?大一的C语言课设,希望能给学弟学妹们一些启发
首先,本程序基于easyx图形接口开发,运行前先下载easyx.h graphics.h 等头文件
百度云链接:https://pan.baidu.com/s/1tru8LykTctCYnyra9dXF2A? 提取码:0326
建议程序在vs上运行
将两个头文件放在下面的目录中
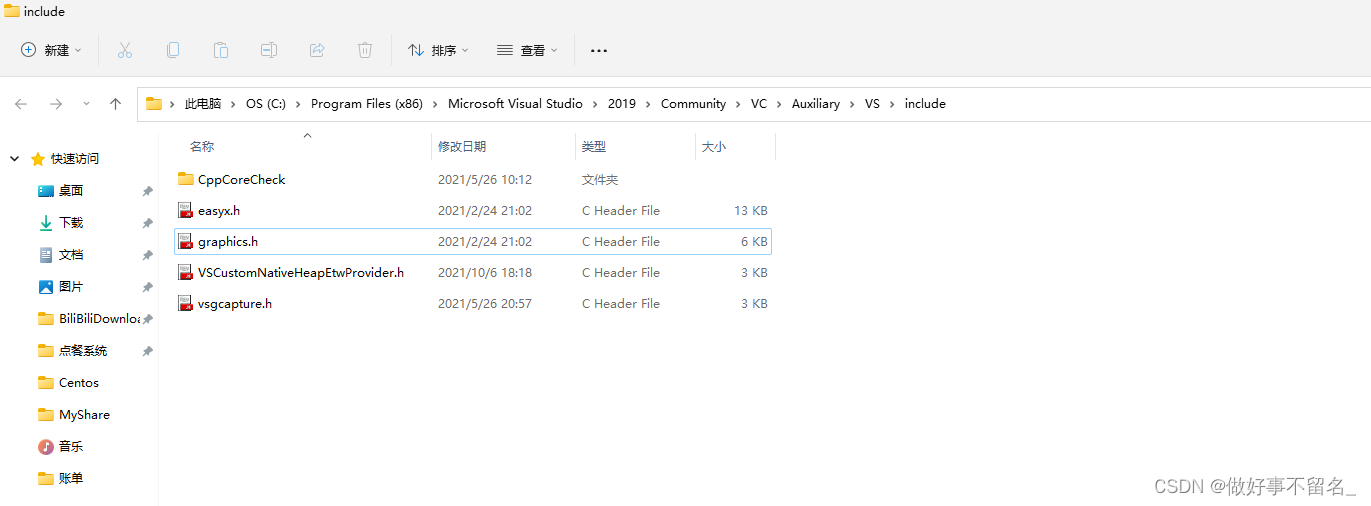
?图片等素材:
链接:https://pan.baidu.com/s/1Xh0mnYk52Xd29i_Us4btlA? 提取码:0326
?
想法来自于海底捞的点餐系统,当时不想课设局限于黑乎乎的黑窗口,显得很low
就从网上找了C语言如何写图形界面的程序,其中对新手最友好的就是easyx了。
上手容易,操作简便。这个程序就是边学边做一周完成的。
写这个的时候还是有些波折的。因为这个程序没有用前端的内容,所以各种东西都要自己找
首先需要就是图形素材,当时在网上找各种菜的图片,程序的背景,还有背景音乐等。
当时有一个问题就是图片经过拉伸之后清晰度会下降很多,后来才想到将图片不等比例拉伸
就会这样,等比例拉伸就好了。
程序的启动页面
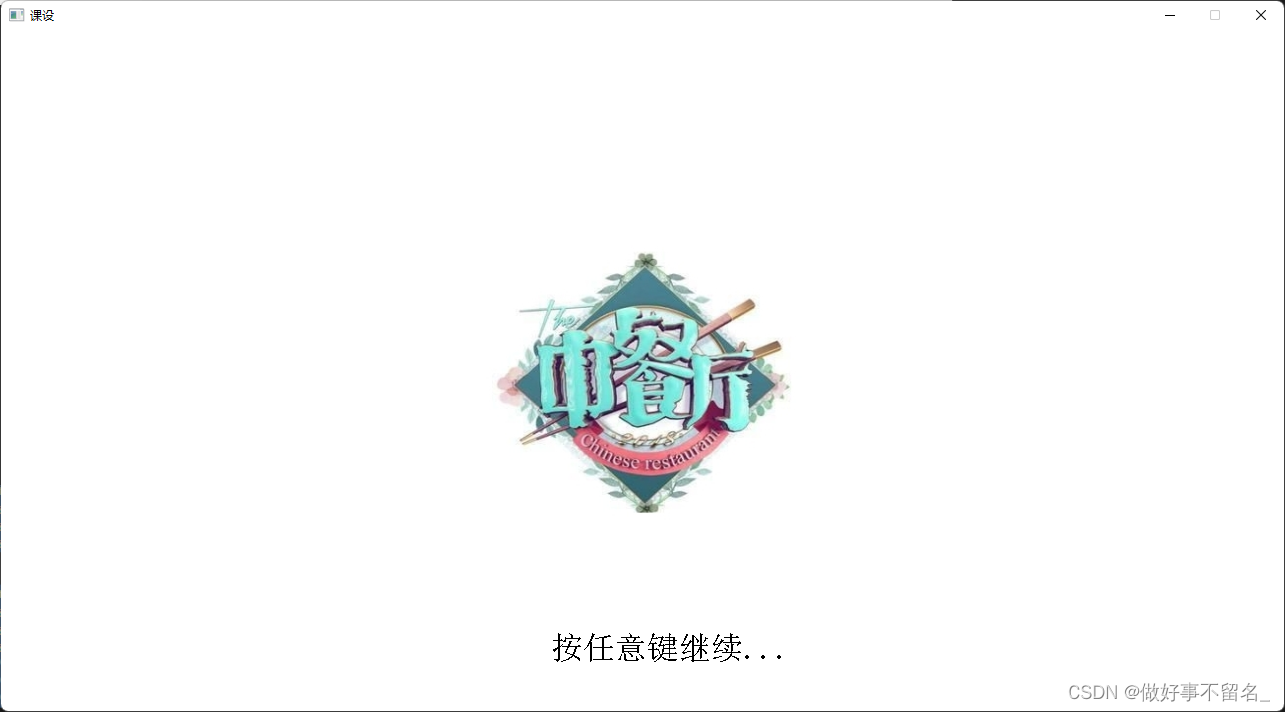
各种图片拼凑成的点餐界面:
? ? ? ? 在点餐界面用户通过点击菜品可将其添加到菜单中(使用链表加结构体储存)
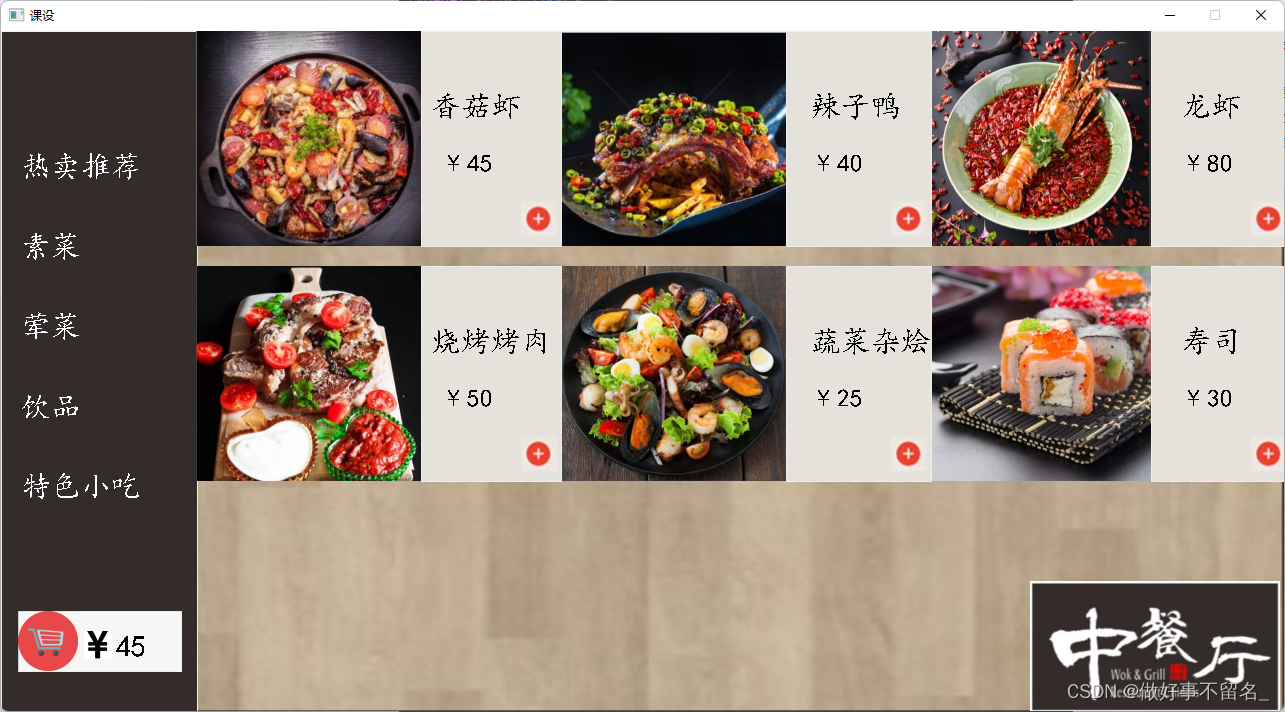
?
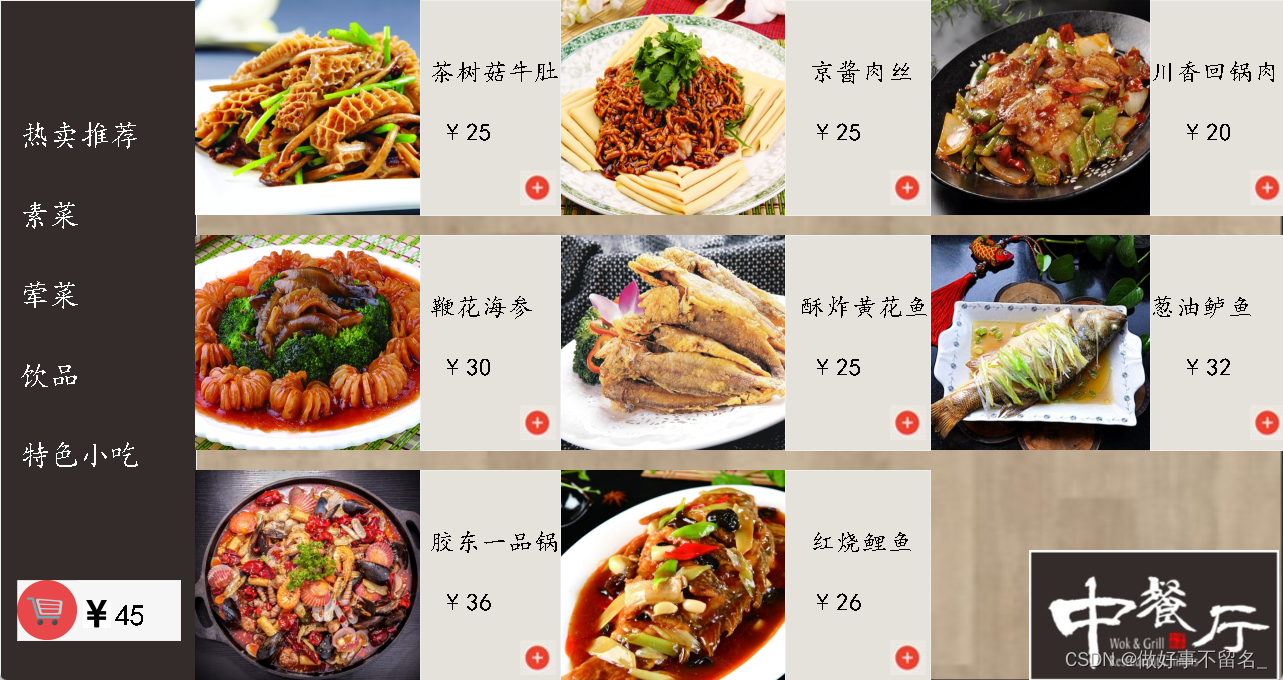
?订单界面
? ? ? ? 可在此页面对自己的菜品进行修改,满意之后提交菜单,此时程序会将生成的菜单信息
生成到后台文件中,以一个xlsx表格文件储存
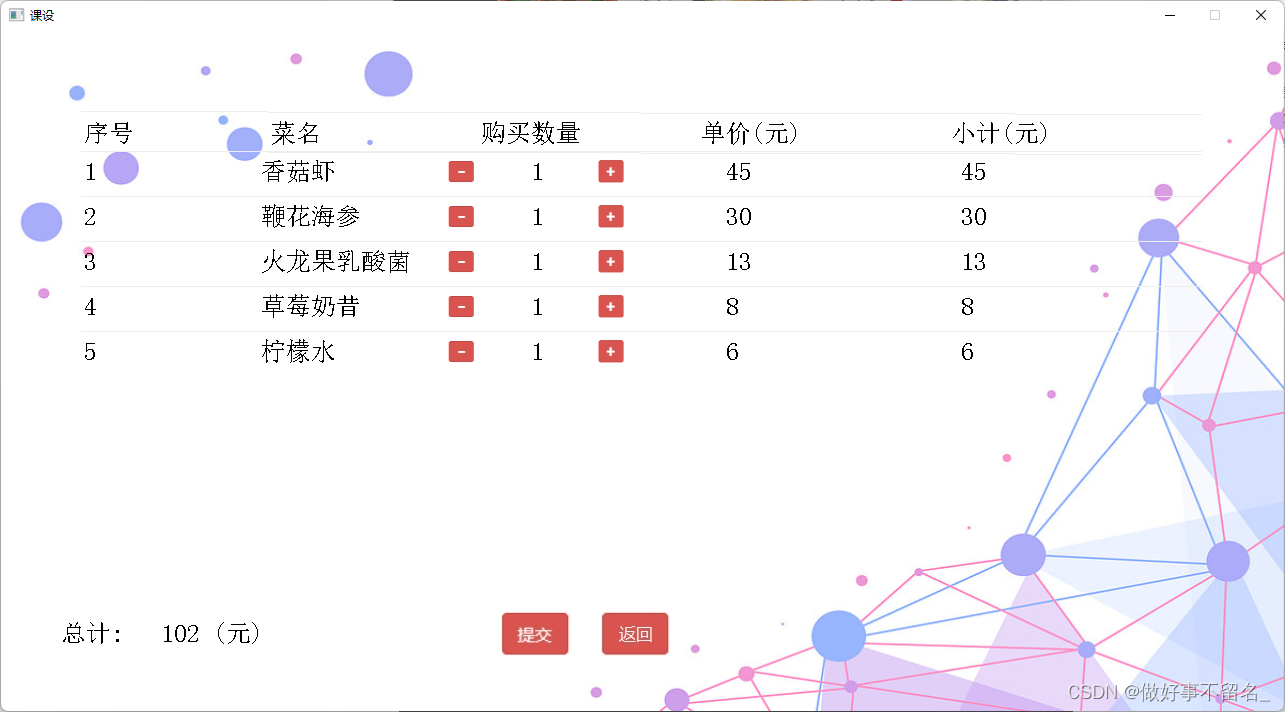
?生成的订单表格
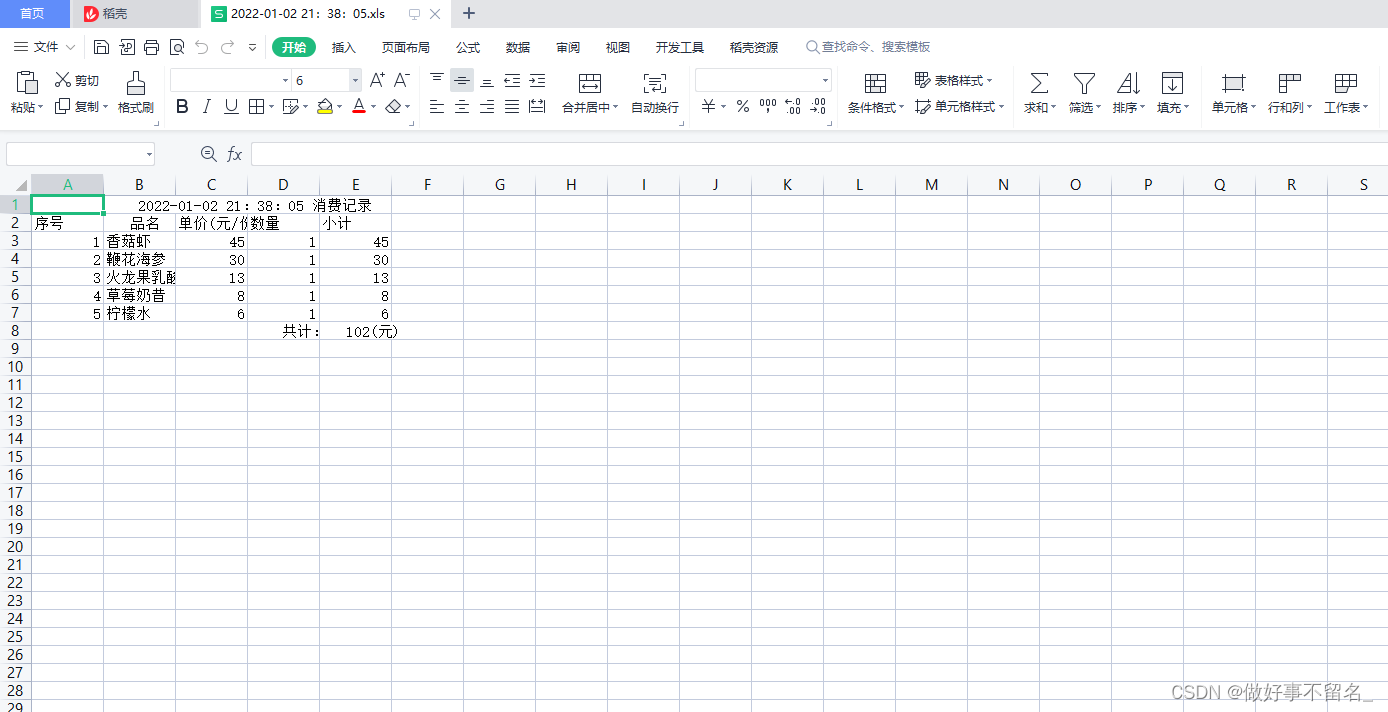
?
?源代码:
#include<stdio.h>
#include<stdlib.h>
#include<io.h>
#include<string.h>
#include<time.h>
#include<graphics.h>
#include<conio.h>//使用_getch();
#include<mmsystem.h>//包含多媒体设备接口头文件
#pragma comment(lib,"winmm.lib") //medium
FILE* CheckBills; //账单
char date[80]; //账单编号
typedef struct Food {//销量
char name[50];
float price;
int sales;
}FOOD;
typedef struct Bill {
char name[30];
int price;
int num;
struct Bill* next;
}BILL;
typedef struct Dishes {
char name[30];
float price;
int sales;
struct Dishes* next;
}DISH;
BILL* head = NULL,*sortHead=NULL,*tail,*r;
IMAGE img0,img,img1,img2,img3,img4,img5,img6,img7,img8, img9, img10, img11, img12, img13, img14, img15, img16;
IMAGE img17, img18, img19, img20, img21, img22, img23, img24, img25, img26, img27, img28, img29, img30;
IMAGE img31, img32, img33, img34, img35, img36, img37, img38, img39, img40, img41, img42, img43, img44;
IMAGE img45, img46, img47, img48, img49, img50, img51, img52, img53, img54, img55, img56, img57, img58;
void BGM();
void login();
void frame();
void selling();
void initial();
void vDish();
void mDish();
void drinks();
void spDishes();
void choose();
void spDishes1();
void oSelling();
void oVdish();
void oMdish();
void oDrinks();
void oSp();
void sMenu();
void addDish();
void print();
void check();
int total=0,_price=0;
char* int_to_string(int num);
int main(void) {
initial();
BGM();
login();
choose();
int a =_getch();
closegraph();
}
void check(int a,int flag) {//订单的刷新
cleardevice();
frame();
switch (flag) {
case 1: {
selling();
break;
}
case 2: {
vDish();
break;
}
case 3: {
mDish();
break;
}
case 4: {
drinks();
break;
}
case 5: {
spDishes();
break;
}
}
total += a;
char s[40];
settextstyle(30,0,"楷体");
strcpy_s(s, int_to_string(total));
outtextxy(115,600,s);
}
void sMenu() {
struct tm t;
time_t now;
time(&now);
localtime_s(&t, &now);
char str[100],str1[100];
strftime(str, sizeof(str), "%Y-%m-%d %H:%M:%S", &t); //在文件命名时要注意部分英文字符不能用
//char date1[100];
strcpy_s(str1,str);
strcat_s(str, ".xls");
char date[100] = "./账单/";
//strcat_s(date1, str);
strcat_s(date,str);
errno_t t1;
if ((t1 = fopen_s(&CheckBills,date,"a+")) != NULL) {//fopen成功返回0值,否则返回其他值
printf("Error!\n");
exit(1);
}
fprintf(CheckBills, "\t %s 消费记录\n", str1);
fprintf(CheckBills, "序号\t 品名 \t单价(元/份)\t数量\t小计\n");
}
void deleteNode() {//对链表进行操作
BILL **t = &head,*r;
int i = 1,sum=0;
while (*t!=NULL) {
r = *t;
fprintf(CheckBills, "%d\t%s \t%d \t%d \t%d\n",i,r->name,r->price,r->num,r->price*r->num);
sum += r->price*r->num;
*t = (*t)->next;
free(r);
i++;
}
fprintf(CheckBills, "\t \t \t %s\t %d%s\n","共计:", sum,"(元)");
fclose(CheckBills);
}
char* int_to_string(int num)
{
int i = 0, j = 0;
char temp[10], str[10];
while (num)
{
temp[i++] = num % 10 + '0'; //将数字加字符0就变成相应字符
num /= 10; //此时的字符串为逆序
}
temp[i] = '\0';
i = i - 1;
while (i >= 0)
str[j++] = temp[i--]; //将逆序的字符串转为正序
str[j] = '\0'; //字符串结束标志
return str;
}
void changeNum(int num,int flag) {
BILL* t = head;
for (int i = 0; i < num-1;i++) {
t = t->next;
}
if (t != NULL) {
if (flag == 0) {
if (t->num <= 0) {
return;
}
else {
t->num--;
}
}
else if (flag == 1) {
t->num++;
}
}
}
void sortNode() {
}
void getMes() {//可以通过点击按钮对链表元素进行修改
while (1) {
if (MouseHit()) {
MOUSEMSG msg = GetMouseMsg(); //消息分发
switch (msg.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg.x >= 500 && msg.x <= 570 && msg.y >= 580 && msg.y <= 630) {// 结算窗口 处理订单
setbkmode(TRANSPARENT);
HWND hnd = GetHWnd();
SetWindowText(hnd, "中餐厅");
int isok = MessageBox(hnd, "下单成功!", "中餐厅", MB_OKCANCEL);
deleteNode();
total = 0;
_price = 0;
choose();
}
else if(msg.x >= 600 && msg.x <= 670 && msg.y >= 580 && msg.y <= 630){
choose();
}
/*1*/ else if( msg.y >= 128 && msg.y <= 153){
if ( msg.x >= 445 && msg.x <= 475) {
changeNum(1, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(1, 1);
print();
}
}
/*2*/ else if (msg.y >= 173 && msg.y <= 198) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(2, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(2, 1);
print();
}
}
else if (msg.y >= 218 && msg.y <= 243) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(3, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(3, 1);
print();
}
}
else if (msg.y >= 263 && msg.y <= 288) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(4, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(4, 1);
print();
}
}
else if (msg.y >= 308 && msg.y <= 333) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(5, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(5, 1);
print();
}
}
else if (msg.y >= 353 && msg.y <= 378) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(6, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(6, 1);
print();
}
}
else if (msg.y >= 398 && msg.y <= 423) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(7, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(7, 1);
print();
}
}
else if (msg.y >= 443 && msg.y <= 468) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(8, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(8, 1);
print();
}
}
else if (msg.y >= 488 && msg.y <= 513) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(9, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(9, 1);
print();
}
}
else if (msg.y >= 533 && msg.y <= 558) {
if (msg.x >= 445 && msg.x <= 475) {
changeNum(10, 0);
print();
}
else if (msg.x >= 595 && msg.x <= 625) {
changeNum(10, 1);
print();
}
}
}
}
}
}
}
void getDish() {
int sum = 0;
BILL* t = head;
char name[30],ii[20],s[30],sum_[30],s1[30],num[10];
int price,x=88,y=120,i=1;
setlinecolor(RGB(238, 238, 238));
settextcolor(BLACK);
while (t!=NULL) {
strcpy_s(name,t->name);
strcpy_s(ii,int_to_string(i));
strcpy_s(s,int_to_string(t->price));
strcpy_s(s1, int_to_string(t->num * t->price));
strcpy_s(num,int_to_string(t->num));
outtextxy(83, y + 8, ii);
outtextxy(260,y+8,name);
putimage(445, y+8, &img52);//30 25
outtextxy(530,y+8,num);
putimage(595, y+8, &img53);//30 25
outtextxy(725,y+8,s);
outtextxy(960,y+8,s1);
line(80, y, 1200, y);
y += 45;
i++;
sum += (t->price)*(t->num);
t = t->next;
}
outtextxy(60,590,"总计:");
_price = sum;
strcpy_s(sum_, int_to_string(sum));
strcat_s(sum_, " (元)");
outtextxy(160,590,sum_);
getMes();
}
void print() {
system("cls");
BILL* t = head;
while (t != NULL) {
printf("%-14s%d\n",t->name,t->price);
t = t->next;
}
cleardevice();
putimage(0,0,&img51);
putimage(500,580,&img54);//69 46 提交
putimage(600,580,&img55);//69 46 返回
setfillcolor(RGB(202, 81, 0));
//fillrectangle(410,20,810,80);// 1283, 680
settextcolor(WHITE);
/*outtextxy(550,30,"我点的菜");*/
setlinecolor(RGB(238, 238, 238));
line(80, 80, 1200, 83);
line(80, 120, 1200, 123);
settextstyle(25,0,"宋体");
settextcolor(BLACK);
outtextxy(83,90,"序号");
outtextxy(270, 90,"菜名");
outtextxy(480, 90,"购买数量");
outtextxy(700, 90,"单价(元)");
outtextxy(950, 90,"小计(元)");
getDish();//获取点过的菜
}
void login() {
setbkcolor(WHITE);
cleardevice();
putimage(0,0,&img0);
setfillstyle(TRANSPARENT);
settextcolor(BLACK);
settextstyle(32,0,"宋体");
outtextxy(550,600,"按任意键继续...");
while (1) {
if (MouseHit()) {
MOUSEMSG msg = GetMouseMsg(); //消息分发
switch (msg.uMsg)
{
case WM_MOUSEMOVE: {
continue;
}
default: {
break;
}
}
break;
}
}
cleardevice();
graphdefaults();
}
//程序初始化界面,加载图片,背景,文字等
void frame() {
setbkcolor(WHITE);
cleardevice();
putimage(0, 0, &img);
setfillcolor(RGB(52, 44, 42));
fillrectangle(0, 0, 196, 680);
setfillcolor(RGB(246, 246, 246));
fillrectangle(17, 580, 180, 640);
putimage(17,580, &img49);
settextcolor(BLACK);
settextstyle(30,0,"楷体");
putimage(83,600,&img50);//¥
settextcolor(WHITE);
settextstyle(30, 0, "楷体");
setbkmode(TRANSPARENT);
outtextxy(20, 120, "热卖推荐");
outtextxy(20, 200, "素菜");
outtextxy(20, 280, "荤菜");
outtextxy(20, 360, "饮品");
outtextxy(20, 440, "特色小吃");
settextcolor(BLACK);
}
void choose() {//开始的选择界面
sMenu();
frame();
settextstyle(30, 0, "宋体");
char price[30];
strcpy_s(price,int_to_string(_price));
outtextxy(120, 600, price);
selling();
oSelling();
}
void addDish(char *name,int price) {
BILL* search=head;//遍历指针,寻找之前是否点过
while (search!=NULL) {
if (strcmp(search->name, name) == 0) {
(search->num)++;
return;
}
search = search->next;
}
r = (BILL*)malloc(sizeof(BILL));
strcpy_s(r->name,name);
r->price = price;
r->num = 1;
if (head==NULL) {
head = r;
tail = r;
}
else {
tail->next = r;
tail = tail->next;
}
r->next = NULL;
}
void oSelling() {
while (1) {
if (MouseHit()) {
MOUSEMSG msg1 = GetMouseMsg();
switch (msg1.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg1.x >= 20 && msg1.x <= 150 && msg1.y >= 120 && msg1.y <= 150) {
cleardevice();
frame();
selling();
check(0,1);
oSelling();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 200 && msg1.y <= 230) {
vDish();
oVdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 280 && msg1.y <= 310) {
mDish();
oMdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 360 && msg1.y <= 390) {
drinks();
oDrinks();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 440 && msg1.y <= 470) {
spDishes();
oSp();
}
else if (msg1.x >= 17 && msg1.x <= 77 && msg1.y >= 580 && msg1.y <= 640) {
print();
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 170 && msg1.y <= 205) {//香菇虾
char name[] = "香菇虾";
int price = 45;
addDish(name, price);
check(45,1);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 170 && msg1.y <= 205) {//辣子鸭
char name[] = "辣子鸭";
int price = 40;
addDish(name, price);
check(40, 1);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 170 && msg1.y <= 205) {//龙虾
char name[] = "龙虾";
int price = 80;
addDish(name, price);
check(80, 1);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 405 && msg1.y <= 440) {//烧烤烤肉
char name[] = "烧烤烤肉";
int price = 50;
addDish(name, price);
check(50, 1);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 405 && msg1.y <= 440) {//蔬菜杂烩
char name[] = "蔬菜杂烩";
int price = 25;
addDish(name, price);
check(25, 1);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 405 && msg1.y <= 440) {//寿司
char name[] = "寿司";
int price = 30;
addDish(name, price);
check(30, 1);
}
}
}
}
}
}
void oVdish() {
while (1) {
if (MouseHit()) {
MOUSEMSG msg1 = GetMouseMsg();
switch (msg1.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg1.x >= 20 && msg1.x <= 150 && msg1.y >= 120 && msg1.y <= 150) {
cleardevice();
frame();
selling();
check(0, 1);
oSelling();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 200 && msg1.y <= 230) {
vDish();
oVdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 280 && msg1.y <= 310) {
mDish();
oMdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 360 && msg1.y <= 390) {
drinks();
oDrinks();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 440 && msg1.y <= 470) {
spDishes();
oSp();
}
else if (msg1.x >= 17 && msg1.x <= 77 && msg1.y >= 580 && msg1.y <= 640) {
print();
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "蒜蓉黄瓜";
int price = 20;
addDish(name, price);
check(20,2);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "甘蓝藕片";
int price = 18;
addDish(name, price);
check(18, 2);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "土豆丝 ";
int price = 15;
addDish(name, price);
check(15, 2);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "麻辣鸡丝";
int price = 15;
addDish(name, price);
check(15, 2);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "蜜豆蛋干";
int price = 20;
addDish(name, price);
check(20, 2);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "桃仁木耳";
int price = 18;
addDish(name, price);
check(18, 2);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "樱桃沙拉";
int price = 20;
addDish(name, price);
check(20, 2);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "糖醋萝卜";
int price = 25;
addDish(name, price);
check(25, 2);
}
}
}
}
}
}
void oMdish() {
while (1) {
if (MouseHit()) {
MOUSEMSG msg1 = GetMouseMsg();
switch (msg1.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg1.x >= 20 && msg1.x <= 150 && msg1.y >= 120 && msg1.y <= 150) {
cleardevice();
frame();
selling();
check(0, 1);
oSelling();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 200 && msg1.y <= 230) {
vDish();
oVdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 280 && msg1.y <= 310) {
mDish();
oMdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 360 && msg1.y <= 390) {
drinks();
oDrinks();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 440 && msg1.y <= 470) {
spDishes();
oSp();
}
else if (msg1.x >= 17 && msg1.x <= 77 && msg1.y >= 580 && msg1.y <= 640) {
print();
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "茶树菇牛肚";
int price = 25;
addDish(name, price);
check(25,3);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "京酱肉丝";
int price = 25;
addDish(name, price);
check(25, 3);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "川香回锅肉";
int price = 20;
addDish(name, price);
check(20, 3);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "鞭花海参";
int price = 30;
addDish(name, price);
check(30, 3);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "酥炸黄花鱼";
int price = 25;
addDish(name, price);
check(25, 3);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "葱油鲈鱼";
int price = 32;
addDish(name, price);
check(32, 3);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "胶东一品锅";
int price = 36;
addDish(name, price);
check(36, 3);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "红烧鲤鱼";
int price = 26;
addDish(name, price);
check(26, 3);
}
}
}
}
}
}
void oDrinks() {
while(1){
if (MouseHit()) {
MOUSEMSG msg1 = GetMouseMsg();
switch (msg1.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg1.x >= 20 && msg1.x <= 150 && msg1.y >= 120 && msg1.y <= 150) {
cleardevice();
frame();
selling();
check(0, 1);
oSelling();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 200 && msg1.y <= 230) {
vDish();
oVdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 280 && msg1.y <= 310) {
mDish();
oMdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 360 && msg1.y <= 390) {
drinks();
oDrinks();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 440 && msg1.y <= 470) {
spDishes();
oSp();
}
else if (msg1.x >= 17 && msg1.x <= 77 && msg1.y >= 580 && msg1.y <= 640) {
print();
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "冰蓝优酸乳";
int price = 12;
addDish(name, price);
check(12, 4);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "火龙果乳酸菌";
int price = 13;
addDish(name, price);
check(13, 4);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "黑糖爆珠奶茶";
int price = 16;
addDish(name, price);
check(16, 4);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "仙柠大红袍";
int price = 12;
addDish(name, price);
check(12, 4);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "草莓奶昔";
int price = 8;
addDish(name, price);
check(8, 4);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "菠萝奶昔";
int price = 8;
addDish(name, price);
check(8, 4);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "柠檬水";
int price = 6;
addDish(name, price);
check(6, 4);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "草莓双皮奶";
int price = 8;
addDish(name, price);
check(8, 4);
}
}
}
}
}
}
void oSp() {
while (1) {
if (MouseHit()) {
MOUSEMSG msg1 = GetMouseMsg();
switch (msg1.uMsg)
{
case WM_LBUTTONDOWN: {
if (msg1.x >= 20 && msg1.x <= 150 && msg1.y >= 120 && msg1.y <= 150) {
cleardevice();
frame();
selling();
check(0, 1);
oSelling();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 200 && msg1.y <= 230) {
vDish();
oVdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 280 && msg1.y <= 310) {
mDish();
oMdish();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 360 && msg1.y <= 390) {
drinks();
oDrinks();
}
else if (msg1.x >= 20 && msg1.x <= 100 && msg1.y >= 440 && msg1.y <= 470) {
spDishes();
oSp();
}
else if (msg1.x >= 17 && msg1.x <= 77 && msg1.y >= 580 && msg1.y <= 640) {
print();
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "兰州拉面";
int price = 20;
addDish(name, price);
check(20,5);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "重庆酸辣粉";
int price = 18;
addDish(name, price);
check(18, 5);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 170 && msg1.y <= 205) {
char name[] = "武汉热干面";
int price = 18;
addDish(name, price);
check(18, 5);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "老北京炸酱面";
int price = 18;
addDish(name, price);
check(18, 5);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "北京烤鸭";
int price = 30;
addDish(name, price);
check(30, 5);
}
else if (msg1.x >= 1250 && msg1.x <= 1285 && msg1.y >= 405 && msg1.y <= 440) {
char name[] = "狗不理包子";
int price = 20;
addDish(name, price);
check(20, 5);
}
else if (msg1.x >= 520 && msg1.x <= 555 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "驴肉火烧";
int price = 26;
addDish(name, price);
check(26, 5);
}
else if (msg1.x >= 890 && msg1.x <= 925 && msg1.y >= 640 && msg1.y <= 675) {
char name[] = "平遥牛肉";
int price = 26;
addDish(name, price);
check(26, 5);
}
}
}
}
}
}
void initial() {
initgraph(1283, 680, EW_DBLCLKS | SHOWCONSOLE); /*SHOWCONSOLE*/
loadimage(&img0,"中餐厅登录.jpg",1283,680);
loadimage(&img, "./背景.jpg", 1283, 680);
loadimage(&img1, "./中餐厅.jpg", 250, 130);
loadimage(&img49, "购物车.png",60,60);
loadimage(&img50, "钱.jpg",28,28);
loadimage(&img51,"结算界面背景.jpg",1283,680);
loadimage(&img52,"减.jpg",30,25);
loadimage(&img53,"加.jpg",30,25);
loadimage(&img54,"提交.jpg",69,46);
loadimage(&img55,"返回.jpg",69,46);
loadimage(&img2, "./香菇虾.jpg", 225, 215);
loadimage(&img3, "./加号.jpg", 36, 35);
loadimage(&img4, "./辣子鸭.jpg", 225, 215);
loadimage(&img5, "./龙虾.jpg", 225, 215);
loadimage(&img6, "./烧烤烤肉.jpg", 225, 215);
loadimage(&img7, "./蔬菜杂烩.jpg", 225, 215);
loadimage(&img8, "./寿司.jpg", 225, 215);
loadimage(&img9, "./蒜蓉黄瓜.png", 225, 215);
loadimage(&img10, "./甘蓝藕片.jpg", 225, 215);
loadimage(&img11, "./凉拌土豆丝.jpg", 225, 215);
loadimage(&img12, "./麻辣鸡丝.jpg", 225, 215);
loadimage(&img13, "./蜜豆蛋干.jpg", 225, 215);
loadimage(&img14, "./桃仁木耳.jpg", 225, 215);
loadimage(&img15, "./樱桃沙拉.jpg", 225, 215);
loadimage(&img16, "./糖醋萝卜.jpg", 225, 215);
loadimage(&img17, "./茶树茹牛肚.jpg", 225, 215);
loadimage(&img18, "./京酱肉丝.jpg", 225, 215);
loadimage(&img19, "./川香回锅肉.jpg", 225, 215);
loadimage(&img20, "./鞭花海参.jpg", 225, 215);
loadimage(&img21, "./酥炸黄花鱼.jpg", 225, 215);
loadimage(&img22, "./葱油鲈鱼.jpg", 225, 215);
loadimage(&img23, "./胶东一品锅.jpg", 225, 215);
loadimage(&img24, "./红烧鲤鱼.jpg", 225, 215);
loadimage(&img25, "./冰蓝优酸乳.jpg", 225, 215);
loadimage(&img26, "./火龙锅乳酸菌.jpg", 225, 215);
loadimage(&img27, "./黑糖爆珠奶茶.jpg", 225, 215);
loadimage(&img28, "./仙柠大红袍.jpg", 225, 215);
loadimage(&img29, "./草莓奶昔.jpg", 225, 215);
loadimage(&img30, "./菠萝奶昔.jpg", 225, 215);
loadimage(&img31, "./柠檬水.jpg", 225, 215);
loadimage(&img32, "./草莓双皮奶.jpg", 225, 215);
loadimage(&img33, "./兰州拉面.jpg", 225, 215);
loadimage(&img34, "./重庆酸辣粉.jpg", 225, 215);
loadimage(&img35, "./武汉热干面.jpg", 225, 215);
loadimage(&img36, "./老北京炸酱面.jpg", 225, 215);
loadimage(&img37, "./北京烤鸭.jpg", 225, 215);
loadimage(&img38, "./狗不理包子.jpg", 225, 215);
loadimage(&img39, "./驴肉火烧.jpg", 225, 215);
loadimage(&img40, "./平遥牛肉.jpg", 225, 215);
loadimage(&img41, "./岐山臊子面.jpg", 225, 215);
loadimage(&img42, "./桂花糯米藕.jpg", 225, 215);
loadimage(&img43, "./龙井虾仁.jpg", 225, 215);
loadimage(&img44, "./蟹黄汤包.jpg", 225, 215);
loadimage(&img45, "./狮子头.jpg", 225, 215);
loadimage(&img46, "./九转大肠.jpg", 225, 215);
loadimage(&img47, "./腌笃鲜.jpg", 225, 215);
loadimage(&img48, "./烤羊腿.jpg", 225, 215);
}
void BGM() {//Someone You Loved
if ((mciSendString("open ./SomeoneYouLoved.mp3 alias bgm", 0, 0, 0)) != 0) {
printf("错误!\n");
}
mciSendString("play bgm repeat", 0, 0, 0);//repeat 重复播放
}
void vDish() {
//右边的图片
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img9); putimage(560, 0, &img10); putimage(925, 0, &img11);
putimage(195, 235, &img12); putimage(560, 235, &img13); putimage(925, 235, &img14);
putimage(195, 470, &img15); putimage(560, 470, &img16);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
fillrectangle(420, 470, 560, 685); fillrectangle(785, 470, 930, 685);
settextcolor(BLACK);
outtextxy(430, 60, "蒜蓉黄瓜"); outtextxy(810, 60, "甘蓝藕片"); outtextxy(1180, 60, "土豆丝");
outtextxy(430, 295, "麻辣鸡丝"); outtextxy(810, 295, "蜜豆蛋干"); outtextxy(1180, 295, "桃仁木耳");
outtextxy(430, 530, "樱桃沙拉"); outtextxy(810, 530, "糖醋萝卜");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥20"); outtextxy(810, 120, "¥18"); outtextxy(1180, 120, "¥15");
outtextxy(440, 355, "¥15"); outtextxy(810, 355, "¥20"); outtextxy(1180, 335 + 20, "¥18");
outtextxy(440, 590, "¥20"); outtextxy(810, 590, "¥25");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 405, &img3); putimage(890, 405, &img3); putimage(1250, 170 + 235, &img3);
putimage(520, 640, &img3); putimage(890, 640, &img3);
}
void mDish() {
//右边的图片
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img17); putimage(560, 0, &img18); putimage(925, 0, &img19);
putimage(195, 235, &img20); putimage(560, 235, &img21); putimage(925, 235, &img22);
putimage(195, 470, &img23); putimage(560, 470, &img24);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
fillrectangle(420, 470, 560, 685); fillrectangle(785, 470, 930, 685);
settextcolor(BLACK);
settextstyle(25,0,"楷体");
outtextxy(430, 60, "茶树菇牛肚"); outtextxy(810, 60, "京酱肉丝"); outtextxy(1150, 60, "川香回锅肉");
outtextxy(430, 295, "鞭花海参"); outtextxy(800, 295, "酥炸黄花鱼"); outtextxy(1150, 295, "葱油鲈鱼");
outtextxy(430, 530, "胶东一品锅"); outtextxy(810, 530, "红烧鲤鱼");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥25"); outtextxy(810, 120, "¥25"); outtextxy(1180, 120, "¥20");
outtextxy(440, 355, "¥30"); outtextxy(810, 355, "¥25"); outtextxy(1180, 335 + 20, "¥32");
outtextxy(440, 590, "¥36"); outtextxy(810, 590, "¥26");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 405, &img3); putimage(890, 405, &img3); putimage(1250, 170 + 235, &img3);
putimage(520, 640, &img3); putimage(890, 640, &img3);
}
void drinks() {
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img25); putimage(560, 0, &img26); putimage(925, 0, &img27);
putimage(195, 235, &img28); putimage(560, 235, &img29); putimage(925, 235, &img30);
putimage(195, 470, &img31); putimage(560, 470, &img32);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
fillrectangle(420, 470, 560, 685); fillrectangle(785, 470, 930, 685);
settextcolor(BLACK);
settextstyle(25, 0, "楷体");
outtextxy(430, 60, "冰蓝优酸乳"); outtextxy(810, 60, "火龙果乳酸菌"); outtextxy(1150, 60, "黑糖爆珠奶茶");
outtextxy(430, 295, "仙柠大红袍"); outtextxy(800, 295, "草莓奶昔"); outtextxy(1150, 295, "菠萝奶昔");
outtextxy(430, 530, "柠檬水"); outtextxy(803, 530, "草莓双皮奶");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥12"); outtextxy(810, 120, "¥13"); outtextxy(1180, 120, "¥16");
outtextxy(440, 355, "¥12"); outtextxy(810, 355, "¥8"); outtextxy(1180, 335 + 20, "¥8");
outtextxy(440, 590, "¥6"); outtextxy(810, 590, "¥8");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 405, &img3); putimage(890, 405, &img3); putimage(1250, 170 + 235, &img3);
putimage(520, 640, &img3); putimage(890, 640, &img3);
}
void selling() {
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img2); putimage(560, 0, &img4); putimage(925, 0, &img5);
putimage(195, 235, &img6); putimage(560, 235, &img7); putimage(925, 235, &img8);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
settextcolor(BLACK);
outtextxy(430, 60, "香菇虾"); outtextxy(810, 60, "辣子鸭"); outtextxy(1180, 60, "龙虾");
outtextxy(430, 295, "烧烤烤肉"); outtextxy(810, 295, "蔬菜杂烩"); outtextxy(1180, 295, "寿司");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥45"); outtextxy(810, 120, "¥40"); outtextxy(1180, 120, "¥80");
outtextxy(440, 335 + 20, "¥50"); outtextxy(810, 335 + 20, "¥25"); outtextxy(1180, 335 + 20, "¥30");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 170 + 215 + 20, &img3); putimage(890, 170 + 215 + 20, &img3); putimage(1250, 170 + 235, &img3);
settextstyle(30,0,"楷体");
}
void spDishes() {
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img33); putimage(560, 0, &img34); putimage(925, 0, &img35);
putimage(195, 235, &img36); putimage(560, 235, &img37); putimage(925, 235, &img38);
putimage(195, 470, &img39); putimage(560, 470, &img40);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
fillrectangle(420, 470, 560, 685); fillrectangle(785, 470, 930, 685);
settextcolor(BLACK);
settextstyle(25, 0, "楷体");
outtextxy(430, 60, "兰州拉面"); outtextxy(800, 60, "重庆酸辣粉"); outtextxy(1150, 60, "武汉热干面");
outtextxy(430, 295, "北京炸酱面"); outtextxy(800, 295, "北京烤鸭"); outtextxy(1150, 295, "狗不理包子");
outtextxy(430, 530, "驴肉火烧"); outtextxy(810, 530, "平遥牛肉");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥20"); outtextxy(810, 120, "¥18"); outtextxy(1180, 120, "¥18");
outtextxy(440, 355, "¥18"); outtextxy(810, 355, "¥30"); outtextxy(1180, 335 + 20, "¥20");
outtextxy(440, 590, "¥26"); outtextxy(810, 590, "¥26");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 405, &img3); putimage(890, 405, &img3); putimage(1250, 170 + 235, &img3);
putimage(520, 640, &img3); putimage(890, 640, &img3);
}
void spDishes1() {
putimage(1283 - 254, 680 - 130, &img1);
putimage(195, 0, &img41); putimage(560, 0, &img42); putimage(925, 0, &img43);
putimage(195, 235, &img44); putimage(560, 235, &img45); putimage(925, 235, &img46);
putimage(195, 470, &img47); putimage(560, 470, &img48);
//右边的文字背景
setfillcolor(RGB(228, 226, 218));
fillrectangle(420, 0, 560, 215); fillrectangle(785, 0, 930, 215); fillrectangle(1150, 0, 795 + 370 + 140 - 5, 215);
fillrectangle(420, 235, 560, 450); fillrectangle(785, 235, 930, 450); fillrectangle(1150, 235, 795 + 370 + 140 - 5, 450);
fillrectangle(420, 470, 560, 685); fillrectangle(785, 450, 930, 685);
settextcolor(BLACK);
settextstyle(25, 0, "楷体");
outtextxy(430, 60, "岐山臊子面"); outtextxy(800, 60, "桂花糯米藕"); outtextxy(1150, 60, "龙井虾仁");
outtextxy(430, 295, "蟹黄汤包"); outtextxy(800, 295, "狮子头"); outtextxy(1150, 295, "九转大肠");
outtextxy(430, 530, "腌笃鲜"); outtextxy(810, 530, "烤羊腿");
settextstyle(25, 0, "楷体");
outtextxy(440, 120, "¥18"); outtextxy(810, 120, "¥16"); outtextxy(1180, 120, "¥26");
outtextxy(440, 355, "¥18"); outtextxy(810, 355, "¥35"); outtextxy(1180, 335 + 20, "¥28");
outtextxy(440, 590, "¥26"); outtextxy(810, 590, "¥38");
putimage(520, 170, &img3); putimage(890, 170, &img3); putimage(1250, 170, &img3);
putimage(520, 405, &img3); putimage(890, 405, &img3); putimage(1250, 170 + 235, &img3);
putimage(520, 640, &img3); putimage(890, 640, &img3);
}
void cs() {
POINT pts[] = { {50, 200}, {200, 200}, {200, 50} }; //画一个三角形,给出各个点,依次相连
fillpolygon(pts, 3);
POINT pts1[] = { {1,1,},{2,2},{3,3} };
/*fillpolygon*/
}
|