1、多线程示例
中间需要注意的地方: 1、代码中中文的忌讳,代码中文要么编译不过,要么显示为乱码,如果要显示中文可以在ui文件中修改为中文。 对于代码生成界面的最好只是用英文。 2、类名与数组名同名的忌讳,数组名与类名相同时会混淆导致编译错误,因为数组 []和类构造()使用时无法区分是谁。所以变量名与类名不可以相同。
threaddialog.h
#ifndef THREADDIALOG_H
#define THREADDIALOG_H
#include <QDialog>
#include <QPushButton>
#include "WorkThread.h"
#define MAXSIZE 5
QT_BEGIN_NAMESPACE
namespace Ui { class ThreadDialog; }
QT_END_NAMESPACE
class ThreadDialog : public QDialog
{
Q_OBJECT
public:
ThreadDialog(QWidget *parent = nullptr);
~ThreadDialog();
public slots:
void slotStart();
void slotStop();
private:
Ui::ThreadDialog *ui;
QPushButton *startBtn;
QPushButton *stopBtn;
QPushButton *quitBtn;
WorkThread *workthread[MAXSIZE];
};
#endif
WorkThread.h
#ifndef WorkThread_H
#define WorkThread_H
#include <QThread>
class WorkThread:public QThread
{
public:
WorkThread();
protected:
void run();
};
#endif
main.cpp
#include "threaddialog.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
ThreadDialog w;
w.show();
return a.exec();
}
threaddialog.cpp
#include "threaddialog.h"
#include "ui_threaddialog.h"
#include <QHBoxLayout>
ThreadDialog::ThreadDialog(QWidget *parent)
: QDialog(parent)
, ui(new Ui::ThreadDialog)
{
ui->setupUi(this);
setWindowTitle(tr("Thread"));
startBtn = new QPushButton(tr("Start"));
stopBtn = new QPushButton(tr("Stop"));
quitBtn = new QPushButton(tr("Quit"));
QHBoxLayout *mainLayout = new QHBoxLayout(this);
mainLayout->addWidget(startBtn);
mainLayout->addWidget(stopBtn);
mainLayout->addWidget(quitBtn);
connect(startBtn, SIGNAL(clicked()), this, SLOT(slotStart()) );
connect(stopBtn, SIGNAL(clicked()), this, SLOT(slotStop()) );
connect(quitBtn, SIGNAL(clicked()), this, SLOT(close()) );
}
ThreadDialog::~ThreadDialog()
{
delete ui;
}
void ThreadDialog::slotStart()
{
for (int i = 0; i < MAXSIZE ; i++ ) {
workthread[i] = new WorkThread();
}
for (int i = 0; i < MAXSIZE ; i++ ) {
workthread[i]->start();
}
startBtn->setEnabled(false);
stopBtn->setEnabled(true);
}
void ThreadDialog::slotStop()
{
for (int i = 0; i < MAXSIZE ; i++ ) {
workthread[i]->terminate();
workthread[i]->wait();
}
startBtn->setEnabled(true);
stopBtn->setEnabled(false);
}
WorkThread.cpp
#include "WorkThread.h"
#include <QtDebug>
WorkThread::WorkThread()
{
}
void WorkThread::run()
{
while(true)
{
for(int n = 0; n < 10; n++)
{
qDebug() << n << n << n << n << n << n << n << n ;
}
}
}
测试5个线程,顺序乱序。 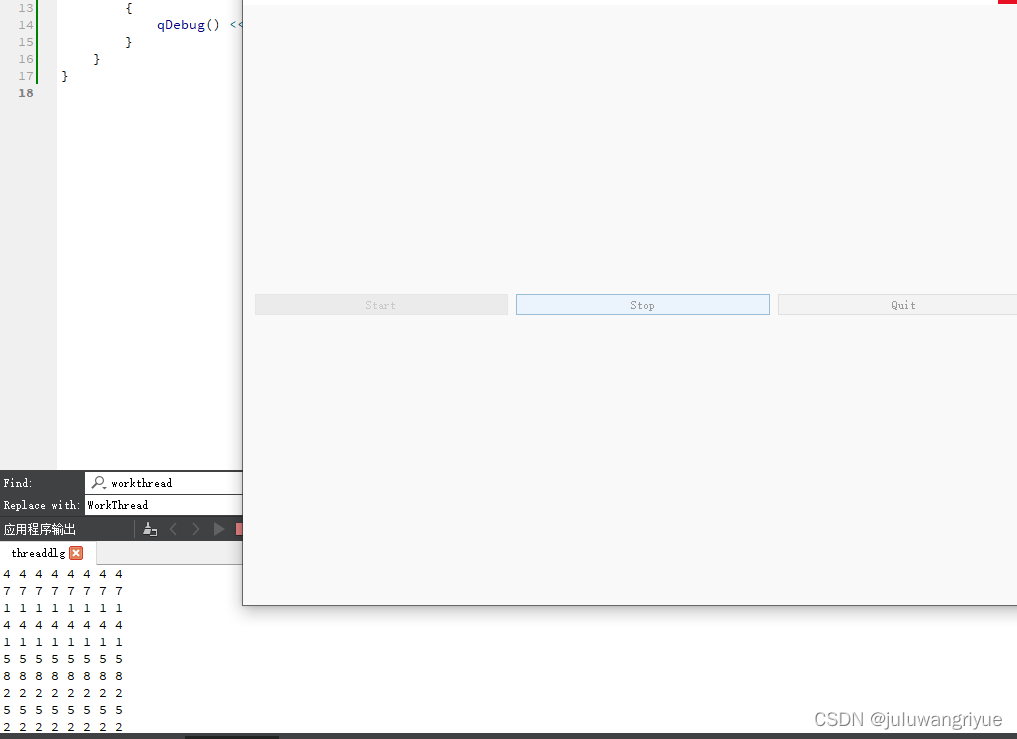
|