string简单介绍:
string类需要包含头文件string,string类封装了串的属性并提供了一系列允许访问这些属性的函数。 细节: string并非是一个独立的类,而是类模板basic_string的一个特化实例。
构造函数的原型:
sting(); //默认构造函数,建立一个长度为0的串 string(const string& str); //赋值拷贝 string(const char* s); //用指针s所指向的字符串常量初始化string类的对象。 string(const string& str , size_t pos , size_t n); //将对象str中的串从位置pos开始取n个字符,用来初始化string类的对象 string(const char* s,size_t n); //用指针s指向的字符串中的前n个字符初始化string类的对象。 string(size_t n, char c); //将参数c中的字符重复n次,用来初始化string类的对象。
注意:string类接收const char*类型的构造函数,因此字符串常量和用字符数组表示的字符串变量都可以隐含地转换为string对象。
如下代码所示:
#include<iostream>
#include<string>
using namespace std;
void testString1()
{
string s1;
string s2("Hello world!");
string s3(s2);
getline(cin, s1);
cout << s1 << endl;
cout << s2 << endl;
cout << s3 << endl;
string s4(s2, 2, 6);
cout << s4 << endl;
string s5(s2, 2);
cout << s5 << endl;
string s6(s2, 2, 100);
cout << s6 << endl;
string s7("Hello String", 3);
cout << s7 << endl;
string s8(10, 'i');
cout << s8 << endl;
}
int main()
{
testString1();
return 0;
}
运行结果如下:
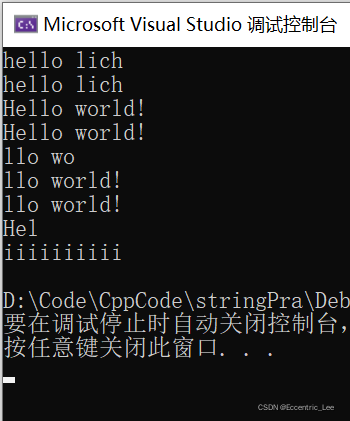
常用的函数:
void testString2()
{
string s1;
getline(cin ,s1);
cout << s1.size() << endl;
cout << s1.length() << endl;
cout << s1.max_size() << endl;
cout << s1 << endl;
cout << s1.capacity() << endl;
s1.clear();
cout << "s1:" << s1 << endl;
cout << s1.capacity() << endl;
}
int main()
{
testString2();
return 0;
}
运行如下: 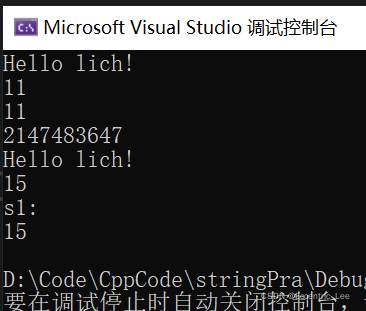
void testString3()
{
string s1("Hello lich");
cout << s1 << endl;
for (size_t i = 0; i < s1.size(); i++)
{
cout << s1.operator[](i) << " ";
}
cout << endl;
for (size_t i = 0; i < s1.size(); i++)
{
cout << s1[i] << " ";
}
cout << endl;
for (size_t i = 0; i < s1.size(); ++i)
{
s1[i] += 1;
}
cout << endl;
cout << s1 << endl;
for (size_t i = 0; i < s1.size(); i++)
{
s1.at(i) -= 1;
}
cout << endl;
cout << s1 << endl;
}
int main()
{
testString3();
return 0;
}
运行结果如下: 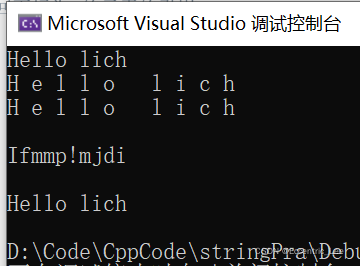
void testString4()
{
string s1;
s1.push_back('h');
s1.append("ello lich ");
cout << s1 << endl;
s1 += ':';
s1 += "studying cpp.";
cout << s1 << endl;
}
int main()
{
testString4();
return 0;
运行结果如下: 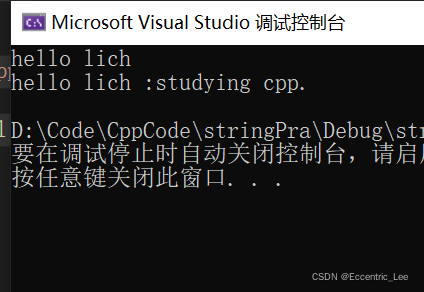
reserve()函数:申请存储字节空间
void test_string3()
{
string s;
s.reserve(1000);
size_t sz = s.capacity();
for (int i = 0; i < 1000; i++)
{
s += 'd';
if (sz != s.capacity())
{
sz = s.capacity();
cout << "capacity change():" << sz << '\n';
}
}
}
int main()
{
string s1("hello lich");
test_string3();
return 0;
}
**resize()函数:**将有效字符的个数该成n个,多出的空间用字符c填充
void test_string4()
{
string s1;
s1.reserve(100);
cout << s1.capacity() << endl;
string s2;
s2.resize(100, 'x');
cout << s2 << endl;
cout << s2.capacity() << endl;
string s3("hello lich");
cout << s3.capacity() << endl;
s3.resize(100);
cout << s3 << endl;
cout << s3.capacity() << endl;
string s5("hello world");
s5.resize(5);
cout << s5 << endl;
cout << s5.size() << endl;
cout << s5.capacity() << endl;
}
int main()
{
string s1("hello lich");
test_string4();
return 0;
}
运行如下: 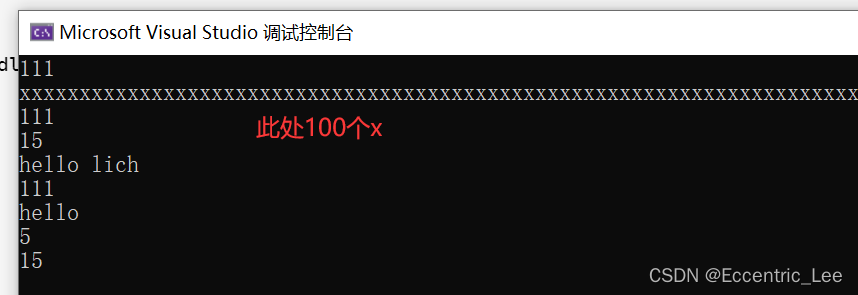 c_str()函数: 返回C格式字符串 我们常用到c_str的形式如:string file(“test.txt”); FILE fout = fopen(file.c_str(), “w”);* 读取文件时,将文件名转化为C格式字符串。
find()函数与substr()函数:
void test_string5()
{
string file("test.cpp.zip");
size_t pos = file.rfind('.');
if (pos != string::npos)
{
string suffix = file.substr(pos);
cout << suffix << endl;
}
}
int main()
{
string s1("hello lich");
test_string5();
return 0;
}
运行如下: 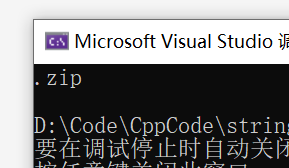
void test_string6()
{
string url("https://editor.csdn.net/md?articleId=123302085");
size_t pos1 = url.find(':');
string protocal = url.substr(0, pos1);
size_t pos2=url.find('/',pos1+3);
string domain = url.substr(pos1 + 3, pos2 - (pos1 + 3));
cout << protocal << endl;
cout << domain << endl;
}
运行结果: 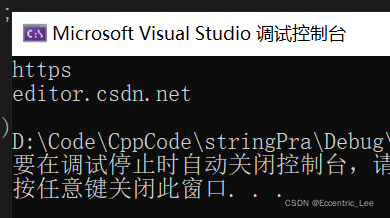 insert()函数:
void test_string7()
{
string s("hello lich");
s += ' ';
s += "!!!!";
cout << endl;
s.insert(0, 2, 'w');
s.insert(s.begin(), 'o');
s.insert(0, "test");
cout << s << endl;
s.insert(4, "****");
cout << s << endl;
}
int main()
{
string s1("hello lich");
test_string7();
return 0;
}
运行结果如下: 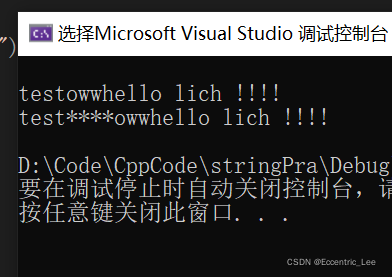 earse()函数:
void test_string8()
{
string s("hello lich");
s.erase(0, 2);
cout << s << endl;
s.erase(s.size() -1, 1);
cout << s << endl;
string s1("hello string");
s1.erase(0, 3);
cout << s1 << endl;
s1.erase(3);
cout << s1 << endl;
}
int main()
{
string s1("hello lich");
test_string8();
return 0;
}
运行结果如下: 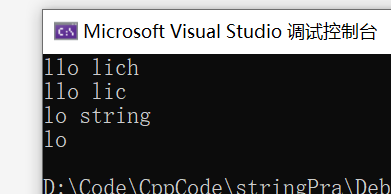
遍历字符串的几种方式:
#include<iostream>
#include<string>
using namespace std;
void test_string1()
{
string s1("hello world");
for (size_t i = 0; i < s1.size(); i++)
{
s1[i] += 1;
}
for (size_t i = 0; i < s1.size(); i++)
{
cout << s1[i] << " ";
}
cout << endl;
string::iterator it = s1.begin();
while (it != s1.end())
{
*it -= 1;
++it;
}
cout << endl;
it = s1.begin();
while (it != s1.end())
{
cout << *it << " ";
it++;
}
cout << endl;
for (auto& e : s1)
{
e += 1;
}
for (auto e : s1)
{
cout << e << " ";
}
cout << endl;
}
int main()
{
test_string1();
return 0;
}
运行代码如下: 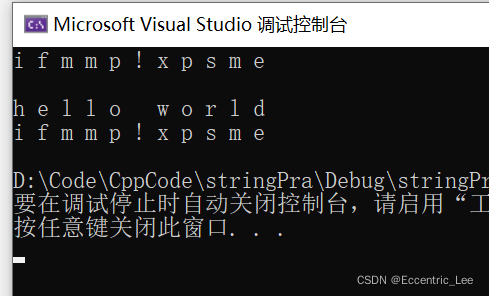
其中迭代器iterator也有两种,分别为:string::iterator 和 string::const_iterator ,前者字符串内容可以修改,后者内容不可以修改。 如下代码所示:
void test_string2(const string& s)
{
auto it = s.begin();
while (it != s.end())
{
cout << *it << " ";
it++;
}
cout << endl;
string::const_reverse_iterator rit = s.rbegin();
while (rit != s.rend())
{
cout << *rit << " ";
rit++;
}
cout << endl;
}
int main()
{
string s1("hello lich");
test_string2(s1);
return 0;
}
运行如下: 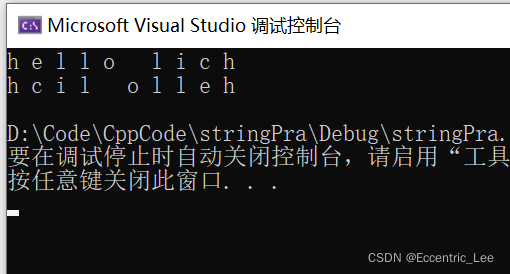 C++找字串:
int frequency(char* substr, char* str)
{
int n = 0, s1 = 0, s2 = 0;
s1 = strlen(str);
s2 = strlen(substr);
while (s1 >= s2)
{
str = strstr(str, substr);
if (str != 0)
{
n++;
str += s2;
}
else
{
break;
}
}
return n;
}
int main()
{
char sub[128], str[1024];
cin.getline(sub, 128);
cin.getline(str, 1024);
int n = frequency(sub, str);
cout << n << endl;
return 0;
}
结果如下: 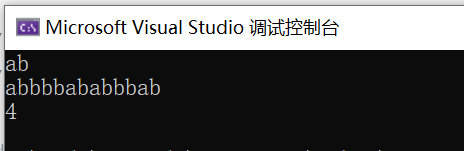 字符串的比较大小: 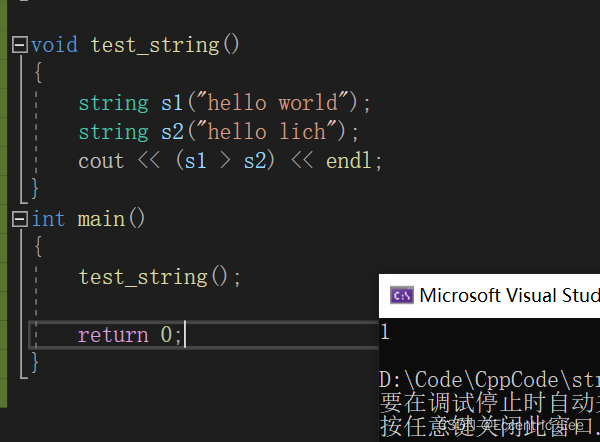 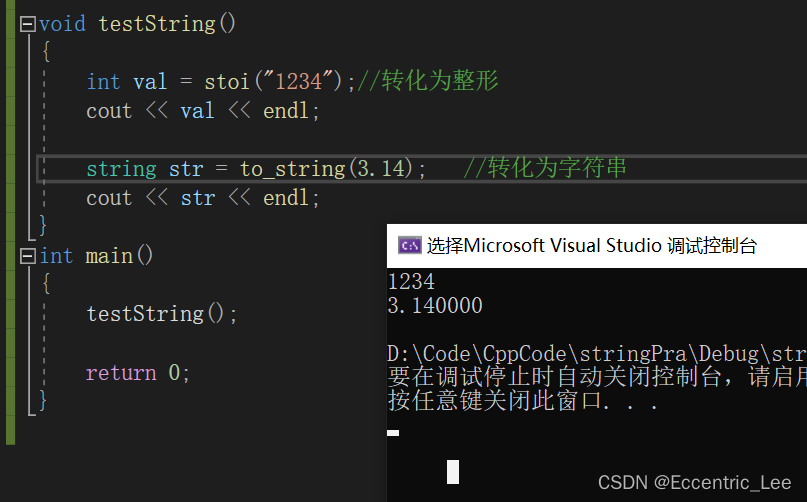
|