写这个案列之前要分析一个问题啊,就是类模板分文件编写问题以及解决
问题: 类模板中的成员函数创建时机是在调用阶段,导致分文件编程时链接不到
第一种解决反式,直接包含源文件(不推荐) #include"xxx(名称).cpp"
第二种解决方式,将.h和.cpp中的内容写到一起,将后缀名改为.hpp文件 #include"xxx(名称).hpp"
学习内容:
案例描述: 实现一个通用的数组类,要求如下: 1.可以对内置数据类型以及自定义数据类型的数据进行存储 2.将数组中的数据存储到堆区 3.构造函数中可以传入数组的容量 4.提供对应的拷贝构造函数以及operator防止浅拷贝问题 5.提供尾插法和尾删法对数组中的数据进行增加和删除 6.可以通过下标的方式访问数组中的元素 7.可以获取数组总当前元素个数和数组的容量 ?
创建 MyArray.hpp
MyArray.hpp代码如下:
#pragma once
#include<iostream>
#include<string>
using namespace std;
template<class T>
class MyArray
{
public:
//有参构造 参数 容量
MyArray(int capacity)
{
this->m_Capacity = capacity;
this->m_Size = 0;
this->pAddress = new T[this->m_Capacity];//将数组中的数据开辟(存储)到堆区
}
//拷贝构造
MyArray(const MyArray& arr)
{
//深拷贝
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
//this->pAddress = arr.pAddress; //浅拷贝 导致堆区的数据重复释放
this->pAddress = new T[arr.m_Capacity];
//将arr中的数据都拷贝过来
for (int i = 0; i < this->m_Size; i++)
{
//元素传递
this->pAddress[i] = arr.pAddress[i];
}
}
//operator= 防止浅拷贝问题(重载=号运算符)
MyArray& operator=(const MyArray& arr)
{
//判断数组是否为空
if (this->pAddress!=NULL)
{
delete[]this->pAddress;
this->pAddress = NULL;
this->m_Capacity = 0;
this->m_Size = 0;
}
//深拷贝
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for (int i = 0; i < this->m_Size; i++)
{
this->pAddress[i] = arr.pAddress[i];
}
return *this;//返回自身
}
//尾插法
void Push_Back(const T & val)
{
//判断容量是否等于大小
if (this->m_Capacity==this->m_Size)
{
return;
}
this->pAddress[this->m_Size] = val;//在数组末尾插入数据
this->m_Size++;//更新数组大小
}
//尾删法
void Pop_back()
{
if (this->m_Size==0)
{
return;
}
this->m_Size--;
}
//通过下标方式访问数组中的元素 arr[0]=100
//函数调用想作为一个左值存在的话 要返回引用才可以 例如 arr[0]=100
//想要100作为arr[0]的左值 必须要用引用 T&
T& operator[](int index)
{
return this->pAddress[index];
}
//返回数组容量
int getCapacity()
{
return this->m_Capacity;
}
//返回数组大小
int getSize()
{
return this->m_Size;
}
//析构函数
~MyArray()
{
if (this->pAddress!=NULL)
{
delete[]this->pAddress;
this->pAddress = NULL;
}
}
private:
T * pAddress;//指针指向堆区开辟的真实数组
int m_Capacity;//数组容量
int m_Size;//数组大小
};
创建 类模板案例-数组类封装.cpp
代码如下:
#define _CRT_SECURE_NO_WARNINGS
#include<iostream>
#include<string>
using namespace std;
#include"MyArray.hpp"
void printIntArray(MyArray <int> & arr1)
{
for (int i = 0; i < arr1.getSize(); i++)
{
cout << arr1[i]<<" ";
}
cout << endl;
}
void test()
{
MyArray <int> arr1(10);
for (int i = 0; i < 5; i++)
{
//利用尾插法向数组插入数据
arr1.Push_Back(i);
}
cout << "arr1的打印输出为:" << endl;
printIntArray(arr1);
cout << "arr1的容量为:" << arr1.getCapacity() << endl;
cout << "arr1的大小为:" << arr1.getSize() << endl;
MyArray<int> arr2(arr1);
cout << "arr2的打印输出:" << endl;
printIntArray(arr2);
//尾删
arr2.Pop_back();
cout << "尾删后:" << endl;
cout << "arr2的容量为:" << arr2.getCapacity() << endl;
cout << "arr2的大小为:" << arr2.getSize() << endl;
//MyArray <int> arr1(arr);//测试拷贝构造函数
//MyArray <int> arr2(100);//测试构造函数
//arr2 = arr1;//测试operator=(等号运算符重载)
}
//测试自定义数据类型
class Person
{
public:
Person(){};//无参构造函数
Person(string name,int age)
{
this->m_Name = name;
this->m_Age = age;
}
string m_Name;
int m_Age;
};
void printPersonArray(MyArray<Person>& arr)
{
cout << endl;
cout << "五虎上将,参见" << endl;
for (int i = 0; i < arr.getSize(); i++)
{
cout << " 姓名: " << arr[i].m_Name << " 年龄: " << arr[i].m_Age << endl;
}
}
void test1()
{
MyArray<Person> arr(10);
Person p1("马超", 20);
Person p2("黄忠", 50);
Person p3("张飞", 40);
Person p4("赵云", 25);
Person p5("关羽", 45);
//将数据插入到数组中
arr.Push_Back(p1);
arr.Push_Back(p2);
arr.Push_Back(p3);
arr.Push_Back(p4);
arr.Push_Back(p5);
printPersonArray(arr);
//容量大小
cout << "arr的容量大小为:" << arr.getCapacity() << endl;
//数组大小
cout << "arr的数组大小为:" << arr.getSize() << endl;
}
int main()
{
test();
test1();
system("pause");
return 0;
}
运行结果:
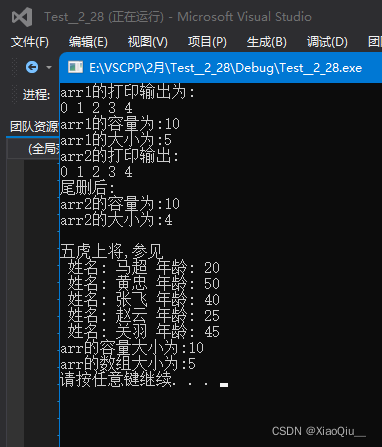
该案例来自黑马程序员,自己对着敲了一遍,感觉挺有用的就分享给大家?!
|