C++ 复杂在于:
- 太多的能够放对象的地方:对象可以放在 堆栈、堆、全局数据区
- 太多可以访问对象的方式:直接掌握对象(变量内放对象)、通过指针访问对象、引用去访问对象
3*3 共 9种组合。
Declaring references
参数是调用函数时候给的。成员变量是构造对象的时候初始化。
references
Rules of references 引用规则
-
references must be initialized when defined -
initialization establishes a binding
-
in declaration
int x = 3;
int& y = x;
const int&z = x;//类似于指针,通过 z 不能修改x, z是x的别名
-
as a function argument
void f(int& x);
f(y); //initialized when function is called
-
bindings don't change at run time, unlike pointers -
assignment changes the object referred-to
int& y = x;
y = 12;//changes value of x
-
the target of a reference must have a location!
void func(int &);
func(i*3); //warning or error,i*3 有结果但是没有名字,不可以
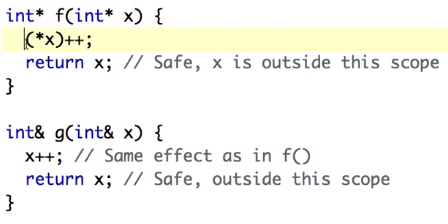
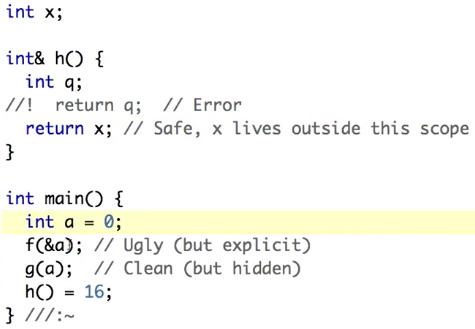
一个函数的返回结果是引用,那么该函数可以做左值。 x 为 16。
一定要去看 g(x)的原型是什么,可能把a 给改了。
int g(int x){}
g(a)不知道调用哪个函数。不能重载。
pointers vs. references
- references
- can't be null
- are dependent on an existing variable, they are an alias(别名) for an variable
- can't change to a new "address" location
- pointers
- can be set to null
- pointer is independent of existing objects
- can be change to point to a different address
引用是const指针实现的,为了让代码简洁。
int* f(int* x){
(*x)++;
return x; //safe,x is outside this scope
}
int& g(int& x){
x++; //same effect as in f()
return x; //safe,outside this scope
}
C++有三种方式放对象,有三种方式访问对象;Java只有一个地方可以放对象,所有对象都放在堆里面,只有一种方式访问对象,就是通过“指针”,因为它只能通过指针访问对象,因此他可以把那个“*”去掉,然后规定说这不叫指针这叫引用,这其实和C++的引用是不一样的,更像是C++的指针,因为引用是不能做引用之间的赋值的,实际上是指针,区别在于外形上没有那个星号,不能做运算(C的指针可以做计算)
references实际上是一种指针,一种const的指针
restrictions
-
no references to references -
no pointers to references int& * p; //illegal, *p是引用。引用的地址是无法取到的
离变量最近的决定了p的基本类型。
引用不是实体,所以没有引用的数组。
|