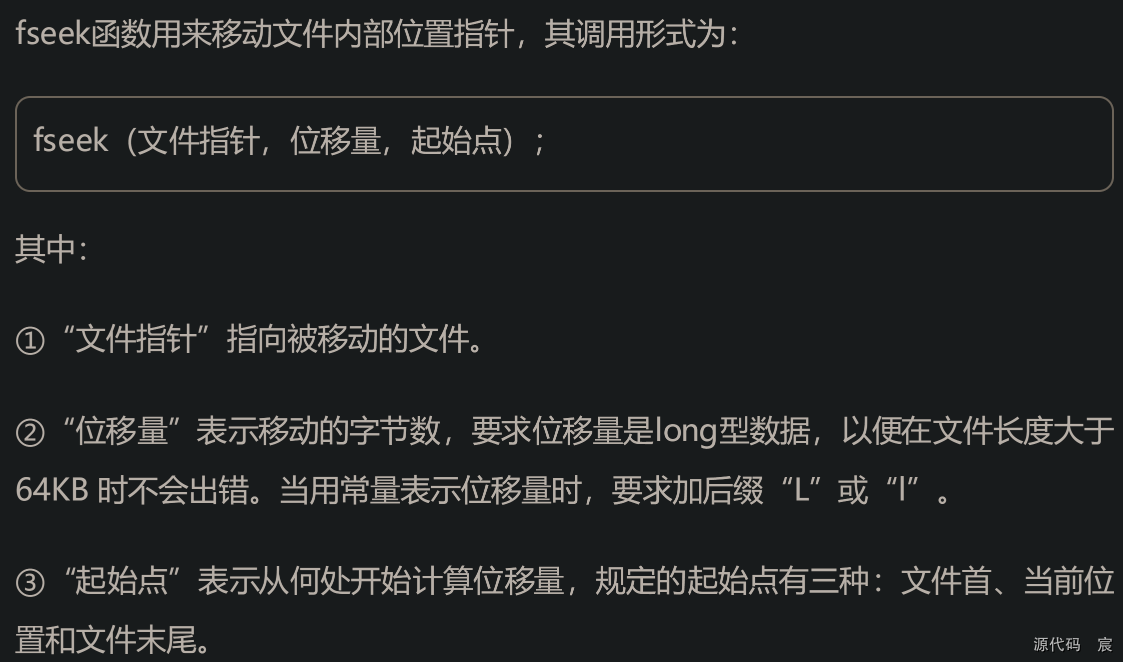 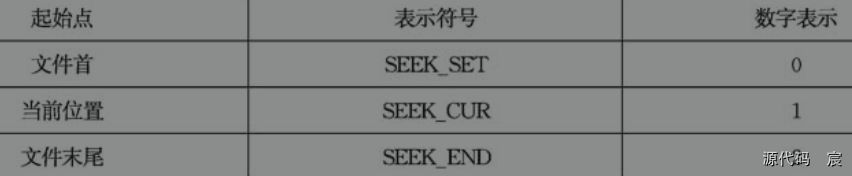 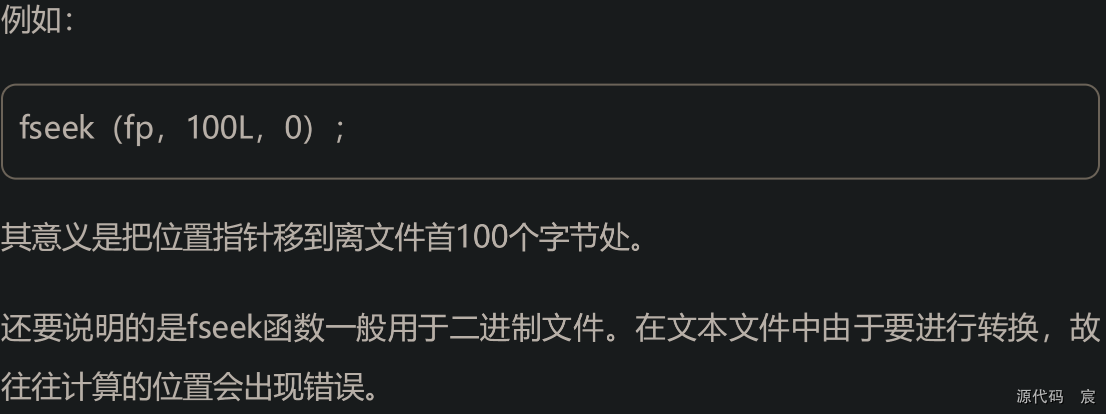 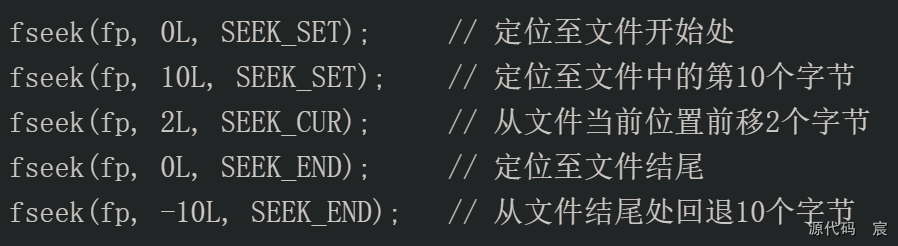 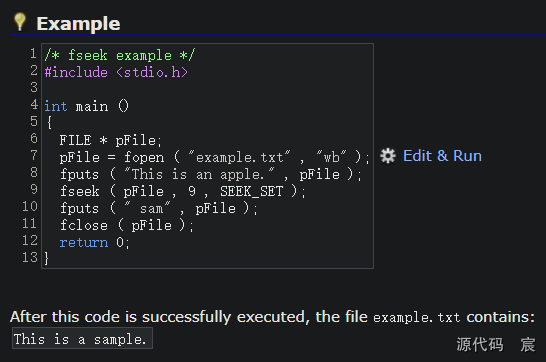
文件相关题目
fopen函数
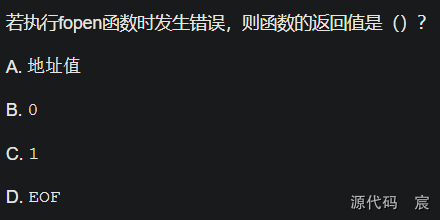 【解释】执行fopen函数时,如果文件打开成功,则返回该文件结构体的指针,如果打开失败(例如,读打开时文件不存在,写打开时文件不能创建),则返回NULL(即0)。
故选择答案是B。
字符串输出函数puts
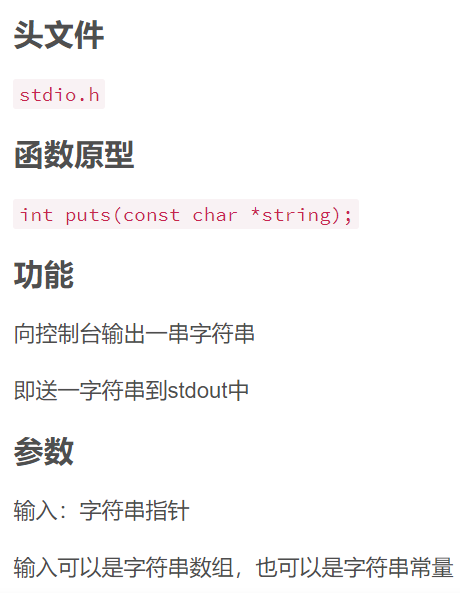 
题目
1

灵活法(全排列)
#include<bits/stdc++.h>
using namespace std;
int book[4] = {0};
int a[4] = {1, 2, 3, 4};
int b[3] = {0};
int n = 3;
int cnt = 0;
void dfs(int step)
{
if(step == n)
{
for(int i = 0; i < 3; i++)
{
cout << b[i] << " ";
}
cout << endl;
cnt++;
return;
}
for(int i = 0; i < 4; i++)
{
if(book[i] == 0)
{
b[step] = a[i];
book[i] = 1;
dfs(step + 1);
book[i] = 0;
}
}
}
int main()
{
dfs(0);
cout << cnt << endl;
return 0;
}
中规中矩法(暴力法)
#include<bits/stdc++.h>
using namespace std;
int main()
{
int i, j, k;
int cnt = 0;
for(i = 1; i < 5; i++)
{
for(j = 1; j < 5; j++)
{
if(j != i)
{
for(k = 1; k < 5; k++)
{
if(k != i && k != j)
{
cout << i << j << k << endl;
cnt++;
}
}
}
}
}
cout << cnt << endl;
return 0;
}
运行结果: 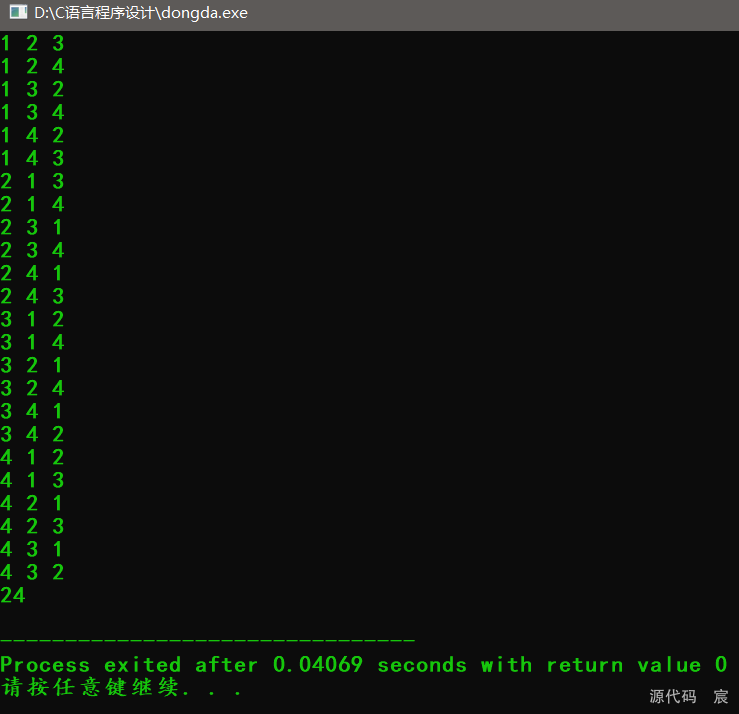
2
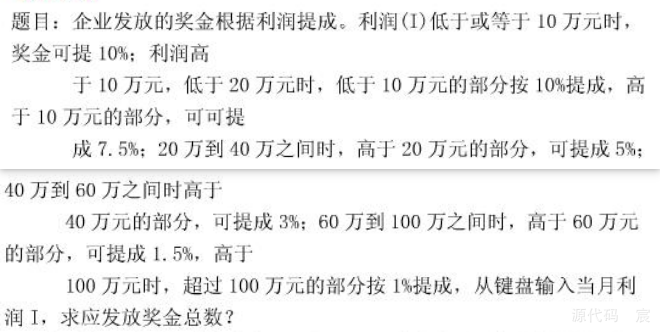
2015年真题大题部分
C程序设计
1、

#include<stdio.h>
#define MAX_SIZE 6
int main()
{
int array[MAX_SIZE] = {10, 0, -1, 2, 3, 4};
int max = array[0];
int min = array[0];
for(int i = 0; i < MAX_SIZE; i++)
{
if(max < array[i])
{
max = array[i];
}
if(min > array[i])
{
min = array[i];
}
}
printf("max:%d min:%d\n", max, min);
return 0;
}
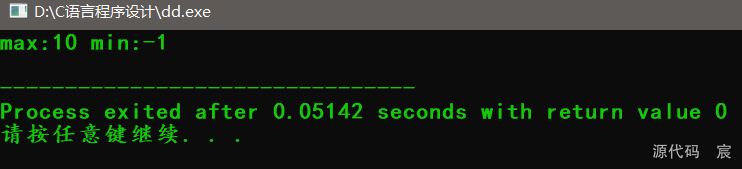
2、

#include<stdio.h>
#define MAX_SIZE 5
int main()
{
double array[MAX_SIZE] = {1.3, 3.1, 4.1, 5.2, 7};
double average = 0;
int i = 0;
double sum = 0;
for(int i = 0; i < MAX_SIZE; i++)
{
sum += array[i];
}
average = sum / MAX_SIZE;
printf("average:%lf\n", average);
return 0;
}
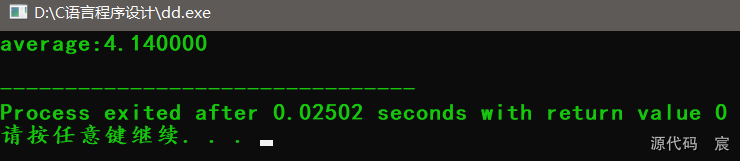
3、

#include<stdio.h>
typedef struct EmployeeInfo{
int id;
char name[10];
float wage;
char position[20];
}EInfo;
typedef struct StatisticResult{
int indexLowestWage;
int indexHighestWage;
float avwage;
}SResult;
int getWageStatistic(EInfo *pinfo, int length, SResult *pres)
{
if(pinfo == NULL || pres == NULL)
{
return 0;
}
int indexHighestWage = 0;
int indexLowestWage = 0;
float sumWage = 0;
for(int i = 0; i < length; i++)
{
sumWage += pinfo[i].wage;
if(pinfo[indexHighestWage].wage < pinfo[i].wage)
{
indexHighestWage = i;
}
if(pinfo[indexLowestWage].wage > pinfo[i].wage)
{
indexLowestWage = i;
}
}
pres->avwage = sumWage / length;
pres->indexHighestWage = indexHighestWage;
pres->indexLowestWage = indexLowestWage;
return 1;
}
int printS(EInfo *pinfo, int length, float avwage)
{
if(pinfo == NULL)
{
return 0;
}
for(int i = 0; i < length; i++)
{
if(avwage < pinfo[i].wage)
{
printf("id:%d name:%s\n", pinfo[i].id, pinfo[i].name);
}
}
return 1;
}
int main()
{
EInfo info[6] = {
{1, "liming", 63000, "助理"},
{2, "lihua", 32000, "助理"},
{3, "liyi", 12000, "讲师"},
{4, "lile", 24000, "秘书"},
{5, "lihang", 35000, "顾问"},
{6, "lirui", 96000, "大佬"},
};
SResult sres = {0};
getWageStatistic(info, 6, &sres);
printf("LowestID:%d\n", info[sres.indexLowestWage].id);
printf("HighestID:%d\n", info[sres.indexHighestWage].id);
printS(info, 6, sres.avwage);
return 0;
}
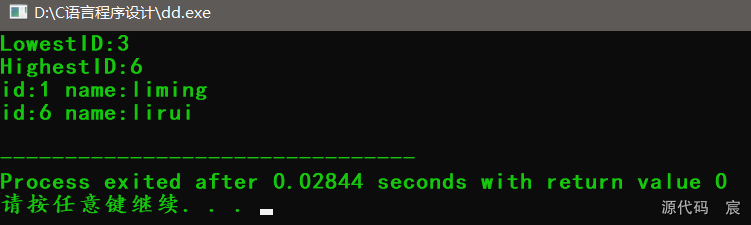
数据结构算法题
1、

#include<stdio.h>
#include<stdlib.h>
#define Maxsize 100
typedef int ElemType;
typedef struct SqList{
ElemType *plist;
int length;
int listsize;
}SqList;
int CreateSqList(SqList *p)
{
ElemType *elem = (ElemType *)malloc(Maxsize * sizeof(ElemType));
if(!elem)
{
return 0;
}
p->plist = elem;
p->length = 0;
return 1;
}
int oneSort(SqList *p, int left, int right)
{
int tmp = p->plist[left];
while(left < right)
{
while(left < right && p->plist[right] >= tmp)
{
right--;
}
p->plist[left] = p->plist[right];
while(left < right && p->plist[left] < tmp)
{
left++;
}
p->plist[right] = p->plist[left];
}
p->plist[left] = tmp;
return left;
}
int main()
{
SqList q;
CreateSqList(&q);
int n = 8;
int array[8] = {24, 6, 3, 9, 8, 1, 0, 33};
int i;
for(i = 0; i < 8; i++)
{
q.plist[i] = array[i];
}
q.length = n;
oneSort(&q, 0, 7);
for(i = 0; i < 8; i++)
{
printf("%5d", q.plist[i]);
}
printf("\n");
return 0;
}
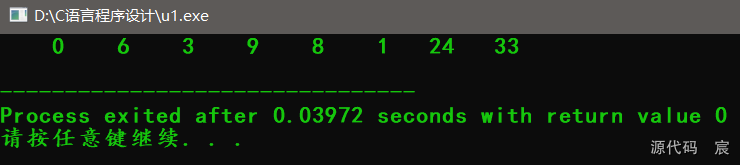 这么写也可以,就是没有写创建顺序表函数,而是直接在主函数将plist指向数组首地址
#include<stdio.h>
#include<stdlib.h>
#define Maxsize 100
typedef int ElemType;
typedef struct SqList{
ElemType *plist;
int length;
int listsize;
}SqList;
int oneSort(SqList *p, int left, int right)
{
int tmp = p->plist[left];
while(left < right)
{
while(left < right && p->plist[right] >= tmp)
{
right--;
}
p->plist[left] = p->plist[right];
while(left < right && p->plist[left] < tmp)
{
left++;
}
p->plist[right] = p->plist[left];
}
p->plist[left] = tmp;
return left;
}
int main()
{
SqList q;
int n = 8;
int array[8] = {24, 6, 3, 9, 8, 1, 0, 33};
int i;
q.plist = array;
q.length = n;
oneSort(&q, 0, 7);
for(i = 0; i < 8; i++)
{
printf("%5d", q.plist[i]);
}
printf("\n");
return 0;
}
2、

#include<stdio.h>
#include<stdlib.h>
typedef char ElemType;
typedef struct BiTNode{
char data;
struct BiTNode *lchild, *rchild;
struct BiTNode *parent;
}BiTNode, *Bitree;
void CreateBitree(Bitree *bt)
{
char ch;
scanf("%c", &ch);
if(ch == '#')
{
*bt = NULL;
}
else
{
*bt = (Bitree)malloc(sizeof(BiTNode));
(*bt)->data = ch;
CreateBitree(&(*bt)->lchild);
CreateBitree(&(*bt)->rchild);
}
}
void preorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
printf("%c ", bt->data);
preorder(bt->lchild);
preorder(bt->rchild);
}
void inorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
inorder(bt->lchild);
printf("%c ", bt->data);
inorder(bt->rchild);
}
void postorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
postorder(bt->lchild);
postorder(bt->rchild);
printf("%c ", bt->data);
}
void preTravelTree(Bitree t, ElemType x, BiTNode *parent)
{
if(t != NULL)
{
t->parent = parent;
if(t->data == x)
{
BiTNode *ancesterNode = t->parent;
while(ancesterNode != NULL)
{
printf("%c ->", ancesterNode->data);
ancesterNode = ancesterNode->parent;
}
}
preTravelTree(t->lchild, x, t);
preTravelTree(t->rchild, x, t);
}
}
void getNodeAncesters(Bitree t, ElemType x)
{
preTravelTree(t, x, NULL);
}
int main()
{
Bitree T;
printf("请输入二叉树结点序列:\n");
CreateBitree(&T);
printf("\n先序遍历:");
preorder(T);
printf("\n中序遍历:");
inorder(T);
printf("\n后序遍历:");
postorder(T);
printf("\n");
char character = 'D';
getNodeAncesters(T, character);
return 0;
}
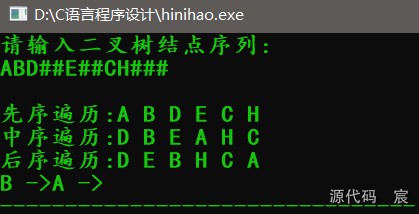
2016真题
选择题
涉及知识点:字符串和字符数组关于’\0’的处理
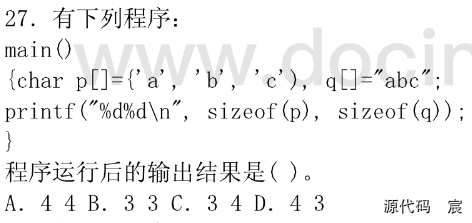  其中字符数组如char c[5] = {‘C’, ‘h’, ‘i’, ‘n’, ‘a’};并不要求它最后一个字符为’\0’,甚至可以不包含’\0’
大题C语言程序设计
1、

#include<stdio.h>
#define MAX_SIZE 7
int main()
{
int age[MAX_SIZE] = {21, 18, 19, 20, 31, 42, 40};
int lowestAge = age[0];
int highestAge = age[0];
double average = 0.0;
int sum = 0;
int i;
for(i = 0; i < MAX_SIZE; i++)
{
if(age[i] < lowestAge)
{
lowestAge = age[i];
}
if(age[i] > highestAge)
{
highestAge = age[i];
}
sum += age[i];
}
average = sum / MAX_SIZE;
printf("%d %d %lf\n", lowestAge, highestAge, average);
return 0;
}
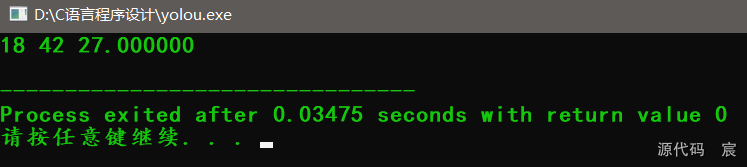
2、

#include<stdio.h>
#define MAX_SIZE 7
int poww(int power)
{
int product = 1;
int i = 0;
int Xi = 0;
printf("Enter Xi:");
scanf("%d", &Xi);
for(i = 1; i <= power; i++)
{
product *= (Xi - 8);
}
return product;
}
int main()
{
int n = 0;
long int sum = 0;
int i = 0;
printf("Enter n:");
scanf("%d", &n);
printf("\n");
for(i = 0; i < n; i++)
{
sum += poww(4);
}
printf("%d", sum);
return 0;
}
3、

#include<stdio.h>
#define MAX_SIZE 7
typedef struct Courses{
int grades_eng;
int grades_math;
int grades_software;
int grades_internet;
}Courses;
typedef struct Person{
char name[10];
Courses course;
double sum;
double aver_grades;
}Person;
int main()
{
Person student[3] = {"Jacky", 97, 98, 99, 96, 0, 0,"Lily", 90, 90, 90, 90, 0, 0,"Alan", 98, 95, 93, 92, 0, 0};
int sum_eng = 0;
int sum_math = 0;
int sum_software = 0;
int sum_internet = 0;
int student_sum = 0;
double aver_student = 0;
double aver_eng = 0;
double aver_math = 0;
double aver_software = 0;
double aver_internet = 0;
int i;
for(i = 0; i < 3; i++)
{
student_sum = 0;
sum_eng += student[i].course.grades_eng;
sum_math += student[i].course.grades_math;
sum_software += student[i].course.grades_software;
sum_internet += student[i].course.grades_internet;
student_sum = student[i].course.grades_eng + student[i].course.grades_math + student[i].course.grades_software + student[i].course.grades_internet;
student[i].aver_grades = student_sum / 4;
student[i].sum = student_sum;
printf("student %s's scores:%lf\n", student[i].name, student[i].sum);
}
aver_eng = sum_eng / 3;
aver_math = sum_math / 3;
aver_software = sum_software / 3;
aver_internet = sum_internet / 3;
printf("average_eng:%lf average_math:%lf average_software:%lf average_internet:%lf\n", aver_eng, aver_math, aver_software, aver_internet);
}

大题数据结构
1、(我的提示:构造拉链法哈希表用二级指针)

#include<stdio.h>
#include<stdlib.h>
typedef struct Node{
int data;
struct Node *next;
}Node;
typedef struct HashTable{
Node **point;
int table_length;
}HashTable;
int createHashTable(HashTable *table, int size)
{
if(!size)
{
return 0;
}
table->point = (Node **)malloc(sizeof(Node *) * size);
table->table_length = size;
while(size)
{
table->point[--size] = NULL;
}
return 1;
}
int insertNode(HashTable *table, int prime, int number)
{
if(number < 0)
{
return 0;
}
int index = number % prime;
Node *p = (Node *)malloc(sizeof(Node));
p->data = number;
p->next = table->point[index];
table->point[index] = p;
return 1;
}
int lookupHashTable(HashTable table, int number, int prime)
{
if(number < 0)
{
return -1;
}
int index = number % prime;
Node *p = table.point[index];
while(p != NULL && p->data != number)
{
p = p->next;
}
if(p != NULL)
{
return index;
}
return -1;
}
int main()
{
HashTable t;
if(!createHashTable(&t, 5))
{
printf("Fail to create!\n");
return 0;
}
printf("insert 5\n");
insertNode(&t, 5, 4);
printf("insert 6\n");
insertNode(&t, 5, 7);
if(lookupHashTable(t, 7, 5))
{
printf("it is at %d\n", lookupHashTable(t, 7, 5));
}
else
{
printf("it is inexistent\n");
}
return 0;
}
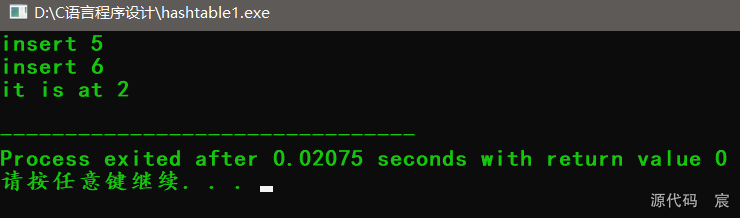
2、

typedef struct BSTNode{
int data;
struct BSTNode *lchild;
struct BSTNode *rchild;
}BSTNode, *BSTree;
BSTNode *tmp;
void travel(BSTree T, ElemType value)
{
if(T != NULL)
{
travel(T->rchild, value);
if(T->data > value)
{
tmp = T;
travel(T->lchild, value);
}
}
}
int getValue(BSTree T, ElemType value)
{
travel(T, value);
return tmp->data;
}
2017真题
C语言大题
1、

#include<stdio.h>
int main()
{
int sales[10] = {82, 75, 58, 79, 64, 48, 96, 55, 81, 69};
int high , low, aver = 0;
int sum = sales[0];
high = sales[0];
low = sales[0];
int h, l;
h = 0, l = 0;
int i;
for(i = 1; i < 10; i++)
{
if(high < sales[i])
{
high = sales[i];
h = i;
}
if(low > sales[i])
{
low = sales[i];
l = i;
}
sum += sales[i];
}
aver = sum / 10;
printf("销售数量最高为第%d天\t销售数量最低为第%d天\n平均销售数量为%d\t最高销售数量为%d\t最低销售数量为%d\n", h + 1, l + 1, aver, high, low);
return 0;
}
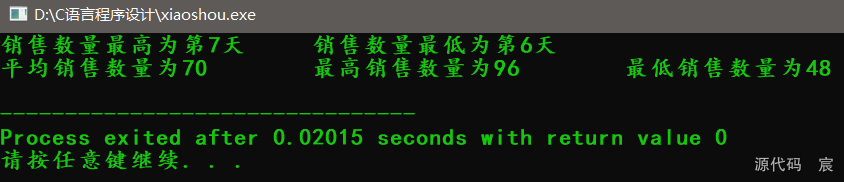
2、

#include<stdio.h>
int main()
{
int i = 1;
int n = 30;
double sum = 0;
for(i = 1; i <= n; i++)
{
int f = 1;
int j = 0;
for(j = i; j >= 1; j--)
{
f *= j;
}
printf("%lf\n", 1.0 / (f * f));
sum += 1.0 / (f * f);
}
printf("%lf\n", sum);
}
3、
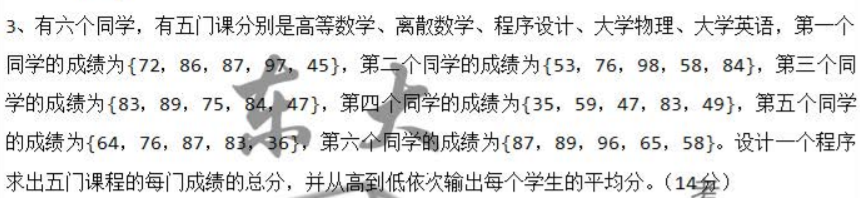
#include<stdio.h>
typedef struct Score{
int Hmath;
int Dmath;
int Program;
int Physics;
int English;
}Score;
int main()
{
Score student[6] = {72, 86, 87, 97, 45, 53, 76, 98, 58, 84, 83, 89, 75, 84, 47, 35, 59, 47, 83, 49, 64, 76, 87, 83, 36, 87, 89, 96, 65, 58};
int hmath = 0, dmath = 0, program = 0, physics = 0, english = 0;
double aver[6];
int i;
for(i = 0; i < 6; i++)
{
double sum = 0;
hmath += student[i].Hmath;
dmath += student[i].Dmath;
program += student[i].Program;
physics += student[i].Physics;
english += student[i].English;
sum = student[i].Hmath + student[i].Dmath + student[i].Program
+ student[i].Physics + student[i].English;
aver[i] = sum / 6.0;
}
int j;
int k;
for(i = 0; i < 5; i++)
{
k = i;
for(j = i + 1; j < 6; j++)
{
if(aver[k] > aver[j])
{
k = j;
}
}
if(k != i)
{
int tmp = i;
i = k;
k = tmp;
}
}
printf("高等数学总分:%d\t离散数学总分:%d\t程序设计总分:%d\t大学物理总分:%d\t大学英语总分:%d\n", hmath, dmath, program, physics, english);
printf("从高到低平均分:\n");
for(i = 0; i < 6; i++)
{
printf("%lf\t", aver[i]);
}
return 0;
}

数据结构大题
1、
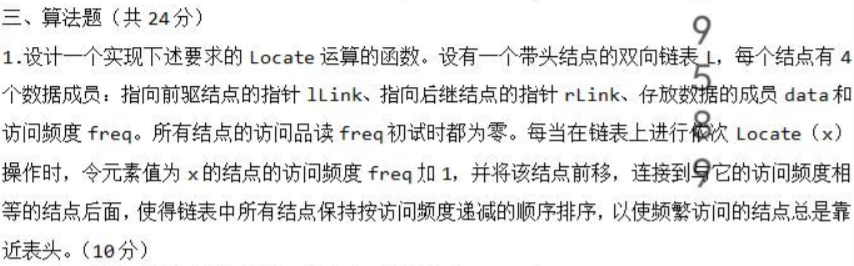
#include<stdio.h>
typedef struct DuLinkNode{
ElemType data;
int freq;
struct DuLinkNode *lLink;
struct DuLinkNode *rLink;
}DLNode, *DLink;
void Locate(DLink L, ElemType x)
{
DLNode *p = L;
while(p->rLink != NULL)
{
if(p->rLink->data == x)
{
break;
}
p = p->rLink;
}
if(p->rLink == NULL)
{
DLNode *r = (DLNode *)malloc(sizeof(DLNode));
r->data = x;
r->freq = 1;
r->rLink = p->rLink;
r->lLink = p;
p->rLink = r;
}
else
{
DLNode *r = p->rLink;
r->rLink->lLink = p;
p->rLink = r->rLink;
r->freq += 1;
DLNode *t = p;
while(t != L)
{
if(r->freq <= t->freq)
{
break;
}
t = t->lLink;
}
r->rLink = t->rLink;
t->rLink->lLink = r;
r->lLink = t;
t->rLink = r;
}
}
2、

#include<stdio.h>
#define maxn 100
int heap[maxn] = {0, 2, 6, 8, 9, 3, 7, 4, 0, 33, 24};
int n = 10;
void swap(int *a, int *b)
{
int tmp = *a;
*a = *b;
*b = tmp;
}
int downAdjust(int low, int high)
{
int i = low;
int j = i * 2;
while(j <= high)
{
if(j + 1 <= high && heap[j + 1] > heap[j])
{
j = j + 1;
}
if(heap[j] > heap[i])
{
swap(&heap[i], &heap[j]);
i = j;
j = i * 2;
}
else
{
break;
}
}
}
void createHeap()
{
int i;
for(i = n / 2; i >= 1; i--)
{
downAdjust(i, n);
}
}
void heapSort()
{
int i;
createHeap();
for(i = n; i > 1; i--)
{
swap(&heap[i], &heap[1]);
downAdjust(1, i - 1);
}
}
2018真题
C语言大题
1、

#include<stdio.h>
#include<math.h>
int main()
{
double xi;
double sum = 0.0;
int cnt;
for(cnt = 1; cnt <= 20; cnt++)
{
scanf("%lf", &xi);
sum += pow((1.5 * xi - 3), 5);
}
printf("%lf", sum);
}
2、

#include<stdio.h>
typedef struct Record{
int workerId;
char workerName[10];
double workerWages;
double workerAllowance;
double workerRealWages;
}Record;
int main()
{
Record worker[10] = {
1, "Alan", 90, 80, 0,
2, "Bob", 90, 86, 0,
3, "Cindy", 90, 88, 0,
4, "Dylan", 92, 80, 0,
5, "Elen", 96, 80, 0,
6, "Frank", 99, 80, 0,
7, "Galen", 98, 80, 0,
8, "Halen", 90, 83, 0,
9, "Iwan", 91, 80, 0,
10, "Jenny", 95, 80, 0,
};
double sumWorkerRealWages = 0.0;
int cnt = 0;
for(; cnt < 10; cnt++)
{
worker[cnt].workerRealWages = worker[cnt].workerWages + worker[cnt].workerAllowance;
sumWorkerRealWages += worker[cnt].workerRealWages;
}
double averageRealWages = sumWorkerRealWages / 10;
for(cnt = 0; cnt < 10; cnt++)
{
if(worker[cnt].workerRealWages > averageRealWages)
{
printf("%d %s %lf ", worker[cnt].workerId, worker[cnt].workerName, worker[cnt].workerWages);
printf("%lf %lf\n", worker[cnt].workerAllowance, worker[cnt].workerRealWages);
}
}
return 0;
}
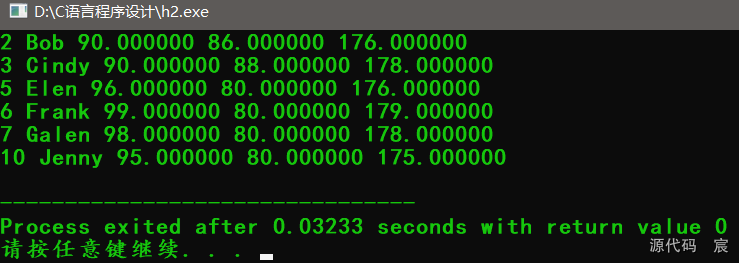
数据结构大题
1、

#include<stdio.h>
#include<stdlib.h>
typedef int ElemType;
typedef struct LNode{
ElemType data;
struct LNode *next;
}LNode, *LinkList;
int initList(LinkList *L)
{
*L = (LNode *)malloc(sizeof(LNode));
if(*L == NULL)
{
return 0;
}
(*L)->next = NULL;
return 1;
}
int listInsert(LinkList *L, int i, ElemType e)
{
if(i < 1)
{
return 0;
}
LNode *p;
int j = 0;
p = *L;
while(p != NULL && j < i - 1)
{
p = p->next;
j++;
}
if(p == NULL)
{
return 0;
}
LNode *s = (LNode *)malloc(sizeof(LNode));
s->data = e;
s->next = p->next;
p->next = s;
return 1;
}
void convertList(LinkList *L, ElemType n)
{
LNode *p = *L;
LNode *q, *r;
int i;
for(i = 0; i < n / 2; i++)
{
p = p->next;
}
q = p->next;
p->next = NULL;
while(q != NULL)
{
r = q->next;
q->next = p->next;
p->next = q;
q = r;
}
q = p->next;
p->next = NULL;
r = (*L)->next;
LNode *tmp = r->next;
LNode *q1 = q->next;
while(r != NULL)
{
q->next = r->next;
r->next = q;
q = q1;
if(q1 == NULL)
{
break;
}
q1 = q1->next;
if(tmp == NULL)
{
break;
}
r = tmp;
tmp = tmp->next;
}
if(tmp == NULL && q != NULL)
{
r->next->next = q;
}
}
int main()
{
int i = 1;
LinkList L;
initList(&L);
for(; i < 6; i++)
{
listInsert(&L, i, i);
}
LNode *p = L->next;
for(i = 0; i < 5; i++)
{
printf("i = %d\t", p->data);
p = p->next;
}
printf("\n奇数转置新链表:\n");
convertList(&L, 5);
p = L->next;
for(i = 0; i < 5; i++)
{
printf("i = %d\t", p->data);
p = p->next;
}
printf("\n\n\n");
LinkList L1;
initList(&L1);
for(i = 1; i < 7; i++)
{
listInsert(&L1, i, i);
}
LNode *p2 = L1->next;
for(i = 0; i < 6; i++)
{
printf("i = %d\t", p2->data);
p2 = p2->next;
}
printf("\n偶数转置新链表:\n");
convertList(&L1, 6);
p2 = L1->next;
for(i = 0; i < 6; i++)
{
printf("i = %d\t", p2->data);
p2 = p2->next;
}
return 0;
}
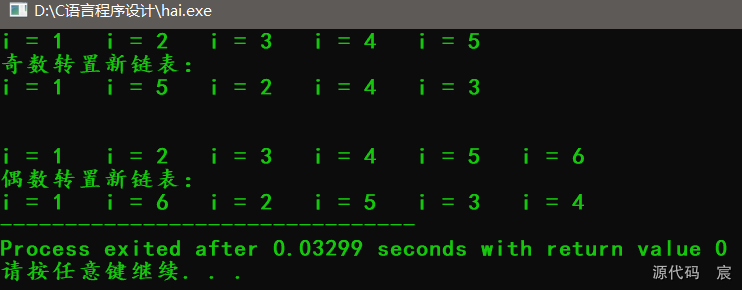
2、

关键答题代码
#include<stdio.h>
typedef struct BiTNode{
char data;
struct BiTNode *lchild;
struct BiTNode *rchild;
}BiTNode, *BiTree;
int L = 1;
void leno(BiTNode *p, char x)
{
if(p != NULL)
{
if(p->data == x)
{
printf("所在第%d层\n", L);
}
++L;
leno(p->lchild, x);
leno(p->rchild, x);
--L;
}
}
int main()
{
BiTree T;
CreateBiTree(&T);
char c = 'E';
leno(T, c);
return 0;
}
测试代码
#include<stdio.h>
#include<stdlib.h>
#include<math.h>
#define MAX_SIZE 30
#define NLAYER 4
typedef struct BiTNode{
char data;
struct BiTNode *lchild;
struct BiTNode *rchild;
}BiTNode, *BiTree;
void CreateBiTree(BiTree *bt)
{
char ch;
scanf("%c", &ch);
if(ch == '#')
{
*bt = NULL;
}
else
{
*bt = (BiTree)malloc(sizeof(BiTNode));
(*bt)->data = ch;
CreateBiTree(&((*bt)->lchild));
CreateBiTree(&((*bt)->rchild));
}
}
void TranslevelPrint(BiTree bt)
{
struct node{
BiTree vec[MAX_SIZE];
int layer[MAX_SIZE];
int locate[MAX_SIZE];
int front, rear;
}q;
int i, j = 1, k = 0, nLocate;
q.front = 0;
q.rear = 0;
printf(" ");
q.vec[q.rear] = bt;
q.layer[q.rear] = 1;
q.locate[q.rear] = 20;
q.rear += 1;
while(q.front < q.rear)
{
bt = q.vec[q.front];
i = q.layer[q.front];
nLocate = q.locate[q.front];
if(j < i)
{
printf("\n");
printf("\n");
j += 1;
k = 0;
while(k < nLocate)
{
printf(" ");
k++;
}
}
while(k < (nLocate - 1))
{
printf(" ");
k++;
}
printf("%c", bt->data);
q.front = q.front + 1;
if(bt->lchild != NULL)
{
q.vec[q.rear] = bt->lchild;
q.layer[q.rear] = i + 1;
q.locate[q.rear] = (int)(nLocate - pow(2, NLAYER - i - 1));
q.rear += 1;
}
if(bt->rchild != NULL)
{
q.vec[q.rear] = bt->rchild;
q.layer[q.rear] = i + 1;
q.locate[q.rear] = (int)(nLocate + pow(2, NLAYER - i - 1));
q.rear += 1;
}
}
}
void PreOrderLeaf(BiTree root)
{
if(root != NULL)
{
if(root->lchild == NULL && root->rchild == NULL)
{
printf("%c ", root->data);
}
PreOrderLeaf(root->lchild);
PreOrderLeaf(root->rchild);
}
}
int L = 1;
void leno(BiTNode *p, char x)
{
if(p != NULL)
{
if(p->data == x)
{
printf("所在第%d层\n", L);
}
++L;
leno(p->lchild, x);
leno(p->rchild, x);
--L;
}
}
int LeafCount(BiTree root)
{
int LeafNum;
if(root == NULL)
{
LeafNum = 0;
}
else if((root->lchild == NULL) && (root->rchild == NULL))
{
LeafNum = 1;
}
else
{
LeafNum = LeafCount(root->lchild) + LeafCount(root->rchild);
}
return LeafNum;
}
int PostTreeDepth(BiTree root)
{
int hl, hr, max;
if(root != NULL)
{
hl = PostTreeDepth(root->lchild);
hr = PostTreeDepth(root->rchild);
max = hl > hr ? hl : hr;
return(max + 1);
}
else
{
return 0;
}
}
int main()
{
BiTree T;
printf("请输入二叉树结点序列:\n");
CreateBiTree(&T);
TranslevelPrint(T);
printf("\n所有叶子结点:");
PreOrderLeaf(T);
printf("\n输出叶子结点个数:%d\n",LeafCount(T));
printf("树的深度为:%d\n",PostTreeDepth(T));
char c = 'E';
leno(T, c);
return 0;
}
很明显E确实是在第三层 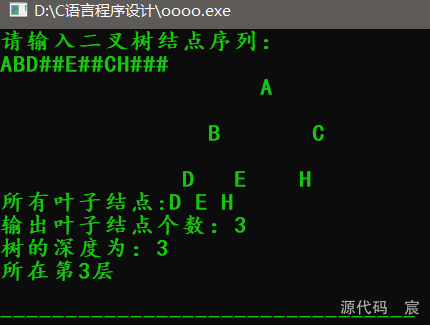
2019真题
C语言大题
1、
 代码一:
#include<stdio.h>
#include<math.h>
int main()
{
int n;
scanf("%d", &n);
int i;
int tmp = 1;;
int sum;
for(i = 1; i <= n; i++)
{
tmp *= i;
sum += tmp;
}
printf("sum = %d\n", sum);
return 0;
}
代码二:
#include<stdio.h>
int func(int n)
{
int sum = 0;
int i;
for(i = 1; i <= n; i++)
{
int mul = 1;
int j;
for(j = i; j >= 1; j--)
{
mul *= j;
}
sum += mul;
}
return sum;
}
int main()
{
int n;
scanf("%d", &n);
printf("%d", func(n));
return 0;
}
2、
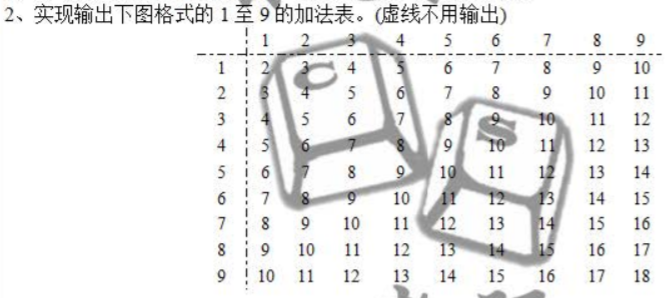 代码一:
#include<stdio.h>
int main()
{
int a[10][10] = {0};
int i;
int j;
for(i = 1; i < 10; i++)
{
a[0][i] = i;
}
for(j = 1; j < 10; j++)
{
a[j][0] = j;
}
for(i = 1; i < 10; i++)
{
for(j = 0; j < 9; j++)
{
a[i][j + 1] = a[i][j] + 1;
}
}
for(i = 0; i < 10; i++)
{
for(j = 0; j < 10; j++)
{
if(a[i][j] == 0)
{
printf(" ");
}
else
{
printf("%5d ", a[i][j]);
}
}
printf("\n");
}
return 0;
}
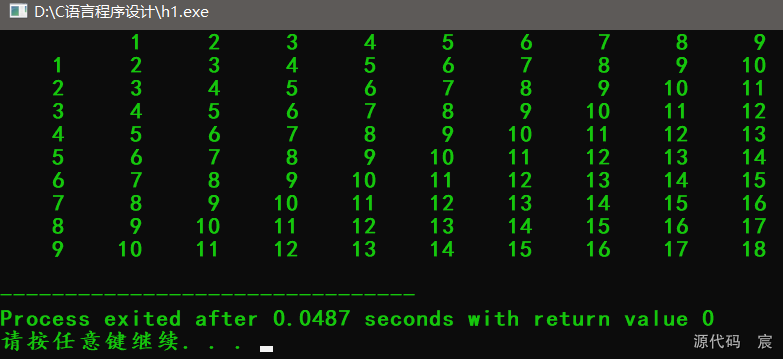 代码二:
#include<stdio.h>
int multiTable()
{
printf("\t|");
int colNum = 1;
for(; colNum < 10; colNum++)
{
printf("%d\t", colNum);
}
printf("\n");
int j;
for(j = 1; j <= 10; j++)
{
printf("-------\t");
}
int rowNum;
printf("\n");
for(rowNum = 1; rowNum < 10; rowNum++)
{
printf("%d\t|", rowNum);
colNum = 1;
for(; colNum < 10; colNum++)
{
printf("%d\t", rowNum + colNum);
}
printf("\n");
}
}
int main()
{
multiTable();
return 0;
}
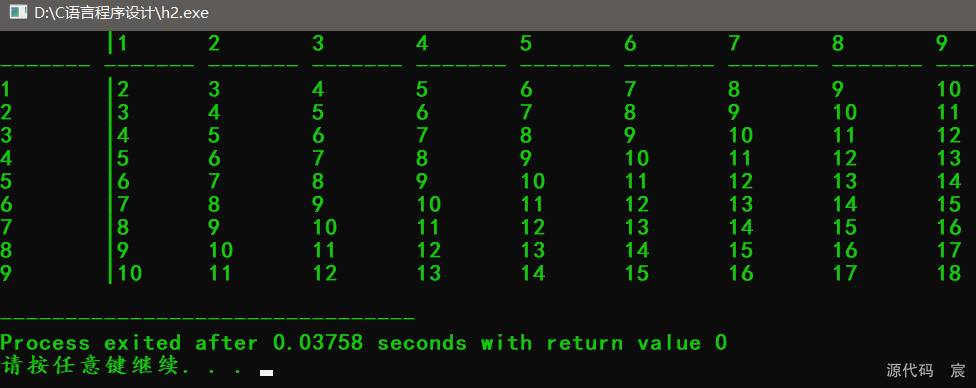
数据结构大题
1、
 需考虑的特殊情况: 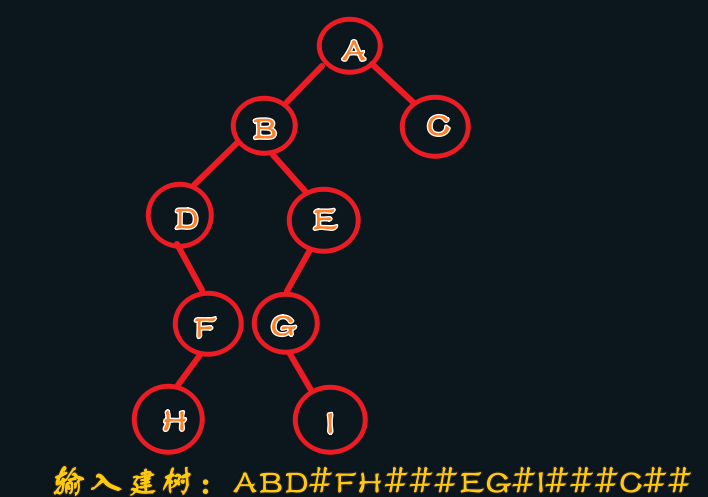 关键代码:
#include<stdio.h>
#include<stdlib.h>
typedef struct BiTNode{
char data;
struct BiTNode *lchild, *rchild;
}BiTNode, *Bitree;
void CreateBitree(Bitree *bt)
{
char ch;
scanf("%c", &ch);
if(ch == '#')
{
*bt = NULL;
}
else
{
*bt = (Bitree)malloc(sizeof(BiTNode));
(*bt)->data = ch;
CreateBitree(&(*bt)->lchild);
CreateBitree(&(*bt)->rchild);
}
}
int PostTreeDepth(Bitree root)
{
int hl, hr, max;
if(root != NULL)
{
hl = PostTreeDepth(root->lchild);
hr = PostTreeDepth(root->rchild);
max = hl > hr ? hl : hr;
return(max + 1);
}
else
{
return 0;
}
}
int maxdistance = 0;
int maxDistance(Bitree T)
{
if(T == NULL)
{
return maxdistance;
}
int l = PostTreeDepth(T->lchild);
int r = PostTreeDepth(T->rchild);
int cnt = l + r;
if(cnt > maxdistance)
{
maxdistance = cnt;
}
maxDistance(T->lchild);
maxDistance(T->rchild);
return maxdistance;
}
int main()
{
Bitree T;
printf("请输入二叉树结点序列:\n");
CreateBitree(&T);
printf("\n");
PostTreeDepth(T);
printf("最远距离:%d\n", maxDistance(T));
return 0;
}
完整代码:
#include<stdio.h>
#include<stdlib.h>
#include<math.h>
#define MAX_SIZE 30
#define NLAYER 5
typedef struct BiTNode{
char data;
struct BiTNode *lchild, *rchild;
}BiTNode, *Bitree;
void CreateBitree(Bitree *bt)
{
char ch;
scanf("%c", &ch);
if(ch == '#')
{
*bt = NULL;
}
else
{
*bt = (Bitree)malloc(sizeof(BiTNode));
(*bt)->data = ch;
CreateBitree(&(*bt)->lchild);
CreateBitree(&(*bt)->rchild);
}
}
void preorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
printf("%c ", bt->data);
preorder(bt->lchild);
preorder(bt->rchild);
}
void inorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
inorder(bt->lchild);
printf("%c ", bt->data);
inorder(bt->rchild);
}
void postorder(Bitree bt)
{
if(bt == NULL)
{
return;
}
postorder(bt->lchild);
postorder(bt->rchild);
printf("%c ", bt->data);
}
int PostTreeDepth(Bitree root)
{
int hl, hr, max;
if(root != NULL)
{
hl = PostTreeDepth(root->lchild);
hr = PostTreeDepth(root->rchild);
max = hl > hr ? hl : hr;
return(max + 1);
}
else
{
return 0;
}
}
int maxdistance = 0;
int maxDistance(Bitree T)
{
if(T == NULL)
{
return maxdistance;
}
int l = PostTreeDepth(T->lchild);
int r = PostTreeDepth(T->rchild);
int cnt = l + r;
if(cnt > maxdistance)
{
maxdistance = cnt;
}
maxDistance(T->lchild);
maxDistance(T->rchild);
return maxdistance;
}
void TranslevelPrint(Bitree bt)
{
struct node{
Bitree vec[MAX_SIZE];
int layer[MAX_SIZE];
int locate[MAX_SIZE];
int front, rear;
}q;
int i, j = 1, k = 0, nLocate;
q.front = 0;
q.rear = 0;
printf(" ");
q.vec[q.rear] = bt;
q.layer[q.rear] = 1;
q.locate[q.rear] = 20;
q.rear += 1;
while(q.front < q.rear)
{
bt = q.vec[q.front];
i = q.layer[q.front];
nLocate = q.locate[q.front];
if(j < i)
{
printf("\n");
printf("\n");
j += 1;
k = 0;
while(k < nLocate)
{
printf(" ");
k++;
}
}
while(k < (nLocate - 1))
{
printf(" ");
k++;
}
printf("%c", bt->data);
q.front = q.front + 1;
if(bt->lchild != NULL)
{
q.vec[q.rear] = bt->lchild;
q.layer[q.rear] = i + 1;
q.locate[q.rear] = (int)(nLocate - pow(2, NLAYER - i - 1));
q.rear += 1;
}
if(bt->rchild != NULL)
{
q.vec[q.rear] = bt->rchild;
q.layer[q.rear] = i + 1;
q.locate[q.rear] = (int)(nLocate + pow(2, NLAYER - i - 1));
q.rear += 1;
}
}
}
int main()
{
Bitree T;
printf("请输入二叉树结点序列:\n");
CreateBitree(&T);
printf("\n");
TranslevelPrint(T);
printf("\n");
PostTreeDepth(T);
printf("最远距离:%d\n", maxDistance(T));
return 0;
}
测试样例: ABD#FH###EG#I###C## 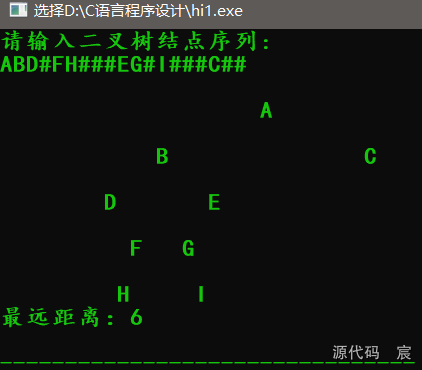
2、

#define MAXSIZE 100
typedef int ElemType
typedef struct SqList{
ElemType *elem;
int length;
int maxsize;
}SqList;
void moveValueBehind(ElemType value, SqList *L, int value)
{
int lowIndex = 0;
int highInedex = L->length - 1;
while(lowIndex < highIndex)
{
while(lowIndex < highIndex)
{
if(L->elem[lowIndex] == value)
{
break;
}
else
{
lowIndex++;
}
}
while(lowIndex < highIndex)
{
if(L->elem[highIndex] != value)
{
break;
}
else
{
highIndex--;
}
}
if(lowIndex < highInex)
{
ElemType tmp = L->elem[lowIndex];
L->elem[lowIndex] = L->elem[highIndex];
L->elem[highIndex] = tmp;
}
}
}
2020真题
C语言大题
1、
 代码一:
#include<stdio.h>
int main()
{
int n;
scanf("%d", &n);
double s;
int i;
int tmp1 = 0;
for(i = 1; i <= n; i++)
{
tmp1 += i;
s += 1.0/ tmp1;
}
printf("%lf\n", s);
return 0;
}
代码二:
#include<stdio.h>
float getResult(int n)
{
float s = 0;
int deno = 0;
int i;
for(i = 1; i <= n; i++)
{
deno += i;
s += 1.0 / deno;
}
return s;
}
int main()
{
int n;
scanf("%d", &n);
float res = getResult(n);
printf("%f\n", res);
return 0;
}
2、
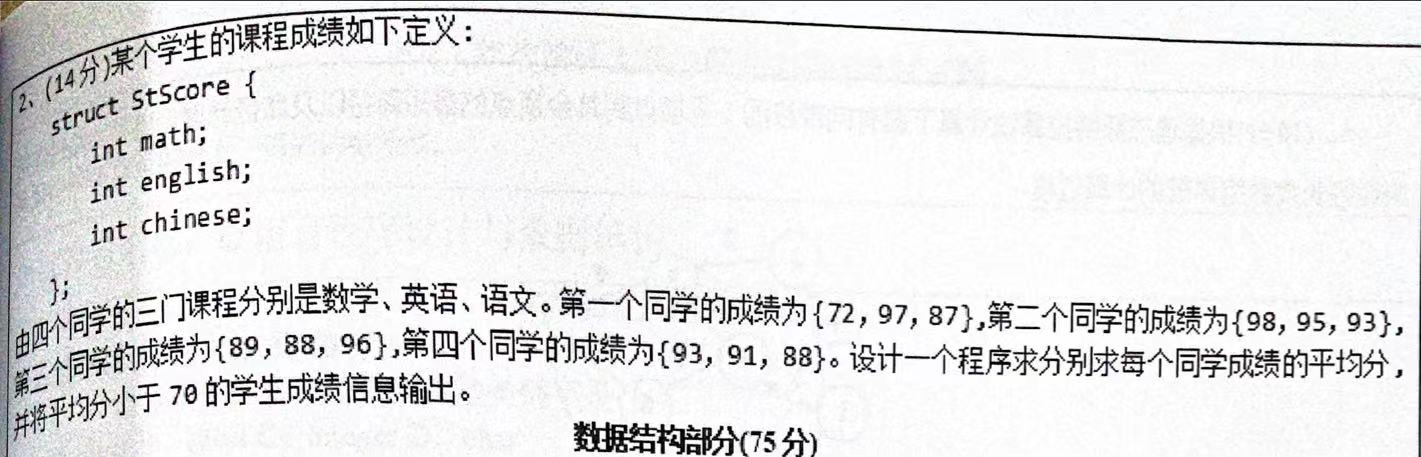
#include<stdio.h>
typedef struct StScore{
int math;
int english;
int chinese;
}StScore;
int main()
{
StScore student[4] = {
72, 97, 87,
98, 95, 93,
89, 88, 96,
93, 91, 88
};
int i;
int aver = 0;
for(i = 0; i < 4; i++)
{
aver = student[i].math + student[i].english + student[i].chinese;
aver /= 4;
if(aver < 70)
{
printf("No.%d student's info:\nmath:%d\tenglish:%d\tchinese:%d\n", i + 1, student[i].math, student[i].english, student[i].chinese);
}
}
return 0;
}
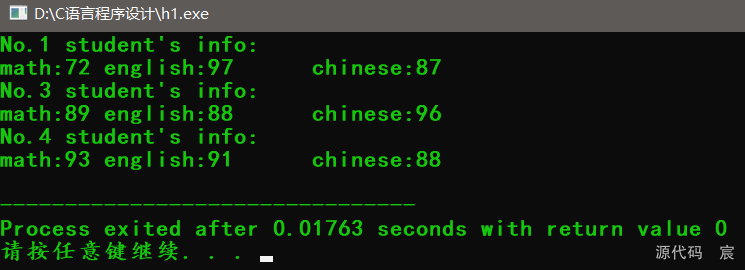
数据结构大题
1、请设计算法将循环单链表中每个结点左移K
typedef int ElemType;
typedef struct CircularLinkNode{
ElemType data;
struct CircularLinkNode *nextNode;
}CircularLinkNode, CircularLinkList;
void move(int k, CircularLinkList *L)
{
CircularLinkNode *pCurNode = L;
int cnt = 1;
while(cnt <= k)
{
pCurNode = pCurNode->nextNode;
cnt++;
}
L = pCurNode;
}
2、设计算法求二叉链表结构存储的二叉树中每层结点的最大值
typedef char ElemType;
typedef struct BiTNode{
ElemType data;
struct BiTNode *lchild, *rchild;
}BiTNode, *BiTree;
typedef struct QElemType{
BiTNode *pNode;
int nodeLevel;
}QElemType;
int getWidthBiTree(BiTree T)
{
if(T == NULL)
{
return 0;
}
Queue Q;
QElemType e;
e.pNode = T;
e.nodeLevel = 1;
initialQueue(Q);
enQueue(Q, e);
int currentLevel = 1;
int maxValue = T->data;
while(!isEmpty(Q))
{
deQueue(Q, e);
ElemType elemData = (e.pNode)->data;
if(currentLevel == e.nodeLevel)
{
if(maxValue < elemData)
{
maxValue = elemData;
}
}
else
{
printf("%d", maxValue);
currentLevel = e.nodeLevel;
maxValue = elemData;
}
QElemType tmp;
BiTNode *p = e.pNode;
if(NULL != (e.pNode)->lchild)
{
tmp.pNode = (e.pNode)->lchild;
tmp.nodeLevel = e.nodeLevel + 1;
enQueue(Q, tmp);
}
if(NULL != (e.pNode)->rchild)
{
tmp.pNode = (e.pNode)->rchild;
tmp.nodeLevel = e.nodeLevel + 1;
enQueue(Q, tmp);
}
}
}
2021真题
C代码:(注意一定要模块化!!!)
#include<stdio.h>
#define MAX_LENGTH 10
typedef struct BankInfo{
int ID;
char name[MAX_LENGTH];
char passWord[MAX_LENGTH];
float balance;
}BankInfo;
float getAverage(BankInfo infList[], int infListLength)
{
float sum = 0;
int i;
for(i = 0; i < infListLength; i++)
{
sum += infList[i].balance;
}
return sum / infListLength;
}
void printBigger(float avg, BankInfo infList[], int infListLength)
{
int i;
for(i = 0; i < infListLength; i++)
{
if(avg <= infList[i].balance)
{
printf("%d %s %s %f\n",infList[i].ID, infList[i].name, infList[i].passWord, infList[i].balance);
}
}
}
int enterInf(BankInfo infList[])
{
int infListLength = 0;
scanf("%d", &infListLength);
int i = 0;
for(; i < infListLength; i++)
{
scanf("%d %s %f", &infList[i].ID, infList[i].name, &infList[i].balance);
gets(infList[i].passWord);
}
return infListLength;
}
int main()
{
BankInfo infList[90];
int infListLength = 0;
infListLength = enterInf(infList);
float avg = getAverage(infList, infListLength);
printBigger(avg, infList, infListLength);
return 0;
}
下面这个代码是反面教材
#include<stdio.h>
typedef struct BankAccount{
int ID;
char name[20];
char password[30];
double money;
}BankAccount;
int main()
{
BankAccount account[90] = {1, "LiMing", "123", 50.0, 2, "LiHua", "321", 99.9, 3, "Alan", "132", 96.9};
int i = 0;
for(i = 3; i < 90; i++)
{
account[i].ID = -1;
}
double sumMoney = 0;
double averMoney = 0;
i = 0;
while(account[i].ID != -1)
{
sumMoney += account[i].money;
i++;
}
int cnt = i;
averMoney = sumMoney / i;
for(i = 0; i < cnt; i++)
{
if(account[i].money > averMoney)
{
printf("UserID:%d\tUsername:%s\tUserpassword:%s\tUsermoney:%lf\n", account[i].ID, account[i].name, account[i].password, account[i].money);
}
}
return 0;
}
第二个题的C代码:
#include<stdio.h>
float fun(int m)
{
float sum = 1;
int i;
for(i = 2; i <= m; i++)
{
sum = sum - 1.0 / (i * i);
}
return sum;
}
int main()
{
int m;
scanf("%d", &m);
float sum = fun(m);
printf("%f", sum);
return 0;
}
数据结构大题
1、设计算法在有序单链表中删除重复元素e,使得单链表删除e后依然有序
#include<stdio.h>
typedef int ElemType;
typedef struct LNode{
ElemType data;
struct LNode *next;
int length;
}LNode, *LinkList;
void deleteValue(int e, LinkList *L)
{
int cnt = 0;
int i;
int flag = 0;
LNode *p = (*L)->next;
LNode *q;
for(i = 0; i < (*L)->length; i++)
{
if(p->data == e)
{
if(flag == 0)
{
flag = 1;
}
else
{
LNode *p1 = p;
p = p->next;
q->next = p;
free(p1);
}
}
q = p;
p = p->next;
}
}
2、设计算法在二叉排序树中查找第k大的结点
BSTNode *pNodeK = NULL;
int iCurOrder = 0;
void inTravsing(BSTree T, int k)
{
if(T != NULL)
{
inTravsing(T->rchild, k);
iCurOrder += 1;
if(iCurOrder == k)
{
pNodeK = T;
}
else if(pNodeK == NULL)
{
inTravsing(T->lchild, k);
}
}
}
BSTNode *getTop(int k, BSTree T)
{
inTravsing(T, k);
return pNodeK;
}
之后我会持续更新,如果喜欢我的文章,请记得一键三连哦,点赞关注收藏,你的每一个赞每一份关注每一次收藏都将是我前进路上的无限动力 !!!↖(▔▽▔)↗感谢支持!
|