来源:C语言实现简单的飞机大战_Cielfire-CSDN博客_c语言飞机大战代码
运行效果:
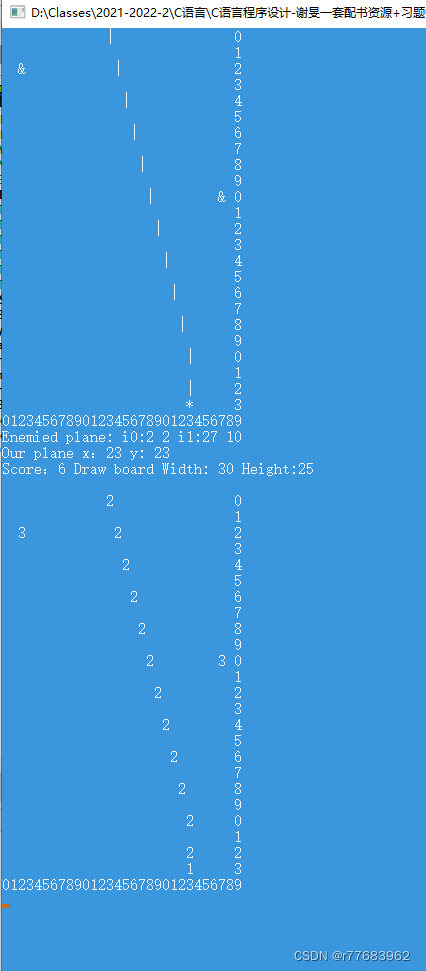
源代码:
#include <stdio.h>
#include <windows.h>
#include <conio.h>
#define SCREEN_HEIGHT 10 //定义边界
#define SCREEN_WIDTH 10
#define ENEMY_COUNT 2 //敌机数目
typedef struct
{
unsigned int x;
unsigned int y;
}PLANE;
typedef enum
{
CELL_TYPE_NOTHING = 0,
CELL_TYPE_OUR_PLANE,
CELL_TYPE_OUR_BULLET,
CELL_TYPE_ENEMY_PLANE,
CELL_TYPE_BORDER,
CELL_TYPE_MAX
}CELL_TYPE;
//得分
unsigned int GameScore;
//定义元素,0为空格,1为飞机,2为子弹,3为敌机,4为右下边界
unsigned int DrawBoard[SCREEN_HEIGHT][SCREEN_WIDTH]={0};
//敌机坐标
PLANE EnemyPlanes[ENEMY_COUNT] = {0};
PLANE OurPlane = {0};
void CursorHide() //隐藏光标
{
// 光标百分比厚度(1~100),是否可见
CONSOLE_CURSOR_INFO CursorInfo = {1, 0};
//c++中SetConsoleCursorInfo是用来设置指定的控制台光标的大小和可见性。
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &CursorInfo);
}
//数据初始化
void DataInitial()
{
unsigned int i = 0;
//初始化飞机位置(从0开始记数)
OurPlane.x = SCREEN_WIDTH / 2;
OurPlane.y = SCREEN_HEIGHT - 2;
DrawBoard[OurPlane.y][OurPlane.x] = CELL_TYPE_OUR_PLANE;
for (i = 0; i < ENEMY_COUNT; i++)
{
EnemyPlanes[i].x = rand() % (SCREEN_WIDTH - 1);
//初始化敌机位置
EnemyPlanes[i].y = rand() % 2;
DrawBoard[EnemyPlanes[i].y][EnemyPlanes[i].x] = CELL_TYPE_ENEMY_PLANE;
}
for(i = 0; i <= SCREEN_HEIGHT - 1; i++)
{
DrawBoard[i][SCREEN_WIDTH - 1] = '0' + i % 10;
}
for(i = 0; i <= SCREEN_WIDTH - 1; i++)
{
DrawBoard[SCREEN_HEIGHT - 1][i] = '0' + i % 10;
}
//得分初始化
GameScore = 0;
CursorHide();
}
void CursorPositionMoveToZero(int x,int y) //光标移动到(x,y)位置
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
//通过修改pos.X和pos.Y的值就可以实现光标的位置控制。
SetConsoleCursorPosition(handle,pos);
}
void DrawBoardUpdate() //显示界面
{
unsigned int y, x;
char cell;
CursorPositionMoveToZero(0,0);
for (y = 0; y < SCREEN_HEIGHT; y++)
{
for (x = 0; x < SCREEN_WIDTH; x++)
{
switch (DrawBoard[y][x])
{
case CELL_TYPE_NOTHING: cell = ' '; break;
case CELL_TYPE_OUR_PLANE: cell = '*'; break;
case CELL_TYPE_OUR_BULLET: cell = '|'; break;
case CELL_TYPE_ENEMY_PLANE: cell = '&'; break;
case CELL_TYPE_BORDER: cell = '#'; break;
default: ;
}
if (DrawBoard[y][x] >= '0' && DrawBoard[y][x] <= '9')
cell = DrawBoard[y][x];
printf("%c", cell);
}
printf("\n");
}
printf("Enemied plane: ");
for(unsigned int i = 0; i < ENEMY_COUNT; i++)
{
printf("i%d:%d %d ", i, EnemyPlanes[i].x, EnemyPlanes[i].y);
}
putchar('\n');
printf("Our plane x:%d y: %d\n", OurPlane.x, OurPlane.y);
printf("Score:%d Draw board Width: %d Height:%d\n", GameScore, SCREEN_WIDTH,SCREEN_HEIGHT );
putchar('\n');
for (y = 0; y < SCREEN_HEIGHT; y++)
{
for (x = 0; x < SCREEN_WIDTH; x++)
{
if (DrawBoard[y][x] == 0)
{
printf(" ");
}
else
{
if (DrawBoard[y][x] >= '0')
printf("%d", DrawBoard[y][x] - '0');
else
printf("%d", DrawBoard[y][x]);
}
}
putchar('\n');
}
}
void UpdatedDrawboardWithoutInput() //无需用户输入的更新
{
unsigned int y, x, EnemyID;
for (y = 0; y < SCREEN_HEIGHT; y++)
{
for (x = 0; x < SCREEN_WIDTH; x++)
{
//如果是我们飞机发出的子弹
if(DrawBoard[y][x] == CELL_TYPE_OUR_BULLET)
{
for (EnemyID = 0; EnemyID < ENEMY_COUNT; EnemyID++)
{
//击中敌机
if(y == EnemyPlanes[EnemyID].y + 1 && x == EnemyPlanes[EnemyID].x)
{
GameScore++;
//生成新的敌机
DrawBoard[y][x] = CELL_TYPE_NOTHING;
EnemyPlanes[EnemyID].x = rand() % (SCREEN_WIDTH - 1);
EnemyPlanes[EnemyID].y = rand() % 2;
DrawBoard[EnemyPlanes[EnemyID].y][EnemyPlanes[EnemyID].x] = CELL_TYPE_ENEMY_PLANE;
}
}
DrawBoard[y][x] = CELL_TYPE_NOTHING;
//子弹自动上升
if(y > 0)
DrawBoard[y - 1][x] = CELL_TYPE_OUR_BULLET;
}
}
}
for (EnemyID = 0; EnemyID < ENEMY_COUNT; EnemyID++)
{
//下落超过屏幕高度,生成新的敌机
if(EnemyPlanes[EnemyID].y >= SCREEN_HEIGHT - 2)
{
DrawBoard[EnemyPlanes[EnemyID].y][EnemyPlanes[EnemyID].x] = CELL_TYPE_NOTHING;
EnemyPlanes[EnemyID].x = rand() % (SCREEN_WIDTH - 1);
EnemyPlanes[EnemyID].y = rand() % 2;
DrawBoard[EnemyPlanes[EnemyID].y][EnemyPlanes[EnemyID].x] = CELL_TYPE_ENEMY_PLANE;
}
}
//敌机自动下落
for (EnemyID = 0; EnemyID < ENEMY_COUNT; EnemyID++)
{
DrawBoard[EnemyPlanes[EnemyID].y][EnemyPlanes[EnemyID].x] = CELL_TYPE_NOTHING;
EnemyPlanes[EnemyID].y++;
DrawBoard[EnemyPlanes[EnemyID].y][EnemyPlanes[EnemyID].x] = CELL_TYPE_ENEMY_PLANE;
}
for (y = 0; y < SCREEN_HEIGHT; y++)
{
for (x = 0; x < SCREEN_WIDTH; x++)
{
//如果是我们飞机发出的子弹
if(DrawBoard[y][x] == CELL_TYPE_OUR_BULLET)
{
DrawBoard[y][x] = CELL_TYPE_NOTHING;
//子弹自动上升
if(y > 0)
DrawBoard[y - 1][x] = CELL_TYPE_OUR_BULLET;
}
}
}
for (EnemyID = 0; EnemyID < ENEMY_COUNT; EnemyID++)
{
if((EnemyPlanes[EnemyID].x == OurPlane.x) && (EnemyPlanes[EnemyID].y == OurPlane.y - 1)) //飞机撞毁
{
printf("Game Over! EnemyPlanes id:%d x: %d y:%d\n", EnemyID, EnemyPlanes[EnemyID].x, EnemyPlanes[EnemyID].y);
//exit(0);
}
}
//飞机自动发射子弹
DrawBoard[OurPlane.y - 1][OurPlane.x] = CELL_TYPE_OUR_BULLET;
}
void UpdatedDrawboardWithInput() //需用户输入的更新
{
// 检查当前是否有键盘输入,若有则返回一个非0值,否则返回0。
if(!kbhit())
return ;
char KeyboardInput = getch();
if(KeyboardInput == 's' && OurPlane.x < SCREEN_WIDTH - 2)
{
DrawBoard[OurPlane.y][OurPlane.x] = CELL_TYPE_NOTHING;
OurPlane.x++;
if (OurPlane.x > SCREEN_WIDTH - 2)
OurPlane.x = SCREEN_WIDTH - 2;
DrawBoard[OurPlane.y][OurPlane.x] = CELL_TYPE_OUR_PLANE;
}
else if(KeyboardInput == 'a' && OurPlane.x > 0)
{
DrawBoard[OurPlane.y][OurPlane.x] = CELL_TYPE_NOTHING;
OurPlane.x--;
if (OurPlane.x >= SCREEN_WIDTH - 2)
OurPlane.x = 0;
DrawBoard[OurPlane.y][OurPlane.x] = CELL_TYPE_OUR_PLANE;
}
else if(KeyboardInput == ' ')
{
DrawBoard[OurPlane.y - 1][OurPlane.x] = CELL_TYPE_OUR_BULLET;
}
}
int main()
{
DataInitial();
/* 第一背景 第二个则为前景, 每个数字可以为以下任何值之一
0 = 黑色 8 = 灰色
1 = 蓝色 9 = 淡蓝色
2 = 绿色 A = 淡绿色
3 = 湖蓝色 B = 淡浅绿色
4 = 红色 C = 淡红色
5 = 紫色 D = 淡紫色
6 = 黄色 E = 淡黄色
7 = 白色 F = 亮白色
*/
//在C语言中,system()函数用于执行shell命令,也就是向dos发送一条指令;
system("color 3f");
while(1)
{
//显示界面
DrawBoardUpdate();
//需用户输入的更新
UpdatedDrawboardWithInput();
//无需用户输入的更新
UpdatedDrawboardWithoutInput();
Sleep(200);
}
}
|