最终效果展示
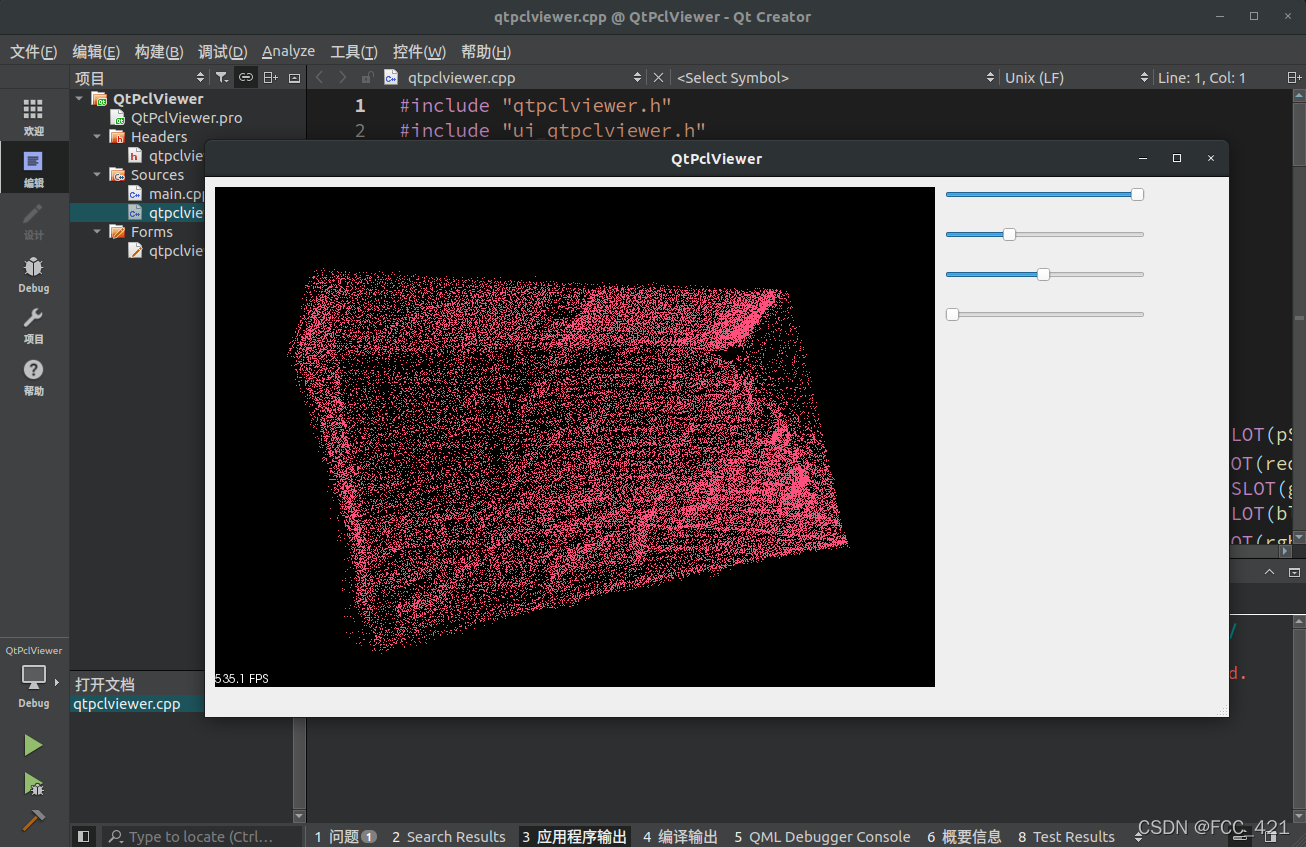
本人环境:Ubuntu18.04 / Qt 5.14.2 / PCL 1.8
下面介绍具体原理和实现步骤。
原理
其实核心就是这一行代码 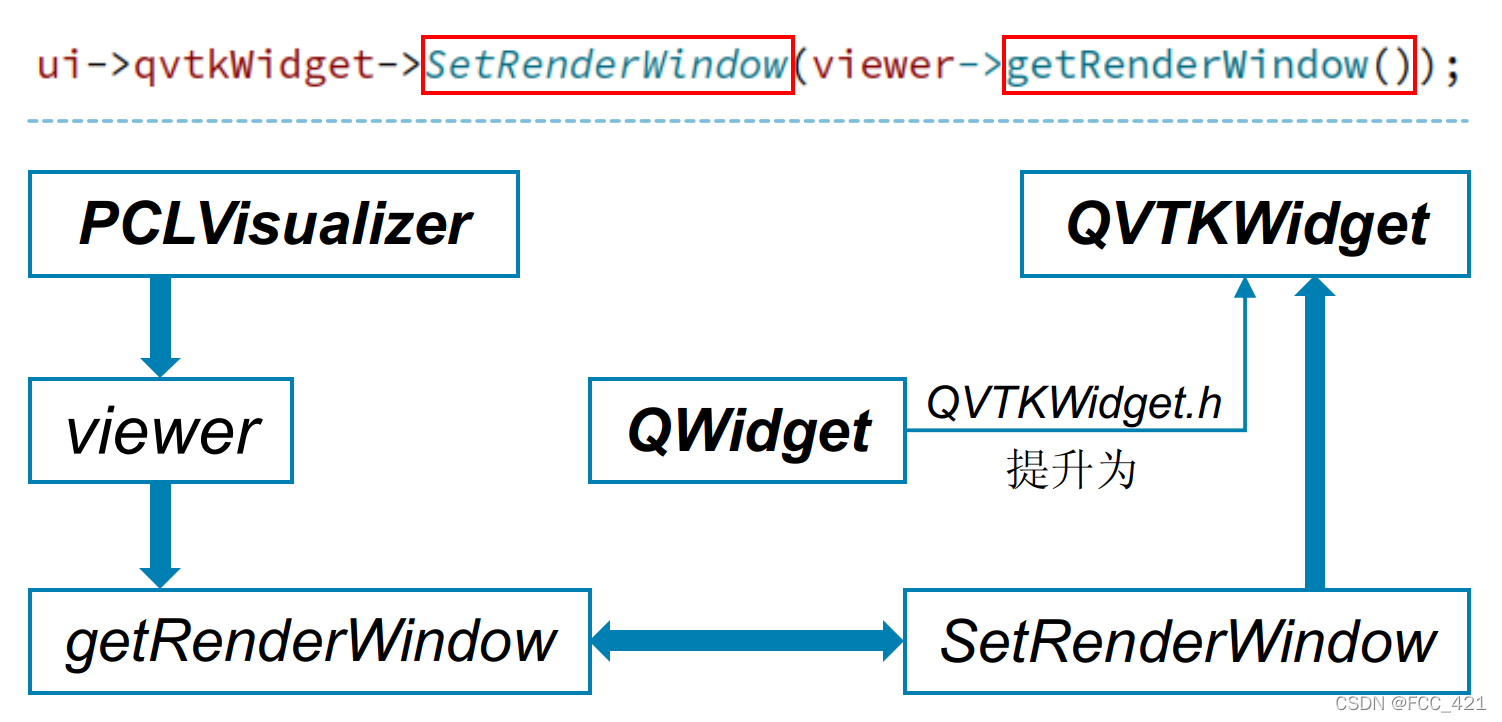 将数据从pclviewer 传输到 QVTKWidget。
具体实现
1、.ui 设计
插入一个QWidget,然后右键QWidget => 提升为 => QVTKWidget (注意QVTKWidget.h区分大小写!!) 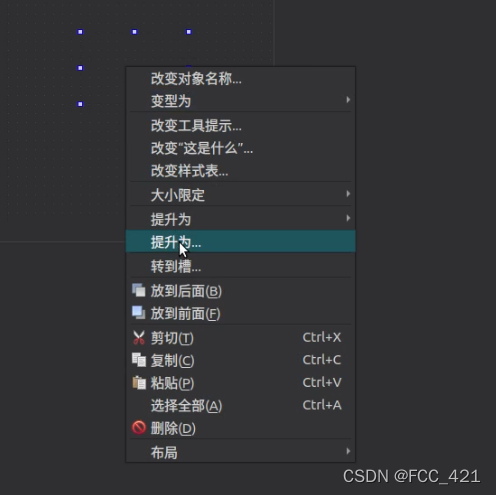 再新建四个horizontalSlider,分别命名为horizontalSlider_Red,horizontalSlider_Green,horizontalSlider_Blue,horizontalSlider_Size。用于控制点云的颜色和大小。
2、代码
.pro
QT += core gui
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
CONFIG += c++11
# 主要需要修改的部分在下方,注意库的地址!
# -------------------------------------------------------
INCLUDEPATH += /usr/include/eigen3 #注意路径可能不一样
INCLUDEPATH += /usr/include/vtk-6.3
LIBS += /usr/lib/x86_64-linux-gnu/libvtk*.so #注意路径可能不一样
INCLUDEPATH += /usr/include/boost #注意路径可能不一样
LIBS += /usr/lib/x86_64-linux-gnu/libboost_*.so
INCLUDEPATH += /usr/include/pcl-1.8 #注意路径可能不一样
LIBS += /usr/lib/x86_64-linux-gnu/libpcl_*.so
# -------------------------------------------------------
DEFINES += QT_DEPRECATED_WARNINGS
SOURCES += \
main.cpp \
qtpclviewer.cpp
HEADERS += \
qtpclviewer.h
FORMS += \
qtpclviewer.ui
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
qtpclviewer.h
#ifndef QTPCLVIEWER_H
#define QTPCLVIEWER_H
#include <QMainWindow>
#include "ui_qtpclviewer.h"
#ifndef INITIAL_OPENGL
#define INITIAL_OPENGL
#include <vtkAutoInit.h>
VTK_MODULE_INIT(vtkRenderingOpenGL)
VTK_MODULE_INIT(vtkInteractionStyle)
#endif
#include <QFileDialog>
#include <vtkRenderWindow.h>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/visualization/pcl_visualizer.h>
class QtPclViewer : public QMainWindow
{
Q_OBJECT
public:
QtPclViewer(QWidget *parent = nullptr);
~QtPclViewer();
private:
Ui::QtPclViewer *ui;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud;
boost::shared_ptr<pcl::visualization::PCLVisualizer> viewer;
void initialVtkWidget();
protected:
unsigned red;
unsigned green;
unsigned blue;
double size;
private slots :
void onOpen();
void rgbSliderReleased( );
void pSliderValueChangeed(int value);
void redSliderValueChangeed(int value);
void greenSliderValueChangeed(int value);
void blueSliderValueChangeed(int value);
};
#endif
main.cpp
#include "qtpclviewer.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QtPclViewer w;
w.show();
return a.exec();
}
qtpclviewer.cpp
#include "qtpclviewer.h"
#include "ui_qtpclviewer.h"
QtPclViewer::QtPclViewer(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::QtPclViewer)
{
ui->setupUi(this);
initialVtkWidget();
onOpen();
connect(ui->horizontalSlider_Size, SIGNAL(valueChanged(int)), this, SLOT(pSliderValueChangeed(int)));
connect(ui->horizontalSlider_Red, SIGNAL(valueChanged(int)), this, SLOT(redSliderValueChangeed(int)));
connect(ui->horizontalSlider_Green, SIGNAL(valueChanged(int)), this, SLOT(greenSliderValueChangeed(int)));
connect(ui->horizontalSlider_Blue, SIGNAL(valueChanged(int)), this, SLOT(blueSliderValueChangeed(int)));
connect(ui->horizontalSlider_Red, SIGNAL(sliderReleased( )), this, SLOT(rgbSliderReleased( )));
connect(ui->horizontalSlider_Green, SIGNAL(sliderReleased( )), this, SLOT(rgbSliderReleased( )));
connect(ui->horizontalSlider_Blue, SIGNAL(sliderReleased( )), this, SLOT(rgbSliderReleased( )));
}
QtPclViewer::~QtPclViewer()
{
delete ui;
}
void QtPclViewer::initialVtkWidget()
{
red = 255;
green = 255;
blue = 255;
size = 1.0;
cloud.reset(new pcl::PointCloud<pcl::PointXYZ>);
viewer.reset(new pcl::visualization::PCLVisualizer("viewer", false));
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> single_color(cloud, red, green, blue);
viewer->addPointCloud<pcl::PointXYZ>(cloud, single_color, "cloud");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, size, "cloud");
ui->qvtkWidget->SetRenderWindow(viewer->getRenderWindow());
viewer->setupInteractor(ui->qvtkWidget->GetInteractor(), ui->qvtkWidget->GetRenderWindow());
ui->qvtkWidget->update();
}
void QtPclViewer::onOpen()
{
QString fileName = QFileDialog::getOpenFileName(this, "Open PointCloud", ".", "Open PCD files(*.pcd)");
if (!fileName.isEmpty())
{
std::string file_name = fileName.toStdString();
pcl::PCLPointCloud2 cloud2;
Eigen::Vector4f origin;
Eigen::Quaternionf orientation;
int pcd_version;
int data_type;
unsigned int data_idx;
int offset = 0;
pcl::PCDReader rd;
rd.readHeader(file_name, cloud2, origin, orientation, pcd_version, data_type, data_idx);
if (data_type == 0)
{
pcl::io::loadPCDFile(fileName.toStdString(),*cloud);
}
else if (data_type == 2)
{
pcl::PCDReader reader;
reader.read<pcl::PointXYZ>(fileName.toStdString(), *cloud);
}
qDebug("cloud->points.size: %ld", cloud->points.size());
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> single_color(cloud, red, green, blue);
viewer->updatePointCloud<pcl::PointXYZ>(cloud, single_color, "cloud");
viewer->resetCamera();
ui->qvtkWidget->update();
}
}
void QtPclViewer::rgbSliderReleased()
{
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> single_color(cloud, red, green, blue);
viewer->updatePointCloud<pcl::PointXYZ>(cloud, single_color, "cloud");
ui->qvtkWidget->update();
}
void QtPclViewer::redSliderValueChangeed(int value)
{
red= value;
}
void QtPclViewer::greenSliderValueChangeed(int value)
{
green= value;
}
void QtPclViewer::blueSliderValueChangeed(int value)
{
blue= value;
}
void QtPclViewer::pSliderValueChangeed(int value)
{
size=double(10+value)/10;
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, size, "cloud");
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ>single_color(cloud, red, green, blue);
viewer->updatePointCloud<pcl::PointXYZ>(cloud,single_color, "cloud");
ui->qvtkWidget->update();
}
|