最近几天在搞关于C++输出html文件。在GitHub上找到了Google开源的ctemplete库。前几天编译好文件(怎么在window上编译参考上一篇)。今天看着例子,自己写了一个demo,其中有自己的感悟,写文记录下。
思路:其实ctemplete的思想其实就是替换模板中的变量,C++要想导出一个html文档首先得有一个html模板文件。所以你想导处什么格式的文件,先写关于模板文件。在模板文件中添加变量使用C++替换其中的变量即可。
至于怎么引用库百度一下即可,我这里使用的是引用动态库。
先写个demo
html模板:
<html>
<head>
<title>ctemplateDemo</title>
</head>
<body>
{{table1_name}}
<table>
<tr>
<th>ID</th>
<th>name</th>
<th>sax</th>
</tr>
<tbody>
{{#TABLE1}}
<tr>
<th>ASD:{{field1}}</th>
<th>{{field2}}</th>
<th>{{field3}}</th>
</tr>
{{/TABLE1}}
</tbody>
</table>
</body>
</html>
C++ 代码:
// testHTML.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include <stdio.h>
#include <string>
#include <fstream>
#include <iostream>
#include <ctemplate/template.h>
int write_string_to_file_append(const std::string & file_string, const std::string str)
{
std::ofstream OsWrite(file_string, std::ofstream::app);
OsWrite << str;
OsWrite << std::endl;
OsWrite.close();
return 0;
}
int main()
{
ctemplate::TemplateDictionary dict("aa");
dict.SetValue("table1_name", "qwe");
for (int i = 0; i<4; ++i)
{
ctemplate::TemplateDictionary* table1_dict;
table1_dict = dict.AddSectionDictionary("TABLE1");
table1_dict->SetValue("field1", "1");
table1_dict->SetValue("field2", "2");
table1_dict->SetValue("field3", "3");
// 这里有点类似于printf
//table1_dict->SetFormattedValue("field3", "%d", i);
}
std::string output;
ctemplate::Template* tpl;
tpl = ctemplate::Template::GetTemplate("example1.html", ctemplate::DO_NOT_STRIP);
tpl->Expand(&output, &dict);
printf("%s\n", output.c_str());
write_string_to_file_append(std::string("file.html"), output);
return 0;
}
结果:最终输出的file.html
<html>
<head>
<title>ctemplateDemo</title>
</head>
<body>
qwe
<table>
<tr>
<th>ID</th>
<th>name</th>
<th>sax</th>
</tr>
<tbody>
<tr>
<th>ASD:1</th>
<th>2</th>
<th>3</th>
</tr>
<tr>
<th>ASD:1</th>
<th>2</th>
<th>3</th>
</tr>
<tr>
<th>ASD:1</th>
<th>2</th>
<th>3</th>
</tr>
<tr>
<th>ASD:1</th>
<th>2</th>
<th>3</th>
</tr>
</tbody>
</table>
</body>
</html>
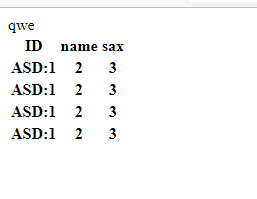
其中用到的核心就是变量替换和循环:
?
dict.SetValue("table1_name", "qwe");
这行代码就是变量替换,非常的简单。
循环就需要注意一点,需要在模板中设置循环体。
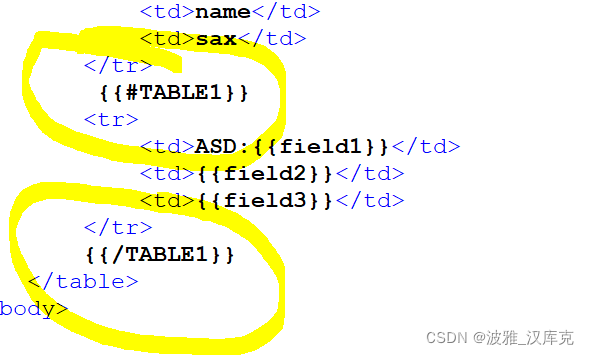
?在模板中
{{#TABLE1}}和{{/TABLE1}}之间的就是循环体。C++对应的代码就是
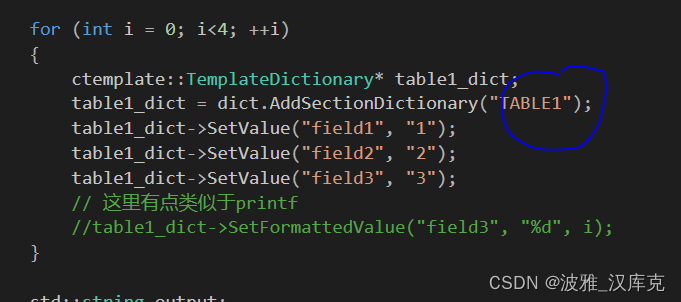
?一定要有这行,
table1_dict = dict.AddSectionDictionary("TABLE1");
下面的就是变量替换了。这个在插入表格中是非常好用的。
|