QT参数文件
qt寻找参数文件
//头文件:
#include<QFileDialog>
#include<QSettings>
//寻找参数文件
QSettings *IniFile = new QSettings("./Config/Config.ini", QSettings::IniFormat);//参数文件路径
ui->lineEdit->setText(IniFile->value( "control/user").toString());//本机名
ui->lineEdit_2->setText(IniFile->value( "control/bagPath").toString());//获取bag路径
comPath=IniFile->value( "control/comPath").toString();//获取command路径
参数文件写法 命名为:Config.ini 放到qt可执行文件下(若将可执行文件放到桌面,需要将该参数文件置于同路径下)
[control]
#设备名
user=xx
#工控机名
host=nefu
#IP地址
IP=192.168.1.121
# bagBash
# bag路径
bagPath=/home/xx/
# 运行指令文本路径
comPath=/home/xx/command.txt
运行指令文本
取名为:command.txt 放到:home目录下(由参数文件定义)
//***********************************************************************************************************************
// 【rosbash 运行指令集】
//
// 1.指令书写格式:name: xxxx (指令索引名)
// command: xxxx (运行指令)
// 2.以行为单位读取指令,“name”、“command”为关键字
// 3.本文件路径由参数文件定义,且不能含中文
//
//***********************************************************************************************************************
//启动红外相机
name: launch_usb_cam
command: source /home/xx/catkin_ws/devel/setup.bash && roslaunch usb_cam usb_cam-test.launch
//启动雷达
name: launch_velodyne
command: source /home/xx/catkin_ws/devel/setup.bash && roslaunch velodyne_pointcloud VLP16_points.launch
qt读取命令行
新建一个comboBox和一个按钮
//读取命令行
void MainWindow::on_actionxx_triggered()
{
ui->comboBox_2->clear();
nameList.clear();
commandList.clear();
QFile file(comPath);
if (file.open(QIODevice::ReadOnly | QIODevice::Text))
{
bool com=false;
while (!file.atEnd())
{
QByteArray line = file.readLine();
QString str(line);
if(str!= "\n")
{
if(str.startsWith("name"))
{
int index = str.lastIndexOf(":");
QString str2 = str.mid(index+1);
//ui->textEdit->append(str2);
nameList.push_back(str2);
com=true;
}else if(com)
{
com=false;
if(str.startsWith("command"))
{
int index = str.lastIndexOf(":");
QString str2 = str.mid(index+1);
if(str.lastIndexOf("ros")<1)
commandList.push_back("NO Command!");
else
commandList.push_back(str2);
}else
commandList.push_back("NO Command!");
}
}
}
file.close();
for(int i;i<nameList.size();i++)
ui->comboBox_2->addItem(nameList.at(i));
}
}
执行命令函数:
void MainWindow::on_pushButton_8_clicked()
{
if(nameList.empty())
return;
QString str =commandList.at(ui->comboBox_2->currentIndex());
ui->textEdit->append(str);
if(str.lastIndexOf("NO")>0)
return;
QString str2=QString("gnome-terminal -- bash -c '%1 '& \n").arg(str);
char *n;
QByteArray m=str2.toLatin1();
n=m.data();
system(n);
}
界面
运行按钮为:com 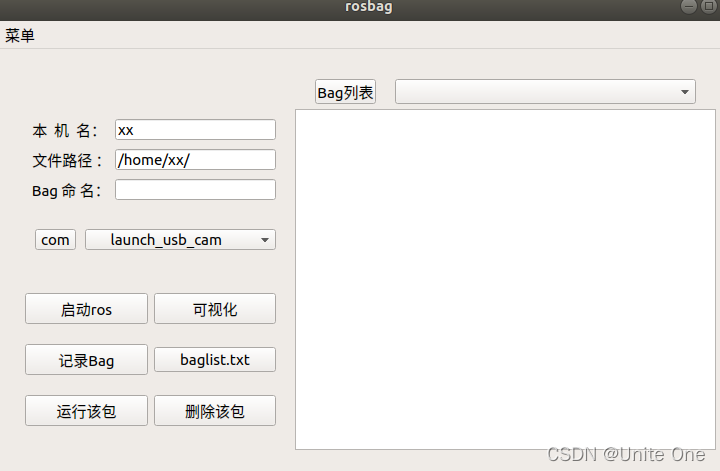
完整程序
mainWindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QProcess>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_pushButton_clicked();
void on_pushButton_2_clicked();
void on_pushButton_3_clicked();
void readoutput();
void readerror();
void on_pushButton_4_clicked();
void on_pushButton_5_clicked();
void on_pushButton_6_clicked();
void on_pushButton_7_clicked();
void on_actionxx_triggered();
void on_action_triggered();
void on_pushButton_8_clicked();
private:
Ui::MainWindow *ui;
QProcess *process;
QString comPath;
QVector<QString> nameList;
QVector<QString> commandList;
};
#endif // MAINWINDOW_H
mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include<QProcess>
#include<QDir>
#include<QFileDialog>
#include<QDebug>
#include<QTime>
#include<QSettings>
#include<QMessageBox>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
this->setWindowTitle("rosbag");
QSettings *IniFile = new QSettings("./Config/Config.ini", QSettings::IniFormat);
ui->lineEdit->setText(IniFile->value( "control/user").toString());//本机名
ui->lineEdit_2->setText(IniFile->value( "control/bagPath").toString());//获取bag路径
comPath=IniFile->value( "control/comPath").toString();//获取command路径
process = new QProcess(this);
process->start("rosbash"); //启动终端(Windows下改为cmd)
process->waitForStarted(); //等待启动完成
connect(process , &QProcess::readyReadStandardOutput, this , &MainWindow::readoutput);
connect(process , &QProcess::readyReadStandardError, this , &MainWindow::readerror);
on_actionxx_triggered();
}
MainWindow::~MainWindow()
{
delete ui;
if(process)
{
process->close();
process->waitForFinished();
}
}
//获取线程输出
void MainWindow::readoutput()
{
ui->textEdit->append(process->readAllStandardOutput().data()); //将输出信息读取到编辑框
}
void MainWindow::readerror()
{
QMessageBox::information(0, "Error", process->readAllStandardError().data()); //弹出信息框提示错误信息
}
//启动ros
void MainWindow::on_pushButton_clicked()
{
QString strget=ui->lineEdit->text();
QString str=QString("/home/%1/rosbash/roscore.sh").arg(strget);
char *n;
QByteArray m=str.toLatin1();
n=m.data();
QProcess::startDetached(n);
}
//添加bag列表
void MainWindow::on_pushButton_2_clicked()
{
ui->comboBox->clear();
//QString strget=ui->lineEdit->text();
QString pathget=ui->lineEdit_2->text();
QString Path=QString("%1").arg(pathget);//指定文件路径
QDir dir(Path);
//QDir dir(QDir::currentPath());//当前文件路径
QString filtername = "*.bag";
QStringList filter;
filter << filtername;
dir.setNameFilters(filter);
QStringList Neuronindex = dir.entryList();
ui->comboBox->addItems(Neuronindex);//把列表加载到comboBox
QString s = QString::number(Neuronindex.size());//txt文件个数
ui->textEdit->append(QString("rosbash $: 共有%1个bag文件").arg(s));
}
//运行该bag文件
void MainWindow::on_pushButton_3_clicked()
{
QString strget=ui->lineEdit->text();
QString pathget=ui->lineEdit_2->text();
QString getbag=ui->comboBox->currentText();
QString str=QString("/home/%1/rosbash/bagplay.sh %2 %3 \n").arg(strget).arg(pathget).arg(getbag);
char *n;
QByteArray m=str.toLatin1();
ui->textEdit->append(QString("rosbash $: %1").arg(str));
n=m.data();
//process->write(n); //向终端写入命令,注意尾部的“\n”不可省略
system(n);
}
//删除该bag文件
void MainWindow::on_pushButton_4_clicked()
{
QString strget=ui->lineEdit->text();
QString pathget=ui->lineEdit_2->text();
QString getbag=ui->comboBox->currentText();
QString path =QString("%1/%2").arg(pathget).arg(getbag);//指定文件路径
QFile fileTemp(path);
QMessageBox msg(this);//对话框设置父组件
msg.setWindowTitle("Prompt");//对话框标题
msg.setText("Confirm the delete action ?");//对话框提示文本
msg.setIcon(QMessageBox::Question);//设置图标类型Information
msg.setStandardButtons(QMessageBox::Yes | QMessageBox::No);//对话框上包含的按钮
if(msg.exec() == QMessageBox::Yes)//模态调用
{
fileTemp.remove();//删除
ui->textEdit->append(QString("rosbash $: 成功删除%1文件").arg(path));
on_pushButton_2_clicked();
}
}
//记录ros包
void MainWindow::on_pushButton_5_clicked()
{
QString nameget=ui->lineEdit_3->text();
QDate D;
D=QDate::currentDate();
QTime T;
T=QTime::currentTime();
QString bagname =QString("%1%2-%3-%4-%5-%6-%7.bag").arg(nameget).arg(D.year()).arg(D.month()).arg(D.day()).arg(T.hour()).arg(T.minute()).arg(T.second());//指定文件路径
qDebug() << bagname;
ui->textEdit->append(QString("rosbash $: 记录的包将被命名为:%1").arg(bagname));
QString strget=ui->lineEdit->text();
QString str4=QString("gnome-terminal -- bash -c '/home/%1/rosbash/bagrecord.sh %2'& \n").arg(strget).arg(bagname);
ui->textEdit->append(QString("rosbash $: /home/%1/rosbash/bagrecord.sh %2").arg(strget).arg(bagname));
char *n;
QByteArray m=str4.toLatin1();
n=m.data();
system(n);
//process->write(n);
}
void MainWindow::on_pushButton_6_clicked()
{
QString strget=ui->lineEdit->text();
QString str4=QString("gnome-terminal -- bash -c '/home/%1/rosbash/view.sh'& \n").arg(strget);
ui->textEdit->append(QString("rosbash $: /home/%1/rosbash/view.sh").arg(strget));
char *n;
QByteArray m=str4.toLatin1();
n=m.data();
system(n);
}
//获取baglist.txt
void MainWindow::on_pushButton_7_clicked()
{
QString strget=ui->lineEdit->text();
QString pathget=ui->lineEdit_2->text();
QString str4=QString("gnome-terminal -- bash -c '/home/%1/rosbash/baglist.sh %2'& \n").arg(strget).arg(pathget);
ui->textEdit->append(QString("rosbash $: /home/%1/rosbash/baglist.sh %2").arg(strget).arg(pathget));
char *n;
QByteArray m=str4.toLatin1();
n=m.data();
system(n);
}
//读取命令行
void MainWindow::on_actionxx_triggered()
{
ui->comboBox_2->clear();
nameList.clear();
commandList.clear();
QFile file(comPath);
if (file.open(QIODevice::ReadOnly | QIODevice::Text))
{
bool com=false;
while (!file.atEnd())
{
QByteArray line = file.readLine();
QString str(line);
if(str!= "\n")
{
if(str.startsWith("name"))
{
int index = str.lastIndexOf(":");
QString str2 = str.mid(index+1);
//ui->textEdit->append(str2);
nameList.push_back(str2);
com=true;
}else if(com)
{
com=false;
if(str.startsWith("command"))
{
int index = str.lastIndexOf(":");
QString str2 = str.mid(index+1);
if(str.lastIndexOf("ros")<1)
commandList.push_back("NO Command!");
else
commandList.push_back(str2);
}else
commandList.push_back("NO Command!");
}
}
}
file.close();
for(int i;i<nameList.size();i++)
ui->comboBox_2->addItem(nameList.at(i));
}
}
void MainWindow::on_pushButton_8_clicked()
{
if(nameList.empty())
return;
QString str =commandList.at(ui->comboBox_2->currentIndex());
ui->textEdit->append(str);
if(str.lastIndexOf("NO")>0)
return;
QString str2=QString("gnome-terminal -- bash -c '%1 '& \n").arg(str);
char *n;
QByteArray m=str2.toLatin1();
n=m.data();
system(n);
}
// 关闭所有终端
void MainWindow::on_action_triggered()
{
system("gnome-terminal -- bash -c 'killall -9 bash'&");
ui->textEdit->append("rosbash $: 关闭所有终端...");
}
main.cpp
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
|