方法一:使用QThread类 继承QThread类,覆写run函数,使用run方法启动线程,使用wait方法等待线程退出 例子: mythread.h
#ifndef MYTHREAD_H
#define MYTHREAD_H
#include <QThread>
#include<QDebug>
class myThread : public QThread
{
Q_OBJECT
public:
explicit myThread(int threadId,QObject *parent = 0);
void run();
int threadId;
signals:
public slots:
};
#endif
mythread.cpp
#include "mythread.h"
myThread::myThread(int threadId,QObject *parent) :
threadId(threadId),
QThread(parent)
{
}
void myThread::run(){
for(int i=0;i<5;i++){
QThread::msleep(100);
qDebug()<<"Thread "<<threadId<<" is running,i="<<i;
}
}
main.cpp
#include <QCoreApplication>
#include <mythread.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
myThread mt(1);
myThread mt1(2);
mt.start();
mt1.start();
mt.wait();
mt1.wait();
return a.exec();
}
运行结果: 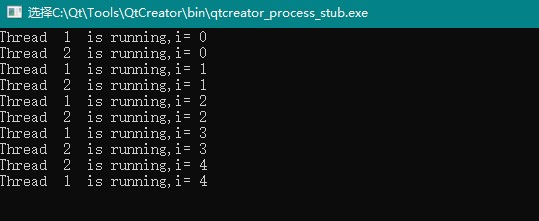
方法二:使用QRunnable 继承QRunnable类,覆写run方法,使用QThreadPool的start方法启动线程,使用waitForDone方法等待线程退出 例子: mythread.h
#ifndef MYTHREAD_H
#define MYTHREAD_H
#include <QRunnable>
#include<QThread>
#include<QDebug>
class myThread : public QRunnable
{
public:
explicit myThread(int threadId,QObject *parent = 0);
void run();
int threadId;
signals:
public slots:
};
#endif
mythread.cpp
#include "mythread.h"
myThread::myThread(int threadId,QObject *parent) :
threadId(threadId)
{
}
void myThread::run(){
for(int i=0;i<5;i++){
QThread::msleep(100);
qDebug()<<"Thread "<<threadId<<" is running,i="<<i;
}
}
main.cpp
#include <QCoreApplication>
#include<mythread.h>
#include<QThreadPool>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
myThread *mt=new myThread(1);
myThread *mt1=new myThread(2);
QThreadPool tp;
tp.start(mt);
tp.start(mt1);
tp.waitForDone();
return a.exec();
}
运行结果: 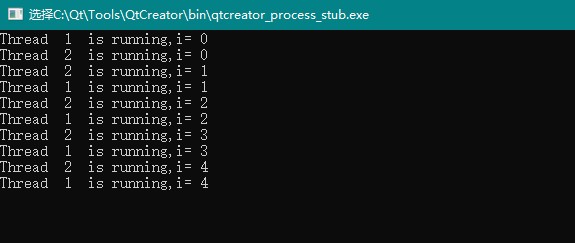
|