void * memcpy ( void * destination, const void * source, size_t num );
目录
使用
模拟实现
注意事项?
复制内存块,将?num?字节值从源指向的位置直接复制到目标所指向的内存块。
(strcpy只能针对字符串)
使用
#include<stdlib.h>
#include<stdio.h>
int main()
{
int arr[] = { 1,2,3,4,5,6,7,8,9,10 };
int str[10] = { 0 };
memcpy(str, arr, 5*sizeof(arr[0]));
int i = 0;
for (i = 0; i < 5; i++)
{
printf("%d ", str[i]);
}
return 0;
}
参数
目的地:指向要在其中复制内容的目标数组的指针,以类型转换为 void* 类型的指针。
源:指向要复制的数据源的指针,类型转换为 const void* 类型的指针。
数字:要复制的字节数。? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? size_t是无符号整数类型
返回值:返回到目的地。
模拟实现

?我么可以用viod*的指针来接受参数,src是源,并不需要被修改加上const确保。
char* my_memcpy(void* dest, const void* src, size_t num)
{
}
接下来便可以将dest与src强转为char*类型的数据,根据num个字节数一次修改。
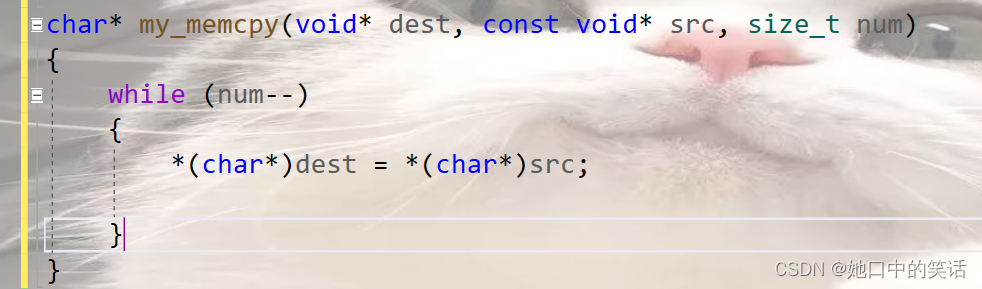
?值得注意的是将dest与src强转只是临时的,用的时候强制转换,其他时候还是void*的指针。所以并不能用dest++,src++。而需要用
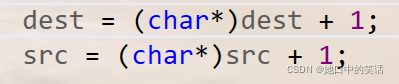
void* 可以接受任意指针类型。
#include<assert.h>
#include<stdlib.h>
#include<stdio.h>
char* my_memcpy(void* dest, const void* src, size_t num)
{
assert(dest && src);
void* ret = dest;
while (num--)
{
*(char*)dest = *(char*)src;
dest = (char*)dest + 1;
src = (char*)src + 1;
}
return ret;
}
int main()
{
int arr[] = { 1,2,3,4,5,6,7,8,9,10 };
int str[10] = { 0 };
my_memcpy(str, arr, 5*sizeof(arr[0]));
int i = 0;
for (i = 0; i < 5; i++)
{
printf("%d ", str[i]);
}
return 0;
}
最后得到
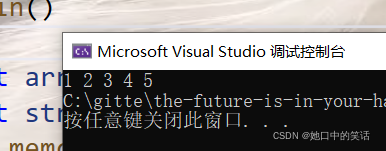
注意事项?
需要注意的是对于重叠的内存块,memmove?是一种更安全的方法。
|